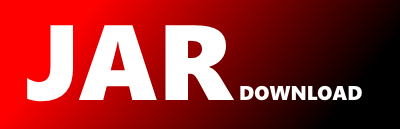
com.pulumi.gcp.privilegedaccessmanager.kotlin.Entitlement.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.privilegedaccessmanager.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementAdditionalNotificationTargets
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementApprovalWorkflow
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementEligibleUser
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementPrivilegedAccess
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementRequesterJustificationConfig
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementAdditionalNotificationTargets.Companion.toKotlin as entitlementAdditionalNotificationTargetsToKotlin
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementApprovalWorkflow.Companion.toKotlin as entitlementApprovalWorkflowToKotlin
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementEligibleUser.Companion.toKotlin as entitlementEligibleUserToKotlin
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementPrivilegedAccess.Companion.toKotlin as entitlementPrivilegedAccessToKotlin
import com.pulumi.gcp.privilegedaccessmanager.kotlin.outputs.EntitlementRequesterJustificationConfig.Companion.toKotlin as entitlementRequesterJustificationConfigToKotlin
/**
* Builder for [Entitlement].
*/
@PulumiTagMarker
public class EntitlementResourceBuilder internal constructor() {
public var name: String? = null
public var args: EntitlementArgs = EntitlementArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EntitlementArgsBuilder.() -> Unit) {
val builder = EntitlementArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Entitlement {
val builtJavaResource =
com.pulumi.gcp.privilegedaccessmanager.Entitlement(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Entitlement(builtJavaResource)
}
}
/**
* ## Example Usage
* ### Privileged Access Manager Entitlement Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const tfentitlement = new gcp.privilegedaccessmanager.Entitlement("tfentitlement", {
* entitlementId: "example-entitlement",
* location: "global",
* maxRequestDuration: "43200s",
* parent: "projects/my-project-name",
* requesterJustificationConfig: {
* unstructured: {},
* },
* eligibleUsers: [{
* principals: ["group:test@google.com"],
* }],
* privilegedAccess: {
* gcpIamAccess: {
* roleBindings: [{
* role: "roles/storage.admin",
* conditionExpression: "request.time < timestamp(\"2024-04-23T18:30:00.000Z\")",
* }],
* resource: "//cloudresourcemanager.googleapis.com/projects/my-project-name",
* resourceType: "cloudresourcemanager.googleapis.com/Project",
* },
* },
* additionalNotificationTargets: {
* adminEmailRecipients: ["user@example.com"],
* requesterEmailRecipients: ["user@example.com"],
* },
* approvalWorkflow: {
* manualApprovals: {
* requireApproverJustification: true,
* steps: [{
* approvalsNeeded: 1,
* approverEmailRecipients: ["user@example.com"],
* approvers: {
* principals: ["group:test@google.com"],
* },
* }],
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* tfentitlement = gcp.privilegedaccessmanager.Entitlement("tfentitlement",
* entitlement_id="example-entitlement",
* location="global",
* max_request_duration="43200s",
* parent="projects/my-project-name",
* requester_justification_config=gcp.privilegedaccessmanager.EntitlementRequesterJustificationConfigArgs(
* unstructured=gcp.privilegedaccessmanager.EntitlementRequesterJustificationConfigUnstructuredArgs(),
* ),
* eligible_users=[gcp.privilegedaccessmanager.EntitlementEligibleUserArgs(
* principals=["group:test@google.com"],
* )],
* privileged_access=gcp.privilegedaccessmanager.EntitlementPrivilegedAccessArgs(
* gcp_iam_access=gcp.privilegedaccessmanager.EntitlementPrivilegedAccessGcpIamAccessArgs(
* role_bindings=[gcp.privilegedaccessmanager.EntitlementPrivilegedAccessGcpIamAccessRoleBindingArgs(
* role="roles/storage.admin",
* condition_expression="request.time < timestamp(\"2024-04-23T18:30:00.000Z\")",
* )],
* resource="//cloudresourcemanager.googleapis.com/projects/my-project-name",
* resource_type="cloudresourcemanager.googleapis.com/Project",
* ),
* ),
* additional_notification_targets=gcp.privilegedaccessmanager.EntitlementAdditionalNotificationTargetsArgs(
* admin_email_recipients=["user@example.com"],
* requester_email_recipients=["user@example.com"],
* ),
* approval_workflow=gcp.privilegedaccessmanager.EntitlementApprovalWorkflowArgs(
* manual_approvals=gcp.privilegedaccessmanager.EntitlementApprovalWorkflowManualApprovalsArgs(
* require_approver_justification=True,
* steps=[gcp.privilegedaccessmanager.EntitlementApprovalWorkflowManualApprovalsStepArgs(
* approvals_needed=1,
* approver_email_recipients=["user@example.com"],
* approvers=gcp.privilegedaccessmanager.EntitlementApprovalWorkflowManualApprovalsStepApproversArgs(
* principals=["group:test@google.com"],
* ),
* )],
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var tfentitlement = new Gcp.PrivilegedAccessManager.Entitlement("tfentitlement", new()
* {
* EntitlementId = "example-entitlement",
* Location = "global",
* MaxRequestDuration = "43200s",
* Parent = "projects/my-project-name",
* RequesterJustificationConfig = new Gcp.PrivilegedAccessManager.Inputs.EntitlementRequesterJustificationConfigArgs
* {
* Unstructured = null,
* },
* EligibleUsers = new[]
* {
* new Gcp.PrivilegedAccessManager.Inputs.EntitlementEligibleUserArgs
* {
* Principals = new[]
* {
* "group:[email protected]",
* },
* },
* },
* PrivilegedAccess = new Gcp.PrivilegedAccessManager.Inputs.EntitlementPrivilegedAccessArgs
* {
* GcpIamAccess = new Gcp.PrivilegedAccessManager.Inputs.EntitlementPrivilegedAccessGcpIamAccessArgs
* {
* RoleBindings = new[]
* {
* new Gcp.PrivilegedAccessManager.Inputs.EntitlementPrivilegedAccessGcpIamAccessRoleBindingArgs
* {
* Role = "roles/storage.admin",
* ConditionExpression = "request.time < timestamp(\"2024-04-23T18:30:00.000Z\")",
* },
* },
* Resource = "//cloudresourcemanager.googleapis.com/projects/my-project-name",
* ResourceType = "cloudresourcemanager.googleapis.com/Project",
* },
* },
* AdditionalNotificationTargets = new Gcp.PrivilegedAccessManager.Inputs.EntitlementAdditionalNotificationTargetsArgs
* {
* AdminEmailRecipients = new[]
* {
* "[email protected]",
* },
* RequesterEmailRecipients = new[]
* {
* "[email protected]",
* },
* },
* ApprovalWorkflow = new Gcp.PrivilegedAccessManager.Inputs.EntitlementApprovalWorkflowArgs
* {
* ManualApprovals = new Gcp.PrivilegedAccessManager.Inputs.EntitlementApprovalWorkflowManualApprovalsArgs
* {
* RequireApproverJustification = true,
* Steps = new[]
* {
* new Gcp.PrivilegedAccessManager.Inputs.EntitlementApprovalWorkflowManualApprovalsStepArgs
* {
* ApprovalsNeeded = 1,
* ApproverEmailRecipients = new[]
* {
* "[email protected]",
* },
* Approvers = new Gcp.PrivilegedAccessManager.Inputs.EntitlementApprovalWorkflowManualApprovalsStepApproversArgs
* {
* Principals = new[]
* {
* "group:[email protected]",
* },
* },
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/privilegedaccessmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := privilegedaccessmanager.Newentitlement(ctx, "tfentitlement", &privilegedaccessmanager.entitlementArgs{
* EntitlementId: pulumi.String("example-entitlement"),
* Location: pulumi.String("global"),
* MaxRequestDuration: pulumi.String("43200s"),
* Parent: pulumi.String("projects/my-project-name"),
* RequesterJustificationConfig: &privilegedaccessmanager.EntitlementRequesterJustificationConfigArgs{
* Unstructured: nil,
* },
* EligibleUsers: privilegedaccessmanager.EntitlementEligibleUserArray{
* &privilegedaccessmanager.EntitlementEligibleUserArgs{
* Principals: pulumi.StringArray{
* pulumi.String("group:[email protected]"),
* },
* },
* },
* PrivilegedAccess: &privilegedaccessmanager.EntitlementPrivilegedAccessArgs{
* GcpIamAccess: &privilegedaccessmanager.EntitlementPrivilegedAccessGcpIamAccessArgs{
* RoleBindings: privilegedaccessmanager.EntitlementPrivilegedAccessGcpIamAccessRoleBindingArray{
* &privilegedaccessmanager.EntitlementPrivilegedAccessGcpIamAccessRoleBindingArgs{
* Role: pulumi.String("roles/storage.admin"),
* ConditionExpression: pulumi.String("request.time < timestamp(\"2024-04-23T18:30:00.000Z\")"),
* },
* },
* Resource: pulumi.String("//cloudresourcemanager.googleapis.com/projects/my-project-name"),
* ResourceType: pulumi.String("cloudresourcemanager.googleapis.com/Project"),
* },
* },
* AdditionalNotificationTargets: &privilegedaccessmanager.EntitlementAdditionalNotificationTargetsArgs{
* AdminEmailRecipients: pulumi.StringArray{
* pulumi.String("[email protected]"),
* },
* RequesterEmailRecipients: pulumi.StringArray{
* pulumi.String("[email protected]"),
* },
* },
* ApprovalWorkflow: &privilegedaccessmanager.EntitlementApprovalWorkflowArgs{
* ManualApprovals: &privilegedaccessmanager.EntitlementApprovalWorkflowManualApprovalsArgs{
* RequireApproverJustification: pulumi.Bool(true),
* Steps: privilegedaccessmanager.EntitlementApprovalWorkflowManualApprovalsStepArray{
* &privilegedaccessmanager.EntitlementApprovalWorkflowManualApprovalsStepArgs{
* ApprovalsNeeded: pulumi.Int(1),
* ApproverEmailRecipients: pulumi.StringArray{
* pulumi.String("[email protected]"),
* },
* Approvers: &privilegedaccessmanager.EntitlementApprovalWorkflowManualApprovalsStepApproversArgs{
* Principals: pulumi.StringArray{
* pulumi.String("group:[email protected]"),
* },
* },
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.privilegedaccessmanager.entitlement;
* import com.pulumi.gcp.privilegedaccessmanager.EntitlementArgs;
* import com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementRequesterJustificationConfigArgs;
* import com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementRequesterJustificationConfigUnstructuredArgs;
* import com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementEligibleUserArgs;
* import com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementPrivilegedAccessArgs;
* import com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementPrivilegedAccessGcpIamAccessArgs;
* import com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementAdditionalNotificationTargetsArgs;
* import com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementApprovalWorkflowArgs;
* import com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementApprovalWorkflowManualApprovalsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var tfentitlement = new Entitlement("tfentitlement", EntitlementArgs.builder()
* .entitlementId("example-entitlement")
* .location("global")
* .maxRequestDuration("43200s")
* .parent("projects/my-project-name")
* .requesterJustificationConfig(EntitlementRequesterJustificationConfigArgs.builder()
* .unstructured()
* .build())
* .eligibleUsers(EntitlementEligibleUserArgs.builder()
* .principals("group:[email protected]")
* .build())
* .privilegedAccess(EntitlementPrivilegedAccessArgs.builder()
* .gcpIamAccess(EntitlementPrivilegedAccessGcpIamAccessArgs.builder()
* .roleBindings(EntitlementPrivilegedAccessGcpIamAccessRoleBindingArgs.builder()
* .role("roles/storage.admin")
* .conditionExpression("request.time < timestamp(\"2024-04-23T18:30:00.000Z\")")
* .build())
* .resource("//cloudresourcemanager.googleapis.com/projects/my-project-name")
* .resourceType("cloudresourcemanager.googleapis.com/Project")
* .build())
* .build())
* .additionalNotificationTargets(EntitlementAdditionalNotificationTargetsArgs.builder()
* .adminEmailRecipients("[email protected]")
* .requesterEmailRecipients("[email protected]")
* .build())
* .approvalWorkflow(EntitlementApprovalWorkflowArgs.builder()
* .manualApprovals(EntitlementApprovalWorkflowManualApprovalsArgs.builder()
* .requireApproverJustification(true)
* .steps(EntitlementApprovalWorkflowManualApprovalsStepArgs.builder()
* .approvalsNeeded(1)
* .approverEmailRecipients("[email protected]")
* .approvers(EntitlementApprovalWorkflowManualApprovalsStepApproversArgs.builder()
* .principals("group:[email protected]")
* .build())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* tfentitlement:
* type: gcp:privilegedaccessmanager:entitlement
* properties:
* entitlementId: example-entitlement
* location: global
* maxRequestDuration: 43200s
* parent: projects/my-project-name
* requesterJustificationConfig:
* unstructured: {}
* eligibleUsers:
* - principals:
* - group:[email protected]
* privilegedAccess:
* gcpIamAccess:
* roleBindings:
* - role: roles/storage.admin
* conditionExpression: request.time < timestamp("2024-04-23T18:30:00.000Z")
* resource: //cloudresourcemanager.googleapis.com/projects/my-project-name
* resourceType: cloudresourcemanager.googleapis.com/Project
* additionalNotificationTargets:
* adminEmailRecipients:
* - [email protected]
* requesterEmailRecipients:
* - [email protected]
* approvalWorkflow:
* manualApprovals:
* requireApproverJustification: true
* steps:
* - approvalsNeeded: 1
* approverEmailRecipients:
* - [email protected]
* approvers:
* principals:
* - group:[email protected]
* ```
*
* ## Import
* Entitlement can be imported using any of these accepted formats:
* * `{{parent}}/locations/{{location}}/entitlements/{{entitlement_id}}`
* When using the `pulumi import` command, Entitlement can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:privilegedaccessmanager/entitlement:entitlement default {{parent}}/locations/{{location}}/entitlements/{{entitlement_id}}
* ```
*/
public class Entitlement internal constructor(
override val javaResource: com.pulumi.gcp.privilegedaccessmanager.Entitlement,
) : KotlinCustomResource(javaResource, EntitlementMapper) {
/**
* AdditionalNotificationTargets includes email addresses to be notified.
*/
public val additionalNotificationTargets: Output?
get() = javaResource.additionalNotificationTargets().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
entitlementAdditionalNotificationTargetsToKotlin(args0)
})
}).orElse(null)
})
/**
* The approvals needed before access will be granted to a requester. No approvals will be needed if this field is null.
* Different types of approval workflows that can be used to gate privileged access granting.
*/
public val approvalWorkflow: Output?
get() = javaResource.approvalWorkflow().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> entitlementApprovalWorkflowToKotlin(args0) })
}).orElse(null)
})
/**
* Output only. Create time stamp. A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits.
* Examples: "2014-10-02T15:01:23Z" and "2014-10-02T15:01:23.045123456Z"
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* Who can create Grants using Entitlement. This list should contain at most one entry
* Structure is documented below.
*/
public val eligibleUsers: Output>
get() = javaResource.eligibleUsers().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> entitlementEligibleUserToKotlin(args0) })
})
})
/**
* The ID to use for this Entitlement. This will become the last part of the resource name.
* This value should be 4-63 characters, and valid characters are "[a-z]", "[0-9]", and "-". The first character should be from [a-z].
* This value should be unique among all other Entitlements under the specified `parent`.
*/
public val entitlementId: Output
get() = javaResource.entitlementId().applyValue({ args0 -> args0 })
/**
* For Resource freshness validation (https://google.aip.dev/154)
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* The region of the Entitlement resource.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The maximum amount of time for which access would be granted for a request.
* A requester can choose to ask for access for less than this duration but never more.
* Format: calculate the time in seconds and concatenate it with 's' i.e. 2 hours = "7200s", 45 minutes = "2700s"
*/
public val maxRequestDuration: Output
get() = javaResource.maxRequestDuration().applyValue({ args0 -> args0 })
/**
* Output Only. The entitlement's name follows a hierarchical structure, comprising the organization, folder, or project, alongside the region and a unique entitlement ID.
* Formats: organizations/{organization-number}/locations/{region}/entitlements/{entitlement-id}, folders/{folder-number}/locations/{region}/entitlements/{entitlement-id}, and projects/{project-id|project-number}/locations/{region}/entitlements/{entitlement-id}.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Format: project/{project_id} or organization/{organization_number} or folder/{folder_number}
*/
public val parent: Output
get() = javaResource.parent().applyValue({ args0 -> args0 })
/**
* Privileged access that this service can be used to gate.
* Structure is documented below.
*/
public val privilegedAccess: Output
get() = javaResource.privilegedAccess().applyValue({ args0 ->
args0.let({ args0 ->
entitlementPrivilegedAccessToKotlin(args0)
})
})
/**
* Defines the ways in which a requester should provide the justification while requesting for access.
* Structure is documented below.
*/
public val requesterJustificationConfig: Output
get() = javaResource.requesterJustificationConfig().applyValue({ args0 ->
args0.let({ args0 ->
entitlementRequesterJustificationConfigToKotlin(args0)
})
})
/**
* Output only. The current state of the Entitlement.
*/
public val state: Output
get() = javaResource.state().applyValue({ args0 -> args0 })
/**
* Output only. Update time stamp. A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits.
* Examples: "2014-10-02T15:01:23Z" and "2014-10-02T15:01:23.045123456Z".
*/
public val updateTime: Output
get() = javaResource.updateTime().applyValue({ args0 -> args0 })
}
public object EntitlementMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.privilegedaccessmanager.Entitlement::class == javaResource::class
override fun map(javaResource: Resource): Entitlement = Entitlement(
javaResource as
com.pulumi.gcp.privilegedaccessmanager.Entitlement,
)
}
/**
* @see [entitlement].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Entitlement].
*/
public suspend fun entitlement(name: String, block: suspend EntitlementResourceBuilder.() -> Unit): Entitlement {
val builder = EntitlementResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [entitlement].
* @param name The _unique_ name of the resulting resource.
*/
public fun entitlement(name: String): Entitlement {
val builder = EntitlementResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy