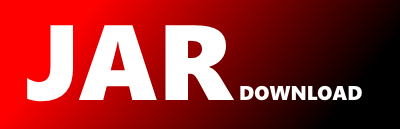
com.pulumi.gcp.networkservices.kotlin.inputs.HttpRouteRuleMatchHeaderArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkservices.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.networkservices.inputs.HttpRouteRuleMatchHeaderArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property exactMatch The value of the header should match exactly the content of exactMatch.
* @property header The name of the HTTP header to match against.
* @property invertMatch If specified, the match result will be inverted before checking. Default value is set to false.
* @property prefixMatch The value of the header must start with the contents of prefixMatch.
* @property presentMatch A header with headerName must exist. The match takes place whether or not the header has a value.
* @property rangeMatch If specified, the rule will match if the request header value is within the range.
* Structure is documented below.
* @property regexMatch The value of the header must match the regular expression specified in regexMatch.
* @property suffixMatch The value of the header must end with the contents of suffixMatch.
*/
public data class HttpRouteRuleMatchHeaderArgs(
public val exactMatch: Output? = null,
public val `header`: Output? = null,
public val invertMatch: Output? = null,
public val prefixMatch: Output? = null,
public val presentMatch: Output? = null,
public val rangeMatch: Output? = null,
public val regexMatch: Output? = null,
public val suffixMatch: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.networkservices.inputs.HttpRouteRuleMatchHeaderArgs =
com.pulumi.gcp.networkservices.inputs.HttpRouteRuleMatchHeaderArgs.builder()
.exactMatch(exactMatch?.applyValue({ args0 -> args0 }))
.`header`(`header`?.applyValue({ args0 -> args0 }))
.invertMatch(invertMatch?.applyValue({ args0 -> args0 }))
.prefixMatch(prefixMatch?.applyValue({ args0 -> args0 }))
.presentMatch(presentMatch?.applyValue({ args0 -> args0 }))
.rangeMatch(rangeMatch?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.regexMatch(regexMatch?.applyValue({ args0 -> args0 }))
.suffixMatch(suffixMatch?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [HttpRouteRuleMatchHeaderArgs].
*/
@PulumiTagMarker
public class HttpRouteRuleMatchHeaderArgsBuilder internal constructor() {
private var exactMatch: Output? = null
private var `header`: Output? = null
private var invertMatch: Output? = null
private var prefixMatch: Output? = null
private var presentMatch: Output? = null
private var rangeMatch: Output? = null
private var regexMatch: Output? = null
private var suffixMatch: Output? = null
/**
* @param value The value of the header should match exactly the content of exactMatch.
*/
@JvmName("mmybaxgiphotfrxi")
public suspend fun exactMatch(`value`: Output) {
this.exactMatch = value
}
/**
* @param value The name of the HTTP header to match against.
*/
@JvmName("mnwdutjpekrbjgaq")
public suspend fun `header`(`value`: Output) {
this.`header` = value
}
/**
* @param value If specified, the match result will be inverted before checking. Default value is set to false.
*/
@JvmName("yoycjpooibjpomwu")
public suspend fun invertMatch(`value`: Output) {
this.invertMatch = value
}
/**
* @param value The value of the header must start with the contents of prefixMatch.
*/
@JvmName("bokfkaoqiuhwjset")
public suspend fun prefixMatch(`value`: Output) {
this.prefixMatch = value
}
/**
* @param value A header with headerName must exist. The match takes place whether or not the header has a value.
*/
@JvmName("msurfjcojqvdqmyt")
public suspend fun presentMatch(`value`: Output) {
this.presentMatch = value
}
/**
* @param value If specified, the rule will match if the request header value is within the range.
* Structure is documented below.
*/
@JvmName("sugwixaesptrvouk")
public suspend fun rangeMatch(`value`: Output) {
this.rangeMatch = value
}
/**
* @param value The value of the header must match the regular expression specified in regexMatch.
*/
@JvmName("ftyiohgssurrjhqs")
public suspend fun regexMatch(`value`: Output) {
this.regexMatch = value
}
/**
* @param value The value of the header must end with the contents of suffixMatch.
*/
@JvmName("gclgadwjnywthmlq")
public suspend fun suffixMatch(`value`: Output) {
this.suffixMatch = value
}
/**
* @param value The value of the header should match exactly the content of exactMatch.
*/
@JvmName("dphtwekdkjmerunv")
public suspend fun exactMatch(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.exactMatch = mapped
}
/**
* @param value The name of the HTTP header to match against.
*/
@JvmName("uqvaamajbrflweko")
public suspend fun `header`(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`header` = mapped
}
/**
* @param value If specified, the match result will be inverted before checking. Default value is set to false.
*/
@JvmName("gbwossofirksnsav")
public suspend fun invertMatch(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.invertMatch = mapped
}
/**
* @param value The value of the header must start with the contents of prefixMatch.
*/
@JvmName("buostbemytwvtsvp")
public suspend fun prefixMatch(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.prefixMatch = mapped
}
/**
* @param value A header with headerName must exist. The match takes place whether or not the header has a value.
*/
@JvmName("ypwundhybfclhgil")
public suspend fun presentMatch(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.presentMatch = mapped
}
/**
* @param value If specified, the rule will match if the request header value is within the range.
* Structure is documented below.
*/
@JvmName("qhdotgqxtkuwyovd")
public suspend fun rangeMatch(`value`: HttpRouteRuleMatchHeaderRangeMatchArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rangeMatch = mapped
}
/**
* @param argument If specified, the rule will match if the request header value is within the range.
* Structure is documented below.
*/
@JvmName("unqhmpyxibjnyrqq")
public suspend fun rangeMatch(argument: suspend HttpRouteRuleMatchHeaderRangeMatchArgsBuilder.() -> Unit) {
val toBeMapped = HttpRouteRuleMatchHeaderRangeMatchArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rangeMatch = mapped
}
/**
* @param value The value of the header must match the regular expression specified in regexMatch.
*/
@JvmName("tcugommqiysyvkmr")
public suspend fun regexMatch(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.regexMatch = mapped
}
/**
* @param value The value of the header must end with the contents of suffixMatch.
*/
@JvmName("jwvbagyfygtwyweu")
public suspend fun suffixMatch(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.suffixMatch = mapped
}
internal fun build(): HttpRouteRuleMatchHeaderArgs = HttpRouteRuleMatchHeaderArgs(
exactMatch = exactMatch,
`header` = `header`,
invertMatch = invertMatch,
prefixMatch = prefixMatch,
presentMatch = presentMatch,
rangeMatch = rangeMatch,
regexMatch = regexMatch,
suffixMatch = suffixMatch,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy