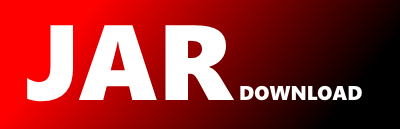
com.pulumi.gcp.pubsub.kotlin.inputs.SubscriptionCloudStorageConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.pubsub.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.pubsub.inputs.SubscriptionCloudStorageConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property avroConfig If set, message data will be written to Cloud Storage in Avro format.
* Structure is documented below.
* @property bucket User-provided name for the Cloud Storage bucket. The bucket must be created by the user. The bucket name must be without any prefix like "gs://".
* @property filenamePrefix User-provided prefix for Cloud Storage filename.
* @property filenameSuffix User-provided suffix for Cloud Storage filename. Must not end in "/".
* @property maxBytes The maximum bytes that can be written to a Cloud Storage file before a new file is created. Min 1 KB, max 10 GiB.
* The maxBytes limit may be exceeded in cases where messages are larger than the limit.
* @property maxDuration The maximum duration that can elapse before a new Cloud Storage file is created. Min 1 minute, max 10 minutes, default 5 minutes.
* May not exceed the subscription's acknowledgement deadline.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
* @property state (Output)
* An output-only field that indicates whether or not the subscription can receive messages.
*/
public data class SubscriptionCloudStorageConfigArgs(
public val avroConfig: Output? = null,
public val bucket: Output,
public val filenamePrefix: Output? = null,
public val filenameSuffix: Output? = null,
public val maxBytes: Output? = null,
public val maxDuration: Output? = null,
public val state: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.pubsub.inputs.SubscriptionCloudStorageConfigArgs =
com.pulumi.gcp.pubsub.inputs.SubscriptionCloudStorageConfigArgs.builder()
.avroConfig(avroConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.bucket(bucket.applyValue({ args0 -> args0 }))
.filenamePrefix(filenamePrefix?.applyValue({ args0 -> args0 }))
.filenameSuffix(filenameSuffix?.applyValue({ args0 -> args0 }))
.maxBytes(maxBytes?.applyValue({ args0 -> args0 }))
.maxDuration(maxDuration?.applyValue({ args0 -> args0 }))
.state(state?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SubscriptionCloudStorageConfigArgs].
*/
@PulumiTagMarker
public class SubscriptionCloudStorageConfigArgsBuilder internal constructor() {
private var avroConfig: Output? = null
private var bucket: Output? = null
private var filenamePrefix: Output? = null
private var filenameSuffix: Output? = null
private var maxBytes: Output? = null
private var maxDuration: Output? = null
private var state: Output? = null
/**
* @param value If set, message data will be written to Cloud Storage in Avro format.
* Structure is documented below.
*/
@JvmName("vpijogmtrrniyxxy")
public suspend fun avroConfig(`value`: Output) {
this.avroConfig = value
}
/**
* @param value User-provided name for the Cloud Storage bucket. The bucket must be created by the user. The bucket name must be without any prefix like "gs://".
*/
@JvmName("teqhxgegsvjuxata")
public suspend fun bucket(`value`: Output) {
this.bucket = value
}
/**
* @param value User-provided prefix for Cloud Storage filename.
*/
@JvmName("xplotdiuwwlikyke")
public suspend fun filenamePrefix(`value`: Output) {
this.filenamePrefix = value
}
/**
* @param value User-provided suffix for Cloud Storage filename. Must not end in "/".
*/
@JvmName("anuxrwgtvemkpdix")
public suspend fun filenameSuffix(`value`: Output) {
this.filenameSuffix = value
}
/**
* @param value The maximum bytes that can be written to a Cloud Storage file before a new file is created. Min 1 KB, max 10 GiB.
* The maxBytes limit may be exceeded in cases where messages are larger than the limit.
*/
@JvmName("tjewgriofbstubpx")
public suspend fun maxBytes(`value`: Output) {
this.maxBytes = value
}
/**
* @param value The maximum duration that can elapse before a new Cloud Storage file is created. Min 1 minute, max 10 minutes, default 5 minutes.
* May not exceed the subscription's acknowledgement deadline.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
*/
@JvmName("ifiywtaphbbfpvry")
public suspend fun maxDuration(`value`: Output) {
this.maxDuration = value
}
/**
* @param value (Output)
* An output-only field that indicates whether or not the subscription can receive messages.
*/
@JvmName("ugccufqyxdlegpeg")
public suspend fun state(`value`: Output) {
this.state = value
}
/**
* @param value If set, message data will be written to Cloud Storage in Avro format.
* Structure is documented below.
*/
@JvmName("qadomfwowhgfxqbm")
public suspend fun avroConfig(`value`: SubscriptionCloudStorageConfigAvroConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.avroConfig = mapped
}
/**
* @param argument If set, message data will be written to Cloud Storage in Avro format.
* Structure is documented below.
*/
@JvmName("lrycjblkcbirpabb")
public suspend fun avroConfig(argument: suspend SubscriptionCloudStorageConfigAvroConfigArgsBuilder.() -> Unit) {
val toBeMapped = SubscriptionCloudStorageConfigAvroConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.avroConfig = mapped
}
/**
* @param value User-provided name for the Cloud Storage bucket. The bucket must be created by the user. The bucket name must be without any prefix like "gs://".
*/
@JvmName("uupcxcjaqafduqcr")
public suspend fun bucket(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bucket = mapped
}
/**
* @param value User-provided prefix for Cloud Storage filename.
*/
@JvmName("dfvlheofbavvbrdt")
public suspend fun filenamePrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filenamePrefix = mapped
}
/**
* @param value User-provided suffix for Cloud Storage filename. Must not end in "/".
*/
@JvmName("buvtafudkfbnolhk")
public suspend fun filenameSuffix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filenameSuffix = mapped
}
/**
* @param value The maximum bytes that can be written to a Cloud Storage file before a new file is created. Min 1 KB, max 10 GiB.
* The maxBytes limit may be exceeded in cases where messages are larger than the limit.
*/
@JvmName("xutdfcaxbyguxqbo")
public suspend fun maxBytes(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxBytes = mapped
}
/**
* @param value The maximum duration that can elapse before a new Cloud Storage file is created. Min 1 minute, max 10 minutes, default 5 minutes.
* May not exceed the subscription's acknowledgement deadline.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
*/
@JvmName("jjtpwqtluoysbylo")
public suspend fun maxDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxDuration = mapped
}
/**
* @param value (Output)
* An output-only field that indicates whether or not the subscription can receive messages.
*/
@JvmName("aubbjwwjemxxmvni")
public suspend fun state(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
internal fun build(): SubscriptionCloudStorageConfigArgs = SubscriptionCloudStorageConfigArgs(
avroConfig = avroConfig,
bucket = bucket ?: throw PulumiNullFieldException("bucket"),
filenamePrefix = filenamePrefix,
filenameSuffix = filenameSuffix,
maxBytes = maxBytes,
maxDuration = maxDuration,
state = state,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy