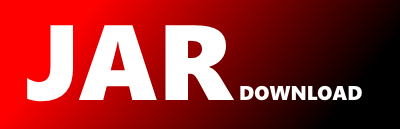
com.pulumi.gcp.osconfig.kotlin.inputs.GuestPoliciesRecipeUpdateStepArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.osconfig.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.osconfig.inputs.GuestPoliciesRecipeUpdateStepArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property archiveExtraction Extracts an archive into the specified directory.
* Structure is documented below.
* @property dpkgInstallation Installs a deb file via dpkg.
* Structure is documented below.
* @property fileCopy Copies a file onto the instance.
* Structure is documented below.
* @property fileExec Executes an artifact or local file.
* Structure is documented below.
* @property msiInstallation Installs an MSI file.
* Structure is documented below.
* @property rpmInstallation Installs an rpm file via the rpm utility.
* Structure is documented below.
* @property scriptRun Runs commands in a shell.
* Structure is documented below.
*/
public data class GuestPoliciesRecipeUpdateStepArgs(
public val archiveExtraction: Output? = null,
public val dpkgInstallation: Output? = null,
public val fileCopy: Output? = null,
public val fileExec: Output? = null,
public val msiInstallation: Output? = null,
public val rpmInstallation: Output? = null,
public val scriptRun: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.osconfig.inputs.GuestPoliciesRecipeUpdateStepArgs =
com.pulumi.gcp.osconfig.inputs.GuestPoliciesRecipeUpdateStepArgs.builder()
.archiveExtraction(archiveExtraction?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.dpkgInstallation(dpkgInstallation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.fileCopy(fileCopy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.fileExec(fileExec?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.msiInstallation(msiInstallation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.rpmInstallation(rpmInstallation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.scriptRun(scriptRun?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [GuestPoliciesRecipeUpdateStepArgs].
*/
@PulumiTagMarker
public class GuestPoliciesRecipeUpdateStepArgsBuilder internal constructor() {
private var archiveExtraction: Output? = null
private var dpkgInstallation: Output? = null
private var fileCopy: Output? = null
private var fileExec: Output? = null
private var msiInstallation: Output? = null
private var rpmInstallation: Output? = null
private var scriptRun: Output? = null
/**
* @param value Extracts an archive into the specified directory.
* Structure is documented below.
*/
@JvmName("mdjbxlvuhmtfwmul")
public suspend fun archiveExtraction(`value`: Output) {
this.archiveExtraction = value
}
/**
* @param value Installs a deb file via dpkg.
* Structure is documented below.
*/
@JvmName("godnknkpgsivxnie")
public suspend fun dpkgInstallation(`value`: Output) {
this.dpkgInstallation = value
}
/**
* @param value Copies a file onto the instance.
* Structure is documented below.
*/
@JvmName("ivriyphcogoxkelx")
public suspend fun fileCopy(`value`: Output) {
this.fileCopy = value
}
/**
* @param value Executes an artifact or local file.
* Structure is documented below.
*/
@JvmName("mpnyqfcuuyjyyuyp")
public suspend fun fileExec(`value`: Output) {
this.fileExec = value
}
/**
* @param value Installs an MSI file.
* Structure is documented below.
*/
@JvmName("uoervkenkbtyfvdq")
public suspend fun msiInstallation(`value`: Output) {
this.msiInstallation = value
}
/**
* @param value Installs an rpm file via the rpm utility.
* Structure is documented below.
*/
@JvmName("cksxxeiypywcedpf")
public suspend fun rpmInstallation(`value`: Output) {
this.rpmInstallation = value
}
/**
* @param value Runs commands in a shell.
* Structure is documented below.
*/
@JvmName("xkgcscsehsakjsyt")
public suspend fun scriptRun(`value`: Output) {
this.scriptRun = value
}
/**
* @param value Extracts an archive into the specified directory.
* Structure is documented below.
*/
@JvmName("dgjmdjrihchthtcu")
public suspend fun archiveExtraction(`value`: GuestPoliciesRecipeUpdateStepArchiveExtractionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.archiveExtraction = mapped
}
/**
* @param argument Extracts an archive into the specified directory.
* Structure is documented below.
*/
@JvmName("ekpkikahfxyfvppy")
public suspend fun archiveExtraction(argument: suspend GuestPoliciesRecipeUpdateStepArchiveExtractionArgsBuilder.() -> Unit) {
val toBeMapped = GuestPoliciesRecipeUpdateStepArchiveExtractionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.archiveExtraction = mapped
}
/**
* @param value Installs a deb file via dpkg.
* Structure is documented below.
*/
@JvmName("jadjrgjwvghiyefl")
public suspend fun dpkgInstallation(`value`: GuestPoliciesRecipeUpdateStepDpkgInstallationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dpkgInstallation = mapped
}
/**
* @param argument Installs a deb file via dpkg.
* Structure is documented below.
*/
@JvmName("sqvxnphfcykxgqra")
public suspend fun dpkgInstallation(argument: suspend GuestPoliciesRecipeUpdateStepDpkgInstallationArgsBuilder.() -> Unit) {
val toBeMapped = GuestPoliciesRecipeUpdateStepDpkgInstallationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dpkgInstallation = mapped
}
/**
* @param value Copies a file onto the instance.
* Structure is documented below.
*/
@JvmName("wefsmdwytxgkypeg")
public suspend fun fileCopy(`value`: GuestPoliciesRecipeUpdateStepFileCopyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fileCopy = mapped
}
/**
* @param argument Copies a file onto the instance.
* Structure is documented below.
*/
@JvmName("bpfamefdbygkpogf")
public suspend fun fileCopy(argument: suspend GuestPoliciesRecipeUpdateStepFileCopyArgsBuilder.() -> Unit) {
val toBeMapped = GuestPoliciesRecipeUpdateStepFileCopyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.fileCopy = mapped
}
/**
* @param value Executes an artifact or local file.
* Structure is documented below.
*/
@JvmName("loqtwncfaisxevie")
public suspend fun fileExec(`value`: GuestPoliciesRecipeUpdateStepFileExecArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fileExec = mapped
}
/**
* @param argument Executes an artifact or local file.
* Structure is documented below.
*/
@JvmName("nuoqrgsximfyrkxx")
public suspend fun fileExec(argument: suspend GuestPoliciesRecipeUpdateStepFileExecArgsBuilder.() -> Unit) {
val toBeMapped = GuestPoliciesRecipeUpdateStepFileExecArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.fileExec = mapped
}
/**
* @param value Installs an MSI file.
* Structure is documented below.
*/
@JvmName("yxesuwlrgrhdywjx")
public suspend fun msiInstallation(`value`: GuestPoliciesRecipeUpdateStepMsiInstallationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.msiInstallation = mapped
}
/**
* @param argument Installs an MSI file.
* Structure is documented below.
*/
@JvmName("asoxuatkyihdtctb")
public suspend fun msiInstallation(argument: suspend GuestPoliciesRecipeUpdateStepMsiInstallationArgsBuilder.() -> Unit) {
val toBeMapped = GuestPoliciesRecipeUpdateStepMsiInstallationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.msiInstallation = mapped
}
/**
* @param value Installs an rpm file via the rpm utility.
* Structure is documented below.
*/
@JvmName("monkjmeplrgklkdf")
public suspend fun rpmInstallation(`value`: GuestPoliciesRecipeUpdateStepRpmInstallationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rpmInstallation = mapped
}
/**
* @param argument Installs an rpm file via the rpm utility.
* Structure is documented below.
*/
@JvmName("iecrlvkkdnoulwnv")
public suspend fun rpmInstallation(argument: suspend GuestPoliciesRecipeUpdateStepRpmInstallationArgsBuilder.() -> Unit) {
val toBeMapped = GuestPoliciesRecipeUpdateStepRpmInstallationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rpmInstallation = mapped
}
/**
* @param value Runs commands in a shell.
* Structure is documented below.
*/
@JvmName("kqlghmfpkpybuigf")
public suspend fun scriptRun(`value`: GuestPoliciesRecipeUpdateStepScriptRunArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scriptRun = mapped
}
/**
* @param argument Runs commands in a shell.
* Structure is documented below.
*/
@JvmName("phylbcsmnywmuvdx")
public suspend fun scriptRun(argument: suspend GuestPoliciesRecipeUpdateStepScriptRunArgsBuilder.() -> Unit) {
val toBeMapped = GuestPoliciesRecipeUpdateStepScriptRunArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.scriptRun = mapped
}
internal fun build(): GuestPoliciesRecipeUpdateStepArgs = GuestPoliciesRecipeUpdateStepArgs(
archiveExtraction = archiveExtraction,
dpkgInstallation = dpkgInstallation,
fileCopy = fileCopy,
fileExec = fileExec,
msiInstallation = msiInstallation,
rpmInstallation = rpmInstallation,
scriptRun = scriptRun,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy