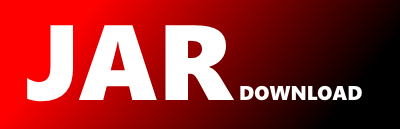
com.pulumi.gcp.pubsub.kotlin.SubscriptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.pubsub.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.pubsub.SubscriptionArgs.builder
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionBigqueryConfigArgs
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionBigqueryConfigArgsBuilder
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionCloudStorageConfigArgs
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionCloudStorageConfigArgsBuilder
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionDeadLetterPolicyArgs
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionDeadLetterPolicyArgsBuilder
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionExpirationPolicyArgs
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionExpirationPolicyArgsBuilder
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionPushConfigArgs
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionPushConfigArgsBuilder
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionRetryPolicyArgs
import com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionRetryPolicyArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A named resource representing the stream of messages from a single,
* specific topic, to be delivered to the subscribing application.
* To get more information about Subscription, see:
* * [API documentation](https://cloud.google.com/pubsub/docs/reference/rest/v1/projects.subscriptions)
* * How-to Guides
* * [Managing Subscriptions](https://cloud.google.com/pubsub/docs/admin#managing_subscriptions)
* > **Note:** You can retrieve the email of the Google Managed Pub/Sub Service Account used for forwarding
* by using the `gcp.projects.ServiceIdentity` resource.
* ## Example Usage
* ### Pubsub Subscription Push
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.pubsub.Topic("example", {name: "example-topic"});
* const exampleSubscription = new gcp.pubsub.Subscription("example", {
* name: "example-subscription",
* topic: example.id,
* ackDeadlineSeconds: 20,
* labels: {
* foo: "bar",
* },
* pushConfig: {
* pushEndpoint: "https://example.com/push",
* attributes: {
* "x-goog-version": "v1",
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.pubsub.Topic("example", name="example-topic")
* example_subscription = gcp.pubsub.Subscription("example",
* name="example-subscription",
* topic=example.id,
* ack_deadline_seconds=20,
* labels={
* "foo": "bar",
* },
* push_config=gcp.pubsub.SubscriptionPushConfigArgs(
* push_endpoint="https://example.com/push",
* attributes={
* "x-goog-version": "v1",
* },
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.PubSub.Topic("example", new()
* {
* Name = "example-topic",
* });
* var exampleSubscription = new Gcp.PubSub.Subscription("example", new()
* {
* Name = "example-subscription",
* Topic = example.Id,
* AckDeadlineSeconds = 20,
* Labels =
* {
* { "foo", "bar" },
* },
* PushConfig = new Gcp.PubSub.Inputs.SubscriptionPushConfigArgs
* {
* PushEndpoint = "https://example.com/push",
* Attributes =
* {
* { "x-goog-version", "v1" },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := pubsub.NewTopic(ctx, "example", &pubsub.TopicArgs{
* Name: pulumi.String("example-topic"),
* })
* if err != nil {
* return err
* }
* _, err = pubsub.NewSubscription(ctx, "example", &pubsub.SubscriptionArgs{
* Name: pulumi.String("example-subscription"),
* Topic: example.ID(),
* AckDeadlineSeconds: pulumi.Int(20),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* PushConfig: &pubsub.SubscriptionPushConfigArgs{
* PushEndpoint: pulumi.String("https://example.com/push"),
* Attributes: pulumi.StringMap{
* "x-goog-version": pulumi.String("v1"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import com.pulumi.gcp.pubsub.Subscription;
* import com.pulumi.gcp.pubsub.SubscriptionArgs;
* import com.pulumi.gcp.pubsub.inputs.SubscriptionPushConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Topic("example", TopicArgs.builder()
* .name("example-topic")
* .build());
* var exampleSubscription = new Subscription("exampleSubscription", SubscriptionArgs.builder()
* .name("example-subscription")
* .topic(example.id())
* .ackDeadlineSeconds(20)
* .labels(Map.of("foo", "bar"))
* .pushConfig(SubscriptionPushConfigArgs.builder()
* .pushEndpoint("https://example.com/push")
* .attributes(Map.of("x-goog-version", "v1"))
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:pubsub:Topic
* properties:
* name: example-topic
* exampleSubscription:
* type: gcp:pubsub:Subscription
* name: example
* properties:
* name: example-subscription
* topic: ${example.id}
* ackDeadlineSeconds: 20
* labels:
* foo: bar
* pushConfig:
* pushEndpoint: https://example.com/push
* attributes:
* x-goog-version: v1
* ```
*
* ### Pubsub Subscription Pull
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.pubsub.Topic("example", {name: "example-topic"});
* const exampleSubscription = new gcp.pubsub.Subscription("example", {
* name: "example-subscription",
* topic: example.id,
* labels: {
* foo: "bar",
* },
* messageRetentionDuration: "1200s",
* retainAckedMessages: true,
* ackDeadlineSeconds: 20,
* expirationPolicy: {
* ttl: "300000.5s",
* },
* retryPolicy: {
* minimumBackoff: "10s",
* },
* enableMessageOrdering: false,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.pubsub.Topic("example", name="example-topic")
* example_subscription = gcp.pubsub.Subscription("example",
* name="example-subscription",
* topic=example.id,
* labels={
* "foo": "bar",
* },
* message_retention_duration="1200s",
* retain_acked_messages=True,
* ack_deadline_seconds=20,
* expiration_policy=gcp.pubsub.SubscriptionExpirationPolicyArgs(
* ttl="300000.5s",
* ),
* retry_policy=gcp.pubsub.SubscriptionRetryPolicyArgs(
* minimum_backoff="10s",
* ),
* enable_message_ordering=False)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.PubSub.Topic("example", new()
* {
* Name = "example-topic",
* });
* var exampleSubscription = new Gcp.PubSub.Subscription("example", new()
* {
* Name = "example-subscription",
* Topic = example.Id,
* Labels =
* {
* { "foo", "bar" },
* },
* MessageRetentionDuration = "1200s",
* RetainAckedMessages = true,
* AckDeadlineSeconds = 20,
* ExpirationPolicy = new Gcp.PubSub.Inputs.SubscriptionExpirationPolicyArgs
* {
* Ttl = "300000.5s",
* },
* RetryPolicy = new Gcp.PubSub.Inputs.SubscriptionRetryPolicyArgs
* {
* MinimumBackoff = "10s",
* },
* EnableMessageOrdering = false,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := pubsub.NewTopic(ctx, "example", &pubsub.TopicArgs{
* Name: pulumi.String("example-topic"),
* })
* if err != nil {
* return err
* }
* _, err = pubsub.NewSubscription(ctx, "example", &pubsub.SubscriptionArgs{
* Name: pulumi.String("example-subscription"),
* Topic: example.ID(),
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* MessageRetentionDuration: pulumi.String("1200s"),
* RetainAckedMessages: pulumi.Bool(true),
* AckDeadlineSeconds: pulumi.Int(20),
* ExpirationPolicy: &pubsub.SubscriptionExpirationPolicyArgs{
* Ttl: pulumi.String("300000.5s"),
* },
* RetryPolicy: &pubsub.SubscriptionRetryPolicyArgs{
* MinimumBackoff: pulumi.String("10s"),
* },
* EnableMessageOrdering: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import com.pulumi.gcp.pubsub.Subscription;
* import com.pulumi.gcp.pubsub.SubscriptionArgs;
* import com.pulumi.gcp.pubsub.inputs.SubscriptionExpirationPolicyArgs;
* import com.pulumi.gcp.pubsub.inputs.SubscriptionRetryPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Topic("example", TopicArgs.builder()
* .name("example-topic")
* .build());
* var exampleSubscription = new Subscription("exampleSubscription", SubscriptionArgs.builder()
* .name("example-subscription")
* .topic(example.id())
* .labels(Map.of("foo", "bar"))
* .messageRetentionDuration("1200s")
* .retainAckedMessages(true)
* .ackDeadlineSeconds(20)
* .expirationPolicy(SubscriptionExpirationPolicyArgs.builder()
* .ttl("300000.5s")
* .build())
* .retryPolicy(SubscriptionRetryPolicyArgs.builder()
* .minimumBackoff("10s")
* .build())
* .enableMessageOrdering(false)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:pubsub:Topic
* properties:
* name: example-topic
* exampleSubscription:
* type: gcp:pubsub:Subscription
* name: example
* properties:
* name: example-subscription
* topic: ${example.id}
* labels:
* foo: bar
* messageRetentionDuration: 1200s
* retainAckedMessages: true
* ackDeadlineSeconds: 20
* expirationPolicy:
* ttl: 300000.5s
* retryPolicy:
* minimumBackoff: 10s
* enableMessageOrdering: false
* ```
*
* ### Pubsub Subscription Dead Letter
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.pubsub.Topic("example", {name: "example-topic"});
* const exampleDeadLetter = new gcp.pubsub.Topic("example_dead_letter", {name: "example-topic-dead-letter"});
* const exampleSubscription = new gcp.pubsub.Subscription("example", {
* name: "example-subscription",
* topic: example.id,
* deadLetterPolicy: {
* deadLetterTopic: exampleDeadLetter.id,
* maxDeliveryAttempts: 10,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.pubsub.Topic("example", name="example-topic")
* example_dead_letter = gcp.pubsub.Topic("example_dead_letter", name="example-topic-dead-letter")
* example_subscription = gcp.pubsub.Subscription("example",
* name="example-subscription",
* topic=example.id,
* dead_letter_policy=gcp.pubsub.SubscriptionDeadLetterPolicyArgs(
* dead_letter_topic=example_dead_letter.id,
* max_delivery_attempts=10,
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.PubSub.Topic("example", new()
* {
* Name = "example-topic",
* });
* var exampleDeadLetter = new Gcp.PubSub.Topic("example_dead_letter", new()
* {
* Name = "example-topic-dead-letter",
* });
* var exampleSubscription = new Gcp.PubSub.Subscription("example", new()
* {
* Name = "example-subscription",
* Topic = example.Id,
* DeadLetterPolicy = new Gcp.PubSub.Inputs.SubscriptionDeadLetterPolicyArgs
* {
* DeadLetterTopic = exampleDeadLetter.Id,
* MaxDeliveryAttempts = 10,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := pubsub.NewTopic(ctx, "example", &pubsub.TopicArgs{
* Name: pulumi.String("example-topic"),
* })
* if err != nil {
* return err
* }
* exampleDeadLetter, err := pubsub.NewTopic(ctx, "example_dead_letter", &pubsub.TopicArgs{
* Name: pulumi.String("example-topic-dead-letter"),
* })
* if err != nil {
* return err
* }
* _, err = pubsub.NewSubscription(ctx, "example", &pubsub.SubscriptionArgs{
* Name: pulumi.String("example-subscription"),
* Topic: example.ID(),
* DeadLetterPolicy: &pubsub.SubscriptionDeadLetterPolicyArgs{
* DeadLetterTopic: exampleDeadLetter.ID(),
* MaxDeliveryAttempts: pulumi.Int(10),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import com.pulumi.gcp.pubsub.Subscription;
* import com.pulumi.gcp.pubsub.SubscriptionArgs;
* import com.pulumi.gcp.pubsub.inputs.SubscriptionDeadLetterPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Topic("example", TopicArgs.builder()
* .name("example-topic")
* .build());
* var exampleDeadLetter = new Topic("exampleDeadLetter", TopicArgs.builder()
* .name("example-topic-dead-letter")
* .build());
* var exampleSubscription = new Subscription("exampleSubscription", SubscriptionArgs.builder()
* .name("example-subscription")
* .topic(example.id())
* .deadLetterPolicy(SubscriptionDeadLetterPolicyArgs.builder()
* .deadLetterTopic(exampleDeadLetter.id())
* .maxDeliveryAttempts(10)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:pubsub:Topic
* properties:
* name: example-topic
* exampleDeadLetter:
* type: gcp:pubsub:Topic
* name: example_dead_letter
* properties:
* name: example-topic-dead-letter
* exampleSubscription:
* type: gcp:pubsub:Subscription
* name: example
* properties:
* name: example-subscription
* topic: ${example.id}
* deadLetterPolicy:
* deadLetterTopic: ${exampleDeadLetter.id}
* maxDeliveryAttempts: 10
* ```
*
* ### Pubsub Subscription Push Bq
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.pubsub.Topic("example", {name: "example-topic"});
* const test = new gcp.bigquery.Dataset("test", {datasetId: "example_dataset"});
* const testTable = new gcp.bigquery.Table("test", {
* deletionProtection: false,
* tableId: "example_table",
* datasetId: test.datasetId,
* schema: `[
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* `,
* });
* const exampleSubscription = new gcp.pubsub.Subscription("example", {
* name: "example-subscription",
* topic: example.id,
* bigqueryConfig: {
* table: pulumi.interpolate`${testTable.project}.${testTable.datasetId}.${testTable.tableId}`,
* },
* });
* const project = gcp.organizations.getProject({});
* const viewer = new gcp.projects.IAMMember("viewer", {
* project: project.then(project => project.projectId),
* role: "roles/bigquery.metadataViewer",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com`),
* });
* const editor = new gcp.projects.IAMMember("editor", {
* project: project.then(project => project.projectId),
* role: "roles/bigquery.dataEditor",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com`),
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.pubsub.Topic("example", name="example-topic")
* test = gcp.bigquery.Dataset("test", dataset_id="example_dataset")
* test_table = gcp.bigquery.Table("test",
* deletion_protection=False,
* table_id="example_table",
* dataset_id=test.dataset_id,
* schema="""[
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* """)
* example_subscription = gcp.pubsub.Subscription("example",
* name="example-subscription",
* topic=example.id,
* bigquery_config=gcp.pubsub.SubscriptionBigqueryConfigArgs(
* table=pulumi.Output.all(test_table.project, test_table.dataset_id, test_table.table_id).apply(lambda project, dataset_id, table_id: f"{project}.{dataset_id}.{table_id}"),
* ))
* project = gcp.organizations.get_project()
* viewer = gcp.projects.IAMMember("viewer",
* project=project.project_id,
* role="roles/bigquery.metadataViewer",
* member=f"serviceAccount:service-{project.number}@gcp-sa-pubsub.iam.gserviceaccount.com")
* editor = gcp.projects.IAMMember("editor",
* project=project.project_id,
* role="roles/bigquery.dataEditor",
* member=f"serviceAccount:service-{project.number}@gcp-sa-pubsub.iam.gserviceaccount.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.PubSub.Topic("example", new()
* {
* Name = "example-topic",
* });
* var test = new Gcp.BigQuery.Dataset("test", new()
* {
* DatasetId = "example_dataset",
* });
* var testTable = new Gcp.BigQuery.Table("test", new()
* {
* DeletionProtection = false,
* TableId = "example_table",
* DatasetId = test.DatasetId,
* Schema = @"[
* {
* ""name"": ""data"",
* ""type"": ""STRING"",
* ""mode"": ""NULLABLE"",
* ""description"": ""The data""
* }
* ]
* ",
* });
* var exampleSubscription = new Gcp.PubSub.Subscription("example", new()
* {
* Name = "example-subscription",
* Topic = example.Id,
* BigqueryConfig = new Gcp.PubSub.Inputs.SubscriptionBigqueryConfigArgs
* {
* Table = Output.Tuple(testTable.Project, testTable.DatasetId, testTable.TableId).Apply(values =>
* {
* var project = values.Item1;
* var datasetId = values.Item2;
* var tableId = values.Item3;
* return $"{project}.{datasetId}.{tableId}";
* }),
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var viewer = new Gcp.Projects.IAMMember("viewer", new()
* {
* Project = project.Apply(getProjectResult => getProjectResult.ProjectId),
* Role = "roles/bigquery.metadataViewer",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-pubsub.iam.gserviceaccount.com",
* });
* var editor = new Gcp.Projects.IAMMember("editor", new()
* {
* Project = project.Apply(getProjectResult => getProjectResult.ProjectId),
* Role = "roles/bigquery.dataEditor",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-pubsub.iam.gserviceaccount.com",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/bigquery"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/projects"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := pubsub.NewTopic(ctx, "example", &pubsub.TopicArgs{
* Name: pulumi.String("example-topic"),
* })
* if err != nil {
* return err
* }
* test, err := bigquery.NewDataset(ctx, "test", &bigquery.DatasetArgs{
* DatasetId: pulumi.String("example_dataset"),
* })
* if err != nil {
* return err
* }
* testTable, err := bigquery.NewTable(ctx, "test", &bigquery.TableArgs{
* DeletionProtection: pulumi.Bool(false),
* TableId: pulumi.String("example_table"),
* DatasetId: test.DatasetId,
* Schema: pulumi.String(`[
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* `),
* })
* if err != nil {
* return err
* }
* _, err = pubsub.NewSubscription(ctx, "example", &pubsub.SubscriptionArgs{
* Name: pulumi.String("example-subscription"),
* Topic: example.ID(),
* BigqueryConfig: &pubsub.SubscriptionBigqueryConfigArgs{
* Table: pulumi.All(testTable.Project, testTable.DatasetId, testTable.TableId).ApplyT(func(_args []interface{}) (string, error) {
* project := _args[0].(string)
* datasetId := _args[1].(string)
* tableId := _args[2].(string)
* return fmt.Sprintf("%v.%v.%v", project, datasetId, tableId), nil
* }).(pulumi.StringOutput),
* },
* })
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = projects.NewIAMMember(ctx, "viewer", &projects.IAMMemberArgs{
* Project: pulumi.String(project.ProjectId),
* Role: pulumi.String("roles/bigquery.metadataViewer"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* _, err = projects.NewIAMMember(ctx, "editor", &projects.IAMMemberArgs{
* Project: pulumi.String(project.ProjectId),
* Role: pulumi.String("roles/bigquery.dataEditor"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import com.pulumi.gcp.bigquery.Dataset;
* import com.pulumi.gcp.bigquery.DatasetArgs;
* import com.pulumi.gcp.bigquery.Table;
* import com.pulumi.gcp.bigquery.TableArgs;
* import com.pulumi.gcp.pubsub.Subscription;
* import com.pulumi.gcp.pubsub.SubscriptionArgs;
* import com.pulumi.gcp.pubsub.inputs.SubscriptionBigqueryConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.projects.IAMMember;
* import com.pulumi.gcp.projects.IAMMemberArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Topic("example", TopicArgs.builder()
* .name("example-topic")
* .build());
* var test = new Dataset("test", DatasetArgs.builder()
* .datasetId("example_dataset")
* .build());
* var testTable = new Table("testTable", TableArgs.builder()
* .deletionProtection(false)
* .tableId("example_table")
* .datasetId(test.datasetId())
* .schema("""
* [
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* """)
* .build());
* var exampleSubscription = new Subscription("exampleSubscription", SubscriptionArgs.builder()
* .name("example-subscription")
* .topic(example.id())
* .bigqueryConfig(SubscriptionBigqueryConfigArgs.builder()
* .table(Output.tuple(testTable.project(), testTable.datasetId(), testTable.tableId()).applyValue(values -> {
* var project = values.t1;
* var datasetId = values.t2;
* var tableId = values.t3;
* return String.format("%s.%s.%s", project,datasetId,tableId);
* }))
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var viewer = new IAMMember("viewer", IAMMemberArgs.builder()
* .project(project.applyValue(getProjectResult -> getProjectResult.projectId()))
* .role("roles/bigquery.metadataViewer")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* var editor = new IAMMember("editor", IAMMemberArgs.builder()
* .project(project.applyValue(getProjectResult -> getProjectResult.projectId()))
* .role("roles/bigquery.dataEditor")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:pubsub:Topic
* properties:
* name: example-topic
* exampleSubscription:
* type: gcp:pubsub:Subscription
* name: example
* properties:
* name: example-subscription
* topic: ${example.id}
* bigqueryConfig:
* table: ${testTable.project}.${testTable.datasetId}.${testTable.tableId}
* viewer:
* type: gcp:projects:IAMMember
* properties:
* project: ${project.projectId}
* role: roles/bigquery.metadataViewer
* member: serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com
* editor:
* type: gcp:projects:IAMMember
* properties:
* project: ${project.projectId}
* role: roles/bigquery.dataEditor
* member: serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com
* test:
* type: gcp:bigquery:Dataset
* properties:
* datasetId: example_dataset
* testTable:
* type: gcp:bigquery:Table
* name: test
* properties:
* deletionProtection: false
* tableId: example_table
* datasetId: ${test.datasetId}
* schema: |
* [
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Pubsub Subscription Push Bq Table Schema
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.pubsub.Topic("example", {name: "example-topic"});
* const test = new gcp.bigquery.Dataset("test", {datasetId: "example_dataset"});
* const testTable = new gcp.bigquery.Table("test", {
* deletionProtection: false,
* tableId: "example_table",
* datasetId: test.datasetId,
* schema: `[
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* `,
* });
* const exampleSubscription = new gcp.pubsub.Subscription("example", {
* name: "example-subscription",
* topic: example.id,
* bigqueryConfig: {
* table: pulumi.interpolate`${testTable.project}.${testTable.datasetId}.${testTable.tableId}`,
* useTableSchema: true,
* },
* });
* const project = gcp.organizations.getProject({});
* const viewer = new gcp.projects.IAMMember("viewer", {
* project: project.then(project => project.projectId),
* role: "roles/bigquery.metadataViewer",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com`),
* });
* const editor = new gcp.projects.IAMMember("editor", {
* project: project.then(project => project.projectId),
* role: "roles/bigquery.dataEditor",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com`),
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.pubsub.Topic("example", name="example-topic")
* test = gcp.bigquery.Dataset("test", dataset_id="example_dataset")
* test_table = gcp.bigquery.Table("test",
* deletion_protection=False,
* table_id="example_table",
* dataset_id=test.dataset_id,
* schema="""[
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* """)
* example_subscription = gcp.pubsub.Subscription("example",
* name="example-subscription",
* topic=example.id,
* bigquery_config=gcp.pubsub.SubscriptionBigqueryConfigArgs(
* table=pulumi.Output.all(test_table.project, test_table.dataset_id, test_table.table_id).apply(lambda project, dataset_id, table_id: f"{project}.{dataset_id}.{table_id}"),
* use_table_schema=True,
* ))
* project = gcp.organizations.get_project()
* viewer = gcp.projects.IAMMember("viewer",
* project=project.project_id,
* role="roles/bigquery.metadataViewer",
* member=f"serviceAccount:service-{project.number}@gcp-sa-pubsub.iam.gserviceaccount.com")
* editor = gcp.projects.IAMMember("editor",
* project=project.project_id,
* role="roles/bigquery.dataEditor",
* member=f"serviceAccount:service-{project.number}@gcp-sa-pubsub.iam.gserviceaccount.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.PubSub.Topic("example", new()
* {
* Name = "example-topic",
* });
* var test = new Gcp.BigQuery.Dataset("test", new()
* {
* DatasetId = "example_dataset",
* });
* var testTable = new Gcp.BigQuery.Table("test", new()
* {
* DeletionProtection = false,
* TableId = "example_table",
* DatasetId = test.DatasetId,
* Schema = @"[
* {
* ""name"": ""data"",
* ""type"": ""STRING"",
* ""mode"": ""NULLABLE"",
* ""description"": ""The data""
* }
* ]
* ",
* });
* var exampleSubscription = new Gcp.PubSub.Subscription("example", new()
* {
* Name = "example-subscription",
* Topic = example.Id,
* BigqueryConfig = new Gcp.PubSub.Inputs.SubscriptionBigqueryConfigArgs
* {
* Table = Output.Tuple(testTable.Project, testTable.DatasetId, testTable.TableId).Apply(values =>
* {
* var project = values.Item1;
* var datasetId = values.Item2;
* var tableId = values.Item3;
* return $"{project}.{datasetId}.{tableId}";
* }),
* UseTableSchema = true,
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var viewer = new Gcp.Projects.IAMMember("viewer", new()
* {
* Project = project.Apply(getProjectResult => getProjectResult.ProjectId),
* Role = "roles/bigquery.metadataViewer",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-pubsub.iam.gserviceaccount.com",
* });
* var editor = new Gcp.Projects.IAMMember("editor", new()
* {
* Project = project.Apply(getProjectResult => getProjectResult.ProjectId),
* Role = "roles/bigquery.dataEditor",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-pubsub.iam.gserviceaccount.com",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/bigquery"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/projects"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := pubsub.NewTopic(ctx, "example", &pubsub.TopicArgs{
* Name: pulumi.String("example-topic"),
* })
* if err != nil {
* return err
* }
* test, err := bigquery.NewDataset(ctx, "test", &bigquery.DatasetArgs{
* DatasetId: pulumi.String("example_dataset"),
* })
* if err != nil {
* return err
* }
* testTable, err := bigquery.NewTable(ctx, "test", &bigquery.TableArgs{
* DeletionProtection: pulumi.Bool(false),
* TableId: pulumi.String("example_table"),
* DatasetId: test.DatasetId,
* Schema: pulumi.String(`[
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* `),
* })
* if err != nil {
* return err
* }
* _, err = pubsub.NewSubscription(ctx, "example", &pubsub.SubscriptionArgs{
* Name: pulumi.String("example-subscription"),
* Topic: example.ID(),
* BigqueryConfig: &pubsub.SubscriptionBigqueryConfigArgs{
* Table: pulumi.All(testTable.Project, testTable.DatasetId, testTable.TableId).ApplyT(func(_args []interface{}) (string, error) {
* project := _args[0].(string)
* datasetId := _args[1].(string)
* tableId := _args[2].(string)
* return fmt.Sprintf("%v.%v.%v", project, datasetId, tableId), nil
* }).(pulumi.StringOutput),
* UseTableSchema: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = projects.NewIAMMember(ctx, "viewer", &projects.IAMMemberArgs{
* Project: pulumi.String(project.ProjectId),
* Role: pulumi.String("roles/bigquery.metadataViewer"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* _, err = projects.NewIAMMember(ctx, "editor", &projects.IAMMemberArgs{
* Project: pulumi.String(project.ProjectId),
* Role: pulumi.String("roles/bigquery.dataEditor"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import com.pulumi.gcp.bigquery.Dataset;
* import com.pulumi.gcp.bigquery.DatasetArgs;
* import com.pulumi.gcp.bigquery.Table;
* import com.pulumi.gcp.bigquery.TableArgs;
* import com.pulumi.gcp.pubsub.Subscription;
* import com.pulumi.gcp.pubsub.SubscriptionArgs;
* import com.pulumi.gcp.pubsub.inputs.SubscriptionBigqueryConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.projects.IAMMember;
* import com.pulumi.gcp.projects.IAMMemberArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Topic("example", TopicArgs.builder()
* .name("example-topic")
* .build());
* var test = new Dataset("test", DatasetArgs.builder()
* .datasetId("example_dataset")
* .build());
* var testTable = new Table("testTable", TableArgs.builder()
* .deletionProtection(false)
* .tableId("example_table")
* .datasetId(test.datasetId())
* .schema("""
* [
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* """)
* .build());
* var exampleSubscription = new Subscription("exampleSubscription", SubscriptionArgs.builder()
* .name("example-subscription")
* .topic(example.id())
* .bigqueryConfig(SubscriptionBigqueryConfigArgs.builder()
* .table(Output.tuple(testTable.project(), testTable.datasetId(), testTable.tableId()).applyValue(values -> {
* var project = values.t1;
* var datasetId = values.t2;
* var tableId = values.t3;
* return String.format("%s.%s.%s", project,datasetId,tableId);
* }))
* .useTableSchema(true)
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var viewer = new IAMMember("viewer", IAMMemberArgs.builder()
* .project(project.applyValue(getProjectResult -> getProjectResult.projectId()))
* .role("roles/bigquery.metadataViewer")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* var editor = new IAMMember("editor", IAMMemberArgs.builder()
* .project(project.applyValue(getProjectResult -> getProjectResult.projectId()))
* .role("roles/bigquery.dataEditor")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:pubsub:Topic
* properties:
* name: example-topic
* exampleSubscription:
* type: gcp:pubsub:Subscription
* name: example
* properties:
* name: example-subscription
* topic: ${example.id}
* bigqueryConfig:
* table: ${testTable.project}.${testTable.datasetId}.${testTable.tableId}
* useTableSchema: true
* viewer:
* type: gcp:projects:IAMMember
* properties:
* project: ${project.projectId}
* role: roles/bigquery.metadataViewer
* member: serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com
* editor:
* type: gcp:projects:IAMMember
* properties:
* project: ${project.projectId}
* role: roles/bigquery.dataEditor
* member: serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com
* test:
* type: gcp:bigquery:Dataset
* properties:
* datasetId: example_dataset
* testTable:
* type: gcp:bigquery:Table
* name: test
* properties:
* deletionProtection: false
* tableId: example_table
* datasetId: ${test.datasetId}
* schema: |
* [
* {
* "name": "data",
* "type": "STRING",
* "mode": "NULLABLE",
* "description": "The data"
* }
* ]
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Pubsub Subscription Push Cloudstorage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.storage.Bucket("example", {
* name: "example-bucket",
* location: "US",
* uniformBucketLevelAccess: true,
* });
* const exampleTopic = new gcp.pubsub.Topic("example", {name: "example-topic"});
* const exampleSubscription = new gcp.pubsub.Subscription("example", {
* name: "example-subscription",
* topic: exampleTopic.id,
* cloudStorageConfig: {
* bucket: example.name,
* filenamePrefix: "pre-",
* filenameSuffix: "-_2067",
* maxBytes: 1000,
* maxDuration: "300s",
* },
* });
* const project = gcp.organizations.getProject({});
* const admin = new gcp.storage.BucketIAMMember("admin", {
* bucket: example.name,
* role: "roles/storage.admin",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com`),
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.storage.Bucket("example",
* name="example-bucket",
* location="US",
* uniform_bucket_level_access=True)
* example_topic = gcp.pubsub.Topic("example", name="example-topic")
* example_subscription = gcp.pubsub.Subscription("example",
* name="example-subscription",
* topic=example_topic.id,
* cloud_storage_config=gcp.pubsub.SubscriptionCloudStorageConfigArgs(
* bucket=example.name,
* filename_prefix="pre-",
* filename_suffix="-_2067",
* max_bytes=1000,
* max_duration="300s",
* ))
* project = gcp.organizations.get_project()
* admin = gcp.storage.BucketIAMMember("admin",
* bucket=example.name,
* role="roles/storage.admin",
* member=f"serviceAccount:service-{project.number}@gcp-sa-pubsub.iam.gserviceaccount.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.Storage.Bucket("example", new()
* {
* Name = "example-bucket",
* Location = "US",
* UniformBucketLevelAccess = true,
* });
* var exampleTopic = new Gcp.PubSub.Topic("example", new()
* {
* Name = "example-topic",
* });
* var exampleSubscription = new Gcp.PubSub.Subscription("example", new()
* {
* Name = "example-subscription",
* Topic = exampleTopic.Id,
* CloudStorageConfig = new Gcp.PubSub.Inputs.SubscriptionCloudStorageConfigArgs
* {
* Bucket = example.Name,
* FilenamePrefix = "pre-",
* FilenameSuffix = "-_2067",
* MaxBytes = 1000,
* MaxDuration = "300s",
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var admin = new Gcp.Storage.BucketIAMMember("admin", new()
* {
* Bucket = example.Name,
* Role = "roles/storage.admin",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-pubsub.iam.gserviceaccount.com",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := storage.NewBucket(ctx, "example", &storage.BucketArgs{
* Name: pulumi.String("example-bucket"),
* Location: pulumi.String("US"),
* UniformBucketLevelAccess: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* exampleTopic, err := pubsub.NewTopic(ctx, "example", &pubsub.TopicArgs{
* Name: pulumi.String("example-topic"),
* })
* if err != nil {
* return err
* }
* _, err = pubsub.NewSubscription(ctx, "example", &pubsub.SubscriptionArgs{
* Name: pulumi.String("example-subscription"),
* Topic: exampleTopic.ID(),
* CloudStorageConfig: &pubsub.SubscriptionCloudStorageConfigArgs{
* Bucket: example.Name,
* FilenamePrefix: pulumi.String("pre-"),
* FilenameSuffix: pulumi.String("-_2067"),
* MaxBytes: pulumi.Int(1000),
* MaxDuration: pulumi.String("300s"),
* },
* })
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = storage.NewBucketIAMMember(ctx, "admin", &storage.BucketIAMMemberArgs{
* Bucket: example.Name,
* Role: pulumi.String("roles/storage.admin"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import com.pulumi.gcp.pubsub.Subscription;
* import com.pulumi.gcp.pubsub.SubscriptionArgs;
* import com.pulumi.gcp.pubsub.inputs.SubscriptionCloudStorageConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.storage.BucketIAMMember;
* import com.pulumi.gcp.storage.BucketIAMMemberArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Bucket("example", BucketArgs.builder()
* .name("example-bucket")
* .location("US")
* .uniformBucketLevelAccess(true)
* .build());
* var exampleTopic = new Topic("exampleTopic", TopicArgs.builder()
* .name("example-topic")
* .build());
* var exampleSubscription = new Subscription("exampleSubscription", SubscriptionArgs.builder()
* .name("example-subscription")
* .topic(exampleTopic.id())
* .cloudStorageConfig(SubscriptionCloudStorageConfigArgs.builder()
* .bucket(example.name())
* .filenamePrefix("pre-")
* .filenameSuffix("-_2067")
* .maxBytes(1000)
* .maxDuration("300s")
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var admin = new BucketIAMMember("admin", BucketIAMMemberArgs.builder()
* .bucket(example.name())
* .role("roles/storage.admin")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:storage:Bucket
* properties:
* name: example-bucket
* location: US
* uniformBucketLevelAccess: true
* exampleTopic:
* type: gcp:pubsub:Topic
* name: example
* properties:
* name: example-topic
* exampleSubscription:
* type: gcp:pubsub:Subscription
* name: example
* properties:
* name: example-subscription
* topic: ${exampleTopic.id}
* cloudStorageConfig:
* bucket: ${example.name}
* filenamePrefix: pre-
* filenameSuffix: -_2067
* maxBytes: 1000
* maxDuration: 300s
* admin:
* type: gcp:storage:BucketIAMMember
* properties:
* bucket: ${example.name}
* role: roles/storage.admin
* member: serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Pubsub Subscription Push Cloudstorage Avro
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.storage.Bucket("example", {
* name: "example-bucket",
* location: "US",
* uniformBucketLevelAccess: true,
* });
* const exampleTopic = new gcp.pubsub.Topic("example", {name: "example-topic"});
* const exampleSubscription = new gcp.pubsub.Subscription("example", {
* name: "example-subscription",
* topic: exampleTopic.id,
* cloudStorageConfig: {
* bucket: example.name,
* filenamePrefix: "pre-",
* filenameSuffix: "-_40785",
* maxBytes: 1000,
* maxDuration: "300s",
* avroConfig: {
* writeMetadata: true,
* },
* },
* });
* const project = gcp.organizations.getProject({});
* const admin = new gcp.storage.BucketIAMMember("admin", {
* bucket: example.name,
* role: "roles/storage.admin",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com`),
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.storage.Bucket("example",
* name="example-bucket",
* location="US",
* uniform_bucket_level_access=True)
* example_topic = gcp.pubsub.Topic("example", name="example-topic")
* example_subscription = gcp.pubsub.Subscription("example",
* name="example-subscription",
* topic=example_topic.id,
* cloud_storage_config=gcp.pubsub.SubscriptionCloudStorageConfigArgs(
* bucket=example.name,
* filename_prefix="pre-",
* filename_suffix="-_40785",
* max_bytes=1000,
* max_duration="300s",
* avro_config=gcp.pubsub.SubscriptionCloudStorageConfigAvroConfigArgs(
* write_metadata=True,
* ),
* ))
* project = gcp.organizations.get_project()
* admin = gcp.storage.BucketIAMMember("admin",
* bucket=example.name,
* role="roles/storage.admin",
* member=f"serviceAccount:service-{project.number}@gcp-sa-pubsub.iam.gserviceaccount.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.Storage.Bucket("example", new()
* {
* Name = "example-bucket",
* Location = "US",
* UniformBucketLevelAccess = true,
* });
* var exampleTopic = new Gcp.PubSub.Topic("example", new()
* {
* Name = "example-topic",
* });
* var exampleSubscription = new Gcp.PubSub.Subscription("example", new()
* {
* Name = "example-subscription",
* Topic = exampleTopic.Id,
* CloudStorageConfig = new Gcp.PubSub.Inputs.SubscriptionCloudStorageConfigArgs
* {
* Bucket = example.Name,
* FilenamePrefix = "pre-",
* FilenameSuffix = "-_40785",
* MaxBytes = 1000,
* MaxDuration = "300s",
* AvroConfig = new Gcp.PubSub.Inputs.SubscriptionCloudStorageConfigAvroConfigArgs
* {
* WriteMetadata = true,
* },
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var admin = new Gcp.Storage.BucketIAMMember("admin", new()
* {
* Bucket = example.Name,
* Role = "roles/storage.admin",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-pubsub.iam.gserviceaccount.com",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := storage.NewBucket(ctx, "example", &storage.BucketArgs{
* Name: pulumi.String("example-bucket"),
* Location: pulumi.String("US"),
* UniformBucketLevelAccess: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* exampleTopic, err := pubsub.NewTopic(ctx, "example", &pubsub.TopicArgs{
* Name: pulumi.String("example-topic"),
* })
* if err != nil {
* return err
* }
* _, err = pubsub.NewSubscription(ctx, "example", &pubsub.SubscriptionArgs{
* Name: pulumi.String("example-subscription"),
* Topic: exampleTopic.ID(),
* CloudStorageConfig: &pubsub.SubscriptionCloudStorageConfigArgs{
* Bucket: example.Name,
* FilenamePrefix: pulumi.String("pre-"),
* FilenameSuffix: pulumi.String("-_40785"),
* MaxBytes: pulumi.Int(1000),
* MaxDuration: pulumi.String("300s"),
* AvroConfig: &pubsub.SubscriptionCloudStorageConfigAvroConfigArgs{
* WriteMetadata: pulumi.Bool(true),
* },
* },
* })
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = storage.NewBucketIAMMember(ctx, "admin", &storage.BucketIAMMemberArgs{
* Bucket: example.Name,
* Role: pulumi.String("roles/storage.admin"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import com.pulumi.gcp.pubsub.Subscription;
* import com.pulumi.gcp.pubsub.SubscriptionArgs;
* import com.pulumi.gcp.pubsub.inputs.SubscriptionCloudStorageConfigArgs;
* import com.pulumi.gcp.pubsub.inputs.SubscriptionCloudStorageConfigAvroConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.storage.BucketIAMMember;
* import com.pulumi.gcp.storage.BucketIAMMemberArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Bucket("example", BucketArgs.builder()
* .name("example-bucket")
* .location("US")
* .uniformBucketLevelAccess(true)
* .build());
* var exampleTopic = new Topic("exampleTopic", TopicArgs.builder()
* .name("example-topic")
* .build());
* var exampleSubscription = new Subscription("exampleSubscription", SubscriptionArgs.builder()
* .name("example-subscription")
* .topic(exampleTopic.id())
* .cloudStorageConfig(SubscriptionCloudStorageConfigArgs.builder()
* .bucket(example.name())
* .filenamePrefix("pre-")
* .filenameSuffix("-_40785")
* .maxBytes(1000)
* .maxDuration("300s")
* .avroConfig(SubscriptionCloudStorageConfigAvroConfigArgs.builder()
* .writeMetadata(true)
* .build())
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var admin = new BucketIAMMember("admin", BucketIAMMemberArgs.builder()
* .bucket(example.name())
* .role("roles/storage.admin")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:storage:Bucket
* properties:
* name: example-bucket
* location: US
* uniformBucketLevelAccess: true
* exampleTopic:
* type: gcp:pubsub:Topic
* name: example
* properties:
* name: example-topic
* exampleSubscription:
* type: gcp:pubsub:Subscription
* name: example
* properties:
* name: example-subscription
* topic: ${exampleTopic.id}
* cloudStorageConfig:
* bucket: ${example.name}
* filenamePrefix: pre-
* filenameSuffix: -_40785
* maxBytes: 1000
* maxDuration: 300s
* avroConfig:
* writeMetadata: true
* admin:
* type: gcp:storage:BucketIAMMember
* properties:
* bucket: ${example.name}
* role: roles/storage.admin
* member: serviceAccount:service-${project.number}@gcp-sa-pubsub.iam.gserviceaccount.com
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* Subscription can be imported using any of these accepted formats:
* * `projects/{{project}}/subscriptions/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, Subscription can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:pubsub/subscription:Subscription default projects/{{project}}/subscriptions/{{name}}
* ```
* ```sh
* $ pulumi import gcp:pubsub/subscription:Subscription default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:pubsub/subscription:Subscription default {{name}}
* ```
* @property ackDeadlineSeconds This value is the maximum time after a subscriber receives a message
* before the subscriber should acknowledge the message. After message
* delivery but before the ack deadline expires and before the message is
* acknowledged, it is an outstanding message and will not be delivered
* again during that time (on a best-effort basis).
* For pull subscriptions, this value is used as the initial value for
* the ack deadline. To override this value for a given message, call
* subscriptions.modifyAckDeadline with the corresponding ackId if using
* pull. The minimum custom deadline you can specify is 10 seconds. The
* maximum custom deadline you can specify is 600 seconds (10 minutes).
* If this parameter is 0, a default value of 10 seconds is used.
* For push delivery, this value is also used to set the request timeout
* for the call to the push endpoint.
* If the subscriber never acknowledges the message, the Pub/Sub system
* will eventually redeliver the message.
* @property bigqueryConfig If delivery to BigQuery is used with this subscription, this field is used to configure it.
* Either pushConfig, bigQueryConfig or cloudStorageConfig can be set, but not combined.
* If all three are empty, then the subscriber will pull and ack messages using API methods.
* Structure is documented below.
* @property cloudStorageConfig If delivery to Cloud Storage is used with this subscription, this field is used to configure it.
* Either pushConfig, bigQueryConfig or cloudStorageConfig can be set, but not combined.
* If all three are empty, then the subscriber will pull and ack messages using API methods.
* Structure is documented below.
* @property deadLetterPolicy A policy that specifies the conditions for dead lettering messages in
* this subscription. If dead_letter_policy is not set, dead lettering
* is disabled.
* The Cloud Pub/Sub service account associated with this subscription's
* parent project (i.e.,
* service-{project_number}@gcp-sa-pubsub.iam.gserviceaccount.com) must have
* permission to Acknowledge() messages on this subscription.
* Structure is documented below.
* @property enableExactlyOnceDelivery If `true`, Pub/Sub provides the following guarantees for the delivery
* of a message with a given value of messageId on this Subscriptions':
* - The message sent to a subscriber is guaranteed not to be resent before the message's acknowledgement deadline expires.
* - An acknowledged message will not be resent to a subscriber.
* Note that subscribers may still receive multiple copies of a message when `enable_exactly_once_delivery`
* is true if the message was published multiple times by a publisher client. These copies are considered distinct by Pub/Sub and have distinct messageId values
* @property enableMessageOrdering If `true`, messages published with the same orderingKey in PubsubMessage will be delivered to
* the subscribers in the order in which they are received by the Pub/Sub system. Otherwise, they
* may be delivered in any order.
* @property expirationPolicy A policy that specifies the conditions for this subscription's expiration.
* A subscription is considered active as long as any connected subscriber
* is successfully consuming messages from the subscription or is issuing
* operations on the subscription. If expirationPolicy is not set, a default
* policy with ttl of 31 days will be used. If it is set but ttl is "", the
* resource never expires. The minimum allowed value for expirationPolicy.ttl
* is 1 day.
* Structure is documented below.
* @property filter The subscription only delivers the messages that match the filter.
* Pub/Sub automatically acknowledges the messages that don't match the filter. You can filter messages
* by their attributes. The maximum length of a filter is 256 bytes. After creating the subscription,
* you can't modify the filter.
* @property labels A set of key/value label pairs to assign to this Subscription.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property messageRetentionDuration How long to retain unacknowledged messages in the subscription's
* backlog, from the moment a message is published. If
* retain_acked_messages is true, then this also configures the retention
* of acknowledged messages, and thus configures how far back in time a
* subscriptions.seek can be done. Defaults to 7 days. Cannot be more
* than 7 days (`"604800s"`) or less than 10 minutes (`"600s"`).
* A duration in seconds with up to nine fractional digits, terminated
* by 's'. Example: `"600.5s"`.
* @property name Name of the subscription.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property pushConfig If push delivery is used with this subscription, this field is used to
* configure it. An empty pushConfig signifies that the subscriber will
* pull and ack messages using API methods.
* Structure is documented below.
* @property retainAckedMessages Indicates whether to retain acknowledged messages. If `true`, then
* messages are not expunged from the subscription's backlog, even if
* they are acknowledged, until they fall out of the
* messageRetentionDuration window.
* @property retryPolicy A policy that specifies how Pub/Sub retries message delivery for this subscription.
* If not set, the default retry policy is applied. This generally implies that messages will be retried as soon as possible for healthy subscribers.
* RetryPolicy will be triggered on NACKs or acknowledgement deadline exceeded events for a given message
* Structure is documented below.
* @property topic A reference to a Topic resource, of the form projects/{project}/topics/{{name}}
* (as in the id property of a google_pubsub_topic), or just a topic name if
* the topic is in the same project as the subscription.
* - - -
*/
public data class SubscriptionArgs(
public val ackDeadlineSeconds: Output? = null,
public val bigqueryConfig: Output? = null,
public val cloudStorageConfig: Output? = null,
public val deadLetterPolicy: Output? = null,
public val enableExactlyOnceDelivery: Output? = null,
public val enableMessageOrdering: Output? = null,
public val expirationPolicy: Output? = null,
public val filter: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy