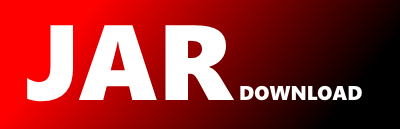
com.pulumi.gcp.networkservices.kotlin.inputs.LbTrafficExtensionExtensionChainExtensionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkservices.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.networkservices.inputs.LbTrafficExtensionExtensionChainExtensionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property authority The :authority header in the gRPC request sent from Envoy to the extension service.
* @property failOpen Determines how the proxy behaves if the call to the extension fails or times out.
* When set to TRUE, request or response processing continues without error.
* Any subsequent extensions in the extension chain are also executed.
* When set to FALSE: * If response headers have not been delivered to the downstream client,
* a generic 500 error is returned to the client. The error response can be tailored by
* configuring a custom error response in the load balancer.
* @property forwardHeaders List of the HTTP headers to forward to the extension (from the client or backend).
* If omitted, all headers are sent. Each element is a string indicating the header name.
* @property name The name for this extension. The name is logged as part of the HTTP request logs.
* The name must conform with RFC-1034, is restricted to lower-cased letters, numbers and hyphens,
* and can have a maximum length of 63 characters. Additionally, the first character must be a letter
* and the last a letter or a number.
* @property service The reference to the service that runs the extension. Must be a reference to a backend service
* @property supportedEvents A set of events during request or response processing for which this extension is called.
* This field is required for the LbTrafficExtension resource. It's not relevant for the LbRouteExtension
* resource. Possible values:`EVENT_TYPE_UNSPECIFIED`, `REQUEST_HEADERS`, `REQUEST_BODY`, `RESPONSE_HEADERS`,
* `RESPONSE_BODY`, `RESPONSE_BODY` and `RESPONSE_BODY`.
* - - -
* @property timeout Specifies the timeout for each individual message on the stream. The timeout must be between 10-1000 milliseconds.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
*/
public data class LbTrafficExtensionExtensionChainExtensionArgs(
public val authority: Output,
public val failOpen: Output? = null,
public val forwardHeaders: Output>? = null,
public val name: Output,
public val service: Output,
public val supportedEvents: Output>? = null,
public val timeout: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.networkservices.inputs.LbTrafficExtensionExtensionChainExtensionArgs =
com.pulumi.gcp.networkservices.inputs.LbTrafficExtensionExtensionChainExtensionArgs.builder()
.authority(authority.applyValue({ args0 -> args0 }))
.failOpen(failOpen?.applyValue({ args0 -> args0 }))
.forwardHeaders(forwardHeaders?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.name(name.applyValue({ args0 -> args0 }))
.service(service.applyValue({ args0 -> args0 }))
.supportedEvents(supportedEvents?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.timeout(timeout.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LbTrafficExtensionExtensionChainExtensionArgs].
*/
@PulumiTagMarker
public class LbTrafficExtensionExtensionChainExtensionArgsBuilder internal constructor() {
private var authority: Output? = null
private var failOpen: Output? = null
private var forwardHeaders: Output>? = null
private var name: Output? = null
private var service: Output? = null
private var supportedEvents: Output>? = null
private var timeout: Output? = null
/**
* @param value The :authority header in the gRPC request sent from Envoy to the extension service.
*/
@JvmName("fncmkgsinrkjetpn")
public suspend fun authority(`value`: Output) {
this.authority = value
}
/**
* @param value Determines how the proxy behaves if the call to the extension fails or times out.
* When set to TRUE, request or response processing continues without error.
* Any subsequent extensions in the extension chain are also executed.
* When set to FALSE: * If response headers have not been delivered to the downstream client,
* a generic 500 error is returned to the client. The error response can be tailored by
* configuring a custom error response in the load balancer.
*/
@JvmName("fqeqaagqabtepqbj")
public suspend fun failOpen(`value`: Output) {
this.failOpen = value
}
/**
* @param value List of the HTTP headers to forward to the extension (from the client or backend).
* If omitted, all headers are sent. Each element is a string indicating the header name.
*/
@JvmName("linkiyuepnegejnt")
public suspend fun forwardHeaders(`value`: Output>) {
this.forwardHeaders = value
}
@JvmName("llyrfcvycdbkepvh")
public suspend fun forwardHeaders(vararg values: Output) {
this.forwardHeaders = Output.all(values.asList())
}
/**
* @param values List of the HTTP headers to forward to the extension (from the client or backend).
* If omitted, all headers are sent. Each element is a string indicating the header name.
*/
@JvmName("edwyxldhoeuaeumf")
public suspend fun forwardHeaders(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy