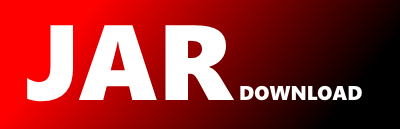
com.pulumi.gcp.notebooks.kotlin.inputs.RuntimeVirtualMachineVirtualMachineConfigDataDiskInitializeParamsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.notebooks.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.notebooks.inputs.RuntimeVirtualMachineVirtualMachineConfigDataDiskInitializeParamsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property description Provide this property when creating the disk.
* @property diskName Specifies the disk name. If not specified, the default is
* to use the name of the instance. If the disk with the
* instance name exists already in the given zone/region, a
* new name will be automatically generated.
* @property diskSizeGb Specifies the size of the disk in base-2 GB. If not
* specified, the disk will be the same size as the image
* (usually 10GB). If specified, the size must be equal to
* or larger than 10GB. Default 100 GB.
* @property diskType The type of the boot disk attached to this runtime,
* defaults to standard persistent disk. For valid values,
* see `https://cloud.google.com/vertex-ai/docs/workbench/
* reference/rest/v1/projects.locations.runtimes#disktype`
* @property labels Labels to apply to this disk. These can be later modified
* by the disks.setLabels method. This field is only
* applicable for persistent disks.
*/
public data class RuntimeVirtualMachineVirtualMachineConfigDataDiskInitializeParamsArgs(
public val description: Output? = null,
public val diskName: Output? = null,
public val diskSizeGb: Output? = null,
public val diskType: Output? = null,
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy