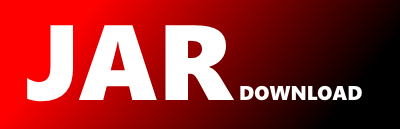
com.pulumi.gcp.notebooks.kotlin.InstanceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.notebooks.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.notebooks.InstanceArgs.builder
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceAcceleratorConfigArgs
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceAcceleratorConfigArgsBuilder
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceContainerImageArgs
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceContainerImageArgsBuilder
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceReservationAffinityArgs
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceReservationAffinityArgsBuilder
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceShieldedInstanceConfigArgs
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceShieldedInstanceConfigArgsBuilder
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceVmImageArgs
import com.pulumi.gcp.notebooks.kotlin.inputs.InstanceVmImageArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* > **Warning:** `google_notebook_instance` is deprecated and will be removed in a future major release. Use `gcp.workbench.Instance` instead.
* A Cloud AI Platform Notebook instance.
* > **Note:** Due to limitations of the Notebooks Instance API, many fields
* in this resource do not properly detect drift. These fields will also not
* appear in state once imported.
* To get more information about Instance, see:
* * [API documentation](https://cloud.google.com/ai-platform/notebooks/docs/reference/rest)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/ai-platform-notebooks)
* ## Example Usage
* ### Notebook Instance Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.notebooks.Instance("instance", {
* name: "notebooks-instance",
* location: "us-west1-a",
* machineType: "e2-medium",
* vmImage: {
* project: "deeplearning-platform-release",
* imageFamily: "tf-latest-cpu",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.notebooks.Instance("instance",
* name="notebooks-instance",
* location="us-west1-a",
* machine_type="e2-medium",
* vm_image=gcp.notebooks.InstanceVmImageArgs(
* project="deeplearning-platform-release",
* image_family="tf-latest-cpu",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.Notebooks.Instance("instance", new()
* {
* Name = "notebooks-instance",
* Location = "us-west1-a",
* MachineType = "e2-medium",
* VmImage = new Gcp.Notebooks.Inputs.InstanceVmImageArgs
* {
* Project = "deeplearning-platform-release",
* ImageFamily = "tf-latest-cpu",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/notebooks"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := notebooks.NewInstance(ctx, "instance", ¬ebooks.InstanceArgs{
* Name: pulumi.String("notebooks-instance"),
* Location: pulumi.String("us-west1-a"),
* MachineType: pulumi.String("e2-medium"),
* VmImage: ¬ebooks.InstanceVmImageArgs{
* Project: pulumi.String("deeplearning-platform-release"),
* ImageFamily: pulumi.String("tf-latest-cpu"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.notebooks.Instance;
* import com.pulumi.gcp.notebooks.InstanceArgs;
* import com.pulumi.gcp.notebooks.inputs.InstanceVmImageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new Instance("instance", InstanceArgs.builder()
* .name("notebooks-instance")
* .location("us-west1-a")
* .machineType("e2-medium")
* .vmImage(InstanceVmImageArgs.builder()
* .project("deeplearning-platform-release")
* .imageFamily("tf-latest-cpu")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:notebooks:Instance
* properties:
* name: notebooks-instance
* location: us-west1-a
* machineType: e2-medium
* vmImage:
* project: deeplearning-platform-release
* imageFamily: tf-latest-cpu
* ```
*
* ### Notebook Instance Basic Stopped
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.notebooks.Instance("instance", {
* name: "notebooks-instance",
* location: "us-west1-a",
* machineType: "e2-medium",
* vmImage: {
* project: "deeplearning-platform-release",
* imageFamily: "tf-latest-cpu",
* },
* desiredState: "STOPPED",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.notebooks.Instance("instance",
* name="notebooks-instance",
* location="us-west1-a",
* machine_type="e2-medium",
* vm_image=gcp.notebooks.InstanceVmImageArgs(
* project="deeplearning-platform-release",
* image_family="tf-latest-cpu",
* ),
* desired_state="STOPPED")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.Notebooks.Instance("instance", new()
* {
* Name = "notebooks-instance",
* Location = "us-west1-a",
* MachineType = "e2-medium",
* VmImage = new Gcp.Notebooks.Inputs.InstanceVmImageArgs
* {
* Project = "deeplearning-platform-release",
* ImageFamily = "tf-latest-cpu",
* },
* DesiredState = "STOPPED",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/notebooks"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := notebooks.NewInstance(ctx, "instance", ¬ebooks.InstanceArgs{
* Name: pulumi.String("notebooks-instance"),
* Location: pulumi.String("us-west1-a"),
* MachineType: pulumi.String("e2-medium"),
* VmImage: ¬ebooks.InstanceVmImageArgs{
* Project: pulumi.String("deeplearning-platform-release"),
* ImageFamily: pulumi.String("tf-latest-cpu"),
* },
* DesiredState: pulumi.String("STOPPED"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.notebooks.Instance;
* import com.pulumi.gcp.notebooks.InstanceArgs;
* import com.pulumi.gcp.notebooks.inputs.InstanceVmImageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new Instance("instance", InstanceArgs.builder()
* .name("notebooks-instance")
* .location("us-west1-a")
* .machineType("e2-medium")
* .vmImage(InstanceVmImageArgs.builder()
* .project("deeplearning-platform-release")
* .imageFamily("tf-latest-cpu")
* .build())
* .desiredState("STOPPED")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:notebooks:Instance
* properties:
* name: notebooks-instance
* location: us-west1-a
* machineType: e2-medium
* vmImage:
* project: deeplearning-platform-release
* imageFamily: tf-latest-cpu
* desiredState: STOPPED
* ```
*
* ### Notebook Instance Basic Container
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.notebooks.Instance("instance", {
* name: "notebooks-instance",
* location: "us-west1-a",
* machineType: "e2-medium",
* metadata: {
* "proxy-mode": "service_account",
* },
* containerImage: {
* repository: "gcr.io/deeplearning-platform-release/base-cpu",
* tag: "latest",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.notebooks.Instance("instance",
* name="notebooks-instance",
* location="us-west1-a",
* machine_type="e2-medium",
* metadata={
* "proxy-mode": "service_account",
* },
* container_image=gcp.notebooks.InstanceContainerImageArgs(
* repository="gcr.io/deeplearning-platform-release/base-cpu",
* tag="latest",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.Notebooks.Instance("instance", new()
* {
* Name = "notebooks-instance",
* Location = "us-west1-a",
* MachineType = "e2-medium",
* Metadata =
* {
* { "proxy-mode", "service_account" },
* },
* ContainerImage = new Gcp.Notebooks.Inputs.InstanceContainerImageArgs
* {
* Repository = "gcr.io/deeplearning-platform-release/base-cpu",
* Tag = "latest",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/notebooks"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := notebooks.NewInstance(ctx, "instance", ¬ebooks.InstanceArgs{
* Name: pulumi.String("notebooks-instance"),
* Location: pulumi.String("us-west1-a"),
* MachineType: pulumi.String("e2-medium"),
* Metadata: pulumi.StringMap{
* "proxy-mode": pulumi.String("service_account"),
* },
* ContainerImage: ¬ebooks.InstanceContainerImageArgs{
* Repository: pulumi.String("gcr.io/deeplearning-platform-release/base-cpu"),
* Tag: pulumi.String("latest"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.notebooks.Instance;
* import com.pulumi.gcp.notebooks.InstanceArgs;
* import com.pulumi.gcp.notebooks.inputs.InstanceContainerImageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new Instance("instance", InstanceArgs.builder()
* .name("notebooks-instance")
* .location("us-west1-a")
* .machineType("e2-medium")
* .metadata(Map.of("proxy-mode", "service_account"))
* .containerImage(InstanceContainerImageArgs.builder()
* .repository("gcr.io/deeplearning-platform-release/base-cpu")
* .tag("latest")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:notebooks:Instance
* properties:
* name: notebooks-instance
* location: us-west1-a
* machineType: e2-medium
* metadata:
* proxy-mode: service_account
* containerImage:
* repository: gcr.io/deeplearning-platform-release/base-cpu
* tag: latest
* ```
*
* ### Notebook Instance Basic Gpu
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.notebooks.Instance("instance", {
* name: "notebooks-instance",
* location: "us-west1-a",
* machineType: "n1-standard-1",
* installGpuDriver: true,
* acceleratorConfig: {
* type: "NVIDIA_TESLA_T4",
* coreCount: 1,
* },
* vmImage: {
* project: "deeplearning-platform-release",
* imageFamily: "tf-latest-gpu",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.notebooks.Instance("instance",
* name="notebooks-instance",
* location="us-west1-a",
* machine_type="n1-standard-1",
* install_gpu_driver=True,
* accelerator_config=gcp.notebooks.InstanceAcceleratorConfigArgs(
* type="NVIDIA_TESLA_T4",
* core_count=1,
* ),
* vm_image=gcp.notebooks.InstanceVmImageArgs(
* project="deeplearning-platform-release",
* image_family="tf-latest-gpu",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.Notebooks.Instance("instance", new()
* {
* Name = "notebooks-instance",
* Location = "us-west1-a",
* MachineType = "n1-standard-1",
* InstallGpuDriver = true,
* AcceleratorConfig = new Gcp.Notebooks.Inputs.InstanceAcceleratorConfigArgs
* {
* Type = "NVIDIA_TESLA_T4",
* CoreCount = 1,
* },
* VmImage = new Gcp.Notebooks.Inputs.InstanceVmImageArgs
* {
* Project = "deeplearning-platform-release",
* ImageFamily = "tf-latest-gpu",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/notebooks"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := notebooks.NewInstance(ctx, "instance", ¬ebooks.InstanceArgs{
* Name: pulumi.String("notebooks-instance"),
* Location: pulumi.String("us-west1-a"),
* MachineType: pulumi.String("n1-standard-1"),
* InstallGpuDriver: pulumi.Bool(true),
* AcceleratorConfig: ¬ebooks.InstanceAcceleratorConfigArgs{
* Type: pulumi.String("NVIDIA_TESLA_T4"),
* CoreCount: pulumi.Int(1),
* },
* VmImage: ¬ebooks.InstanceVmImageArgs{
* Project: pulumi.String("deeplearning-platform-release"),
* ImageFamily: pulumi.String("tf-latest-gpu"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.notebooks.Instance;
* import com.pulumi.gcp.notebooks.InstanceArgs;
* import com.pulumi.gcp.notebooks.inputs.InstanceAcceleratorConfigArgs;
* import com.pulumi.gcp.notebooks.inputs.InstanceVmImageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new Instance("instance", InstanceArgs.builder()
* .name("notebooks-instance")
* .location("us-west1-a")
* .machineType("n1-standard-1")
* .installGpuDriver(true)
* .acceleratorConfig(InstanceAcceleratorConfigArgs.builder()
* .type("NVIDIA_TESLA_T4")
* .coreCount(1)
* .build())
* .vmImage(InstanceVmImageArgs.builder()
* .project("deeplearning-platform-release")
* .imageFamily("tf-latest-gpu")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:notebooks:Instance
* properties:
* name: notebooks-instance
* location: us-west1-a
* machineType: n1-standard-1
* installGpuDriver: true
* acceleratorConfig:
* type: NVIDIA_TESLA_T4
* coreCount: 1
* vmImage:
* project: deeplearning-platform-release
* imageFamily: tf-latest-gpu
* ```
*
* ### Notebook Instance Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const myNetwork = gcp.compute.getNetwork({
* name: "default",
* });
* const mySubnetwork = gcp.compute.getSubnetwork({
* name: "default",
* region: "us-central1",
* });
* const instance = new gcp.notebooks.Instance("instance", {
* name: "notebooks-instance",
* location: "us-central1-a",
* machineType: "e2-medium",
* vmImage: {
* project: "deeplearning-platform-release",
* imageFamily: "tf-latest-cpu",
* },
* instanceOwners: ["my@service-account.com"],
* serviceAccount: "[email protected]",
* installGpuDriver: true,
* bootDiskType: "PD_SSD",
* bootDiskSizeGb: 110,
* noPublicIp: true,
* noProxyAccess: true,
* network: myNetwork.then(myNetwork => myNetwork.id),
* subnet: mySubnetwork.then(mySubnetwork => mySubnetwork.id),
* labels: {
* k: "val",
* },
* metadata: {
* terraform: "true",
* },
* serviceAccountScopes: [
* "https://www.googleapis.com/auth/bigquery",
* "https://www.googleapis.com/auth/devstorage.read_write",
* "https://www.googleapis.com/auth/cloud-platform",
* "https://www.googleapis.com/auth/userinfo.email",
* ],
* tags: [
* "foo",
* "bar",
* ],
* diskEncryption: "CMEK",
* kmsKey: "my-crypto-key",
* desiredState: "ACTIVE",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_network = gcp.compute.get_network(name="default")
* my_subnetwork = gcp.compute.get_subnetwork(name="default",
* region="us-central1")
* instance = gcp.notebooks.Instance("instance",
* name="notebooks-instance",
* location="us-central1-a",
* machine_type="e2-medium",
* vm_image=gcp.notebooks.InstanceVmImageArgs(
* project="deeplearning-platform-release",
* image_family="tf-latest-cpu",
* ),
* instance_owners=["my@service-account.com"],
* service_account="[email protected]",
* install_gpu_driver=True,
* boot_disk_type="PD_SSD",
* boot_disk_size_gb=110,
* no_public_ip=True,
* no_proxy_access=True,
* network=my_network.id,
* subnet=my_subnetwork.id,
* labels={
* "k": "val",
* },
* metadata={
* "terraform": "true",
* },
* service_account_scopes=[
* "https://www.googleapis.com/auth/bigquery",
* "https://www.googleapis.com/auth/devstorage.read_write",
* "https://www.googleapis.com/auth/cloud-platform",
* "https://www.googleapis.com/auth/userinfo.email",
* ],
* tags=[
* "foo",
* "bar",
* ],
* disk_encryption="CMEK",
* kms_key="my-crypto-key",
* desired_state="ACTIVE")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var myNetwork = Gcp.Compute.GetNetwork.Invoke(new()
* {
* Name = "default",
* });
* var mySubnetwork = Gcp.Compute.GetSubnetwork.Invoke(new()
* {
* Name = "default",
* Region = "us-central1",
* });
* var instance = new Gcp.Notebooks.Instance("instance", new()
* {
* Name = "notebooks-instance",
* Location = "us-central1-a",
* MachineType = "e2-medium",
* VmImage = new Gcp.Notebooks.Inputs.InstanceVmImageArgs
* {
* Project = "deeplearning-platform-release",
* ImageFamily = "tf-latest-cpu",
* },
* InstanceOwners = new[]
* {
* "[email protected]",
* },
* ServiceAccount = "[email protected]",
* InstallGpuDriver = true,
* BootDiskType = "PD_SSD",
* BootDiskSizeGb = 110,
* NoPublicIp = true,
* NoProxyAccess = true,
* Network = myNetwork.Apply(getNetworkResult => getNetworkResult.Id),
* Subnet = mySubnetwork.Apply(getSubnetworkResult => getSubnetworkResult.Id),
* Labels =
* {
* { "k", "val" },
* },
* Metadata =
* {
* { "terraform", "true" },
* },
* ServiceAccountScopes = new[]
* {
* "https://www.googleapis.com/auth/bigquery",
* "https://www.googleapis.com/auth/devstorage.read_write",
* "https://www.googleapis.com/auth/cloud-platform",
* "https://www.googleapis.com/auth/userinfo.email",
* },
* Tags = new[]
* {
* "foo",
* "bar",
* },
* DiskEncryption = "CMEK",
* KmsKey = "my-crypto-key",
* DesiredState = "ACTIVE",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/notebooks"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* myNetwork, err := compute.LookupNetwork(ctx, &compute.LookupNetworkArgs{
* Name: "default",
* }, nil)
* if err != nil {
* return err
* }
* mySubnetwork, err := compute.LookupSubnetwork(ctx, &compute.LookupSubnetworkArgs{
* Name: pulumi.StringRef("default"),
* Region: pulumi.StringRef("us-central1"),
* }, nil)
* if err != nil {
* return err
* }
* _, err = notebooks.NewInstance(ctx, "instance", ¬ebooks.InstanceArgs{
* Name: pulumi.String("notebooks-instance"),
* Location: pulumi.String("us-central1-a"),
* MachineType: pulumi.String("e2-medium"),
* VmImage: ¬ebooks.InstanceVmImageArgs{
* Project: pulumi.String("deeplearning-platform-release"),
* ImageFamily: pulumi.String("tf-latest-cpu"),
* },
* InstanceOwners: pulumi.StringArray{
* pulumi.String("[email protected]"),
* },
* ServiceAccount: pulumi.String("[email protected]"),
* InstallGpuDriver: pulumi.Bool(true),
* BootDiskType: pulumi.String("PD_SSD"),
* BootDiskSizeGb: pulumi.Int(110),
* NoPublicIp: pulumi.Bool(true),
* NoProxyAccess: pulumi.Bool(true),
* Network: pulumi.String(myNetwork.Id),
* Subnet: pulumi.String(mySubnetwork.Id),
* Labels: pulumi.StringMap{
* "k": pulumi.String("val"),
* },
* Metadata: pulumi.StringMap{
* "terraform": pulumi.String("true"),
* },
* ServiceAccountScopes: pulumi.StringArray{
* pulumi.String("https://www.googleapis.com/auth/bigquery"),
* pulumi.String("https://www.googleapis.com/auth/devstorage.read_write"),
* pulumi.String("https://www.googleapis.com/auth/cloud-platform"),
* pulumi.String("https://www.googleapis.com/auth/userinfo.email"),
* },
* Tags: pulumi.StringArray{
* pulumi.String("foo"),
* pulumi.String("bar"),
* },
* DiskEncryption: pulumi.String("CMEK"),
* KmsKey: pulumi.String("my-crypto-key"),
* DesiredState: pulumi.String("ACTIVE"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.ComputeFunctions;
* import com.pulumi.gcp.compute.inputs.GetNetworkArgs;
* import com.pulumi.gcp.compute.inputs.GetSubnetworkArgs;
* import com.pulumi.gcp.notebooks.Instance;
* import com.pulumi.gcp.notebooks.InstanceArgs;
* import com.pulumi.gcp.notebooks.inputs.InstanceVmImageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var myNetwork = ComputeFunctions.getNetwork(GetNetworkArgs.builder()
* .name("default")
* .build());
* final var mySubnetwork = ComputeFunctions.getSubnetwork(GetSubnetworkArgs.builder()
* .name("default")
* .region("us-central1")
* .build());
* var instance = new Instance("instance", InstanceArgs.builder()
* .name("notebooks-instance")
* .location("us-central1-a")
* .machineType("e2-medium")
* .vmImage(InstanceVmImageArgs.builder()
* .project("deeplearning-platform-release")
* .imageFamily("tf-latest-cpu")
* .build())
* .instanceOwners("[email protected]")
* .serviceAccount("[email protected]")
* .installGpuDriver(true)
* .bootDiskType("PD_SSD")
* .bootDiskSizeGb(110)
* .noPublicIp(true)
* .noProxyAccess(true)
* .network(myNetwork.applyValue(getNetworkResult -> getNetworkResult.id()))
* .subnet(mySubnetwork.applyValue(getSubnetworkResult -> getSubnetworkResult.id()))
* .labels(Map.of("k", "val"))
* .metadata(Map.of("terraform", "true"))
* .serviceAccountScopes(
* "https://www.googleapis.com/auth/bigquery",
* "https://www.googleapis.com/auth/devstorage.read_write",
* "https://www.googleapis.com/auth/cloud-platform",
* "https://www.googleapis.com/auth/userinfo.email")
* .tags(
* "foo",
* "bar")
* .diskEncryption("CMEK")
* .kmsKey("my-crypto-key")
* .desiredState("ACTIVE")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:notebooks:Instance
* properties:
* name: notebooks-instance
* location: us-central1-a
* machineType: e2-medium
* vmImage:
* project: deeplearning-platform-release
* imageFamily: tf-latest-cpu
* instanceOwners:
* - [email protected]
* serviceAccount: [email protected]
* installGpuDriver: true
* bootDiskType: PD_SSD
* bootDiskSizeGb: 110
* noPublicIp: true
* noProxyAccess: true
* network: ${myNetwork.id}
* subnet: ${mySubnetwork.id}
* labels:
* k: val
* metadata:
* terraform: 'true'
* serviceAccountScopes:
* - https://www.googleapis.com/auth/bigquery
* - https://www.googleapis.com/auth/devstorage.read_write
* - https://www.googleapis.com/auth/cloud-platform
* - https://www.googleapis.com/auth/userinfo.email
* tags:
* - foo
* - bar
* diskEncryption: CMEK
* kmsKey: my-crypto-key
* desiredState: ACTIVE
* variables:
* myNetwork:
* fn::invoke:
* Function: gcp:compute:getNetwork
* Arguments:
* name: default
* mySubnetwork:
* fn::invoke:
* Function: gcp:compute:getSubnetwork
* Arguments:
* name: default
* region: us-central1
* ```
*
* ## Import
* Instance can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/instances/{{name}}`
* * `{{project}}/{{location}}/{{name}}`
* * `{{location}}/{{name}}`
* When using the `pulumi import` command, Instance can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:notebooks/instance:Instance default projects/{{project}}/locations/{{location}}/instances/{{name}}
* ```
* ```sh
* $ pulumi import gcp:notebooks/instance:Instance default {{project}}/{{location}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:notebooks/instance:Instance default {{location}}/{{name}}
* ```
* @property acceleratorConfig The hardware accelerator used on this instance. If you use accelerators,
* make sure that your configuration has enough vCPUs and memory to support the
* machineType you have selected.
* Structure is documented below.
* @property bootDiskSizeGb The size of the boot disk in GB attached to this instance,
* up to a maximum of 64000 GB (64 TB). The minimum recommended value is 100 GB.
* If not specified, this defaults to 100.
* @property bootDiskType Possible disk types for notebook instances.
* Possible values are: `DISK_TYPE_UNSPECIFIED`, `PD_STANDARD`, `PD_SSD`, `PD_BALANCED`, `PD_EXTREME`.
* @property containerImage Use a container image to start the notebook instance.
* Structure is documented below.
* @property createTime Instance creation time
* @property customGpuDriverPath Specify a custom Cloud Storage path where the GPU driver is stored.
* If not specified, we'll automatically choose from official GPU drivers.
* @property dataDiskSizeGb The size of the data disk in GB attached to this instance,
* up to a maximum of 64000 GB (64 TB).
* You can choose the size of the data disk based on how big your notebooks and data are.
* If not specified, this defaults to 100.
* @property dataDiskType Possible disk types for notebook instances.
* Possible values are: `DISK_TYPE_UNSPECIFIED`, `PD_STANDARD`, `PD_SSD`, `PD_BALANCED`, `PD_EXTREME`.
* @property desiredState Desired state of the Notebook Instance. Set this field to `ACTIVE` to start the Instance, and `STOPPED` to stop the Instance.
* @property diskEncryption Disk encryption method used on the boot and data disks, defaults to GMEK.
* Possible values are: `DISK_ENCRYPTION_UNSPECIFIED`, `GMEK`, `CMEK`.
* @property installGpuDriver Whether the end user authorizes Google Cloud to install GPU driver
* on this instance. If this field is empty or set to false, the GPU driver
* won't be installed. Only applicable to instances with GPUs.
* @property instanceOwners The list of owners of this instance after creation.
* Format: [email protected].
* Currently supports one owner only.
* If not specified, all of the service account users of
* your VM instance's service account can use the instance.
* @property kmsKey The KMS key used to encrypt the disks, only applicable if diskEncryption is CMEK.
* Format: projects/{project_id}/locations/{location}/keyRings/{key_ring_id}/cryptoKeys/{key_id}
* @property labels Labels to apply to this instance. These can be later modified by the setLabels method.
* An object containing a list of "key": value pairs. Example: { "name": "wrench", "mass": "1.3kg", "count": "3" }.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property location A reference to the zone where the machine resides.
* - - -
* @property machineType A reference to a machine type which defines VM kind.
* @property metadata Custom metadata to apply to this instance.
* An object containing a list of "key": value pairs. Example: { "name": "wrench", "mass": "1.3kg", "count": "3" }.
* @property name The name specified for the Notebook instance.
* @property network The name of the VPC that this instance is in.
* Format: projects/{project_id}/global/networks/{network_id}
* @property nicType The type of vNIC driver.
* Possible values are: `UNSPECIFIED_NIC_TYPE`, `VIRTIO_NET`, `GVNIC`.
* @property noProxyAccess The notebook instance will not register with the proxy..
* @property noPublicIp No public IP will be assigned to this instance.
* @property noRemoveDataDisk If true, the data disk will not be auto deleted when deleting the instance.
* @property postStartupScript Path to a Bash script that automatically runs after a
* notebook instance fully boots up. The path must be a URL
* or Cloud Storage path (gs://path-to-file/file-name).
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property reservationAffinity Reservation Affinity for consuming Zonal reservation.
* Structure is documented below.
* @property serviceAccount The service account on this instance, giving access to other
* Google Cloud services. You can use any service account within
* the same project, but you must have the service account user
* permission to use the instance. If not specified,
* the Compute Engine default service account is used.
* @property serviceAccountScopes Optional. The URIs of service account scopes to be included in Compute Engine instances.
* If not specified, the following scopes are defined:
* - https://www.googleapis.com/auth/cloud-platform
* - https://www.googleapis.com/auth/userinfo.email
* @property shieldedInstanceConfig A set of Shielded Instance options. Check [Images using supported Shielded VM features]
* Not all combinations are valid
* Structure is documented below.
* @property subnet The name of the subnet that this instance is in.
* Format: projects/{project_id}/regions/{region}/subnetworks/{subnetwork_id}
* @property tags The Compute Engine tags to add to instance.
* @property updateTime Instance update time.
* @property vmImage Use a Compute Engine VM image to start the notebook instance.
* Structure is documented below.
*/
public data class InstanceArgs(
public val acceleratorConfig: Output? = null,
public val bootDiskSizeGb: Output? = null,
public val bootDiskType: Output? = null,
public val containerImage: Output? = null,
public val createTime: Output? = null,
public val customGpuDriverPath: Output? = null,
public val dataDiskSizeGb: Output? = null,
public val dataDiskType: Output? = null,
public val desiredState: Output? = null,
public val diskEncryption: Output? = null,
public val installGpuDriver: Output? = null,
public val instanceOwners: Output>? = null,
public val kmsKey: Output? = null,
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy