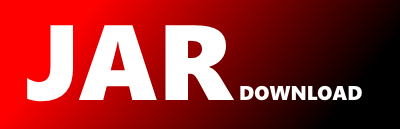
com.pulumi.gcp.networkservices.kotlin.inputs.EdgeCacheOriginTimeoutArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkservices.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.networkservices.inputs.EdgeCacheOriginTimeoutArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property connectTimeout The maximum duration to wait for a single origin connection to be established, including DNS lookup, TLS handshake and TCP/QUIC connection establishment.
* Defaults to 5 seconds. The timeout must be a value between 1s and 15s.
* The connectTimeout capped by the deadline set by the request's maxAttemptsTimeout. The last connection attempt may have a smaller connectTimeout in order to adhere to the overall maxAttemptsTimeout.
* @property maxAttemptsTimeout The maximum time across all connection attempts to the origin, including failover origins, before returning an error to the client. A HTTP 504 will be returned if the timeout is reached before a response is returned.
* Defaults to 15 seconds. The timeout must be a value between 1s and 30s.
* If a failoverOrigin is specified, the maxAttemptsTimeout of the first configured origin sets the deadline for all connection attempts across all failoverOrigins.
* @property readTimeout The maximum duration to wait between reads of a single HTTP connection/stream.
* Defaults to 15 seconds. The timeout must be a value between 1s and 30s.
* The readTimeout is capped by the responseTimeout. All reads of the HTTP connection/stream must be completed by the deadline set by the responseTimeout.
* If the response headers have already been written to the connection, the response will be truncated and logged.
* The `aws_v4_authentication` block supports:
* @property responseTimeout The maximum duration to wait for the last byte of a response to arrive when reading from the HTTP connection/stream.
* Defaults to 30 seconds. The timeout must be a value between 1s and 120s.
* The responseTimeout starts after the connection has been established.
* This also applies to HTTP Chunked Transfer Encoding responses, and/or when an open-ended Range request is made to the origin. Origins that take longer to write additional bytes to the response than the configured responseTimeout will result in an error being returned to the client.
* If the response headers have already been written to the connection, the response will be truncated and logged.
*/
public data class EdgeCacheOriginTimeoutArgs(
public val connectTimeout: Output? = null,
public val maxAttemptsTimeout: Output? = null,
public val readTimeout: Output? = null,
public val responseTimeout: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.networkservices.inputs.EdgeCacheOriginTimeoutArgs =
com.pulumi.gcp.networkservices.inputs.EdgeCacheOriginTimeoutArgs.builder()
.connectTimeout(connectTimeout?.applyValue({ args0 -> args0 }))
.maxAttemptsTimeout(maxAttemptsTimeout?.applyValue({ args0 -> args0 }))
.readTimeout(readTimeout?.applyValue({ args0 -> args0 }))
.responseTimeout(responseTimeout?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EdgeCacheOriginTimeoutArgs].
*/
@PulumiTagMarker
public class EdgeCacheOriginTimeoutArgsBuilder internal constructor() {
private var connectTimeout: Output? = null
private var maxAttemptsTimeout: Output? = null
private var readTimeout: Output? = null
private var responseTimeout: Output? = null
/**
* @param value The maximum duration to wait for a single origin connection to be established, including DNS lookup, TLS handshake and TCP/QUIC connection establishment.
* Defaults to 5 seconds. The timeout must be a value between 1s and 15s.
* The connectTimeout capped by the deadline set by the request's maxAttemptsTimeout. The last connection attempt may have a smaller connectTimeout in order to adhere to the overall maxAttemptsTimeout.
*/
@JvmName("vfqedqjugniimtue")
public suspend fun connectTimeout(`value`: Output) {
this.connectTimeout = value
}
/**
* @param value The maximum time across all connection attempts to the origin, including failover origins, before returning an error to the client. A HTTP 504 will be returned if the timeout is reached before a response is returned.
* Defaults to 15 seconds. The timeout must be a value between 1s and 30s.
* If a failoverOrigin is specified, the maxAttemptsTimeout of the first configured origin sets the deadline for all connection attempts across all failoverOrigins.
*/
@JvmName("giwisknubveoejqv")
public suspend fun maxAttemptsTimeout(`value`: Output) {
this.maxAttemptsTimeout = value
}
/**
* @param value The maximum duration to wait between reads of a single HTTP connection/stream.
* Defaults to 15 seconds. The timeout must be a value between 1s and 30s.
* The readTimeout is capped by the responseTimeout. All reads of the HTTP connection/stream must be completed by the deadline set by the responseTimeout.
* If the response headers have already been written to the connection, the response will be truncated and logged.
* The `aws_v4_authentication` block supports:
*/
@JvmName("qfuffckybhcycsxj")
public suspend fun readTimeout(`value`: Output) {
this.readTimeout = value
}
/**
* @param value The maximum duration to wait for the last byte of a response to arrive when reading from the HTTP connection/stream.
* Defaults to 30 seconds. The timeout must be a value between 1s and 120s.
* The responseTimeout starts after the connection has been established.
* This also applies to HTTP Chunked Transfer Encoding responses, and/or when an open-ended Range request is made to the origin. Origins that take longer to write additional bytes to the response than the configured responseTimeout will result in an error being returned to the client.
* If the response headers have already been written to the connection, the response will be truncated and logged.
*/
@JvmName("syeqsoiebkswxgpa")
public suspend fun responseTimeout(`value`: Output) {
this.responseTimeout = value
}
/**
* @param value The maximum duration to wait for a single origin connection to be established, including DNS lookup, TLS handshake and TCP/QUIC connection establishment.
* Defaults to 5 seconds. The timeout must be a value between 1s and 15s.
* The connectTimeout capped by the deadline set by the request's maxAttemptsTimeout. The last connection attempt may have a smaller connectTimeout in order to adhere to the overall maxAttemptsTimeout.
*/
@JvmName("wplulipjrojwvxxg")
public suspend fun connectTimeout(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectTimeout = mapped
}
/**
* @param value The maximum time across all connection attempts to the origin, including failover origins, before returning an error to the client. A HTTP 504 will be returned if the timeout is reached before a response is returned.
* Defaults to 15 seconds. The timeout must be a value between 1s and 30s.
* If a failoverOrigin is specified, the maxAttemptsTimeout of the first configured origin sets the deadline for all connection attempts across all failoverOrigins.
*/
@JvmName("demdnqawxryplfum")
public suspend fun maxAttemptsTimeout(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxAttemptsTimeout = mapped
}
/**
* @param value The maximum duration to wait between reads of a single HTTP connection/stream.
* Defaults to 15 seconds. The timeout must be a value between 1s and 30s.
* The readTimeout is capped by the responseTimeout. All reads of the HTTP connection/stream must be completed by the deadline set by the responseTimeout.
* If the response headers have already been written to the connection, the response will be truncated and logged.
* The `aws_v4_authentication` block supports:
*/
@JvmName("eqsijcajajjaofpa")
public suspend fun readTimeout(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.readTimeout = mapped
}
/**
* @param value The maximum duration to wait for the last byte of a response to arrive when reading from the HTTP connection/stream.
* Defaults to 30 seconds. The timeout must be a value between 1s and 120s.
* The responseTimeout starts after the connection has been established.
* This also applies to HTTP Chunked Transfer Encoding responses, and/or when an open-ended Range request is made to the origin. Origins that take longer to write additional bytes to the response than the configured responseTimeout will result in an error being returned to the client.
* If the response headers have already been written to the connection, the response will be truncated and logged.
*/
@JvmName("gxgctteklnegshnx")
public suspend fun responseTimeout(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.responseTimeout = mapped
}
internal fun build(): EdgeCacheOriginTimeoutArgs = EdgeCacheOriginTimeoutArgs(
connectTimeout = connectTimeout,
maxAttemptsTimeout = maxAttemptsTimeout,
readTimeout = readTimeout,
responseTimeout = responseTimeout,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy