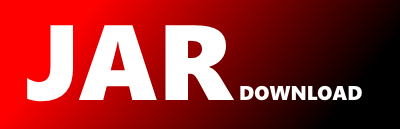
com.pulumi.gcp.networkservices.kotlin.inputs.HttpRouteRuleActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkservices.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.networkservices.inputs.HttpRouteRuleActionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property corsPolicy The specification for allowing client side cross-origin requests.
* Structure is documented below.
* @property destinations The destination to which traffic should be forwarded.
* Structure is documented below.
* @property faultInjectionPolicy The specification for fault injection introduced into traffic to test the resiliency of clients to backend service failure.
* Structure is documented below.
* @property redirect If set, the request is directed as configured by this field.
* Structure is documented below.
* @property requestHeaderModifier The specification for modifying the headers of a matching request prior to delivery of the request to the destination.
* Structure is documented below.
* @property requestMirrorPolicy Specifies the policy on how requests intended for the routes destination are shadowed to a separate mirrored destination.
* Structure is documented below.
* @property responseHeaderModifier The specification for modifying the headers of a response prior to sending the response back to the client.
* Structure is documented below.
* @property retryPolicy Specifies the retry policy associated with this route.
* Structure is documented below.
* @property timeout Specifies the timeout for selected route.
* @property urlRewrite The specification for rewrite URL before forwarding requests to the destination.
* Structure is documented below.
*/
public data class HttpRouteRuleActionArgs(
public val corsPolicy: Output? = null,
public val destinations: Output>? = null,
public val faultInjectionPolicy: Output? = null,
public val redirect: Output? = null,
public val requestHeaderModifier: Output? = null,
public val requestMirrorPolicy: Output? = null,
public val responseHeaderModifier: Output? = null,
public val retryPolicy: Output? = null,
public val timeout: Output? = null,
public val urlRewrite: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.networkservices.inputs.HttpRouteRuleActionArgs =
com.pulumi.gcp.networkservices.inputs.HttpRouteRuleActionArgs.builder()
.corsPolicy(corsPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.destinations(
destinations?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.faultInjectionPolicy(
faultInjectionPolicy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.redirect(redirect?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.requestHeaderModifier(
requestHeaderModifier?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.requestMirrorPolicy(
requestMirrorPolicy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.responseHeaderModifier(
responseHeaderModifier?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.retryPolicy(retryPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.timeout(timeout?.applyValue({ args0 -> args0 }))
.urlRewrite(urlRewrite?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [HttpRouteRuleActionArgs].
*/
@PulumiTagMarker
public class HttpRouteRuleActionArgsBuilder internal constructor() {
private var corsPolicy: Output? = null
private var destinations: Output>? = null
private var faultInjectionPolicy: Output? = null
private var redirect: Output? = null
private var requestHeaderModifier: Output? = null
private var requestMirrorPolicy: Output? = null
private var responseHeaderModifier: Output? = null
private var retryPolicy: Output? = null
private var timeout: Output? = null
private var urlRewrite: Output? = null
/**
* @param value The specification for allowing client side cross-origin requests.
* Structure is documented below.
*/
@JvmName("jcscvhacoyxxuhfc")
public suspend fun corsPolicy(`value`: Output) {
this.corsPolicy = value
}
/**
* @param value The destination to which traffic should be forwarded.
* Structure is documented below.
*/
@JvmName("gxvgeugvyhwtblrv")
public suspend fun destinations(`value`: Output>) {
this.destinations = value
}
@JvmName("huimgfstafpdacvm")
public suspend fun destinations(vararg values: Output) {
this.destinations = Output.all(values.asList())
}
/**
* @param values The destination to which traffic should be forwarded.
* Structure is documented below.
*/
@JvmName("vqlmnbmymjaqbret")
public suspend fun destinations(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy