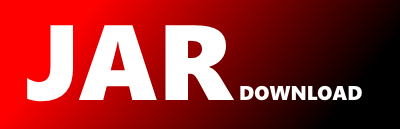
com.pulumi.gcp.networkservices.kotlin.inputs.EdgeCacheServiceRoutingPathMatcherRouteRuleHeaderActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkservices.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.networkservices.inputs.EdgeCacheServiceRoutingPathMatcherRouteRuleHeaderActionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property requestHeaderToAdds Describes a header to add.
* Structure is documented below.
* @property requestHeaderToRemoves A list of header names for headers that need to be removed from the request prior to forwarding the request to the origin.
* Structure is documented below.
* @property responseHeaderToAdds Headers to add to the response prior to sending it back to the client.
* Response headers are only sent to the client, and do not have an effect on the cache serving the response.
* Structure is documented below.
* @property responseHeaderToRemoves A list of header names for headers that need to be removed from the request prior to forwarding the request to the origin.
* Structure is documented below.
*/
public data class EdgeCacheServiceRoutingPathMatcherRouteRuleHeaderActionArgs(
public val requestHeaderToAdds: Output>? =
null,
public val requestHeaderToRemoves: Output>? =
null,
public val responseHeaderToAdds: Output>? =
null,
public val responseHeaderToRemoves: Output>? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.networkservices.inputs.EdgeCacheServiceRoutingPathMatcherRouteRuleHeaderActionArgs =
com.pulumi.gcp.networkservices.inputs.EdgeCacheServiceRoutingPathMatcherRouteRuleHeaderActionArgs.builder()
.requestHeaderToAdds(
requestHeaderToAdds?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.requestHeaderToRemoves(
requestHeaderToRemoves?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.responseHeaderToAdds(
responseHeaderToAdds?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.responseHeaderToRemoves(
responseHeaderToRemoves?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [EdgeCacheServiceRoutingPathMatcherRouteRuleHeaderActionArgs].
*/
@PulumiTagMarker
public class EdgeCacheServiceRoutingPathMatcherRouteRuleHeaderActionArgsBuilder internal constructor() {
private var requestHeaderToAdds:
Output>? =
null
private var requestHeaderToRemoves:
Output>? =
null
private var responseHeaderToAdds:
Output>? =
null
private var responseHeaderToRemoves:
Output>? =
null
/**
* @param value Describes a header to add.
* Structure is documented below.
*/
@JvmName("cccffhqisnanqilt")
public suspend fun requestHeaderToAdds(`value`: Output>) {
this.requestHeaderToAdds = value
}
@JvmName("ytwqrggoglkklrej")
public suspend fun requestHeaderToAdds(vararg values: Output) {
this.requestHeaderToAdds = Output.all(values.asList())
}
/**
* @param values Describes a header to add.
* Structure is documented below.
*/
@JvmName("xtvcvqqittltrovg")
public suspend fun requestHeaderToAdds(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy