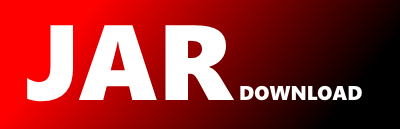
com.pulumi.gcp.osconfig.kotlin.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.osconfig.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.osconfig.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property allowInsecure Defaults to false. When false, files are
* subject to validations based on the file type: Remote: A checksum must be
* specified. Cloud Storage: An object generation number must be specified.
* @property gcs A Cloud Storage object. Structure is
* documented below.
* @property localPath A local path within the VM to use.
* @property remote A generic remote file. Structure is
* documented below.
*/
public data class OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceArgs(
public val allowInsecure: Output? = null,
public val gcs: Output? =
null,
public val localPath: Output? = null,
public val remote: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.osconfig.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceArgs =
com.pulumi.gcp.osconfig.inputs.OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceArgs.builder()
.allowInsecure(allowInsecure?.applyValue({ args0 -> args0 }))
.gcs(gcs?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.localPath(localPath?.applyValue({ args0 -> args0 }))
.remote(remote?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceArgs].
*/
@PulumiTagMarker
public class OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceArgsBuilder internal constructor() {
private var allowInsecure: Output? = null
private var gcs: Output? =
null
private var localPath: Output? = null
private var remote: Output? =
null
/**
* @param value Defaults to false. When false, files are
* subject to validations based on the file type: Remote: A checksum must be
* specified. Cloud Storage: An object generation number must be specified.
*/
@JvmName("gumgkbrrvjltojpa")
public suspend fun allowInsecure(`value`: Output) {
this.allowInsecure = value
}
/**
* @param value A Cloud Storage object. Structure is
* documented below.
*/
@JvmName("wgcnasmenytbteaa")
public suspend fun gcs(`value`: Output) {
this.gcs = value
}
/**
* @param value A local path within the VM to use.
*/
@JvmName("bmlhpeyvubqisegq")
public suspend fun localPath(`value`: Output) {
this.localPath = value
}
/**
* @param value A generic remote file. Structure is
* documented below.
*/
@JvmName("yokhlpqcvcxavpuy")
public suspend fun remote(`value`: Output) {
this.remote = value
}
/**
* @param value Defaults to false. When false, files are
* subject to validations based on the file type: Remote: A checksum must be
* specified. Cloud Storage: An object generation number must be specified.
*/
@JvmName("sbsqsittxtmrwwux")
public suspend fun allowInsecure(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowInsecure = mapped
}
/**
* @param value A Cloud Storage object. Structure is
* documented below.
*/
@JvmName("urspakavxbquaadn")
public suspend fun gcs(`value`: OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceGcsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcs = mapped
}
/**
* @param argument A Cloud Storage object. Structure is
* documented below.
*/
@JvmName("crxfqdemdshrcgas")
public suspend fun gcs(argument: suspend OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceGcsArgsBuilder.() -> Unit) {
val toBeMapped =
OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceGcsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.gcs = mapped
}
/**
* @param value A local path within the VM to use.
*/
@JvmName("npemfpsrtlinqmou")
public suspend fun localPath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.localPath = mapped
}
/**
* @param value A generic remote file. Structure is
* documented below.
*/
@JvmName("sotkkyibxcxdkjbf")
public suspend fun remote(`value`: OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceRemoteArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.remote = mapped
}
/**
* @param argument A generic remote file. Structure is
* documented below.
*/
@JvmName("mfelrhdxeymnrvkt")
public suspend fun remote(argument: suspend OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceRemoteArgsBuilder.() -> Unit) {
val toBeMapped =
OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceRemoteArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.remote = mapped
}
internal fun build(): OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceArgs =
OsPolicyAssignmentOsPolicyResourceGroupResourcePkgDebSourceArgs(
allowInsecure = allowInsecure,
gcs = gcs,
localPath = localPath,
remote = remote,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy