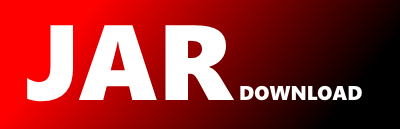
com.pulumi.gcp.osconfig.kotlin.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.osconfig.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.osconfig.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property enforce What to run to bring this resource into the desired
* state. An exit code of 100 indicates "success", any other exit code
* indicates a failure running enforce. Structure is
* documented below.
* @property validate What to run to validate this resource is in the
* desired state. An exit code of 100 indicates "in desired state", and exit
* code of 101 indicates "not in desired state". Any other exit code indicates
* a failure running validate. Structure is
* documented below.
*/
public data class OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgs(
public val enforce: Output? =
null,
public val validate: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.osconfig.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgs =
com.pulumi.gcp.osconfig.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgs.builder()
.enforce(enforce?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.validate(validate.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgs].
*/
@PulumiTagMarker
public class OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgsBuilder internal constructor() {
private var enforce: Output? =
null
private var validate: Output? =
null
/**
* @param value What to run to bring this resource into the desired
* state. An exit code of 100 indicates "success", any other exit code
* indicates a failure running enforce. Structure is
* documented below.
*/
@JvmName("xstpekswnunubxct")
public suspend fun enforce(`value`: Output) {
this.enforce = value
}
/**
* @param value What to run to validate this resource is in the
* desired state. An exit code of 100 indicates "in desired state", and exit
* code of 101 indicates "not in desired state". Any other exit code indicates
* a failure running validate. Structure is
* documented below.
*/
@JvmName("kybigbtcefxhiepp")
public suspend fun validate(`value`: Output) {
this.validate = value
}
/**
* @param value What to run to bring this resource into the desired
* state. An exit code of 100 indicates "success", any other exit code
* indicates a failure running enforce. Structure is
* documented below.
*/
@JvmName("pvxnmufyrdbeifoy")
public suspend fun enforce(`value`: OsPolicyAssignmentOsPolicyResourceGroupResourceExecEnforceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enforce = mapped
}
/**
* @param argument What to run to bring this resource into the desired
* state. An exit code of 100 indicates "success", any other exit code
* indicates a failure running enforce. Structure is
* documented below.
*/
@JvmName("kxpnoyeyuyygqajv")
public suspend fun enforce(argument: suspend OsPolicyAssignmentOsPolicyResourceGroupResourceExecEnforceArgsBuilder.() -> Unit) {
val toBeMapped =
OsPolicyAssignmentOsPolicyResourceGroupResourceExecEnforceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.enforce = mapped
}
/**
* @param value What to run to validate this resource is in the
* desired state. An exit code of 100 indicates "in desired state", and exit
* code of 101 indicates "not in desired state". Any other exit code indicates
* a failure running validate. Structure is
* documented below.
*/
@JvmName("hsyxnhnlpuqkprca")
public suspend fun validate(`value`: OsPolicyAssignmentOsPolicyResourceGroupResourceExecValidateArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.validate = mapped
}
/**
* @param argument What to run to validate this resource is in the
* desired state. An exit code of 100 indicates "in desired state", and exit
* code of 101 indicates "not in desired state". Any other exit code indicates
* a failure running validate. Structure is
* documented below.
*/
@JvmName("wknxohjfrwoabegk")
public suspend fun validate(argument: suspend OsPolicyAssignmentOsPolicyResourceGroupResourceExecValidateArgsBuilder.() -> Unit) {
val toBeMapped =
OsPolicyAssignmentOsPolicyResourceGroupResourceExecValidateArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.validate = mapped
}
internal fun build(): OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgs =
OsPolicyAssignmentOsPolicyResourceGroupResourceExecArgs(
enforce = enforce,
validate = validate ?: throw PulumiNullFieldException("validate"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy