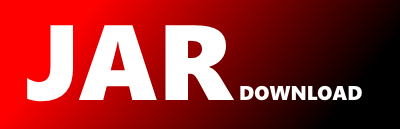
com.pulumi.gcp.securitycenter.kotlin.EventThreatDetectionCustomModule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.securitycenter.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [EventThreatDetectionCustomModule].
*/
@PulumiTagMarker
public class EventThreatDetectionCustomModuleResourceBuilder internal constructor() {
public var name: String? = null
public var args: EventThreatDetectionCustomModuleArgs = EventThreatDetectionCustomModuleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EventThreatDetectionCustomModuleArgsBuilder.() -> Unit) {
val builder = EventThreatDetectionCustomModuleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): EventThreatDetectionCustomModule {
val builtJavaResource =
com.pulumi.gcp.securitycenter.EventThreatDetectionCustomModule(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return EventThreatDetectionCustomModule(builtJavaResource)
}
}
/**
* Represents an instance of an Event Threat Detection custom module, including
* its full module name, display name, enablement state, andlast updated time.
* You can create a custom module at the organization level only.
* To get more information about EventThreatDetectionCustomModule, see:
* * [API documentation](https://cloud.google.com/security-command-center/docs/reference/rest/v1/organizations.eventThreatDetectionSettings.customModules)
* * How-to Guides
* * [Overview of custom modules for Event Threat Detection](https://cloud.google.com/security-command-center/docs/custom-modules-etd-overview)
* ## Example Usage
* ### Scc Event Threat Detection Custom Module
*
* ```yaml
* resources:
* example:
* type: gcp:securitycenter:EventThreatDetectionCustomModule
* properties:
* organization: '123456789'
* displayName: basic_custom_module
* enablementState: ENABLED
* type: CONFIGURABLE_BAD_IP
* description: My Event Threat Detection Custom Module
* config:
* fn::toJSON:
* metadata:
* severity: LOW
* description: Flagged by Forcepoint as malicious
* recommendation: Contact the owner of the relevant project.
* ips:
* - 192.0.2.1
* - 192.0.2.0/24
* ```
*
* ## Import
* EventThreatDetectionCustomModule can be imported using any of these accepted formats:
* * `organizations/{{organization}}/eventThreatDetectionSettings/customModules/{{name}}`
* * `{{organization}}/{{name}}`
* When using the `pulumi import` command, EventThreatDetectionCustomModule can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:securitycenter/eventThreatDetectionCustomModule:EventThreatDetectionCustomModule default organizations/{{organization}}/eventThreatDetectionSettings/customModules/{{name}}
* ```
* ```sh
* $ pulumi import gcp:securitycenter/eventThreatDetectionCustomModule:EventThreatDetectionCustomModule default {{organization}}/{{name}}
* ```
*/
public class EventThreatDetectionCustomModule internal constructor(
override val javaResource: com.pulumi.gcp.securitycenter.EventThreatDetectionCustomModule,
) : KotlinCustomResource(javaResource, EventThreatDetectionCustomModuleMapper) {
/**
* Config for the module. For the resident module, its config value is defined at this level.
* For the inherited module, its config value is inherited from the ancestor module.
*/
public val config: Output
get() = javaResource.config().applyValue({ args0 -> args0 })
/**
* The human readable name to be displayed for the module.
*/
public val displayName: Output?
get() = javaResource.displayName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The state of enablement for the module at the given level of the hierarchy.
* Possible values are: `ENABLED`, `DISABLED`.
*/
public val enablementState: Output
get() = javaResource.enablementState().applyValue({ args0 -> args0 })
/**
* The editor that last updated the custom module
*/
public val lastEditor: Output
get() = javaResource.lastEditor().applyValue({ args0 -> args0 })
/**
* The resource name of the Event Threat Detection custom module.
* Its format is "organizations/{organization}/eventThreatDetectionSettings/customModules/{module}".
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Numerical ID of the parent organization.
* - - -
*/
public val organization: Output
get() = javaResource.organization().applyValue({ args0 -> args0 })
/**
* Immutable. Type for the module. e.g. CONFIGURABLE_BAD_IP.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
/**
* The time at which the custom module was last updated.
* A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and
* up to nine fractional digits. Examples: "2014-10-02T15:01:23Z" and "2014-10-02T15:01:23.045123456Z".
*/
public val updateTime: Output
get() = javaResource.updateTime().applyValue({ args0 -> args0 })
}
public object EventThreatDetectionCustomModuleMapper :
ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.securitycenter.EventThreatDetectionCustomModule::class == javaResource::class
override fun map(javaResource: Resource): EventThreatDetectionCustomModule =
EventThreatDetectionCustomModule(
javaResource as
com.pulumi.gcp.securitycenter.EventThreatDetectionCustomModule,
)
}
/**
* @see [EventThreatDetectionCustomModule].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [EventThreatDetectionCustomModule].
*/
public suspend fun eventThreatDetectionCustomModule(
name: String,
block: suspend EventThreatDetectionCustomModuleResourceBuilder.() -> Unit,
): EventThreatDetectionCustomModule {
val builder = EventThreatDetectionCustomModuleResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [EventThreatDetectionCustomModule].
* @param name The _unique_ name of the resulting resource.
*/
public fun eventThreatDetectionCustomModule(name: String): EventThreatDetectionCustomModule {
val builder = EventThreatDetectionCustomModuleResourceBuilder()
builder.name(name)
return builder.build()
}