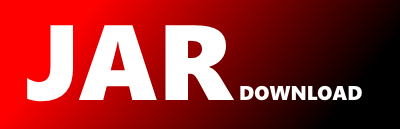
com.pulumi.gcp.serviceaccount.kotlin.Account.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.serviceaccount.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [Account].
*/
@PulumiTagMarker
public class AccountResourceBuilder internal constructor() {
public var name: String? = null
public var args: AccountArgs = AccountArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AccountArgsBuilder.() -> Unit) {
val builder = AccountArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Account {
val builtJavaResource = com.pulumi.gcp.serviceaccount.Account(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Account(builtJavaResource)
}
}
/**
* Allows management of a Google Cloud service account.
* * [API documentation](https://cloud.google.com/iam/reference/rest/v1/projects.serviceAccounts)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/compute/docs/access/service-accounts)
* > **Warning:** If you delete and recreate a service account, you must reapply any IAM roles that it had before.
* > Creation of service accounts is eventually consistent, and that can lead to
* errors when you try to apply ACLs to service accounts immediately after
* creation.
* ## Example Usage
* This snippet creates a service account in a project.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const serviceAccount = new gcp.serviceaccount.Account("service_account", {
* accountId: "service-account-id",
* displayName: "Service Account",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* service_account = gcp.serviceaccount.Account("service_account",
* account_id="service-account-id",
* display_name="Service Account")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var serviceAccount = new Gcp.ServiceAccount.Account("service_account", new()
* {
* AccountId = "service-account-id",
* DisplayName = "Service Account",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := serviceaccount.NewAccount(ctx, "service_account", &serviceaccount.AccountArgs{
* AccountId: pulumi.String("service-account-id"),
* DisplayName: pulumi.String("Service Account"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.Account;
* import com.pulumi.gcp.serviceaccount.AccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var serviceAccount = new Account("serviceAccount", AccountArgs.builder()
* .accountId("service-account-id")
* .displayName("Service Account")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* serviceAccount:
* type: gcp:serviceaccount:Account
* name: service_account
* properties:
* accountId: service-account-id
* displayName: Service Account
* ```
*
* ## Import
* Service accounts can be imported using their URI, e.g.
* * `projects/{{project_id}}/serviceAccounts/{{email}}`
* When using the `pulumi import` command, service accounts can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:serviceaccount/account:Account default projects/{{project_id}}/serviceAccounts/{{email}}
* ```
*/
public class Account internal constructor(
override val javaResource: com.pulumi.gcp.serviceaccount.Account,
) : KotlinCustomResource(javaResource, AccountMapper) {
/**
* The account id that is used to generate the service
* account email address and a stable unique id. It is unique within a project,
* must be 6-30 characters long, and match the regular expression `a-z`
* to comply with RFC1035. Changing this forces a new service account to be created.
*/
public val accountId: Output
get() = javaResource.accountId().applyValue({ args0 -> args0 })
/**
* If set to true, skip service account creation if a service account with the same email already exists.
*/
public val createIgnoreAlreadyExists: Output?
get() = javaResource.createIgnoreAlreadyExists().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A text description of the service account.
* Must be less than or equal to 256 UTF-8 bytes.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether a service account is disabled or not. Defaults to `false`. This field has no effect during creation.
* Must be set after creation to disable a service account.
*/
public val disabled: Output?
get() = javaResource.disabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The display name for the service account.
* Can be updated without creating a new resource.
*/
public val displayName: Output?
get() = javaResource.displayName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The e-mail address of the service account. This value
* should be referenced from any `gcp.organizations.getIAMPolicy` data sources
* that would grant the service account privileges.
*/
public val email: Output
get() = javaResource.email().applyValue({ args0 -> args0 })
/**
* The Identity of the service account in the form `serviceAccount:{email}`. This value is often used to refer to the service account in order to grant IAM permissions.
*/
public val member: Output
get() = javaResource.member().applyValue({ args0 -> args0 })
/**
* The fully-qualified name of the service account.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The ID of the project that the service account will be created in.
* Defaults to the provider project configuration.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The unique id of the service account.
*/
public val uniqueId: Output
get() = javaResource.uniqueId().applyValue({ args0 -> args0 })
}
public object AccountMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.serviceaccount.Account::class == javaResource::class
override fun map(javaResource: Resource): Account = Account(
javaResource as
com.pulumi.gcp.serviceaccount.Account,
)
}
/**
* @see [Account].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Account].
*/
public suspend fun account(name: String, block: suspend AccountResourceBuilder.() -> Unit): Account {
val builder = AccountResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Account].
* @param name The _unique_ name of the resulting resource.
*/
public fun account(name: String): Account {
val builder = AccountResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy