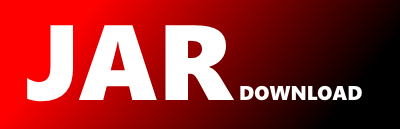
com.pulumi.gcp.osconfig.kotlin.inputs.PatchDeploymentPatchConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.osconfig.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.osconfig.inputs.PatchDeploymentPatchConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property apt Apt update settings. Use this setting to override the default apt patch rules.
* Structure is documented below.
* @property goo goo update settings. Use this setting to override the default goo patch rules.
* Structure is documented below.
* @property migInstancesAllowed Allows the patch job to run on Managed instance groups (MIGs).
* @property postStep The ExecStep to run after the patch update.
* Structure is documented below.
* @property preStep The ExecStep to run before the patch update.
* Structure is documented below.
* @property rebootConfig Post-patch reboot settings.
* Possible values are: `DEFAULT`, `ALWAYS`, `NEVER`.
* @property windowsUpdate Windows update settings. Use this setting to override the default Windows patch rules.
* Structure is documented below.
* @property yum Yum update settings. Use this setting to override the default yum patch rules.
* Structure is documented below.
* @property zypper zypper update settings. Use this setting to override the default zypper patch rules.
* Structure is documented below.
*/
public data class PatchDeploymentPatchConfigArgs(
public val apt: Output? = null,
public val goo: Output? = null,
public val migInstancesAllowed: Output? = null,
public val postStep: Output? = null,
public val preStep: Output? = null,
public val rebootConfig: Output? = null,
public val windowsUpdate: Output? = null,
public val yum: Output? = null,
public val zypper: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.osconfig.inputs.PatchDeploymentPatchConfigArgs =
com.pulumi.gcp.osconfig.inputs.PatchDeploymentPatchConfigArgs.builder()
.apt(apt?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.goo(goo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.migInstancesAllowed(migInstancesAllowed?.applyValue({ args0 -> args0 }))
.postStep(postStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.preStep(preStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.rebootConfig(rebootConfig?.applyValue({ args0 -> args0 }))
.windowsUpdate(windowsUpdate?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.yum(yum?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.zypper(zypper?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [PatchDeploymentPatchConfigArgs].
*/
@PulumiTagMarker
public class PatchDeploymentPatchConfigArgsBuilder internal constructor() {
private var apt: Output? = null
private var goo: Output? = null
private var migInstancesAllowed: Output? = null
private var postStep: Output? = null
private var preStep: Output? = null
private var rebootConfig: Output? = null
private var windowsUpdate: Output? = null
private var yum: Output? = null
private var zypper: Output? = null
/**
* @param value Apt update settings. Use this setting to override the default apt patch rules.
* Structure is documented below.
*/
@JvmName("lrlobgohyhkffjet")
public suspend fun apt(`value`: Output) {
this.apt = value
}
/**
* @param value goo update settings. Use this setting to override the default goo patch rules.
* Structure is documented below.
*/
@JvmName("veulnirmiamjimmc")
public suspend fun goo(`value`: Output) {
this.goo = value
}
/**
* @param value Allows the patch job to run on Managed instance groups (MIGs).
*/
@JvmName("cfbkyhoptgprepin")
public suspend fun migInstancesAllowed(`value`: Output) {
this.migInstancesAllowed = value
}
/**
* @param value The ExecStep to run after the patch update.
* Structure is documented below.
*/
@JvmName("vlayvoybkoibtodx")
public suspend fun postStep(`value`: Output) {
this.postStep = value
}
/**
* @param value The ExecStep to run before the patch update.
* Structure is documented below.
*/
@JvmName("naswgsohlsafdfpm")
public suspend fun preStep(`value`: Output) {
this.preStep = value
}
/**
* @param value Post-patch reboot settings.
* Possible values are: `DEFAULT`, `ALWAYS`, `NEVER`.
*/
@JvmName("kjepxsdtjfluayvk")
public suspend fun rebootConfig(`value`: Output) {
this.rebootConfig = value
}
/**
* @param value Windows update settings. Use this setting to override the default Windows patch rules.
* Structure is documented below.
*/
@JvmName("hnftfcwrrvlldovt")
public suspend fun windowsUpdate(`value`: Output) {
this.windowsUpdate = value
}
/**
* @param value Yum update settings. Use this setting to override the default yum patch rules.
* Structure is documented below.
*/
@JvmName("irngxshcmdlnopgv")
public suspend fun yum(`value`: Output) {
this.yum = value
}
/**
* @param value zypper update settings. Use this setting to override the default zypper patch rules.
* Structure is documented below.
*/
@JvmName("stajgnraeteedhxx")
public suspend fun zypper(`value`: Output) {
this.zypper = value
}
/**
* @param value Apt update settings. Use this setting to override the default apt patch rules.
* Structure is documented below.
*/
@JvmName("kvqbjhmexbcrkwie")
public suspend fun apt(`value`: PatchDeploymentPatchConfigAptArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apt = mapped
}
/**
* @param argument Apt update settings. Use this setting to override the default apt patch rules.
* Structure is documented below.
*/
@JvmName("xfmxomigpfrbhxbu")
public suspend fun apt(argument: suspend PatchDeploymentPatchConfigAptArgsBuilder.() -> Unit) {
val toBeMapped = PatchDeploymentPatchConfigAptArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.apt = mapped
}
/**
* @param value goo update settings. Use this setting to override the default goo patch rules.
* Structure is documented below.
*/
@JvmName("xpbcmekmfookmueh")
public suspend fun goo(`value`: PatchDeploymentPatchConfigGooArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.goo = mapped
}
/**
* @param argument goo update settings. Use this setting to override the default goo patch rules.
* Structure is documented below.
*/
@JvmName("dtnioxiitqbagyka")
public suspend fun goo(argument: suspend PatchDeploymentPatchConfigGooArgsBuilder.() -> Unit) {
val toBeMapped = PatchDeploymentPatchConfigGooArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.goo = mapped
}
/**
* @param value Allows the patch job to run on Managed instance groups (MIGs).
*/
@JvmName("qtdsnqrjrujilvid")
public suspend fun migInstancesAllowed(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.migInstancesAllowed = mapped
}
/**
* @param value The ExecStep to run after the patch update.
* Structure is documented below.
*/
@JvmName("qjgeierfnbvxiuug")
public suspend fun postStep(`value`: PatchDeploymentPatchConfigPostStepArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postStep = mapped
}
/**
* @param argument The ExecStep to run after the patch update.
* Structure is documented below.
*/
@JvmName("acueyfcspgmmumoo")
public suspend fun postStep(argument: suspend PatchDeploymentPatchConfigPostStepArgsBuilder.() -> Unit) {
val toBeMapped = PatchDeploymentPatchConfigPostStepArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.postStep = mapped
}
/**
* @param value The ExecStep to run before the patch update.
* Structure is documented below.
*/
@JvmName("exvuppyiytsinfwh")
public suspend fun preStep(`value`: PatchDeploymentPatchConfigPreStepArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preStep = mapped
}
/**
* @param argument The ExecStep to run before the patch update.
* Structure is documented below.
*/
@JvmName("qulbqsdwamnclgfg")
public suspend fun preStep(argument: suspend PatchDeploymentPatchConfigPreStepArgsBuilder.() -> Unit) {
val toBeMapped = PatchDeploymentPatchConfigPreStepArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.preStep = mapped
}
/**
* @param value Post-patch reboot settings.
* Possible values are: `DEFAULT`, `ALWAYS`, `NEVER`.
*/
@JvmName("vphhjhrolnnpeyhy")
public suspend fun rebootConfig(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rebootConfig = mapped
}
/**
* @param value Windows update settings. Use this setting to override the default Windows patch rules.
* Structure is documented below.
*/
@JvmName("csbpwgdcsvefugeg")
public suspend fun windowsUpdate(`value`: PatchDeploymentPatchConfigWindowsUpdateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.windowsUpdate = mapped
}
/**
* @param argument Windows update settings. Use this setting to override the default Windows patch rules.
* Structure is documented below.
*/
@JvmName("wbxygahubneivkmx")
public suspend fun windowsUpdate(argument: suspend PatchDeploymentPatchConfigWindowsUpdateArgsBuilder.() -> Unit) {
val toBeMapped = PatchDeploymentPatchConfigWindowsUpdateArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.windowsUpdate = mapped
}
/**
* @param value Yum update settings. Use this setting to override the default yum patch rules.
* Structure is documented below.
*/
@JvmName("eauivfsihviypdjh")
public suspend fun yum(`value`: PatchDeploymentPatchConfigYumArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.yum = mapped
}
/**
* @param argument Yum update settings. Use this setting to override the default yum patch rules.
* Structure is documented below.
*/
@JvmName("prujmddjenpwodln")
public suspend fun yum(argument: suspend PatchDeploymentPatchConfigYumArgsBuilder.() -> Unit) {
val toBeMapped = PatchDeploymentPatchConfigYumArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.yum = mapped
}
/**
* @param value zypper update settings. Use this setting to override the default zypper patch rules.
* Structure is documented below.
*/
@JvmName("bhxtnfmdlqvclprp")
public suspend fun zypper(`value`: PatchDeploymentPatchConfigZypperArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.zypper = mapped
}
/**
* @param argument zypper update settings. Use this setting to override the default zypper patch rules.
* Structure is documented below.
*/
@JvmName("wdmyxyfbtjdhudhj")
public suspend fun zypper(argument: suspend PatchDeploymentPatchConfigZypperArgsBuilder.() -> Unit) {
val toBeMapped = PatchDeploymentPatchConfigZypperArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.zypper = mapped
}
internal fun build(): PatchDeploymentPatchConfigArgs = PatchDeploymentPatchConfigArgs(
apt = apt,
goo = goo,
migInstancesAllowed = migInstancesAllowed,
postStep = postStep,
preStep = preStep,
rebootConfig = rebootConfig,
windowsUpdate = windowsUpdate,
yum = yum,
zypper = zypper,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy