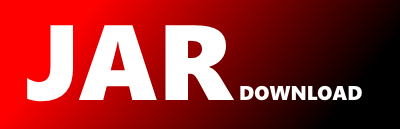
com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionBigqueryConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.pubsub.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.pubsub.inputs.SubscriptionBigqueryConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property dropUnknownFields When true and use_topic_schema or use_table_schema is true, any fields that are a part of the topic schema or message schema that
* are not part of the BigQuery table schema are dropped when writing to BigQuery. Otherwise, the schemas must be kept in sync
* and any messages with extra fields are not written and remain in the subscription's backlog.
* @property table The name of the table to which to write data, of the form {projectId}:{datasetId}.{tableId}
* @property useTableSchema When true, use the BigQuery table's schema as the columns to write to in BigQuery. Messages
* must be published in JSON format. Only one of use_topic_schema and use_table_schema can be set.
* @property useTopicSchema When true, use the topic's schema as the columns to write to in BigQuery, if it exists.
* Only one of use_topic_schema and use_table_schema can be set.
* @property writeMetadata When true, write the subscription name, messageId, publishTime, attributes, and orderingKey to additional columns in the table.
* The subscription name, messageId, and publishTime fields are put in their own columns while all other message properties (other than data) are written to a JSON object in the attributes column.
*/
public data class SubscriptionBigqueryConfigArgs(
public val dropUnknownFields: Output? = null,
public val table: Output,
public val useTableSchema: Output? = null,
public val useTopicSchema: Output? = null,
public val writeMetadata: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.pubsub.inputs.SubscriptionBigqueryConfigArgs =
com.pulumi.gcp.pubsub.inputs.SubscriptionBigqueryConfigArgs.builder()
.dropUnknownFields(dropUnknownFields?.applyValue({ args0 -> args0 }))
.table(table.applyValue({ args0 -> args0 }))
.useTableSchema(useTableSchema?.applyValue({ args0 -> args0 }))
.useTopicSchema(useTopicSchema?.applyValue({ args0 -> args0 }))
.writeMetadata(writeMetadata?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SubscriptionBigqueryConfigArgs].
*/
@PulumiTagMarker
public class SubscriptionBigqueryConfigArgsBuilder internal constructor() {
private var dropUnknownFields: Output? = null
private var table: Output? = null
private var useTableSchema: Output? = null
private var useTopicSchema: Output? = null
private var writeMetadata: Output? = null
/**
* @param value When true and use_topic_schema or use_table_schema is true, any fields that are a part of the topic schema or message schema that
* are not part of the BigQuery table schema are dropped when writing to BigQuery. Otherwise, the schemas must be kept in sync
* and any messages with extra fields are not written and remain in the subscription's backlog.
*/
@JvmName("lnfncrrrchwbimbw")
public suspend fun dropUnknownFields(`value`: Output) {
this.dropUnknownFields = value
}
/**
* @param value The name of the table to which to write data, of the form {projectId}:{datasetId}.{tableId}
*/
@JvmName("qtxkiaedjofxcofr")
public suspend fun table(`value`: Output) {
this.table = value
}
/**
* @param value When true, use the BigQuery table's schema as the columns to write to in BigQuery. Messages
* must be published in JSON format. Only one of use_topic_schema and use_table_schema can be set.
*/
@JvmName("pgavlwhnkktatiie")
public suspend fun useTableSchema(`value`: Output) {
this.useTableSchema = value
}
/**
* @param value When true, use the topic's schema as the columns to write to in BigQuery, if it exists.
* Only one of use_topic_schema and use_table_schema can be set.
*/
@JvmName("jfgbknfsgmbxshjf")
public suspend fun useTopicSchema(`value`: Output) {
this.useTopicSchema = value
}
/**
* @param value When true, write the subscription name, messageId, publishTime, attributes, and orderingKey to additional columns in the table.
* The subscription name, messageId, and publishTime fields are put in their own columns while all other message properties (other than data) are written to a JSON object in the attributes column.
*/
@JvmName("ifrgkqiwmnehuhff")
public suspend fun writeMetadata(`value`: Output) {
this.writeMetadata = value
}
/**
* @param value When true and use_topic_schema or use_table_schema is true, any fields that are a part of the topic schema or message schema that
* are not part of the BigQuery table schema are dropped when writing to BigQuery. Otherwise, the schemas must be kept in sync
* and any messages with extra fields are not written and remain in the subscription's backlog.
*/
@JvmName("lptjrveoycgevqir")
public suspend fun dropUnknownFields(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dropUnknownFields = mapped
}
/**
* @param value The name of the table to which to write data, of the form {projectId}:{datasetId}.{tableId}
*/
@JvmName("hymklqssmpbscfnn")
public suspend fun table(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.table = mapped
}
/**
* @param value When true, use the BigQuery table's schema as the columns to write to in BigQuery. Messages
* must be published in JSON format. Only one of use_topic_schema and use_table_schema can be set.
*/
@JvmName("hmsimeicpwcecwio")
public suspend fun useTableSchema(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useTableSchema = mapped
}
/**
* @param value When true, use the topic's schema as the columns to write to in BigQuery, if it exists.
* Only one of use_topic_schema and use_table_schema can be set.
*/
@JvmName("avxaabbnghbkswlj")
public suspend fun useTopicSchema(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useTopicSchema = mapped
}
/**
* @param value When true, write the subscription name, messageId, publishTime, attributes, and orderingKey to additional columns in the table.
* The subscription name, messageId, and publishTime fields are put in their own columns while all other message properties (other than data) are written to a JSON object in the attributes column.
*/
@JvmName("mcqjmagnrmlfiavy")
public suspend fun writeMetadata(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.writeMetadata = mapped
}
internal fun build(): SubscriptionBigqueryConfigArgs = SubscriptionBigqueryConfigArgs(
dropUnknownFields = dropUnknownFields,
table = table ?: throw PulumiNullFieldException("table"),
useTableSchema = useTableSchema,
useTopicSchema = useTopicSchema,
writeMetadata = writeMetadata,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy