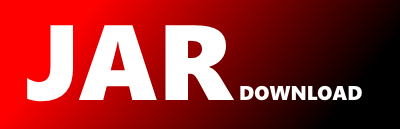
com.pulumi.gcp.notebooks.kotlin.outputs.RuntimeVirtualMachineVirtualMachineConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.notebooks.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property acceleratorConfig The Compute Engine accelerator configuration for this runtime.
* Structure is documented below.
* @property containerImages Use a list of container images to start the notebook instance.
* Structure is documented below.
* @property dataDisk Data disk option configuration settings.
* Structure is documented below.
* @property encryptionConfig Encryption settings for virtual machine data disk.
* Structure is documented below.
* @property guestAttributes (Output)
* The Compute Engine guest attributes. (see [Project and instance
* guest attributes](https://cloud.google.com/compute/docs/
* storing-retrieving-metadata#guest_attributes)).
* @property internalIpOnly If true, runtime will only have internal IP addresses. By default,
* runtimes are not restricted to internal IP addresses, and will
* have ephemeral external IP addresses assigned to each vm. This
* `internal_ip_only` restriction can only be enabled for subnetwork
* enabled networks, and all dependencies must be configured to be
* accessible without external IP addresses.
* @property labels The labels to associate with this runtime. Label **keys** must
* contain 1 to 63 characters, and must conform to [RFC 1035]
* (https://www.ietf.org/rfc/rfc1035.txt). Label **values** may be
* empty, but, if present, must contain 1 to 63 characters, and must
* conform to [RFC 1035](https://www.ietf.org/rfc/rfc1035.txt). No
* more than 32 labels can be associated with a cluster.
* @property machineType The Compute Engine machine type used for runtimes.
* @property metadata The Compute Engine metadata entries to add to virtual machine.
* (see [Project and instance metadata](https://cloud.google.com
* /compute/docs/storing-retrieving-metadata#project_and_instance
* _metadata)).
* @property network The Compute Engine network to be used for machine communications.
* Cannot be specified with subnetwork. If neither `network` nor
* `subnet` is specified, the "default" network of the project is
* used, if it exists. A full URL or partial URI. Examples:
* * `https://www.googleapis.com/compute/v1/projects/[project_id]/
* regions/global/default`
* * `projects/[project_id]/regions/global/default`
* Runtimes are managed resources inside Google Infrastructure.
* Runtimes support the following network configurations:
* * Google Managed Network (Network & subnet are empty)
* * Consumer Project VPC (network & subnet are required). Requires
* configuring Private Service Access.
* * Shared VPC (network & subnet are required). Requires
* configuring Private Service Access.
* @property nicType The type of vNIC to be used on this interface. This may be gVNIC
* or VirtioNet.
* Possible values are: `UNSPECIFIED_NIC_TYPE`, `VIRTIO_NET`, `GVNIC`.
* @property reservedIpRange Reserved IP Range name is used for VPC Peering. The
* subnetwork allocation will use the range *name* if it's assigned.
* @property shieldedInstanceConfig Shielded VM Instance configuration settings.
* Structure is documented below.
* @property subnet The Compute Engine subnetwork to be used for machine
* communications. Cannot be specified with network. A full URL or
* partial URI are valid. Examples:
* * `https://www.googleapis.com/compute/v1/projects/[project_id]/
* regions/us-east1/subnetworks/sub0`
* * `projects/[project_id]/regions/us-east1/subnetworks/sub0`
* @property tags The Compute Engine tags to add to runtime (see [Tagging instances]
* (https://cloud.google.com/compute/docs/
* label-or-tag-resources#tags)).
* @property zone (Output)
* The zone where the virtual machine is located.
*/
public data class RuntimeVirtualMachineVirtualMachineConfig(
public val acceleratorConfig: RuntimeVirtualMachineVirtualMachineConfigAcceleratorConfig? = null,
public val containerImages: List? = null,
public val dataDisk: RuntimeVirtualMachineVirtualMachineConfigDataDisk,
public val encryptionConfig: RuntimeVirtualMachineVirtualMachineConfigEncryptionConfig? = null,
public val guestAttributes: Map? = null,
public val internalIpOnly: Boolean? = null,
public val labels: Map? = null,
public val machineType: String,
public val metadata: Map? = null,
public val network: String? = null,
public val nicType: String? = null,
public val reservedIpRange: String? = null,
public val shieldedInstanceConfig: RuntimeVirtualMachineVirtualMachineConfigShieldedInstanceConfig? = null,
public val subnet: String? = null,
public val tags: List? = null,
public val zone: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.notebooks.outputs.RuntimeVirtualMachineVirtualMachineConfig): RuntimeVirtualMachineVirtualMachineConfig = RuntimeVirtualMachineVirtualMachineConfig(
acceleratorConfig = javaType.acceleratorConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.notebooks.kotlin.outputs.RuntimeVirtualMachineVirtualMachineConfigAcceleratorConfig.Companion.toKotlin(args0)
})
}).orElse(null),
containerImages = javaType.containerImages().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.notebooks.kotlin.outputs.RuntimeVirtualMachineVirtualMachineConfigContainerImage.Companion.toKotlin(args0)
})
}),
dataDisk = javaType.dataDisk().let({ args0 ->
com.pulumi.gcp.notebooks.kotlin.outputs.RuntimeVirtualMachineVirtualMachineConfigDataDisk.Companion.toKotlin(args0)
}),
encryptionConfig = javaType.encryptionConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.notebooks.kotlin.outputs.RuntimeVirtualMachineVirtualMachineConfigEncryptionConfig.Companion.toKotlin(args0)
})
}).orElse(null),
guestAttributes = javaType.guestAttributes().map({ args0 -> args0.key.to(args0.value) }).toMap(),
internalIpOnly = javaType.internalIpOnly().map({ args0 -> args0 }).orElse(null),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
machineType = javaType.machineType(),
metadata = javaType.metadata().map({ args0 -> args0.key.to(args0.value) }).toMap(),
network = javaType.network().map({ args0 -> args0 }).orElse(null),
nicType = javaType.nicType().map({ args0 -> args0 }).orElse(null),
reservedIpRange = javaType.reservedIpRange().map({ args0 -> args0 }).orElse(null),
shieldedInstanceConfig = javaType.shieldedInstanceConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.notebooks.kotlin.outputs.RuntimeVirtualMachineVirtualMachineConfigShieldedInstanceConfig.Companion.toKotlin(args0)
})
}).orElse(null),
subnet = javaType.subnet().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 -> args0 }),
zone = javaType.zone().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy