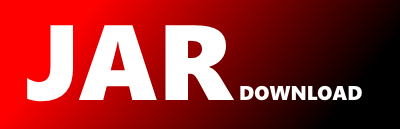
com.pulumi.gcp.securityposture.kotlin.PostureDeploymentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.securityposture.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.securityposture.PostureDeploymentArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Represents a deployment of a security posture on a resource. A posture contains user curated policy sets. A posture can
* be deployed on a project or on a folder or on an organization. To deploy a posture we need to populate the posture's name
* and its revision_id in the posture deployment configuration. Every update to a deployed posture generates a new revision_id.
* Thus, the updated revision_id should be used in the respective posture deployment's configuration to deploy that posture
* on a resource.
* To get more information about PostureDeployment, see:
* * How-to Guides
* * [Create and deploy a posture](https://cloud.google.com/security-command-center/docs/how-to-use-security-posture)
* ## Example Usage
* ### Securityposture Posture Deployment Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const posture1 = new gcp.securityposture.Posture("posture_1", {
* postureId: "posture_1",
* parent: "organizations/123456789",
* location: "global",
* state: "ACTIVE",
* description: "a new posture",
* policySets: [{
* policySetId: "org_policy_set",
* description: "set of org policies",
* policies: [{
* policyId: "policy_1",
* constraint: {
* orgPolicyConstraint: {
* cannedConstraintId: "storage.uniformBucketLevelAccess",
* policyRules: [{
* enforce: true,
* }],
* },
* },
* }],
* }],
* });
* const postureDeployment = new gcp.securityposture.PostureDeployment("postureDeployment", {
* postureDeploymentId: "posture_deployment_1",
* parent: "organizations/123456789",
* location: "global",
* description: "a new posture deployment",
* targetResource: "projects/1111111111111",
* postureId: posture1.name,
* postureRevisionId: posture1.revisionId,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* posture1 = gcp.securityposture.Posture("posture_1",
* posture_id="posture_1",
* parent="organizations/123456789",
* location="global",
* state="ACTIVE",
* description="a new posture",
* policy_sets=[gcp.securityposture.PosturePolicySetArgs(
* policy_set_id="org_policy_set",
* description="set of org policies",
* policies=[gcp.securityposture.PosturePolicySetPolicyArgs(
* policy_id="policy_1",
* constraint=gcp.securityposture.PosturePolicySetPolicyConstraintArgs(
* org_policy_constraint=gcp.securityposture.PosturePolicySetPolicyConstraintOrgPolicyConstraintArgs(
* canned_constraint_id="storage.uniformBucketLevelAccess",
* policy_rules=[gcp.securityposture.PosturePolicySetPolicyConstraintOrgPolicyConstraintPolicyRuleArgs(
* enforce=True,
* )],
* ),
* ),
* )],
* )])
* posture_deployment = gcp.securityposture.PostureDeployment("postureDeployment",
* posture_deployment_id="posture_deployment_1",
* parent="organizations/123456789",
* location="global",
* description="a new posture deployment",
* target_resource="projects/1111111111111",
* posture_id=posture1.name,
* posture_revision_id=posture1.revision_id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var posture1 = new Gcp.SecurityPosture.Posture("posture_1", new()
* {
* PostureId = "posture_1",
* Parent = "organizations/123456789",
* Location = "global",
* State = "ACTIVE",
* Description = "a new posture",
* PolicySets = new[]
* {
* new Gcp.SecurityPosture.Inputs.PosturePolicySetArgs
* {
* PolicySetId = "org_policy_set",
* Description = "set of org policies",
* Policies = new[]
* {
* new Gcp.SecurityPosture.Inputs.PosturePolicySetPolicyArgs
* {
* PolicyId = "policy_1",
* Constraint = new Gcp.SecurityPosture.Inputs.PosturePolicySetPolicyConstraintArgs
* {
* OrgPolicyConstraint = new Gcp.SecurityPosture.Inputs.PosturePolicySetPolicyConstraintOrgPolicyConstraintArgs
* {
* CannedConstraintId = "storage.uniformBucketLevelAccess",
* PolicyRules = new[]
* {
* new Gcp.SecurityPosture.Inputs.PosturePolicySetPolicyConstraintOrgPolicyConstraintPolicyRuleArgs
* {
* Enforce = true,
* },
* },
* },
* },
* },
* },
* },
* },
* });
* var postureDeployment = new Gcp.SecurityPosture.PostureDeployment("postureDeployment", new()
* {
* PostureDeploymentId = "posture_deployment_1",
* Parent = "organizations/123456789",
* Location = "global",
* Description = "a new posture deployment",
* TargetResource = "projects/1111111111111",
* PostureId = posture1.Name,
* PostureRevisionId = posture1.RevisionId,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/securityposture"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* posture1, err := securityposture.NewPosture(ctx, "posture_1", &securityposture.PostureArgs{
* PostureId: pulumi.String("posture_1"),
* Parent: pulumi.String("organizations/123456789"),
* Location: pulumi.String("global"),
* State: pulumi.String("ACTIVE"),
* Description: pulumi.String("a new posture"),
* PolicySets: securityposture.PosturePolicySetArray{
* &securityposture.PosturePolicySetArgs{
* PolicySetId: pulumi.String("org_policy_set"),
* Description: pulumi.String("set of org policies"),
* Policies: securityposture.PosturePolicySetPolicyArray{
* &securityposture.PosturePolicySetPolicyArgs{
* PolicyId: pulumi.String("policy_1"),
* Constraint: &securityposture.PosturePolicySetPolicyConstraintArgs{
* OrgPolicyConstraint: &securityposture.PosturePolicySetPolicyConstraintOrgPolicyConstraintArgs{
* CannedConstraintId: pulumi.String("storage.uniformBucketLevelAccess"),
* PolicyRules: securityposture.PosturePolicySetPolicyConstraintOrgPolicyConstraintPolicyRuleArray{
* &securityposture.PosturePolicySetPolicyConstraintOrgPolicyConstraintPolicyRuleArgs{
* Enforce: pulumi.Bool(true),
* },
* },
* },
* },
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = securityposture.NewPostureDeployment(ctx, "postureDeployment", &securityposture.PostureDeploymentArgs{
* PostureDeploymentId: pulumi.String("posture_deployment_1"),
* Parent: pulumi.String("organizations/123456789"),
* Location: pulumi.String("global"),
* Description: pulumi.String("a new posture deployment"),
* TargetResource: pulumi.String("projects/1111111111111"),
* PostureId: posture1.Name,
* PostureRevisionId: posture1.RevisionId,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.securityposture.Posture;
* import com.pulumi.gcp.securityposture.PostureArgs;
* import com.pulumi.gcp.securityposture.inputs.PosturePolicySetArgs;
* import com.pulumi.gcp.securityposture.PostureDeployment;
* import com.pulumi.gcp.securityposture.PostureDeploymentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var posture1 = new Posture("posture1", PostureArgs.builder()
* .postureId("posture_1")
* .parent("organizations/123456789")
* .location("global")
* .state("ACTIVE")
* .description("a new posture")
* .policySets(PosturePolicySetArgs.builder()
* .policySetId("org_policy_set")
* .description("set of org policies")
* .policies(PosturePolicySetPolicyArgs.builder()
* .policyId("policy_1")
* .constraint(PosturePolicySetPolicyConstraintArgs.builder()
* .orgPolicyConstraint(PosturePolicySetPolicyConstraintOrgPolicyConstraintArgs.builder()
* .cannedConstraintId("storage.uniformBucketLevelAccess")
* .policyRules(PosturePolicySetPolicyConstraintOrgPolicyConstraintPolicyRuleArgs.builder()
* .enforce(true)
* .build())
* .build())
* .build())
* .build())
* .build())
* .build());
* var postureDeployment = new PostureDeployment("postureDeployment", PostureDeploymentArgs.builder()
* .postureDeploymentId("posture_deployment_1")
* .parent("organizations/123456789")
* .location("global")
* .description("a new posture deployment")
* .targetResource("projects/1111111111111")
* .postureId(posture1.name())
* .postureRevisionId(posture1.revisionId())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* posture1:
* type: gcp:securityposture:Posture
* name: posture_1
* properties:
* postureId: posture_1
* parent: organizations/123456789
* location: global
* state: ACTIVE
* description: a new posture
* policySets:
* - policySetId: org_policy_set
* description: set of org policies
* policies:
* - policyId: policy_1
* constraint:
* orgPolicyConstraint:
* cannedConstraintId: storage.uniformBucketLevelAccess
* policyRules:
* - enforce: true
* postureDeployment:
* type: gcp:securityposture:PostureDeployment
* properties:
* postureDeploymentId: posture_deployment_1
* parent: organizations/123456789
* location: global
* description: a new posture deployment
* targetResource: projects/1111111111111
* postureId: ${posture1.name}
* postureRevisionId: ${posture1.revisionId}
* ```
*
* ## Import
* PostureDeployment can be imported using any of these accepted formats:
* * `{{parent}}/locations/{{location}}/postureDeployments/{{posture_deployment_id}}`
* When using the `pulumi import` command, PostureDeployment can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:securityposture/postureDeployment:PostureDeployment default {{parent}}/locations/{{location}}/postureDeployments/{{posture_deployment_id}}
* ```
* @property description Description of the posture deployment.
* @property location The location of the resource, eg. global`.
* @property parent The parent of the resource, an organization. Format should be `organizations/{organization_id}`.
* @property postureDeploymentId ID of the posture deployment.
* - - -
* @property postureId Relative name of the posture which needs to be deployed. It should be in the format:
* organizations/{organization_id}/locations/{location}/postures/{posture_id}
* @property postureRevisionId Revision_id the posture which needs to be deployed.
* @property targetResource The resource on which the posture should be deployed. This can be in one of the following formats:
* projects/{project_number},
* folders/{folder_number},
* organizations/{organization_id}
*/
public data class PostureDeploymentArgs(
public val description: Output? = null,
public val location: Output? = null,
public val parent: Output? = null,
public val postureDeploymentId: Output? = null,
public val postureId: Output? = null,
public val postureRevisionId: Output? = null,
public val targetResource: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.securityposture.PostureDeploymentArgs =
com.pulumi.gcp.securityposture.PostureDeploymentArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.parent(parent?.applyValue({ args0 -> args0 }))
.postureDeploymentId(postureDeploymentId?.applyValue({ args0 -> args0 }))
.postureId(postureId?.applyValue({ args0 -> args0 }))
.postureRevisionId(postureRevisionId?.applyValue({ args0 -> args0 }))
.targetResource(targetResource?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PostureDeploymentArgs].
*/
@PulumiTagMarker
public class PostureDeploymentArgsBuilder internal constructor() {
private var description: Output? = null
private var location: Output? = null
private var parent: Output? = null
private var postureDeploymentId: Output? = null
private var postureId: Output? = null
private var postureRevisionId: Output? = null
private var targetResource: Output? = null
/**
* @param value Description of the posture deployment.
*/
@JvmName("hhwrqxmetnmaiisf")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The location of the resource, eg. global`.
*/
@JvmName("gkocovwhtlagvwfm")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The parent of the resource, an organization. Format should be `organizations/{organization_id}`.
*/
@JvmName("hscqydhvmblhsmoe")
public suspend fun parent(`value`: Output) {
this.parent = value
}
/**
* @param value ID of the posture deployment.
* - - -
*/
@JvmName("ubtukcjmboaydtqg")
public suspend fun postureDeploymentId(`value`: Output) {
this.postureDeploymentId = value
}
/**
* @param value Relative name of the posture which needs to be deployed. It should be in the format:
* organizations/{organization_id}/locations/{location}/postures/{posture_id}
*/
@JvmName("bctgioygnabpugmj")
public suspend fun postureId(`value`: Output) {
this.postureId = value
}
/**
* @param value Revision_id the posture which needs to be deployed.
*/
@JvmName("pubmqvludyfnelec")
public suspend fun postureRevisionId(`value`: Output) {
this.postureRevisionId = value
}
/**
* @param value The resource on which the posture should be deployed. This can be in one of the following formats:
* projects/{project_number},
* folders/{folder_number},
* organizations/{organization_id}
*/
@JvmName("towosicungqtufvh")
public suspend fun targetResource(`value`: Output) {
this.targetResource = value
}
/**
* @param value Description of the posture deployment.
*/
@JvmName("cfxmrxonlsmesxdx")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The location of the resource, eg. global`.
*/
@JvmName("bigvyvovfyxloxxr")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value The parent of the resource, an organization. Format should be `organizations/{organization_id}`.
*/
@JvmName("pbkctyaukmakoncb")
public suspend fun parent(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.parent = mapped
}
/**
* @param value ID of the posture deployment.
* - - -
*/
@JvmName("uhvimbpyytgwqiol")
public suspend fun postureDeploymentId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postureDeploymentId = mapped
}
/**
* @param value Relative name of the posture which needs to be deployed. It should be in the format:
* organizations/{organization_id}/locations/{location}/postures/{posture_id}
*/
@JvmName("mepewyeifvnwabyt")
public suspend fun postureId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postureId = mapped
}
/**
* @param value Revision_id the posture which needs to be deployed.
*/
@JvmName("vpikoxdtudpvyems")
public suspend fun postureRevisionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postureRevisionId = mapped
}
/**
* @param value The resource on which the posture should be deployed. This can be in one of the following formats:
* projects/{project_number},
* folders/{folder_number},
* organizations/{organization_id}
*/
@JvmName("ecclweybyhqpntrl")
public suspend fun targetResource(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetResource = mapped
}
internal fun build(): PostureDeploymentArgs = PostureDeploymentArgs(
description = description,
location = location,
parent = parent,
postureDeploymentId = postureDeploymentId,
postureId = postureId,
postureRevisionId = postureRevisionId,
targetResource = targetResource,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy