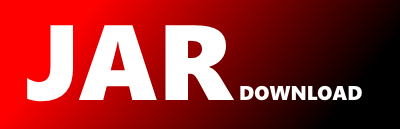
com.pulumi.gcp.networkservices.kotlin.inputs.EdgeCacheServiceRoutingPathMatcherRouteRuleRouteActionCdnPolicyAddSignaturesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkservices.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.networkservices.inputs.EdgeCacheServiceRoutingPathMatcherRouteRuleRouteActionCdnPolicyAddSignaturesArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property actions The actions to take to add signatures to responses.
* Each value may be one of: `GENERATE_COOKIE`, `GENERATE_TOKEN_HLS_COOKIELESS`, `PROPAGATE_TOKEN_HLS_COOKIELESS`.
* @property copiedParameters The parameters to copy from the verified token to the generated token.
* Only the following parameters may be copied:
* * `PathGlobs`
* @property keyset The keyset to use for signature generation.
* The following are both valid paths to an EdgeCacheKeyset resource:
* * `projects/project/locations/global/edgeCacheKeysets/yourKeyset`
* * `yourKeyset`
* This must be specified when the GENERATE_COOKIE or GENERATE_TOKEN_HLS_COOKIELESS actions are specified. This field may not be specified otherwise.
* @property tokenQueryParameter The query parameter in which to put the generated token.
* If not specified, defaults to `edge-cache-token`.
* If specified, the name must be 1-64 characters long and match the regular expression `a-zA-Z*` which means the first character must be a letter, and all following characters must be a dash, underscore, letter or digit.
* This field may only be set when the GENERATE_TOKEN_HLS_COOKIELESS or PROPAGATE_TOKEN_HLS_COOKIELESS actions are specified.
* @property tokenTtl The duration the token is valid starting from the moment the token is first generated.
* Defaults to `86400s` (1 day).
* The TTL must be >= 0 and <= 604,800 seconds (1 week).
* This field may only be specified when the GENERATE_COOKIE or GENERATE_TOKEN_HLS_COOKIELESS actions are specified.
* A duration in seconds with up to nine fractional digits, terminated by 's'. Example: "3.5s".
*/
public data class EdgeCacheServiceRoutingPathMatcherRouteRuleRouteActionCdnPolicyAddSignaturesArgs(
public val actions: Output,
public val copiedParameters: Output>? = null,
public val keyset: Output? = null,
public val tokenQueryParameter: Output? = null,
public val tokenTtl: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.networkservices.inputs.EdgeCacheServiceRoutingPathMatcherRouteRuleRouteActionCdnPolicyAddSignaturesArgs =
com.pulumi.gcp.networkservices.inputs.EdgeCacheServiceRoutingPathMatcherRouteRuleRouteActionCdnPolicyAddSignaturesArgs.builder()
.actions(actions.applyValue({ args0 -> args0 }))
.copiedParameters(copiedParameters?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.keyset(keyset?.applyValue({ args0 -> args0 }))
.tokenQueryParameter(tokenQueryParameter?.applyValue({ args0 -> args0 }))
.tokenTtl(tokenTtl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EdgeCacheServiceRoutingPathMatcherRouteRuleRouteActionCdnPolicyAddSignaturesArgs].
*/
@PulumiTagMarker
public class EdgeCacheServiceRoutingPathMatcherRouteRuleRouteActionCdnPolicyAddSignaturesArgsBuilder
internal constructor() {
private var actions: Output? = null
private var copiedParameters: Output>? = null
private var keyset: Output? = null
private var tokenQueryParameter: Output? = null
private var tokenTtl: Output? = null
/**
* @param value The actions to take to add signatures to responses.
* Each value may be one of: `GENERATE_COOKIE`, `GENERATE_TOKEN_HLS_COOKIELESS`, `PROPAGATE_TOKEN_HLS_COOKIELESS`.
*/
@JvmName("bmgmcdodxddgapbc")
public suspend fun actions(`value`: Output) {
this.actions = value
}
/**
* @param value The parameters to copy from the verified token to the generated token.
* Only the following parameters may be copied:
* * `PathGlobs`
*/
@JvmName("fxxyholhtssvtgbb")
public suspend fun copiedParameters(`value`: Output>) {
this.copiedParameters = value
}
@JvmName("myomaofhxyuqiweh")
public suspend fun copiedParameters(vararg values: Output) {
this.copiedParameters = Output.all(values.asList())
}
/**
* @param values The parameters to copy from the verified token to the generated token.
* Only the following parameters may be copied:
* * `PathGlobs`
*/
@JvmName("qxlgweuwwedukmjj")
public suspend fun copiedParameters(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy