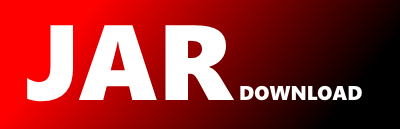
com.pulumi.gcp.osconfig.kotlin.inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.osconfig.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property hours Hours of day in 24 hour format. Should be from 0 to 23.
* An API may choose to allow the value "24:00:00" for scenarios like business closing time.
* @property minutes Minutes of hour of day. Must be from 0 to 59.
* @property nanos Fractions of seconds in nanoseconds. Must be from 0 to 999,999,999.
* @property seconds Seconds of minutes of the time. Must normally be from 0 to 59. An API may allow the value 60 if it allows leap-seconds.
*/
public data class PatchDeploymentRecurringScheduleTimeOfDayArgs(
public val hours: Output? = null,
public val minutes: Output? = null,
public val nanos: Output? = null,
public val seconds: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs =
com.pulumi.gcp.osconfig.inputs.PatchDeploymentRecurringScheduleTimeOfDayArgs.builder()
.hours(hours?.applyValue({ args0 -> args0 }))
.minutes(minutes?.applyValue({ args0 -> args0 }))
.nanos(nanos?.applyValue({ args0 -> args0 }))
.seconds(seconds?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PatchDeploymentRecurringScheduleTimeOfDayArgs].
*/
@PulumiTagMarker
public class PatchDeploymentRecurringScheduleTimeOfDayArgsBuilder internal constructor() {
private var hours: Output? = null
private var minutes: Output? = null
private var nanos: Output? = null
private var seconds: Output? = null
/**
* @param value Hours of day in 24 hour format. Should be from 0 to 23.
* An API may choose to allow the value "24:00:00" for scenarios like business closing time.
*/
@JvmName("mfwemdrpbixtsqoy")
public suspend fun hours(`value`: Output) {
this.hours = value
}
/**
* @param value Minutes of hour of day. Must be from 0 to 59.
*/
@JvmName("ipncohqsfkswsivk")
public suspend fun minutes(`value`: Output) {
this.minutes = value
}
/**
* @param value Fractions of seconds in nanoseconds. Must be from 0 to 999,999,999.
*/
@JvmName("swsngpplpfsgedxo")
public suspend fun nanos(`value`: Output) {
this.nanos = value
}
/**
* @param value Seconds of minutes of the time. Must normally be from 0 to 59. An API may allow the value 60 if it allows leap-seconds.
*/
@JvmName("yarahqfokoojbgep")
public suspend fun seconds(`value`: Output) {
this.seconds = value
}
/**
* @param value Hours of day in 24 hour format. Should be from 0 to 23.
* An API may choose to allow the value "24:00:00" for scenarios like business closing time.
*/
@JvmName("bkiykydfcrvuvtdi")
public suspend fun hours(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hours = mapped
}
/**
* @param value Minutes of hour of day. Must be from 0 to 59.
*/
@JvmName("skmarocrfqkarmdb")
public suspend fun minutes(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minutes = mapped
}
/**
* @param value Fractions of seconds in nanoseconds. Must be from 0 to 999,999,999.
*/
@JvmName("omdppalsxaiaxsey")
public suspend fun nanos(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nanos = mapped
}
/**
* @param value Seconds of minutes of the time. Must normally be from 0 to 59. An API may allow the value 60 if it allows leap-seconds.
*/
@JvmName("feslsawehfbegrrf")
public suspend fun seconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.seconds = mapped
}
internal fun build(): PatchDeploymentRecurringScheduleTimeOfDayArgs =
PatchDeploymentRecurringScheduleTimeOfDayArgs(
hours = hours,
minutes = minutes,
nanos = nanos,
seconds = seconds,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy