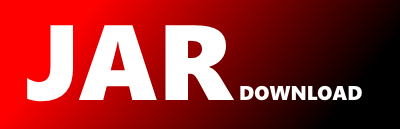
com.pulumi.gcp.privilegedaccessmanager.kotlin.inputs.EntitlementApprovalWorkflowArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.privilegedaccessmanager.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementApprovalWorkflowArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property manualApprovals A manual approval workflow where users who are designated as approvers need to call the ApproveGrant/DenyGrant APIs for an Grant.
* The workflow can consist of multiple serial steps where each step defines who can act as Approver in that step and how many of those users should approve before the workflow moves to the next step.
* This can be used to create approval workflows such as
* * Require an approval from any user in a group G.
* * Require an approval from any k number of users from a Group G.
* * Require an approval from any user in a group G and then from a user U. etc.
* A single user might be part of `approvers` ACL for multiple steps in this workflow but they can only approve once and that approval will only be considered to satisfy the approval step at which it was granted.
* Structure is documented below.
*/
public data class EntitlementApprovalWorkflowArgs(
public val manualApprovals: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementApprovalWorkflowArgs =
com.pulumi.gcp.privilegedaccessmanager.inputs.EntitlementApprovalWorkflowArgs.builder()
.manualApprovals(
manualApprovals.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [EntitlementApprovalWorkflowArgs].
*/
@PulumiTagMarker
public class EntitlementApprovalWorkflowArgsBuilder internal constructor() {
private var manualApprovals: Output? = null
/**
* @param value A manual approval workflow where users who are designated as approvers need to call the ApproveGrant/DenyGrant APIs for an Grant.
* The workflow can consist of multiple serial steps where each step defines who can act as Approver in that step and how many of those users should approve before the workflow moves to the next step.
* This can be used to create approval workflows such as
* * Require an approval from any user in a group G.
* * Require an approval from any k number of users from a Group G.
* * Require an approval from any user in a group G and then from a user U. etc.
* A single user might be part of `approvers` ACL for multiple steps in this workflow but they can only approve once and that approval will only be considered to satisfy the approval step at which it was granted.
* Structure is documented below.
*/
@JvmName("esaswbtgqixjqwff")
public suspend fun manualApprovals(`value`: Output) {
this.manualApprovals = value
}
/**
* @param value A manual approval workflow where users who are designated as approvers need to call the ApproveGrant/DenyGrant APIs for an Grant.
* The workflow can consist of multiple serial steps where each step defines who can act as Approver in that step and how many of those users should approve before the workflow moves to the next step.
* This can be used to create approval workflows such as
* * Require an approval from any user in a group G.
* * Require an approval from any k number of users from a Group G.
* * Require an approval from any user in a group G and then from a user U. etc.
* A single user might be part of `approvers` ACL for multiple steps in this workflow but they can only approve once and that approval will only be considered to satisfy the approval step at which it was granted.
* Structure is documented below.
*/
@JvmName("yrfgwdqsoqpbpqbh")
public suspend fun manualApprovals(`value`: EntitlementApprovalWorkflowManualApprovalsArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.manualApprovals = mapped
}
/**
* @param argument A manual approval workflow where users who are designated as approvers need to call the ApproveGrant/DenyGrant APIs for an Grant.
* The workflow can consist of multiple serial steps where each step defines who can act as Approver in that step and how many of those users should approve before the workflow moves to the next step.
* This can be used to create approval workflows such as
* * Require an approval from any user in a group G.
* * Require an approval from any k number of users from a Group G.
* * Require an approval from any user in a group G and then from a user U. etc.
* A single user might be part of `approvers` ACL for multiple steps in this workflow but they can only approve once and that approval will only be considered to satisfy the approval step at which it was granted.
* Structure is documented below.
*/
@JvmName("dboaisikiaresmwh")
public suspend fun manualApprovals(argument: suspend EntitlementApprovalWorkflowManualApprovalsArgsBuilder.() -> Unit) {
val toBeMapped = EntitlementApprovalWorkflowManualApprovalsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.manualApprovals = mapped
}
internal fun build(): EntitlementApprovalWorkflowArgs = EntitlementApprovalWorkflowArgs(
manualApprovals = manualApprovals ?: throw PulumiNullFieldException("manualApprovals"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy