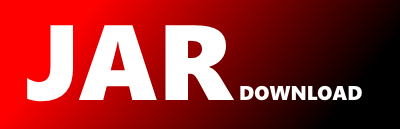
com.pulumi.gcp.networkservices.kotlin.GrpcRouteArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkservices.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.networkservices.GrpcRouteArgs.builder
import com.pulumi.gcp.networkservices.kotlin.inputs.GrpcRouteRuleArgs
import com.pulumi.gcp.networkservices.kotlin.inputs.GrpcRouteRuleArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* ## Example Usage
* ### Network Services Grpc Route Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.networkservices.GrpcRoute("default", {
* name: "my-grpc-route",
* labels: {
* foo: "bar",
* },
* description: "my description",
* hostnames: ["example"],
* rules: [{
* matches: [{
* headers: [{
* key: "key",
* value: "value",
* }],
* }],
* action: {
* retryPolicy: {
* retryConditions: ["cancelled"],
* numRetries: 1,
* },
* },
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.networkservices.GrpcRoute("default",
* name="my-grpc-route",
* labels={
* "foo": "bar",
* },
* description="my description",
* hostnames=["example"],
* rules=[gcp.networkservices.GrpcRouteRuleArgs(
* matches=[gcp.networkservices.GrpcRouteRuleMatchArgs(
* headers=[gcp.networkservices.GrpcRouteRuleMatchHeaderArgs(
* key="key",
* value="value",
* )],
* )],
* action=gcp.networkservices.GrpcRouteRuleActionArgs(
* retry_policy=gcp.networkservices.GrpcRouteRuleActionRetryPolicyArgs(
* retry_conditions=["cancelled"],
* num_retries=1,
* ),
* ),
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.NetworkServices.GrpcRoute("default", new()
* {
* Name = "my-grpc-route",
* Labels =
* {
* { "foo", "bar" },
* },
* Description = "my description",
* Hostnames = new[]
* {
* "example",
* },
* Rules = new[]
* {
* new Gcp.NetworkServices.Inputs.GrpcRouteRuleArgs
* {
* Matches = new[]
* {
* new Gcp.NetworkServices.Inputs.GrpcRouteRuleMatchArgs
* {
* Headers = new[]
* {
* new Gcp.NetworkServices.Inputs.GrpcRouteRuleMatchHeaderArgs
* {
* Key = "key",
* Value = "value",
* },
* },
* },
* },
* Action = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionArgs
* {
* RetryPolicy = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionRetryPolicyArgs
* {
* RetryConditions = new[]
* {
* "cancelled",
* },
* NumRetries = 1,
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/networkservices"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := networkservices.NewGrpcRoute(ctx, "default", &networkservices.GrpcRouteArgs{
* Name: pulumi.String("my-grpc-route"),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Description: pulumi.String("my description"),
* Hostnames: pulumi.StringArray{
* pulumi.String("example"),
* },
* Rules: networkservices.GrpcRouteRuleArray{
* &networkservices.GrpcRouteRuleArgs{
* Matches: networkservices.GrpcRouteRuleMatchArray{
* &networkservices.GrpcRouteRuleMatchArgs{
* Headers: networkservices.GrpcRouteRuleMatchHeaderArray{
* &networkservices.GrpcRouteRuleMatchHeaderArgs{
* Key: pulumi.String("key"),
* Value: pulumi.String("value"),
* },
* },
* },
* },
* Action: &networkservices.GrpcRouteRuleActionArgs{
* RetryPolicy: &networkservices.GrpcRouteRuleActionRetryPolicyArgs{
* RetryConditions: pulumi.StringArray{
* pulumi.String("cancelled"),
* },
* NumRetries: pulumi.Int(1),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.networkservices.GrpcRoute;
* import com.pulumi.gcp.networkservices.GrpcRouteArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionRetryPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new GrpcRoute("default", GrpcRouteArgs.builder()
* .name("my-grpc-route")
* .labels(Map.of("foo", "bar"))
* .description("my description")
* .hostnames("example")
* .rules(GrpcRouteRuleArgs.builder()
* .matches(GrpcRouteRuleMatchArgs.builder()
* .headers(GrpcRouteRuleMatchHeaderArgs.builder()
* .key("key")
* .value("value")
* .build())
* .build())
* .action(GrpcRouteRuleActionArgs.builder()
* .retryPolicy(GrpcRouteRuleActionRetryPolicyArgs.builder()
* .retryConditions("cancelled")
* .numRetries(1)
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:networkservices:GrpcRoute
* properties:
* name: my-grpc-route
* labels:
* foo: bar
* description: my description
* hostnames:
* - example
* rules:
* - matches:
* - headers:
* - key: key
* value: value
* action:
* retryPolicy:
* retryConditions:
* - cancelled
* numRetries: 1
* ```
*
* ### Network Services Grpc Route Matches And Actions
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.networkservices.GrpcRoute("default", {
* name: "my-grpc-route",
* labels: {
* foo: "bar",
* },
* description: "my description",
* hostnames: ["example"],
* rules: [{
* matches: [
* {
* headers: [{
* key: "key",
* value: "value",
* }],
* },
* {
* headers: [{
* key: "key",
* value: "value",
* }],
* method: {
* grpcService: "foo",
* grpcMethod: "bar",
* caseSensitive: true,
* },
* },
* ],
* action: {
* faultInjectionPolicy: {
* delay: {
* fixedDelay: "1s",
* percentage: 1,
* },
* abort: {
* httpStatus: 500,
* percentage: 1,
* },
* },
* retryPolicy: {
* retryConditions: ["cancelled"],
* numRetries: 1,
* },
* },
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.networkservices.GrpcRoute("default",
* name="my-grpc-route",
* labels={
* "foo": "bar",
* },
* description="my description",
* hostnames=["example"],
* rules=[gcp.networkservices.GrpcRouteRuleArgs(
* matches=[
* gcp.networkservices.GrpcRouteRuleMatchArgs(
* headers=[gcp.networkservices.GrpcRouteRuleMatchHeaderArgs(
* key="key",
* value="value",
* )],
* ),
* gcp.networkservices.GrpcRouteRuleMatchArgs(
* headers=[gcp.networkservices.GrpcRouteRuleMatchHeaderArgs(
* key="key",
* value="value",
* )],
* method=gcp.networkservices.GrpcRouteRuleMatchMethodArgs(
* grpc_service="foo",
* grpc_method="bar",
* case_sensitive=True,
* ),
* ),
* ],
* action=gcp.networkservices.GrpcRouteRuleActionArgs(
* fault_injection_policy=gcp.networkservices.GrpcRouteRuleActionFaultInjectionPolicyArgs(
* delay=gcp.networkservices.GrpcRouteRuleActionFaultInjectionPolicyDelayArgs(
* fixed_delay="1s",
* percentage=1,
* ),
* abort=gcp.networkservices.GrpcRouteRuleActionFaultInjectionPolicyAbortArgs(
* http_status=500,
* percentage=1,
* ),
* ),
* retry_policy=gcp.networkservices.GrpcRouteRuleActionRetryPolicyArgs(
* retry_conditions=["cancelled"],
* num_retries=1,
* ),
* ),
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.NetworkServices.GrpcRoute("default", new()
* {
* Name = "my-grpc-route",
* Labels =
* {
* { "foo", "bar" },
* },
* Description = "my description",
* Hostnames = new[]
* {
* "example",
* },
* Rules = new[]
* {
* new Gcp.NetworkServices.Inputs.GrpcRouteRuleArgs
* {
* Matches = new[]
* {
* new Gcp.NetworkServices.Inputs.GrpcRouteRuleMatchArgs
* {
* Headers = new[]
* {
* new Gcp.NetworkServices.Inputs.GrpcRouteRuleMatchHeaderArgs
* {
* Key = "key",
* Value = "value",
* },
* },
* },
* new Gcp.NetworkServices.Inputs.GrpcRouteRuleMatchArgs
* {
* Headers = new[]
* {
* new Gcp.NetworkServices.Inputs.GrpcRouteRuleMatchHeaderArgs
* {
* Key = "key",
* Value = "value",
* },
* },
* Method = new Gcp.NetworkServices.Inputs.GrpcRouteRuleMatchMethodArgs
* {
* GrpcService = "foo",
* GrpcMethod = "bar",
* CaseSensitive = true,
* },
* },
* },
* Action = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionArgs
* {
* FaultInjectionPolicy = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionFaultInjectionPolicyArgs
* {
* Delay = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionFaultInjectionPolicyDelayArgs
* {
* FixedDelay = "1s",
* Percentage = 1,
* },
* Abort = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionFaultInjectionPolicyAbortArgs
* {
* HttpStatus = 500,
* Percentage = 1,
* },
* },
* RetryPolicy = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionRetryPolicyArgs
* {
* RetryConditions = new[]
* {
* "cancelled",
* },
* NumRetries = 1,
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/networkservices"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := networkservices.NewGrpcRoute(ctx, "default", &networkservices.GrpcRouteArgs{
* Name: pulumi.String("my-grpc-route"),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Description: pulumi.String("my description"),
* Hostnames: pulumi.StringArray{
* pulumi.String("example"),
* },
* Rules: networkservices.GrpcRouteRuleArray{
* &networkservices.GrpcRouteRuleArgs{
* Matches: networkservices.GrpcRouteRuleMatchArray{
* &networkservices.GrpcRouteRuleMatchArgs{
* Headers: networkservices.GrpcRouteRuleMatchHeaderArray{
* &networkservices.GrpcRouteRuleMatchHeaderArgs{
* Key: pulumi.String("key"),
* Value: pulumi.String("value"),
* },
* },
* },
* &networkservices.GrpcRouteRuleMatchArgs{
* Headers: networkservices.GrpcRouteRuleMatchHeaderArray{
* &networkservices.GrpcRouteRuleMatchHeaderArgs{
* Key: pulumi.String("key"),
* Value: pulumi.String("value"),
* },
* },
* Method: &networkservices.GrpcRouteRuleMatchMethodArgs{
* GrpcService: pulumi.String("foo"),
* GrpcMethod: pulumi.String("bar"),
* CaseSensitive: pulumi.Bool(true),
* },
* },
* },
* Action: &networkservices.GrpcRouteRuleActionArgs{
* FaultInjectionPolicy: &networkservices.GrpcRouteRuleActionFaultInjectionPolicyArgs{
* Delay: &networkservices.GrpcRouteRuleActionFaultInjectionPolicyDelayArgs{
* FixedDelay: pulumi.String("1s"),
* Percentage: pulumi.Int(1),
* },
* Abort: &networkservices.GrpcRouteRuleActionFaultInjectionPolicyAbortArgs{
* HttpStatus: pulumi.Int(500),
* Percentage: pulumi.Int(1),
* },
* },
* RetryPolicy: &networkservices.GrpcRouteRuleActionRetryPolicyArgs{
* RetryConditions: pulumi.StringArray{
* pulumi.String("cancelled"),
* },
* NumRetries: pulumi.Int(1),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.networkservices.GrpcRoute;
* import com.pulumi.gcp.networkservices.GrpcRouteArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionFaultInjectionPolicyArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionFaultInjectionPolicyDelayArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionFaultInjectionPolicyAbortArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionRetryPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new GrpcRoute("default", GrpcRouteArgs.builder()
* .name("my-grpc-route")
* .labels(Map.of("foo", "bar"))
* .description("my description")
* .hostnames("example")
* .rules(GrpcRouteRuleArgs.builder()
* .matches(
* GrpcRouteRuleMatchArgs.builder()
* .headers(GrpcRouteRuleMatchHeaderArgs.builder()
* .key("key")
* .value("value")
* .build())
* .build(),
* GrpcRouteRuleMatchArgs.builder()
* .headers(GrpcRouteRuleMatchHeaderArgs.builder()
* .key("key")
* .value("value")
* .build())
* .method(GrpcRouteRuleMatchMethodArgs.builder()
* .grpcService("foo")
* .grpcMethod("bar")
* .caseSensitive(true)
* .build())
* .build())
* .action(GrpcRouteRuleActionArgs.builder()
* .faultInjectionPolicy(GrpcRouteRuleActionFaultInjectionPolicyArgs.builder()
* .delay(GrpcRouteRuleActionFaultInjectionPolicyDelayArgs.builder()
* .fixedDelay("1s")
* .percentage(1)
* .build())
* .abort(GrpcRouteRuleActionFaultInjectionPolicyAbortArgs.builder()
* .httpStatus(500)
* .percentage(1)
* .build())
* .build())
* .retryPolicy(GrpcRouteRuleActionRetryPolicyArgs.builder()
* .retryConditions("cancelled")
* .numRetries(1)
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:networkservices:GrpcRoute
* properties:
* name: my-grpc-route
* labels:
* foo: bar
* description: my description
* hostnames:
* - example
* rules:
* - matches:
* - headers:
* - key: key
* value: value
* - headers:
* - key: key
* value: value
* method:
* grpcService: foo
* grpcMethod: bar
* caseSensitive: true
* action:
* faultInjectionPolicy:
* delay:
* fixedDelay: 1s
* percentage: 1
* abort:
* httpStatus: 500
* percentage: 1
* retryPolicy:
* retryConditions:
* - cancelled
* numRetries: 1
* ```
*
* ### Network Services Grpc Route Actions
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.networkservices.GrpcRoute("default", {
* name: "my-grpc-route",
* labels: {
* foo: "bar",
* },
* description: "my description",
* hostnames: ["example"],
* rules: [{
* action: {
* faultInjectionPolicy: {
* delay: {
* fixedDelay: "1s",
* percentage: 1,
* },
* abort: {
* httpStatus: 500,
* percentage: 1,
* },
* },
* retryPolicy: {
* retryConditions: ["cancelled"],
* numRetries: 1,
* },
* },
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.networkservices.GrpcRoute("default",
* name="my-grpc-route",
* labels={
* "foo": "bar",
* },
* description="my description",
* hostnames=["example"],
* rules=[gcp.networkservices.GrpcRouteRuleArgs(
* action=gcp.networkservices.GrpcRouteRuleActionArgs(
* fault_injection_policy=gcp.networkservices.GrpcRouteRuleActionFaultInjectionPolicyArgs(
* delay=gcp.networkservices.GrpcRouteRuleActionFaultInjectionPolicyDelayArgs(
* fixed_delay="1s",
* percentage=1,
* ),
* abort=gcp.networkservices.GrpcRouteRuleActionFaultInjectionPolicyAbortArgs(
* http_status=500,
* percentage=1,
* ),
* ),
* retry_policy=gcp.networkservices.GrpcRouteRuleActionRetryPolicyArgs(
* retry_conditions=["cancelled"],
* num_retries=1,
* ),
* ),
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.NetworkServices.GrpcRoute("default", new()
* {
* Name = "my-grpc-route",
* Labels =
* {
* { "foo", "bar" },
* },
* Description = "my description",
* Hostnames = new[]
* {
* "example",
* },
* Rules = new[]
* {
* new Gcp.NetworkServices.Inputs.GrpcRouteRuleArgs
* {
* Action = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionArgs
* {
* FaultInjectionPolicy = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionFaultInjectionPolicyArgs
* {
* Delay = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionFaultInjectionPolicyDelayArgs
* {
* FixedDelay = "1s",
* Percentage = 1,
* },
* Abort = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionFaultInjectionPolicyAbortArgs
* {
* HttpStatus = 500,
* Percentage = 1,
* },
* },
* RetryPolicy = new Gcp.NetworkServices.Inputs.GrpcRouteRuleActionRetryPolicyArgs
* {
* RetryConditions = new[]
* {
* "cancelled",
* },
* NumRetries = 1,
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/networkservices"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := networkservices.NewGrpcRoute(ctx, "default", &networkservices.GrpcRouteArgs{
* Name: pulumi.String("my-grpc-route"),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Description: pulumi.String("my description"),
* Hostnames: pulumi.StringArray{
* pulumi.String("example"),
* },
* Rules: networkservices.GrpcRouteRuleArray{
* &networkservices.GrpcRouteRuleArgs{
* Action: &networkservices.GrpcRouteRuleActionArgs{
* FaultInjectionPolicy: &networkservices.GrpcRouteRuleActionFaultInjectionPolicyArgs{
* Delay: &networkservices.GrpcRouteRuleActionFaultInjectionPolicyDelayArgs{
* FixedDelay: pulumi.String("1s"),
* Percentage: pulumi.Int(1),
* },
* Abort: &networkservices.GrpcRouteRuleActionFaultInjectionPolicyAbortArgs{
* HttpStatus: pulumi.Int(500),
* Percentage: pulumi.Int(1),
* },
* },
* RetryPolicy: &networkservices.GrpcRouteRuleActionRetryPolicyArgs{
* RetryConditions: pulumi.StringArray{
* pulumi.String("cancelled"),
* },
* NumRetries: pulumi.Int(1),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.networkservices.GrpcRoute;
* import com.pulumi.gcp.networkservices.GrpcRouteArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionFaultInjectionPolicyArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionFaultInjectionPolicyDelayArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionFaultInjectionPolicyAbortArgs;
* import com.pulumi.gcp.networkservices.inputs.GrpcRouteRuleActionRetryPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new GrpcRoute("default", GrpcRouteArgs.builder()
* .name("my-grpc-route")
* .labels(Map.of("foo", "bar"))
* .description("my description")
* .hostnames("example")
* .rules(GrpcRouteRuleArgs.builder()
* .action(GrpcRouteRuleActionArgs.builder()
* .faultInjectionPolicy(GrpcRouteRuleActionFaultInjectionPolicyArgs.builder()
* .delay(GrpcRouteRuleActionFaultInjectionPolicyDelayArgs.builder()
* .fixedDelay("1s")
* .percentage(1)
* .build())
* .abort(GrpcRouteRuleActionFaultInjectionPolicyAbortArgs.builder()
* .httpStatus(500)
* .percentage(1)
* .build())
* .build())
* .retryPolicy(GrpcRouteRuleActionRetryPolicyArgs.builder()
* .retryConditions("cancelled")
* .numRetries(1)
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:networkservices:GrpcRoute
* properties:
* name: my-grpc-route
* labels:
* foo: bar
* description: my description
* hostnames:
* - example
* rules:
* - action:
* faultInjectionPolicy:
* delay:
* fixedDelay: 1s
* percentage: 1
* abort:
* httpStatus: 500
* percentage: 1
* retryPolicy:
* retryConditions:
* - cancelled
* numRetries: 1
* ```
*
* ## Import
* GrpcRoute can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/global/grpcRoutes/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, GrpcRoute can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:networkservices/grpcRoute:GrpcRoute default projects/{{project}}/locations/global/grpcRoutes/{{name}}
* ```
* ```sh
* $ pulumi import gcp:networkservices/grpcRoute:GrpcRoute default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:networkservices/grpcRoute:GrpcRoute default {{name}}
* ```
* @property description A free-text description of the resource. Max length 1024 characters.
* @property gateways List of gateways this GrpcRoute is attached to, as one of the routing rules to route the requests served by the gateway.
* @property hostnames Required. Service hostnames with an optional port for which this route describes traffic.
* @property labels Set of label tags associated with the GrpcRoute resource. **Note**: This field is non-authoritative, and will only
* manage the labels present in your configuration. Please refer to the field 'effective_labels' for all of the labels
* present on the resource.
* @property meshes List of meshes this GrpcRoute is attached to, as one of the routing rules to route the requests served by the mesh.
* @property name Name of the GrpcRoute resource.
* @property project
* @property rules Rules that define how traffic is routed and handled.
* Structure is documented below.
*/
public data class GrpcRouteArgs(
public val description: Output? = null,
public val gateways: Output>? = null,
public val hostnames: Output>? = null,
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy