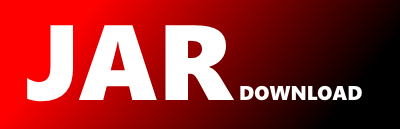
com.pulumi.gcp.osconfig.kotlin.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.osconfig.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.osconfig.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property exec Exec resource Structure is
* documented below.
* @property file File resource Structure is
* documented below.
* @property id The id of the resource with the following restrictions:
* * Must contain only lowercase letters, numbers, and hyphens.
* * Must start with a letter.
* * Must be between 1-63 characters.
* * Must end with a number or a letter.
* * Must be unique within the OS policy.
* @property pkg Package resource Structure is
* documented below.
* @property repository Package repository resource Structure is
* documented below.
*/
public data class OsPolicyAssignmentOsPolicyResourceGroupResourceArgs(
public val exec: Output? = null,
public val `file`: Output? = null,
public val id: Output,
public val pkg: Output? = null,
public val repository: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.osconfig.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourceArgs =
com.pulumi.gcp.osconfig.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourceArgs.builder()
.exec(exec?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.`file`(`file`?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.id(id.applyValue({ args0 -> args0 }))
.pkg(pkg?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.repository(repository?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [OsPolicyAssignmentOsPolicyResourceGroupResourceArgs].
*/
@PulumiTagMarker
public class OsPolicyAssignmentOsPolicyResourceGroupResourceArgsBuilder internal constructor() {
private var exec: Output? = null
private var `file`: Output? = null
private var id: Output? = null
private var pkg: Output? = null
private var repository: Output? =
null
/**
* @param value Exec resource Structure is
* documented below.
*/
@JvmName("byuquxjtmfmrvpbh")
public suspend fun exec(`value`: Output) {
this.exec = value
}
/**
* @param value File resource Structure is
* documented below.
*/
@JvmName("xcmekqfxxkuvijmv")
public suspend fun `file`(`value`: Output) {
this.`file` = value
}
/**
* @param value The id of the resource with the following restrictions:
* * Must contain only lowercase letters, numbers, and hyphens.
* * Must start with a letter.
* * Must be between 1-63 characters.
* * Must end with a number or a letter.
* * Must be unique within the OS policy.
*/
@JvmName("dmeemspbldiodrdn")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value Package resource Structure is
* documented below.
*/
@JvmName("ewpgwfyfrsivnldx")
public suspend fun pkg(`value`: Output) {
this.pkg = value
}
/**
* @param value Package repository resource Structure is
* documented below.
*/
@JvmName("srxsoqlnxkpehvtt")
public suspend fun repository(`value`: Output) {
this.repository = value
}
/**
* @param value Exec resource Structure is
* documented below.
*/
@JvmName("iavyeovgcvpsnlkq")
public suspend fun exec(`value`: OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.exec = mapped
}
/**
* @param argument Exec resource Structure is
* documented below.
*/
@JvmName("oprfgdrfxajxveny")
public suspend fun exec(argument: suspend OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgsBuilder.() -> Unit) {
val toBeMapped = OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.exec = mapped
}
/**
* @param value File resource Structure is
* documented below.
*/
@JvmName("jpacdesqcakwdrgp")
public suspend fun `file`(`value`: OsPolicyAssignmentOsPolicyResourceGroupResourceFileArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`file` = mapped
}
/**
* @param argument File resource Structure is
* documented below.
*/
@JvmName("prifioearwhbfvtk")
public suspend fun `file`(argument: suspend OsPolicyAssignmentOsPolicyResourceGroupResourceFileArgsBuilder.() -> Unit) {
val toBeMapped = OsPolicyAssignmentOsPolicyResourceGroupResourceFileArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.`file` = mapped
}
/**
* @param value The id of the resource with the following restrictions:
* * Must contain only lowercase letters, numbers, and hyphens.
* * Must start with a letter.
* * Must be between 1-63 characters.
* * Must end with a number or a letter.
* * Must be unique within the OS policy.
*/
@JvmName("hkmrrtusgkaslgty")
public suspend fun id(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value Package resource Structure is
* documented below.
*/
@JvmName("iofyjmrmwaaqyijb")
public suspend fun pkg(`value`: OsPolicyAssignmentOsPolicyResourceGroupResourcePkgArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pkg = mapped
}
/**
* @param argument Package resource Structure is
* documented below.
*/
@JvmName("qivihmsdtmqbtnru")
public suspend fun pkg(argument: suspend OsPolicyAssignmentOsPolicyResourceGroupResourcePkgArgsBuilder.() -> Unit) {
val toBeMapped = OsPolicyAssignmentOsPolicyResourceGroupResourcePkgArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.pkg = mapped
}
/**
* @param value Package repository resource Structure is
* documented below.
*/
@JvmName("hqekytnwrfpidaeq")
public suspend fun repository(`value`: OsPolicyAssignmentOsPolicyResourceGroupResourceRepositoryArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.repository = mapped
}
/**
* @param argument Package repository resource Structure is
* documented below.
*/
@JvmName("lmfibgkpgejoqpxy")
public suspend fun repository(argument: suspend OsPolicyAssignmentOsPolicyResourceGroupResourceRepositoryArgsBuilder.() -> Unit) {
val toBeMapped =
OsPolicyAssignmentOsPolicyResourceGroupResourceRepositoryArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.repository = mapped
}
internal fun build(): OsPolicyAssignmentOsPolicyResourceGroupResourceArgs =
OsPolicyAssignmentOsPolicyResourceGroupResourceArgs(
exec = exec,
`file` = `file`,
id = id ?: throw PulumiNullFieldException("id"),
pkg = pkg,
repository = repository,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy