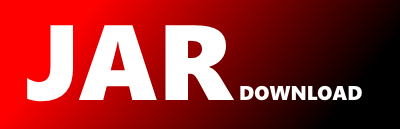
com.pulumi.gcp.osconfig.kotlin.PatchDeployment.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.osconfig.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentInstanceFilter
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentOneTimeSchedule
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentPatchConfig
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentRecurringSchedule
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentRollout
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentInstanceFilter.Companion.toKotlin as patchDeploymentInstanceFilterToKotlin
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentOneTimeSchedule.Companion.toKotlin as patchDeploymentOneTimeScheduleToKotlin
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentPatchConfig.Companion.toKotlin as patchDeploymentPatchConfigToKotlin
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentRecurringSchedule.Companion.toKotlin as patchDeploymentRecurringScheduleToKotlin
import com.pulumi.gcp.osconfig.kotlin.outputs.PatchDeploymentRollout.Companion.toKotlin as patchDeploymentRolloutToKotlin
/**
* Builder for [PatchDeployment].
*/
@PulumiTagMarker
public class PatchDeploymentResourceBuilder internal constructor() {
public var name: String? = null
public var args: PatchDeploymentArgs = PatchDeploymentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend PatchDeploymentArgsBuilder.() -> Unit) {
val builder = PatchDeploymentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): PatchDeployment {
val builtJavaResource = com.pulumi.gcp.osconfig.PatchDeployment(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return PatchDeployment(builtJavaResource)
}
}
/**
* Patch deployments are configurations that individual patch jobs use to complete a patch.
* These configurations include instance filter, package repository settings, and a schedule.
* To get more information about PatchDeployment, see:
* * [API documentation](https://cloud.google.com/compute/docs/osconfig/rest)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/compute/docs/os-patch-management)
* ## Example Usage
* ### Os Config Patch Deployment Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const patch = new gcp.osconfig.PatchDeployment("patch", {
* patchDeploymentId: "patch-deploy",
* instanceFilter: {
* all: true,
* },
* oneTimeSchedule: {
* executeTime: "2999-10-10T10:10:10.045123456Z",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* patch = gcp.osconfig.PatchDeployment("patch",
* patch_deployment_id="patch-deploy",
* instance_filter=gcp.osconfig.PatchDeploymentInstanceFilterArgs(
* all=True,
* ),
* one_time_schedule=gcp.osconfig.PatchDeploymentOneTimeScheduleArgs(
* execute_time="2999-10-10T10:10:10.045123456Z",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var patch = new Gcp.OsConfig.PatchDeployment("patch", new()
* {
* PatchDeploymentId = "patch-deploy",
* InstanceFilter = new Gcp.OsConfig.Inputs.PatchDeploymentInstanceFilterArgs
* {
* All = true,
* },
* OneTimeSchedule = new Gcp.OsConfig.Inputs.PatchDeploymentOneTimeScheduleArgs
* {
* ExecuteTime = "2999-10-10T10:10:10.045123456Z",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/osconfig"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := osconfig.NewPatchDeployment(ctx, "patch", &osconfig.PatchDeploymentArgs{
* PatchDeploymentId: pulumi.String("patch-deploy"),
* InstanceFilter: &osconfig.PatchDeploymentInstanceFilterArgs{
* All: pulumi.Bool(true),
* },
* OneTimeSchedule: &osconfig.PatchDeploymentOneTimeScheduleArgs{
* ExecuteTime: pulumi.String("2999-10-10T10:10:10.045123456Z"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.osconfig.PatchDeployment;
* import com.pulumi.gcp.osconfig.PatchDeploymentArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentInstanceFilterArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentOneTimeScheduleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var patch = new PatchDeployment("patch", PatchDeploymentArgs.builder()
* .patchDeploymentId("patch-deploy")
* .instanceFilter(PatchDeploymentInstanceFilterArgs.builder()
* .all(true)
* .build())
* .oneTimeSchedule(PatchDeploymentOneTimeScheduleArgs.builder()
* .executeTime("2999-10-10T10:10:10.045123456Z")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* patch:
* type: gcp:osconfig:PatchDeployment
* properties:
* patchDeploymentId: patch-deploy
* instanceFilter:
* all: true
* oneTimeSchedule:
* executeTime: 2999-10-10T10:10:10.045123456Z
* ```
*
* ### Os Config Patch Deployment Daily
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const patch = new gcp.osconfig.PatchDeployment("patch", {
* patchDeploymentId: "patch-deploy",
* instanceFilter: {
* all: true,
* },
* recurringSchedule: {
* timeZone: {
* id: "America/New_York",
* },
* timeOfDay: {
* hours: 0,
* minutes: 30,
* seconds: 30,
* nanos: 20,
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* patch = gcp.osconfig.PatchDeployment("patch",
* patch_deployment_id="patch-deploy",
* instance_filter=gcp.osconfig.PatchDeploymentInstanceFilterArgs(
* all=True,
* ),
* recurring_schedule=gcp.osconfig.PatchDeploymentRecurringScheduleArgs(
* time_zone=gcp.osconfig.PatchDeploymentRecurringScheduleTimeZoneArgs(
* id="America/New_York",
* ),
* time_of_day=gcp.osconfig.PatchDeploymentRecurringScheduleTimeOfDayArgs(
* hours=0,
* minutes=30,
* seconds=30,
* nanos=20,
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var patch = new Gcp.OsConfig.PatchDeployment("patch", new()
* {
* PatchDeploymentId = "patch-deploy",
* InstanceFilter = new Gcp.OsConfig.Inputs.PatchDeploymentInstanceFilterArgs
* {
* All = true,
* },
* RecurringSchedule = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleArgs
* {
* TimeZone = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleTimeZoneArgs
* {
* Id = "America/New_York",
* },
* TimeOfDay = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs
* {
* Hours = 0,
* Minutes = 30,
* Seconds = 30,
* Nanos = 20,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/osconfig"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := osconfig.NewPatchDeployment(ctx, "patch", &osconfig.PatchDeploymentArgs{
* PatchDeploymentId: pulumi.String("patch-deploy"),
* InstanceFilter: &osconfig.PatchDeploymentInstanceFilterArgs{
* All: pulumi.Bool(true),
* },
* RecurringSchedule: &osconfig.PatchDeploymentRecurringScheduleArgs{
* TimeZone: &osconfig.PatchDeploymentRecurringScheduleTimeZoneArgs{
* Id: pulumi.String("America/New_York"),
* },
* TimeOfDay: &osconfig.PatchDeploymentRecurringScheduleTimeOfDayArgs{
* Hours: pulumi.Int(0),
* Minutes: pulumi.Int(30),
* Seconds: pulumi.Int(30),
* Nanos: pulumi.Int(20),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.osconfig.PatchDeployment;
* import com.pulumi.gcp.osconfig.PatchDeploymentArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentInstanceFilterArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleTimeZoneArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var patch = new PatchDeployment("patch", PatchDeploymentArgs.builder()
* .patchDeploymentId("patch-deploy")
* .instanceFilter(PatchDeploymentInstanceFilterArgs.builder()
* .all(true)
* .build())
* .recurringSchedule(PatchDeploymentRecurringScheduleArgs.builder()
* .timeZone(PatchDeploymentRecurringScheduleTimeZoneArgs.builder()
* .id("America/New_York")
* .build())
* .timeOfDay(PatchDeploymentRecurringScheduleTimeOfDayArgs.builder()
* .hours(0)
* .minutes(30)
* .seconds(30)
* .nanos(20)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* patch:
* type: gcp:osconfig:PatchDeployment
* properties:
* patchDeploymentId: patch-deploy
* instanceFilter:
* all: true
* recurringSchedule:
* timeZone:
* id: America/New_York
* timeOfDay:
* hours: 0
* minutes: 30
* seconds: 30
* nanos: 20
* ```
*
* ### Os Config Patch Deployment Daily Midnight
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const patch = new gcp.osconfig.PatchDeployment("patch", {
* patchDeploymentId: "patch-deploy",
* instanceFilter: {
* all: true,
* },
* recurringSchedule: {
* timeZone: {
* id: "America/New_York",
* },
* timeOfDay: {
* hours: 0,
* minutes: 0,
* seconds: 0,
* nanos: 0,
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* patch = gcp.osconfig.PatchDeployment("patch",
* patch_deployment_id="patch-deploy",
* instance_filter=gcp.osconfig.PatchDeploymentInstanceFilterArgs(
* all=True,
* ),
* recurring_schedule=gcp.osconfig.PatchDeploymentRecurringScheduleArgs(
* time_zone=gcp.osconfig.PatchDeploymentRecurringScheduleTimeZoneArgs(
* id="America/New_York",
* ),
* time_of_day=gcp.osconfig.PatchDeploymentRecurringScheduleTimeOfDayArgs(
* hours=0,
* minutes=0,
* seconds=0,
* nanos=0,
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var patch = new Gcp.OsConfig.PatchDeployment("patch", new()
* {
* PatchDeploymentId = "patch-deploy",
* InstanceFilter = new Gcp.OsConfig.Inputs.PatchDeploymentInstanceFilterArgs
* {
* All = true,
* },
* RecurringSchedule = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleArgs
* {
* TimeZone = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleTimeZoneArgs
* {
* Id = "America/New_York",
* },
* TimeOfDay = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs
* {
* Hours = 0,
* Minutes = 0,
* Seconds = 0,
* Nanos = 0,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/osconfig"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := osconfig.NewPatchDeployment(ctx, "patch", &osconfig.PatchDeploymentArgs{
* PatchDeploymentId: pulumi.String("patch-deploy"),
* InstanceFilter: &osconfig.PatchDeploymentInstanceFilterArgs{
* All: pulumi.Bool(true),
* },
* RecurringSchedule: &osconfig.PatchDeploymentRecurringScheduleArgs{
* TimeZone: &osconfig.PatchDeploymentRecurringScheduleTimeZoneArgs{
* Id: pulumi.String("America/New_York"),
* },
* TimeOfDay: &osconfig.PatchDeploymentRecurringScheduleTimeOfDayArgs{
* Hours: pulumi.Int(0),
* Minutes: pulumi.Int(0),
* Seconds: pulumi.Int(0),
* Nanos: pulumi.Int(0),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.osconfig.PatchDeployment;
* import com.pulumi.gcp.osconfig.PatchDeploymentArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentInstanceFilterArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleTimeZoneArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var patch = new PatchDeployment("patch", PatchDeploymentArgs.builder()
* .patchDeploymentId("patch-deploy")
* .instanceFilter(PatchDeploymentInstanceFilterArgs.builder()
* .all(true)
* .build())
* .recurringSchedule(PatchDeploymentRecurringScheduleArgs.builder()
* .timeZone(PatchDeploymentRecurringScheduleTimeZoneArgs.builder()
* .id("America/New_York")
* .build())
* .timeOfDay(PatchDeploymentRecurringScheduleTimeOfDayArgs.builder()
* .hours(0)
* .minutes(0)
* .seconds(0)
* .nanos(0)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* patch:
* type: gcp:osconfig:PatchDeployment
* properties:
* patchDeploymentId: patch-deploy
* instanceFilter:
* all: true
* recurringSchedule:
* timeZone:
* id: America/New_York
* timeOfDay:
* hours: 0
* minutes: 0
* seconds: 0
* nanos: 0
* ```
*
* ### Os Config Patch Deployment Instance
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const myImage = gcp.compute.getImage({
* family: "debian-11",
* project: "debian-cloud",
* });
* const foobar = new gcp.compute.Instance("foobar", {
* name: "patch-deploy-inst",
* machineType: "e2-medium",
* zone: "us-central1-a",
* canIpForward: false,
* tags: [
* "foo",
* "bar",
* ],
* bootDisk: {
* initializeParams: {
* image: myImage.then(myImage => myImage.selfLink),
* },
* },
* networkInterfaces: [{
* network: "default",
* }],
* metadata: {
* foo: "bar",
* },
* });
* const patch = new gcp.osconfig.PatchDeployment("patch", {
* patchDeploymentId: "patch-deploy",
* instanceFilter: {
* instances: [foobar.id],
* },
* patchConfig: {
* yum: {
* security: true,
* minimal: true,
* excludes: ["bash"],
* },
* },
* recurringSchedule: {
* timeZone: {
* id: "America/New_York",
* },
* timeOfDay: {
* hours: 0,
* minutes: 30,
* seconds: 30,
* nanos: 20,
* },
* monthly: {
* monthDay: 1,
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_image = gcp.compute.get_image(family="debian-11",
* project="debian-cloud")
* foobar = gcp.compute.Instance("foobar",
* name="patch-deploy-inst",
* machine_type="e2-medium",
* zone="us-central1-a",
* can_ip_forward=False,
* tags=[
* "foo",
* "bar",
* ],
* boot_disk=gcp.compute.InstanceBootDiskArgs(
* initialize_params=gcp.compute.InstanceBootDiskInitializeParamsArgs(
* image=my_image.self_link,
* ),
* ),
* network_interfaces=[gcp.compute.InstanceNetworkInterfaceArgs(
* network="default",
* )],
* metadata={
* "foo": "bar",
* })
* patch = gcp.osconfig.PatchDeployment("patch",
* patch_deployment_id="patch-deploy",
* instance_filter=gcp.osconfig.PatchDeploymentInstanceFilterArgs(
* instances=[foobar.id],
* ),
* patch_config=gcp.osconfig.PatchDeploymentPatchConfigArgs(
* yum=gcp.osconfig.PatchDeploymentPatchConfigYumArgs(
* security=True,
* minimal=True,
* excludes=["bash"],
* ),
* ),
* recurring_schedule=gcp.osconfig.PatchDeploymentRecurringScheduleArgs(
* time_zone=gcp.osconfig.PatchDeploymentRecurringScheduleTimeZoneArgs(
* id="America/New_York",
* ),
* time_of_day=gcp.osconfig.PatchDeploymentRecurringScheduleTimeOfDayArgs(
* hours=0,
* minutes=30,
* seconds=30,
* nanos=20,
* ),
* monthly=gcp.osconfig.PatchDeploymentRecurringScheduleMonthlyArgs(
* month_day=1,
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var myImage = Gcp.Compute.GetImage.Invoke(new()
* {
* Family = "debian-11",
* Project = "debian-cloud",
* });
* var foobar = new Gcp.Compute.Instance("foobar", new()
* {
* Name = "patch-deploy-inst",
* MachineType = "e2-medium",
* Zone = "us-central1-a",
* CanIpForward = false,
* Tags = new[]
* {
* "foo",
* "bar",
* },
* BootDisk = new Gcp.Compute.Inputs.InstanceBootDiskArgs
* {
* InitializeParams = new Gcp.Compute.Inputs.InstanceBootDiskInitializeParamsArgs
* {
* Image = myImage.Apply(getImageResult => getImageResult.SelfLink),
* },
* },
* NetworkInterfaces = new[]
* {
* new Gcp.Compute.Inputs.InstanceNetworkInterfaceArgs
* {
* Network = "default",
* },
* },
* Metadata =
* {
* { "foo", "bar" },
* },
* });
* var patch = new Gcp.OsConfig.PatchDeployment("patch", new()
* {
* PatchDeploymentId = "patch-deploy",
* InstanceFilter = new Gcp.OsConfig.Inputs.PatchDeploymentInstanceFilterArgs
* {
* Instances = new[]
* {
* foobar.Id,
* },
* },
* PatchConfig = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigArgs
* {
* Yum = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigYumArgs
* {
* Security = true,
* Minimal = true,
* Excludes = new[]
* {
* "bash",
* },
* },
* },
* RecurringSchedule = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleArgs
* {
* TimeZone = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleTimeZoneArgs
* {
* Id = "America/New_York",
* },
* TimeOfDay = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs
* {
* Hours = 0,
* Minutes = 30,
* Seconds = 30,
* Nanos = 20,
* },
* Monthly = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleMonthlyArgs
* {
* MonthDay = 1,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/osconfig"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* myImage, err := compute.LookupImage(ctx, &compute.LookupImageArgs{
* Family: pulumi.StringRef("debian-11"),
* Project: pulumi.StringRef("debian-cloud"),
* }, nil)
* if err != nil {
* return err
* }
* foobar, err := compute.NewInstance(ctx, "foobar", &compute.InstanceArgs{
* Name: pulumi.String("patch-deploy-inst"),
* MachineType: pulumi.String("e2-medium"),
* Zone: pulumi.String("us-central1-a"),
* CanIpForward: pulumi.Bool(false),
* Tags: pulumi.StringArray{
* pulumi.String("foo"),
* pulumi.String("bar"),
* },
* BootDisk: &compute.InstanceBootDiskArgs{
* InitializeParams: &compute.InstanceBootDiskInitializeParamsArgs{
* Image: pulumi.String(myImage.SelfLink),
* },
* },
* NetworkInterfaces: compute.InstanceNetworkInterfaceArray{
* &compute.InstanceNetworkInterfaceArgs{
* Network: pulumi.String("default"),
* },
* },
* Metadata: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* })
* if err != nil {
* return err
* }
* _, err = osconfig.NewPatchDeployment(ctx, "patch", &osconfig.PatchDeploymentArgs{
* PatchDeploymentId: pulumi.String("patch-deploy"),
* InstanceFilter: &osconfig.PatchDeploymentInstanceFilterArgs{
* Instances: pulumi.StringArray{
* foobar.ID(),
* },
* },
* PatchConfig: &osconfig.PatchDeploymentPatchConfigArgs{
* Yum: &osconfig.PatchDeploymentPatchConfigYumArgs{
* Security: pulumi.Bool(true),
* Minimal: pulumi.Bool(true),
* Excludes: pulumi.StringArray{
* pulumi.String("bash"),
* },
* },
* },
* RecurringSchedule: &osconfig.PatchDeploymentRecurringScheduleArgs{
* TimeZone: &osconfig.PatchDeploymentRecurringScheduleTimeZoneArgs{
* Id: pulumi.String("America/New_York"),
* },
* TimeOfDay: &osconfig.PatchDeploymentRecurringScheduleTimeOfDayArgs{
* Hours: pulumi.Int(0),
* Minutes: pulumi.Int(30),
* Seconds: pulumi.Int(30),
* Nanos: pulumi.Int(20),
* },
* Monthly: &osconfig.PatchDeploymentRecurringScheduleMonthlyArgs{
* MonthDay: pulumi.Int(1),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.ComputeFunctions;
* import com.pulumi.gcp.compute.inputs.GetImageArgs;
* import com.pulumi.gcp.compute.Instance;
* import com.pulumi.gcp.compute.InstanceArgs;
* import com.pulumi.gcp.compute.inputs.InstanceBootDiskArgs;
* import com.pulumi.gcp.compute.inputs.InstanceBootDiskInitializeParamsArgs;
* import com.pulumi.gcp.compute.inputs.InstanceNetworkInterfaceArgs;
* import com.pulumi.gcp.osconfig.PatchDeployment;
* import com.pulumi.gcp.osconfig.PatchDeploymentArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentInstanceFilterArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentPatchConfigArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentPatchConfigYumArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleTimeZoneArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs;
* import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleMonthlyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var myImage = ComputeFunctions.getImage(GetImageArgs.builder()
* .family("debian-11")
* .project("debian-cloud")
* .build());
* var foobar = new Instance("foobar", InstanceArgs.builder()
* .name("patch-deploy-inst")
* .machineType("e2-medium")
* .zone("us-central1-a")
* .canIpForward(false)
* .tags(
* "foo",
* "bar")
* .bootDisk(InstanceBootDiskArgs.builder()
* .initializeParams(InstanceBootDiskInitializeParamsArgs.builder()
* .image(myImage.applyValue(getImageResult -> getImageResult.selfLink()))
* .build())
* .build())
* .networkInterfaces(InstanceNetworkInterfaceArgs.builder()
* .network("default")
* .build())
* .metadata(Map.of("foo", "bar"))
* .build());
* var patch = new PatchDeployment("patch", PatchDeploymentArgs.builder()
* .patchDeploymentId("patch-deploy")
* .instanceFilter(PatchDeploymentInstanceFilterArgs.builder()
* .instances(foobar.id())
* .build())
* .patchConfig(PatchDeploymentPatchConfigArgs.builder()
* .yum(PatchDeploymentPatchConfigYumArgs.builder()
* .security(true)
* .minimal(true)
* .excludes("bash")
* .build())
* .build())
* .recurringSchedule(PatchDeploymentRecurringScheduleArgs.builder()
* .timeZone(PatchDeploymentRecurringScheduleTimeZoneArgs.builder()
* .id("America/New_York")
* .build())
* .timeOfDay(PatchDeploymentRecurringScheduleTimeOfDayArgs.builder()
* .hours(0)
* .minutes(30)
* .seconds(30)
* .nanos(20)
* .build())
* .monthly(PatchDeploymentRecurringScheduleMonthlyArgs.builder()
* .monthDay(1)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foobar:
* type: gcp:compute:Instance
* properties:
* name: patch-deploy-inst
* machineType: e2-medium
* zone: us-central1-a
* canIpForward: false
* tags:
* - foo
* - bar
* bootDisk:
* initializeParams:
* image: ${myImage.selfLink}
* networkInterfaces:
* - network: default
* metadata:
* foo: bar
* patch:
* type: gcp:osconfig:PatchDeployment
* properties:
* patchDeploymentId: patch-deploy
* instanceFilter:
* instances:
* - ${foobar.id}
* patchConfig:
* yum:
* security: true
* minimal: true
* excludes:
* - bash
* recurringSchedule:
* timeZone:
* id: America/New_York
* timeOfDay:
* hours: 0
* minutes: 30
* seconds: 30
* nanos: 20
* monthly:
* monthDay: 1
* variables:
* myImage:
* fn::invoke:
* Function: gcp:compute:getImage
* Arguments:
* family: debian-11
* project: debian-cloud
* ```
*
* ### Os Config Patch Deployment Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const patch = new gcp.osconfig.PatchDeployment("patch", {
* patchDeploymentId: "patch-deploy",
* instanceFilter: {
* groupLabels: [{
* labels: {
* env: "dev",
* app: "web",
* },
* }],
* instanceNamePrefixes: ["test-"],
* zones: [
* "us-central1-a",
* "us-central-1c",
* ],
* },
* patchConfig: {
* migInstancesAllowed: true,
* rebootConfig: "ALWAYS",
* apt: {
* type: "DIST",
* excludes: ["python"],
* },
* yum: {
* security: true,
* minimal: true,
* excludes: ["bash"],
* },
* goo: {
* enabled: true,
* },
* zypper: {
* categories: ["security"],
* },
* windowsUpdate: {
* classifications: [
* "CRITICAL",
* "SECURITY",
* "UPDATE",
* ],
* excludes: ["5012170"],
* },
* preStep: {
* linuxExecStepConfig: {
* allowedSuccessCodes: [
* 0,
* 3,
* ],
* localPath: "/tmp/pre_patch_script.sh",
* },
* windowsExecStepConfig: {
* interpreter: "SHELL",
* allowedSuccessCodes: [
* 0,
* 2,
* ],
* localPath: "C:\\Users\\user\\pre-patch-script.cmd",
* },
* },
* postStep: {
* linuxExecStepConfig: {
* gcsObject: {
* bucket: "my-patch-scripts",
* generationNumber: "1523477886880",
* object: "linux/post_patch_script",
* },
* },
* windowsExecStepConfig: {
* interpreter: "POWERSHELL",
* gcsObject: {
* bucket: "my-patch-scripts",
* generationNumber: "135920493447",
* object: "windows/post_patch_script.ps1",
* },
* },
* },
* },
* duration: "10s",
* recurringSchedule: {
* timeZone: {
* id: "America/New_York",
* },
* timeOfDay: {
* hours: 0,
* minutes: 30,
* seconds: 30,
* nanos: 20,
* },
* monthly: {
* weekDayOfMonth: {
* weekOrdinal: -1,
* dayOfWeek: "TUESDAY",
* dayOffset: 3,
* },
* },
* },
* rollout: {
* mode: "ZONE_BY_ZONE",
* disruptionBudget: {
* fixed: 1,
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* patch = gcp.osconfig.PatchDeployment("patch",
* patch_deployment_id="patch-deploy",
* instance_filter=gcp.osconfig.PatchDeploymentInstanceFilterArgs(
* group_labels=[gcp.osconfig.PatchDeploymentInstanceFilterGroupLabelArgs(
* labels={
* "env": "dev",
* "app": "web",
* },
* )],
* instance_name_prefixes=["test-"],
* zones=[
* "us-central1-a",
* "us-central-1c",
* ],
* ),
* patch_config=gcp.osconfig.PatchDeploymentPatchConfigArgs(
* mig_instances_allowed=True,
* reboot_config="ALWAYS",
* apt=gcp.osconfig.PatchDeploymentPatchConfigAptArgs(
* type="DIST",
* excludes=["python"],
* ),
* yum=gcp.osconfig.PatchDeploymentPatchConfigYumArgs(
* security=True,
* minimal=True,
* excludes=["bash"],
* ),
* goo=gcp.osconfig.PatchDeploymentPatchConfigGooArgs(
* enabled=True,
* ),
* zypper=gcp.osconfig.PatchDeploymentPatchConfigZypperArgs(
* categories=["security"],
* ),
* windows_update=gcp.osconfig.PatchDeploymentPatchConfigWindowsUpdateArgs(
* classifications=[
* "CRITICAL",
* "SECURITY",
* "UPDATE",
* ],
* excludes=["5012170"],
* ),
* pre_step=gcp.osconfig.PatchDeploymentPatchConfigPreStepArgs(
* linux_exec_step_config=gcp.osconfig.PatchDeploymentPatchConfigPreStepLinuxExecStepConfigArgs(
* allowed_success_codes=[
* 0,
* 3,
* ],
* local_path="/tmp/pre_patch_script.sh",
* ),
* windows_exec_step_config=gcp.osconfig.PatchDeploymentPatchConfigPreStepWindowsExecStepConfigArgs(
* interpreter="SHELL",
* allowed_success_codes=[
* 0,
* 2,
* ],
* local_path="C:\\Users\\user\\pre-patch-script.cmd",
* ),
* ),
* post_step=gcp.osconfig.PatchDeploymentPatchConfigPostStepArgs(
* linux_exec_step_config=gcp.osconfig.PatchDeploymentPatchConfigPostStepLinuxExecStepConfigArgs(
* gcs_object=gcp.osconfig.PatchDeploymentPatchConfigPostStepLinuxExecStepConfigGcsObjectArgs(
* bucket="my-patch-scripts",
* generation_number="1523477886880",
* object="linux/post_patch_script",
* ),
* ),
* windows_exec_step_config=gcp.osconfig.PatchDeploymentPatchConfigPostStepWindowsExecStepConfigArgs(
* interpreter="POWERSHELL",
* gcs_object=gcp.osconfig.PatchDeploymentPatchConfigPostStepWindowsExecStepConfigGcsObjectArgs(
* bucket="my-patch-scripts",
* generation_number="135920493447",
* object="windows/post_patch_script.ps1",
* ),
* ),
* ),
* ),
* duration="10s",
* recurring_schedule=gcp.osconfig.PatchDeploymentRecurringScheduleArgs(
* time_zone=gcp.osconfig.PatchDeploymentRecurringScheduleTimeZoneArgs(
* id="America/New_York",
* ),
* time_of_day=gcp.osconfig.PatchDeploymentRecurringScheduleTimeOfDayArgs(
* hours=0,
* minutes=30,
* seconds=30,
* nanos=20,
* ),
* monthly=gcp.osconfig.PatchDeploymentRecurringScheduleMonthlyArgs(
* week_day_of_month=gcp.osconfig.PatchDeploymentRecurringScheduleMonthlyWeekDayOfMonthArgs(
* week_ordinal=-1,
* day_of_week="TUESDAY",
* day_offset=3,
* ),
* ),
* ),
* rollout=gcp.osconfig.PatchDeploymentRolloutArgs(
* mode="ZONE_BY_ZONE",
* disruption_budget=gcp.osconfig.PatchDeploymentRolloutDisruptionBudgetArgs(
* fixed=1,
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var patch = new Gcp.OsConfig.PatchDeployment("patch", new()
* {
* PatchDeploymentId = "patch-deploy",
* InstanceFilter = new Gcp.OsConfig.Inputs.PatchDeploymentInstanceFilterArgs
* {
* GroupLabels = new[]
* {
* new Gcp.OsConfig.Inputs.PatchDeploymentInstanceFilterGroupLabelArgs
* {
* Labels =
* {
* { "env", "dev" },
* { "app", "web" },
* },
* },
* },
* InstanceNamePrefixes = new[]
* {
* "test-",
* },
* Zones = new[]
* {
* "us-central1-a",
* "us-central-1c",
* },
* },
* PatchConfig = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigArgs
* {
* MigInstancesAllowed = true,
* RebootConfig = "ALWAYS",
* Apt = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigAptArgs
* {
* Type = "DIST",
* Excludes = new[]
* {
* "python",
* },
* },
* Yum = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigYumArgs
* {
* Security = true,
* Minimal = true,
* Excludes = new[]
* {
* "bash",
* },
* },
* Goo = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigGooArgs
* {
* Enabled = true,
* },
* Zypper = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigZypperArgs
* {
* Categories = new[]
* {
* "security",
* },
* },
* WindowsUpdate = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigWindowsUpdateArgs
* {
* Classifications = new[]
* {
* "CRITICAL",
* "SECURITY",
* "UPDATE",
* },
* Excludes = new[]
* {
* "5012170",
* },
* },
* PreStep = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigPreStepArgs
* {
* LinuxExecStepConfig = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigPreStepLinuxExecStepConfigArgs
* {
* AllowedSuccessCodes = new[]
* {
* 0,
* 3,
* },
* LocalPath = "/tmp/pre_patch_script.sh",
* },
* WindowsExecStepConfig = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigPreStepWindowsExecStepConfigArgs
* {
* Interpreter = "SHELL",
* AllowedSuccessCodes = new[]
* {
* 0,
* 2,
* },
* LocalPath = "C:\\Users\\user\\pre-patch-script.cmd",
* },
* },
* PostStep = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigPostStepArgs
* {
* LinuxExecStepConfig = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigPostStepLinuxExecStepConfigArgs
* {
* GcsObject = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigPostStepLinuxExecStepConfigGcsObjectArgs
* {
* Bucket = "my-patch-scripts",
* GenerationNumber = "1523477886880",
* Object = "linux/post_patch_script",
* },
* },
* WindowsExecStepConfig = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigPostStepWindowsExecStepConfigArgs
* {
* Interpreter = "POWERSHELL",
* GcsObject = new Gcp.OsConfig.Inputs.PatchDeploymentPatchConfigPostStepWindowsExecStepConfigGcsObjectArgs
* {
* Bucket = "my-patch-scripts",
* GenerationNumber = "135920493447",
* Object = "windows/post_patch_script.ps1",
* },
* },
* },
* },
* Duration = "10s",
* RecurringSchedule = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleArgs
* {
* TimeZone = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleTimeZoneArgs
* {
* Id = "America/New_York",
* },
* TimeOfDay = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs
* {
* Hours = 0,
* Minutes = 30,
* Seconds = 30,
* Nanos = 20,
* },
* Monthly = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleMonthlyArgs
* {
* WeekDayOfMonth = new Gcp.OsConfig.Inputs.PatchDeploymentRecurringScheduleMonthlyWeekDayOfMonthArgs
* {
* WeekOrdinal = -1,
* DayOfWeek = "TUESDAY",
* DayOffset = 3,
* },
* },
* },
* Rollout = new Gcp.OsConfig.Inputs.PatchDeploymentRolloutArgs
* {
* Mode = "ZONE_BY_ZONE",
* DisruptionBudget = new Gcp.OsConfig.Inputs.PatchDeploymentRolloutDisruptionBudgetArgs
* {
* Fixed = 1,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/osconfig"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := osconfig.NewPatchDeployment(ctx, "patch", &osconfig.PatchDeploymentArgs{
* PatchDeploymentId: pulumi.String("patch-deploy"),
* InstanceFilter: &osconfig.PatchDeploymentInstanceFilterArgs{
* GroupLabels: osconfig.PatchDeploymentInstanceFilterGroupLabelArray{
* &osconfig.PatchDeploymentInstanceFilterGroupLabelArgs{
* Labels: pulumi.StringMap{
* "env": pulumi.String("dev"),
* "app": pulumi.String("web"),
* },
* },
* },
* InstanceNamePrefixes: pulumi.StringArray{
* pulumi.String("test-"),
* },
* Zones: pulumi.StringArray{
* pulumi.String("us-central1-a"),
* pulumi.String("us-central-1c"),
* },
* },
* PatchConfig: &osconfig.PatchDeploymentPatchConfigArgs{
* MigInstancesAllowed: pulumi.Bool(true),
* RebootConfig: pulumi.String("ALWAYS"),
* Apt: &osconfig.PatchDeploymentPatchConfigAptArgs{
* Type: pulumi.String("DIST"),
* Excludes: pulumi.StringArray{
* pulumi.String("python"),
* },
* },
* Yum: &osconfig.PatchDeploymentPatchConfigYumArgs{
* Security: pulumi.Bool(true),
* Minimal: pulumi.Bool(true),
* Excludes: pulumi.StringArray{
* pulumi.String("bash"),
* },
* },
* Goo: &osconfig.PatchDeploymentPatchConfigGooArgs{
* Enabled: pulumi.Bool(true),
* },
* Zypper: &osconfig.PatchDeploymentPatchConfigZypperArgs{
* Categories: pulumi.StringArray{
* pulumi.String("security"),
* },
* },
* WindowsUpdate: &osconfig.PatchDeploymentPatchConfigWindowsUpdateArgs{
* Classifications: pulumi.StringArray{
* pulumi.String("CRITICAL"),
* pulumi.String("SECURITY"),
* pulumi.String("UPDATE"),
* },
* Excludes: pulumi.StringArray{
* pulumi.String("5012170"),
* },
* },
* PreStep: &osconfig.PatchDeploymentPatchConfigPreStepArgs{
* LinuxExecStepConfig: &osconfig.PatchDeploymentPatchConfigPreStepLinuxExecStepConfigArgs{
* AllowedSuccessCodes: pulumi.IntArray{
* pulumi.Int(0),
* pulumi.Int(3),
* },
* LocalPath: pulumi.String("/tmp/pre_patch_script.sh"),
* },
* WindowsExecStepConfig: &osconfig.PatchDeploymentPatchConfigPreStepWindowsExecStepConfigArgs{
* Interpreter: pulumi.String("SHELL"),
* AllowedSuccessCodes: pulumi.IntArray{
* pulumi.Int(0),
* pulumi.Int(2),
* },
* LocalPath: pulumi.String("C:\\Users\\user\\pre-patch-script.cmd"),
* },
* },
* PostStep: &osconfig.PatchDeploymentPatchConfigPostStepArgs{
* LinuxExecStepConfig: &osconfig.PatchDeploymentPatchConfigPostStepLinuxExecStepConfigArgs{
* GcsObject: &osconfig.PatchDeploymentPatchConfigPostStepLinuxExecStepConfigGcsObjectArgs{
* Bucket: pulumi.String("my-patch-scripts"),
* GenerationNumber: pulumi.String("1523477886880"),
* Object: pulumi.String("linux/post_patch_script"),
* },
* },
* WindowsExecStepConfig: &osconfig.PatchDeploymentPatchConfigPostStepWindowsExecStepConfigArgs{
* Interpreter: pulumi.String("POWERSHELL"),
* GcsObject: &osconfig.PatchDeploymentPatchConfigPostStepWindowsExecStepConfigGcsObjectArgs{
* Bucket: pulumi.String("my-patch-scripts"),
* GenerationNumber: pulumi.String("135920493447"),
* Object: pulumi.String("windows/post_patch_script.ps1"),
* },
* },
* },
* },
* Duration: pulumi.String("10s"),
* RecurringSchedule: &osconfig.PatchDeploymentRecurringScheduleArgs{
* TimeZone: &osconfig.PatchDeploymentRecurringScheduleTimeZoneArgs{
* Id: pulumi.String("America/New_York"),
* },
* TimeOfDay: &osconfig.PatchDeploymentRecurringScheduleTimeOfDayArgs{
* Hours: pulumi.Int(0),
* Minutes: pulumi.Int(30),
* Seconds: pulumi.Int(30),
* Nanos: pulumi.Int(20),
* },
* Monthly: &osconfig.PatchDeploymentRecurringScheduleMonthlyArgs{
* WeekDayOfMonth: &osconfig.PatchDeploymentRecurringScheduleMonthlyWeekDayOfMonthArgs{
* WeekOrdinal: -1,
* DayOfWeek: pulumi.String("TUESDAY"),
* DayOffset: pulumi.Int(3),
* },
* },
* },
* Rollout: &osconfig.PatchDeploymentRolloutArgs{
* Mode: pulumi.String("ZONE_BY_ZONE"),
* DisruptionBudget: &osconfig.PatchDeploymentRolloutDisruptionBudgetArgs{
* Fixed: pulumi.Int(1),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```yaml
* resources:
* patch:
* type: gcp:osconfig:PatchDeployment
* properties:
* patchDeploymentId: patch-deploy
* instanceFilter:
* groupLabels:
* - labels:
* env: dev
* app: web
* instanceNamePrefixes:
* - test-
* zones:
* - us-central1-a
* - us-central-1c
* patchConfig:
* migInstancesAllowed: true
* rebootConfig: ALWAYS
* apt:
* type: DIST
* excludes:
* - python
* yum:
* security: true
* minimal: true
* excludes:
* - bash
* goo:
* enabled: true
* zypper:
* categories:
* - security
* windowsUpdate:
* classifications:
* - CRITICAL
* - SECURITY
* - UPDATE
* excludes:
* - '5012170'
* preStep:
* linuxExecStepConfig:
* allowedSuccessCodes:
* - 0
* - 3
* localPath: /tmp/pre_patch_script.sh
* windowsExecStepConfig:
* interpreter: SHELL
* allowedSuccessCodes:
* - 0
* - 2
* localPath: C:\Users\user\pre-patch-script.cmd
* postStep:
* linuxExecStepConfig:
* gcsObject:
* bucket: my-patch-scripts
* generationNumber: '1523477886880'
* object: linux/post_patch_script
* windowsExecStepConfig:
* interpreter: POWERSHELL
* gcsObject:
* bucket: my-patch-scripts
* generationNumber: '135920493447'
* object: windows/post_patch_script.ps1
* duration: 10s
* recurringSchedule:
* timeZone:
* id: America/New_York
* timeOfDay:
* hours: 0
* minutes: 30
* seconds: 30
* nanos: 20
* monthly:
* weekDayOfMonth:
* weekOrdinal: -1
* dayOfWeek: TUESDAY
* dayOffset: 3
* rollout:
* mode: ZONE_BY_ZONE
* disruptionBudget:
* fixed: 1
* ```
*
* ## Import
* PatchDeployment can be imported using any of these accepted formats:
* * `projects/{{project}}/patchDeployments/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, PatchDeployment can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:osconfig/patchDeployment:PatchDeployment default projects/{{project}}/patchDeployments/{{name}}
* ```
* ```sh
* $ pulumi import gcp:osconfig/patchDeployment:PatchDeployment default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:osconfig/patchDeployment:PatchDeployment default {{name}}
* ```
*/
public class PatchDeployment internal constructor(
override val javaResource: com.pulumi.gcp.osconfig.PatchDeployment,
) : KotlinCustomResource(javaResource, PatchDeploymentMapper) {
/**
* Time the patch deployment was created. Timestamp is in RFC3339 text format.
* A timestamp in RFC3339 UTC "Zulu" format, accurate to nanoseconds. Example: "2014-10-02T15:01:23.045123456Z".
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* Description of the patch deployment. Length of the description is limited to 1024 characters.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Duration of the patch. After the duration ends, the patch times out. A duration in seconds with up to nine fractional
* digits, terminated by 's'. Example: "3.5s"
*/
public val duration: Output?
get() = javaResource.duration().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* VM instances to patch.
* Structure is documented below.
*/
public val instanceFilter: Output
get() = javaResource.instanceFilter().applyValue({ args0 ->
args0.let({ args0 ->
patchDeploymentInstanceFilterToKotlin(args0)
})
})
/**
* The last time a patch job was started by this deployment. Timestamp is in RFC3339 text format.
* A timestamp in RFC3339 UTC "Zulu" format, accurate to nanoseconds. Example: "2014-10-02T15:01:23.045123456Z".
*/
public val lastExecuteTime: Output
get() = javaResource.lastExecuteTime().applyValue({ args0 -> args0 })
/**
* Unique name for the patch deployment resource in a project.
* The patch deployment name is in the form: projects/{project_id}/patchDeployments/{patchDeploymentId}.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Schedule a one-time execution.
*/
public val oneTimeSchedule: Output?
get() = javaResource.oneTimeSchedule().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> patchDeploymentOneTimeScheduleToKotlin(args0) })
}).orElse(null)
})
/**
* Patch configuration that is applied.
*/
public val patchConfig: Output?
get() = javaResource.patchConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
patchDeploymentPatchConfigToKotlin(args0)
})
}).orElse(null)
})
/**
* A name for the patch deployment in the project. When creating a name the following rules apply:
* * Must contain only lowercase letters, numbers, and hyphens.
* * Must start with a letter.
* * Must be between 1-63 characters.
* * Must end with a number or a letter.
* * Must be unique within the project.
*/
public val patchDeploymentId: Output
get() = javaResource.patchDeploymentId().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* Schedule recurring executions.
*/
public val recurringSchedule: Output?
get() = javaResource.recurringSchedule().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> patchDeploymentRecurringScheduleToKotlin(args0) })
}).orElse(null)
})
/**
* Rollout strategy of the patch job.
*/
public val rollout: Output?
get() = javaResource.rollout().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
patchDeploymentRolloutToKotlin(args0)
})
}).orElse(null)
})
/**
* Time the patch deployment was last updated. Timestamp is in RFC3339 text format.
* A timestamp in RFC3339 UTC "Zulu" format, accurate to nanoseconds. Example: "2014-10-02T15:01:23.045123456Z".
*/
public val updateTime: Output
get() = javaResource.updateTime().applyValue({ args0 -> args0 })
}
public object PatchDeploymentMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.osconfig.PatchDeployment::class == javaResource::class
override fun map(javaResource: Resource): PatchDeployment = PatchDeployment(
javaResource as
com.pulumi.gcp.osconfig.PatchDeployment,
)
}
/**
* @see [PatchDeployment].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [PatchDeployment].
*/
public suspend fun patchDeployment(
name: String,
block: suspend PatchDeploymentResourceBuilder.() -> Unit,
): PatchDeployment {
val builder = PatchDeploymentResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [PatchDeployment].
* @param name The _unique_ name of the resulting resource.
*/
public fun patchDeployment(name: String): PatchDeployment {
val builder = PatchDeploymentResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy