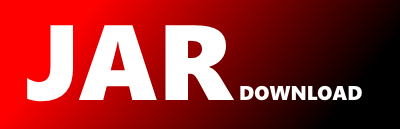
com.pulumi.gcp.parallelstore.kotlin.Instance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.parallelstore.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [Instance].
*/
@PulumiTagMarker
public class InstanceResourceBuilder internal constructor() {
public var name: String? = null
public var args: InstanceArgs = InstanceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend InstanceArgsBuilder.() -> Unit) {
val builder = InstanceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Instance {
val builtJavaResource = com.pulumi.gcp.parallelstore.Instance(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Instance(builtJavaResource)
}
}
/**
* ## Example Usage
* ### Parallelstore Instance Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const network = new gcp.compute.Network("network", {
* name: "network",
* autoCreateSubnetworks: true,
* mtu: 8896,
* });
* const instance = new gcp.parallelstore.Instance("instance", {
* instanceId: "instance",
* location: "us-central1-a",
* description: "test instance",
* capacityGib: "12000",
* network: network.name,
* labels: {
* test: "value",
* },
* });
* // Create an IP address
* const privateIpAlloc = new gcp.compute.GlobalAddress("private_ip_alloc", {
* name: "address",
* purpose: "VPC_PEERING",
* addressType: "INTERNAL",
* prefixLength: 24,
* network: network.id,
* });
* // Create a private connection
* const _default = new gcp.servicenetworking.Connection("default", {
* network: network.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [privateIpAlloc.name],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* network = gcp.compute.Network("network",
* name="network",
* auto_create_subnetworks=True,
* mtu=8896)
* instance = gcp.parallelstore.Instance("instance",
* instance_id="instance",
* location="us-central1-a",
* description="test instance",
* capacity_gib="12000",
* network=network.name,
* labels={
* "test": "value",
* })
* # Create an IP address
* private_ip_alloc = gcp.compute.GlobalAddress("private_ip_alloc",
* name="address",
* purpose="VPC_PEERING",
* address_type="INTERNAL",
* prefix_length=24,
* network=network.id)
* # Create a private connection
* default = gcp.servicenetworking.Connection("default",
* network=network.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[private_ip_alloc.name])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var network = new Gcp.Compute.Network("network", new()
* {
* Name = "network",
* AutoCreateSubnetworks = true,
* Mtu = 8896,
* });
* var instance = new Gcp.ParallelStore.Instance("instance", new()
* {
* InstanceId = "instance",
* Location = "us-central1-a",
* Description = "test instance",
* CapacityGib = "12000",
* Network = network.Name,
* Labels =
* {
* { "test", "value" },
* },
* });
* // Create an IP address
* var privateIpAlloc = new Gcp.Compute.GlobalAddress("private_ip_alloc", new()
* {
* Name = "address",
* Purpose = "VPC_PEERING",
* AddressType = "INTERNAL",
* PrefixLength = 24,
* Network = network.Id,
* });
* // Create a private connection
* var @default = new Gcp.ServiceNetworking.Connection("default", new()
* {
* Network = network.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* privateIpAlloc.Name,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/parallelstore"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* network, err := compute.NewNetwork(ctx, "network", &compute.NetworkArgs{
* Name: pulumi.String("network"),
* AutoCreateSubnetworks: pulumi.Bool(true),
* Mtu: pulumi.Int(8896),
* })
* if err != nil {
* return err
* }
* _, err = parallelstore.NewInstance(ctx, "instance", ¶llelstore.InstanceArgs{
* InstanceId: pulumi.String("instance"),
* Location: pulumi.String("us-central1-a"),
* Description: pulumi.String("test instance"),
* CapacityGib: pulumi.String("12000"),
* Network: network.Name,
* Labels: pulumi.StringMap{
* "test": pulumi.String("value"),
* },
* })
* if err != nil {
* return err
* }
* // Create an IP address
* privateIpAlloc, err := compute.NewGlobalAddress(ctx, "private_ip_alloc", &compute.GlobalAddressArgs{
* Name: pulumi.String("address"),
* Purpose: pulumi.String("VPC_PEERING"),
* AddressType: pulumi.String("INTERNAL"),
* PrefixLength: pulumi.Int(24),
* Network: network.ID(),
* })
* if err != nil {
* return err
* }
* // Create a private connection
* _, err = servicenetworking.NewConnection(ctx, "default", &servicenetworking.ConnectionArgs{
* Network: network.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* privateIpAlloc.Name,
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.parallelstore.Instance;
* import com.pulumi.gcp.parallelstore.InstanceArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var network = new Network("network", NetworkArgs.builder()
* .name("network")
* .autoCreateSubnetworks(true)
* .mtu(8896)
* .build());
* var instance = new Instance("instance", InstanceArgs.builder()
* .instanceId("instance")
* .location("us-central1-a")
* .description("test instance")
* .capacityGib(12000)
* .network(network.name())
* .labels(Map.of("test", "value"))
* .build());
* // Create an IP address
* var privateIpAlloc = new GlobalAddress("privateIpAlloc", GlobalAddressArgs.builder()
* .name("address")
* .purpose("VPC_PEERING")
* .addressType("INTERNAL")
* .prefixLength(24)
* .network(network.id())
* .build());
* // Create a private connection
* var default_ = new Connection("default", ConnectionArgs.builder()
* .network(network.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(privateIpAlloc.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:parallelstore:Instance
* properties:
* instanceId: instance
* location: us-central1-a
* description: test instance
* capacityGib: 12000
* network: ${network.name}
* labels:
* test: value
* network:
* type: gcp:compute:Network
* properties:
* name: network
* autoCreateSubnetworks: true
* mtu: 8896
* # Create an IP address
* privateIpAlloc:
* type: gcp:compute:GlobalAddress
* name: private_ip_alloc
* properties:
* name: address
* purpose: VPC_PEERING
* addressType: INTERNAL
* prefixLength: 24
* network: ${network.id}
* # Create a private connection
* default:
* type: gcp:servicenetworking:Connection
* properties:
* network: ${network.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${privateIpAlloc.name}
* ```
*
* ## Import
* Instance can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/instances/{{instance_id}}`
* * `{{project}}/{{location}}/{{instance_id}}`
* * `{{location}}/{{instance_id}}`
* When using the `pulumi import` command, Instance can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:parallelstore/instance:Instance default projects/{{project}}/locations/{{location}}/instances/{{instance_id}}
* ```
* ```sh
* $ pulumi import gcp:parallelstore/instance:Instance default {{project}}/{{location}}/{{instance_id}}
* ```
* ```sh
* $ pulumi import gcp:parallelstore/instance:Instance default {{location}}/{{instance_id}}
* ```
*/
public class Instance internal constructor(
override val javaResource: com.pulumi.gcp.parallelstore.Instance,
) : KotlinCustomResource(javaResource, InstanceMapper) {
/**
* List of access_points.
* Contains a list of IPv4 addresses used for client side configuration.
*/
public val accessPoints: Output>
get() = javaResource.accessPoints().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Immutable. Storage capacity of Parallelstore instance in Gibibytes (GiB).
*/
public val capacityGib: Output
get() = javaResource.capacityGib().applyValue({ args0 -> args0 })
/**
* The time when the instance was created.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* The version of DAOS software running in the instance
*/
public val daosVersion: Output
get() = javaResource.daosVersion().applyValue({ args0 -> args0 })
/**
* The description of the instance. 2048 characters or less.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
*/
public val effectiveLabels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy