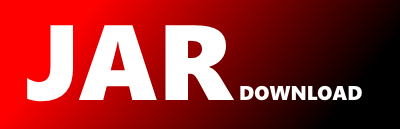
com.pulumi.gcp.projects.kotlin.inputs.ApiKeyRestrictionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.projects.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.projects.inputs.ApiKeyRestrictionsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property androidKeyRestrictions The Android apps that are allowed to use the key.
* @property apiTargets A restriction for a specific service and optionally one or more specific methods. Requests are allowed if they match any of these restrictions. If no restrictions are specified, all targets are allowed.
* @property browserKeyRestrictions The HTTP referrers (websites) that are allowed to use the key.
* @property iosKeyRestrictions The iOS apps that are allowed to use the key.
* @property serverKeyRestrictions The IP addresses of callers that are allowed to use the key.
*/
public data class ApiKeyRestrictionsArgs(
public val androidKeyRestrictions: Output? = null,
public val apiTargets: Output>? = null,
public val browserKeyRestrictions: Output? = null,
public val iosKeyRestrictions: Output? = null,
public val serverKeyRestrictions: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.projects.inputs.ApiKeyRestrictionsArgs =
com.pulumi.gcp.projects.inputs.ApiKeyRestrictionsArgs.builder()
.androidKeyRestrictions(
androidKeyRestrictions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.apiTargets(
apiTargets?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.browserKeyRestrictions(
browserKeyRestrictions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.iosKeyRestrictions(
iosKeyRestrictions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.serverKeyRestrictions(
serverKeyRestrictions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ApiKeyRestrictionsArgs].
*/
@PulumiTagMarker
public class ApiKeyRestrictionsArgsBuilder internal constructor() {
private var androidKeyRestrictions: Output? = null
private var apiTargets: Output>? = null
private var browserKeyRestrictions: Output? = null
private var iosKeyRestrictions: Output? = null
private var serverKeyRestrictions: Output? = null
/**
* @param value The Android apps that are allowed to use the key.
*/
@JvmName("cupkvhsqumkynoyi")
public suspend fun androidKeyRestrictions(`value`: Output) {
this.androidKeyRestrictions = value
}
/**
* @param value A restriction for a specific service and optionally one or more specific methods. Requests are allowed if they match any of these restrictions. If no restrictions are specified, all targets are allowed.
*/
@JvmName("sdpveolodgaafton")
public suspend fun apiTargets(`value`: Output>) {
this.apiTargets = value
}
@JvmName("npdoyxtxhxtgewun")
public suspend fun apiTargets(vararg values: Output) {
this.apiTargets = Output.all(values.asList())
}
/**
* @param values A restriction for a specific service and optionally one or more specific methods. Requests are allowed if they match any of these restrictions. If no restrictions are specified, all targets are allowed.
*/
@JvmName("paudqbrfygtabxyy")
public suspend fun apiTargets(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy