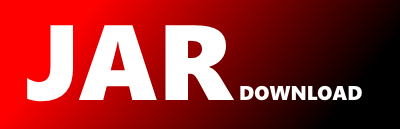
com.pulumi.gcp.serviceaccount.kotlin.ServiceaccountFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.serviceaccount.kotlin
import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions.getAccountAccessTokenPlain
import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions.getAccountIdTokenPlain
import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions.getAccountJwtPlain
import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions.getAccountKeyPlain
import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions.getAccountPlain
import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions.getIamPolicyPlain
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountAccessTokenPlainArgs
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountAccessTokenPlainArgsBuilder
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountIdTokenPlainArgs
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountIdTokenPlainArgsBuilder
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountJwtPlainArgs
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountJwtPlainArgsBuilder
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountKeyPlainArgs
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountKeyPlainArgsBuilder
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountPlainArgs
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountPlainArgsBuilder
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetIamPolicyPlainArgs
import com.pulumi.gcp.serviceaccount.kotlin.inputs.GetIamPolicyPlainArgsBuilder
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountAccessTokenResult
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountIdTokenResult
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountJwtResult
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountKeyResult
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountResult
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetIamPolicyResult
import kotlinx.coroutines.future.await
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountAccessTokenResult.Companion.toKotlin as getAccountAccessTokenResultToKotlin
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountIdTokenResult.Companion.toKotlin as getAccountIdTokenResultToKotlin
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountJwtResult.Companion.toKotlin as getAccountJwtResultToKotlin
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountKeyResult.Companion.toKotlin as getAccountKeyResultToKotlin
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetAccountResult.Companion.toKotlin as getAccountResultToKotlin
import com.pulumi.gcp.serviceaccount.kotlin.outputs.GetIamPolicyResult.Companion.toKotlin as getIamPolicyResultToKotlin
public object ServiceaccountFunctions {
/**
* Get the service account from a project. For more information see
* the official [API](https://cloud.google.com/compute/docs/access/service-accounts) documentation.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const objectViewer = gcp.serviceaccount.getAccount({
* accountId: "object-viewer",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* object_viewer = gcp.serviceaccount.get_account(account_id="object-viewer")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var objectViewer = Gcp.ServiceAccount.GetAccount.Invoke(new()
* {
* AccountId = "object-viewer",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := serviceaccount.LookupAccount(ctx, &serviceaccount.LookupAccountArgs{
* AccountId: "object-viewer",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions;
* import com.pulumi.gcp.serviceaccount.inputs.GetAccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var objectViewer = ServiceaccountFunctions.getAccount(GetAccountArgs.builder()
* .accountId("object-viewer")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* objectViewer:
* fn::invoke:
* Function: gcp:serviceaccount:getAccount
* Arguments:
* accountId: object-viewer
* ```
*
* ### Save Key In Kubernetes Secret
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* import * as kubernetes from "@pulumi/kubernetes";
* import * as std from "@pulumi/std";
* const myaccount = gcp.serviceaccount.getAccount({
* accountId: "myaccount-id",
* });
* const mykey = new gcp.serviceaccount.Key("mykey", {serviceAccountId: myaccount.then(myaccount => myaccount.name)});
* const google_application_credentials = new kubernetes.core.v1.Secret("google-application-credentials", {
* metadata: {
* name: "google-application-credentials",
* },
* data: {
* json: std.base64decodeOutput({
* input: mykey.privateKey,
* }).apply(invoke => invoke.result),
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* import pulumi_kubernetes as kubernetes
* import pulumi_std as std
* myaccount = gcp.serviceaccount.get_account(account_id="myaccount-id")
* mykey = gcp.serviceaccount.Key("mykey", service_account_id=myaccount.name)
* google_application_credentials = kubernetes.core.v1.Secret("google-application-credentials",
* metadata=kubernetes.meta.v1.ObjectMetaArgs(
* name="google-application-credentials",
* ),
* data={
* "json": std.base64decode_output(input=mykey.private_key).apply(lambda invoke: invoke.result),
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* using Kubernetes = Pulumi.Kubernetes;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var myaccount = Gcp.ServiceAccount.GetAccount.Invoke(new()
* {
* AccountId = "myaccount-id",
* });
* var mykey = new Gcp.ServiceAccount.Key("mykey", new()
* {
* ServiceAccountId = myaccount.Apply(getAccountResult => getAccountResult.Name),
* });
* var google_application_credentials = new Kubernetes.Core.V1.Secret("google-application-credentials", new()
* {
* Metadata = new Kubernetes.Types.Inputs.Meta.V1.ObjectMetaArgs
* {
* Name = "google-application-credentials",
* },
* Data =
* {
* { "json", Std.Base64decode.Invoke(new()
* {
* Input = mykey.PrivateKey,
* }).Apply(invoke => invoke.Result) },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* corev1 "github.com/pulumi/pulumi-kubernetes/sdk/v4/go/kubernetes/core/v1"
* metav1 "github.com/pulumi/pulumi-kubernetes/sdk/v4/go/kubernetes/meta/v1"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* myaccount, err := serviceaccount.LookupAccount(ctx, &serviceaccount.LookupAccountArgs{
* AccountId: "myaccount-id",
* }, nil)
* if err != nil {
* return err
* }
* mykey, err := serviceaccount.NewKey(ctx, "mykey", &serviceaccount.KeyArgs{
* ServiceAccountId: pulumi.String(myaccount.Name),
* })
* if err != nil {
* return err
* }
* _, err = corev1.NewSecret(ctx, "google-application-credentials", &corev1.SecretArgs{
* Metadata: &metav1.ObjectMetaArgs{
* Name: pulumi.String("google-application-credentials"),
* },
* Data: pulumi.StringMap{
* "json": std.Base64decodeOutput(ctx, std.Base64decodeOutputArgs{
* Input: mykey.PrivateKey,
* }, nil).ApplyT(func(invoke std.Base64decodeResult) (*string, error) {
* return invoke.Result, nil
* }).(pulumi.StringPtrOutput),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions;
* import com.pulumi.gcp.serviceaccount.inputs.GetAccountArgs;
* import com.pulumi.gcp.serviceaccount.Key;
* import com.pulumi.gcp.serviceaccount.KeyArgs;
* import com.pulumi.kubernetes.core_v1.Secret;
* import com.pulumi.kubernetes.core_v1.SecretArgs;
* import com.pulumi.kubernetes.meta_v1.inputs.ObjectMetaArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var myaccount = ServiceaccountFunctions.getAccount(GetAccountArgs.builder()
* .accountId("myaccount-id")
* .build());
* var mykey = new Key("mykey", KeyArgs.builder()
* .serviceAccountId(myaccount.applyValue(getAccountResult -> getAccountResult.name()))
* .build());
* var google_application_credentials = new Secret("google-application-credentials", SecretArgs.builder()
* .metadata(ObjectMetaArgs.builder()
* .name("google-application-credentials")
* .build())
* .data(Map.of("json", StdFunctions.base64decode().applyValue(invoke -> invoke.result())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* mykey:
* type: gcp:serviceaccount:Key
* properties:
* serviceAccountId: ${myaccount.name}
* google-application-credentials:
* type: kubernetes:core/v1:Secret
* properties:
* metadata:
* name: google-application-credentials
* data:
* json:
* fn::invoke:
* Function: std:base64decode
* Arguments:
* input: ${mykey.privateKey}
* Return: result
* variables:
* myaccount:
* fn::invoke:
* Function: gcp:serviceaccount:getAccount
* Arguments:
* accountId: myaccount-id
* ```
*
* @param argument A collection of arguments for invoking getAccount.
* @return A collection of values returned by getAccount.
*/
public suspend fun getAccount(argument: GetAccountPlainArgs): GetAccountResult =
getAccountResultToKotlin(getAccountPlain(argument.toJava()).await())
/**
* @see [getAccount].
* @param accountId The Google service account ID. This be one of:
* * The name of the service account within the project (e.g. `my-service`)
* * The fully-qualified path to a service account resource (e.g.
* `projects/my-project/serviceAccounts/...`)
* * The email address of the service account (e.g.
* `[email protected]`)
* @param project The ID of the project that the service account is present in.
* Defaults to the provider project configuration.
* @return A collection of values returned by getAccount.
*/
public suspend fun getAccount(accountId: String, project: String? = null): GetAccountResult {
val argument = GetAccountPlainArgs(
accountId = accountId,
project = project,
)
return getAccountResultToKotlin(getAccountPlain(argument.toJava()).await())
}
/**
* @see [getAccount].
* @param argument Builder for [com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountPlainArgs].
* @return A collection of values returned by getAccount.
*/
public suspend fun getAccount(argument: suspend GetAccountPlainArgsBuilder.() -> Unit): GetAccountResult {
val builder = GetAccountPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccountResultToKotlin(getAccountPlain(builtArgument.toJava()).await())
}
/**
* This data source provides a google `oauth2` `access_token` for a different service account than the one initially running the script.
* For more information see
* [the official documentation](https://cloud.google.com/iam/docs/creating-short-lived-service-account-credentials) as well as [iamcredentials.generateAccessToken()](https://cloud.google.com/iam/credentials/reference/rest/v1/projects.serviceAccounts/generateAccessToken)
* ## Example Usage
* To allow `service_A` to impersonate `service_B`, grant the [Service Account Token Creator](https://cloud.google.com/iam/docs/service-accounts#the_service_account_token_creator_role) on B to A.
* In the IAM policy below, `service_A` is given the Token Creator role impersonate `service_B`
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const token_creator_iam = new gcp.serviceaccount.IAMBinding("token-creator-iam", {
* serviceAccountId: "projects/-/serviceAccounts/[email protected]",
* role: "roles/iam.serviceAccountTokenCreator",
* members: ["serviceAccount:service_A@projectA.iam.gserviceaccount.com"],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* token_creator_iam = gcp.serviceaccount.IAMBinding("token-creator-iam",
* service_account_id="projects/-/serviceAccounts/[email protected]",
* role="roles/iam.serviceAccountTokenCreator",
* members=["serviceAccount:service_A@projectA.iam.gserviceaccount.com"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var token_creator_iam = new Gcp.ServiceAccount.IAMBinding("token-creator-iam", new()
* {
* ServiceAccountId = "projects/-/serviceAccounts/[email protected]",
* Role = "roles/iam.serviceAccountTokenCreator",
* Members = new[]
* {
* "serviceAccount:[email protected]",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := serviceaccount.NewIAMBinding(ctx, "token-creator-iam", &serviceaccount.IAMBindingArgs{
* ServiceAccountId: pulumi.String("projects/-/serviceAccounts/[email protected]"),
* Role: pulumi.String("roles/iam.serviceAccountTokenCreator"),
* Members: pulumi.StringArray{
* pulumi.String("serviceAccount:[email protected]"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.IAMBinding;
* import com.pulumi.gcp.serviceaccount.IAMBindingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var token_creator_iam = new IAMBinding("token-creator-iam", IAMBindingArgs.builder()
* .serviceAccountId("projects/-/serviceAccounts/[email protected]")
* .role("roles/iam.serviceAccountTokenCreator")
* .members("serviceAccount:[email protected]")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* token-creator-iam:
* type: gcp:serviceaccount:IAMBinding
* properties:
* serviceAccountId: projects/-/serviceAccounts/[email protected]
* role: roles/iam.serviceAccountTokenCreator
* members:
* - serviceAccount:[email protected]
* ```
*
* Once the IAM permissions are set, you can apply the new token to a provider bootstrapped with it. Any resources that references the aliased provider will run as the new identity.
* In the example below, `gcp.organizations.Project` will run as `service_B`.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* export = async () => {
* const default = await gcp.organizations.getClientConfig({});
* const defaultGetAccountAccessToken = await gcp.serviceaccount.getAccountAccessToken({
* targetServiceAccount: "[email protected]",
* scopes: [
* "userinfo-email",
* "cloud-platform",
* ],
* lifetime: "300s",
* });
* const me = await gcp.organizations.getClientOpenIdUserInfo({});
* return {
* "target-email": me.email,
* };
* }
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.organizations.get_client_config()
* default_get_account_access_token = gcp.serviceaccount.get_account_access_token(target_service_account="[email protected]",
* scopes=[
* "userinfo-email",
* "cloud-platform",
* ],
* lifetime="300s")
* me = gcp.organizations.get_client_open_id_user_info()
* pulumi.export("target-email", me.email)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = Gcp.Organizations.GetClientConfig.Invoke();
* var defaultGetAccountAccessToken = Gcp.ServiceAccount.GetAccountAccessToken.Invoke(new()
* {
* TargetServiceAccount = "[email protected]",
* Scopes = new[]
* {
* "userinfo-email",
* "cloud-platform",
* },
* Lifetime = "300s",
* });
* var me = Gcp.Organizations.GetClientOpenIdUserInfo.Invoke();
* return new Dictionary
* {
* ["target-email"] = me.Apply(getClientOpenIdUserInfoResult => getClientOpenIdUserInfoResult.Email),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := organizations.GetClientConfig(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = serviceaccount.GetAccountAccessToken(ctx, &serviceaccount.GetAccountAccessTokenArgs{
* TargetServiceAccount: "[email protected]",
* Scopes: []string{
* "userinfo-email",
* "cloud-platform",
* },
* Lifetime: pulumi.StringRef("300s"),
* }, nil)
* if err != nil {
* return err
* }
* me, err := organizations.GetClientOpenIdUserInfo(ctx, nil, nil)
* if err != nil {
* return err
* }
* ctx.Export("target-email", me.Email)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions;
* import com.pulumi.gcp.serviceaccount.inputs.GetAccountAccessTokenArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var default = OrganizationsFunctions.getClientConfig();
* final var defaultGetAccountAccessToken = ServiceaccountFunctions.getAccountAccessToken(GetAccountAccessTokenArgs.builder()
* .targetServiceAccount("[email protected]")
* .scopes(
* "userinfo-email",
* "cloud-platform")
* .lifetime("300s")
* .build());
* final var me = OrganizationsFunctions.getClientOpenIdUserInfo();
* ctx.export("target-email", me.applyValue(getClientOpenIdUserInfoResult -> getClientOpenIdUserInfoResult.email()));
* }
* }
* ```
* ```yaml
* variables:
* default:
* fn::invoke:
* Function: gcp:organizations:getClientConfig
* Arguments: {}
* defaultGetAccountAccessToken:
* fn::invoke:
* Function: gcp:serviceaccount:getAccountAccessToken
* Arguments:
* targetServiceAccount: [email protected]
* scopes:
* - userinfo-email
* - cloud-platform
* lifetime: 300s
* me:
* fn::invoke:
* Function: gcp:organizations:getClientOpenIdUserInfo
* Arguments: {}
* outputs:
* target-email: ${me.email}
* ```
*
* > *Note*: the generated token is non-refreshable and can have a maximum `lifetime` of `3600` seconds.
* @param argument A collection of arguments for invoking getAccountAccessToken.
* @return A collection of values returned by getAccountAccessToken.
*/
public suspend fun getAccountAccessToken(argument: GetAccountAccessTokenPlainArgs): GetAccountAccessTokenResult =
getAccountAccessTokenResultToKotlin(getAccountAccessTokenPlain(argument.toJava()).await())
/**
* @see [getAccountAccessToken].
* @param delegates Delegate chain of approvals needed to perform full impersonation. Specify the fully qualified service account name. (e.g. `["projects/-/serviceAccounts/delegate-svc-account@project-id.iam.gserviceaccount.com"]`)
* @param lifetime Lifetime of the impersonated token (defaults to its max: `3600s`).
* @param scopes The scopes the new credential should have (e.g. `["cloud-platform"]`)
* @param targetServiceAccount The service account _to_ impersonate (e.g. `[email protected]`)
* @return A collection of values returned by getAccountAccessToken.
*/
public suspend fun getAccountAccessToken(
delegates: List? = null,
lifetime: String? = null,
scopes: List,
targetServiceAccount: String,
): GetAccountAccessTokenResult {
val argument = GetAccountAccessTokenPlainArgs(
delegates = delegates,
lifetime = lifetime,
scopes = scopes,
targetServiceAccount = targetServiceAccount,
)
return getAccountAccessTokenResultToKotlin(getAccountAccessTokenPlain(argument.toJava()).await())
}
/**
* @see [getAccountAccessToken].
* @param argument Builder for [com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountAccessTokenPlainArgs].
* @return A collection of values returned by getAccountAccessToken.
*/
public suspend fun getAccountAccessToken(argument: suspend GetAccountAccessTokenPlainArgsBuilder.() -> Unit): GetAccountAccessTokenResult {
val builder = GetAccountAccessTokenPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccountAccessTokenResultToKotlin(getAccountAccessTokenPlain(builtArgument.toJava()).await())
}
/**
* This data source provides a Google OpenID Connect (`oidc`) `id_token`. Tokens issued from this data source are typically used to call external services that accept OIDC tokens for authentication (e.g. [Google Cloud Run](https://cloud.google.com/run/docs/authenticating/service-to-service)).
* For more information see
* [OpenID Connect](https://openid.net/specs/openid-connect-core-1_0.html#IDToken).
* ## Example Usage
* ### ServiceAccount JSON Credential File.
* `gcp.serviceaccount.getAccountIdToken` will use the configured provider credentials
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const oidc = gcp.serviceaccount.getAccountIdToken({
* targetAudience: "https://foo.bar/",
* });
* export const oidcToken = oidc.then(oidc => oidc.idToken);
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* oidc = gcp.serviceaccount.get_account_id_token(target_audience="https://foo.bar/")
* pulumi.export("oidcToken", oidc.id_token)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var oidc = Gcp.ServiceAccount.GetAccountIdToken.Invoke(new()
* {
* TargetAudience = "https://foo.bar/",
* });
* return new Dictionary
* {
* ["oidcToken"] = oidc.Apply(getAccountIdTokenResult => getAccountIdTokenResult.IdToken),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* oidc, err := serviceaccount.GetAccountIdToken(ctx, &serviceaccount.GetAccountIdTokenArgs{
* TargetAudience: "https://foo.bar/",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("oidcToken", oidc.IdToken)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions;
* import com.pulumi.gcp.serviceaccount.inputs.GetAccountIdTokenArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var oidc = ServiceaccountFunctions.getAccountIdToken(GetAccountIdTokenArgs.builder()
* .targetAudience("https://foo.bar/")
* .build());
* ctx.export("oidcToken", oidc.applyValue(getAccountIdTokenResult -> getAccountIdTokenResult.idToken()));
* }
* }
* ```
* ```yaml
* variables:
* oidc:
* fn::invoke:
* Function: gcp:serviceaccount:getAccountIdToken
* Arguments:
* targetAudience: https://foo.bar/
* outputs:
* oidcToken: ${oidc.idToken}
* ```
*
* ### Service Account Impersonation.
* `gcp.serviceaccount.getAccountAccessToken` will use background impersonated credentials provided by `gcp.serviceaccount.getAccountAccessToken`.
* Note: to use the following, you must grant `target_service_account` the
* `roles/iam.serviceAccountTokenCreator` role on itself.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const impersonated = gcp.serviceaccount.getAccountAccessToken({
* targetServiceAccount: "[email protected]",
* delegates: [],
* scopes: [
* "userinfo-email",
* "cloud-platform",
* ],
* lifetime: "300s",
* });
* const oidc = gcp.serviceaccount.getAccountIdToken({
* targetServiceAccount: "[email protected]",
* delegates: [],
* includeEmail: true,
* targetAudience: "https://foo.bar/",
* });
* export const oidcToken = oidc.then(oidc => oidc.idToken);
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* impersonated = gcp.serviceaccount.get_account_access_token(target_service_account="[email protected]",
* delegates=[],
* scopes=[
* "userinfo-email",
* "cloud-platform",
* ],
* lifetime="300s")
* oidc = gcp.serviceaccount.get_account_id_token(target_service_account="[email protected]",
* delegates=[],
* include_email=True,
* target_audience="https://foo.bar/")
* pulumi.export("oidcToken", oidc.id_token)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var impersonated = Gcp.ServiceAccount.GetAccountAccessToken.Invoke(new()
* {
* TargetServiceAccount = "[email protected]",
* Delegates = new() { },
* Scopes = new[]
* {
* "userinfo-email",
* "cloud-platform",
* },
* Lifetime = "300s",
* });
* var oidc = Gcp.ServiceAccount.GetAccountIdToken.Invoke(new()
* {
* TargetServiceAccount = "[email protected]",
* Delegates = new() { },
* IncludeEmail = true,
* TargetAudience = "https://foo.bar/",
* });
* return new Dictionary
* {
* ["oidcToken"] = oidc.Apply(getAccountIdTokenResult => getAccountIdTokenResult.IdToken),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := serviceaccount.GetAccountAccessToken(ctx, &serviceaccount.GetAccountAccessTokenArgs{
* TargetServiceAccount: "[email protected]",
* Delegates: []interface{}{},
* Scopes: []string{
* "userinfo-email",
* "cloud-platform",
* },
* Lifetime: pulumi.StringRef("300s"),
* }, nil)
* if err != nil {
* return err
* }
* oidc, err := serviceaccount.GetAccountIdToken(ctx, &serviceaccount.GetAccountIdTokenArgs{
* TargetServiceAccount: pulumi.StringRef("[email protected]"),
* Delegates: []interface{}{},
* IncludeEmail: pulumi.BoolRef(true),
* TargetAudience: "https://foo.bar/",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("oidcToken", oidc.IdToken)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions;
* import com.pulumi.gcp.serviceaccount.inputs.GetAccountAccessTokenArgs;
* import com.pulumi.gcp.serviceaccount.inputs.GetAccountIdTokenArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var impersonated = ServiceaccountFunctions.getAccountAccessToken(GetAccountAccessTokenArgs.builder()
* .targetServiceAccount("[email protected]")
* .delegates()
* .scopes(
* "userinfo-email",
* "cloud-platform")
* .lifetime("300s")
* .build());
* final var oidc = ServiceaccountFunctions.getAccountIdToken(GetAccountIdTokenArgs.builder()
* .targetServiceAccount("[email protected]")
* .delegates()
* .includeEmail(true)
* .targetAudience("https://foo.bar/")
* .build());
* ctx.export("oidcToken", oidc.applyValue(getAccountIdTokenResult -> getAccountIdTokenResult.idToken()));
* }
* }
* ```
* ```yaml
* variables:
* impersonated:
* fn::invoke:
* Function: gcp:serviceaccount:getAccountAccessToken
* Arguments:
* targetServiceAccount: [email protected]
* delegates: []
* scopes:
* - userinfo-email
* - cloud-platform
* lifetime: 300s
* oidc:
* fn::invoke:
* Function: gcp:serviceaccount:getAccountIdToken
* Arguments:
* targetServiceAccount: [email protected]
* delegates: []
* includeEmail: true
* targetAudience: https://foo.bar/
* outputs:
* oidcToken: ${oidc.idToken}
* ```
*
* ### Invoking Cloud Run Endpoint
* The following configuration will invoke [Cloud Run](https://cloud.google.com/run/docs/authenticating/service-to-service) endpoint where the service account for the provider has been granted `roles/run.invoker` role previously.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* import * as http from "@pulumi/http";
* const oidc = gcp.serviceaccount.getAccountIdToken({
* targetAudience: "https://your.cloud.run.app/",
* });
* const cloudrun = oidc.then(oidc => http.getHttp({
* url: "https://your.cloud.run.app/",
* requestHeaders: {
* Authorization: `Bearer ${oidc.idToken}`,
* },
* }));
* export const cloudRunResponse = cloudrun.then(cloudrun => cloudrun.body);
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* import pulumi_http as http
* oidc = gcp.serviceaccount.get_account_id_token(target_audience="https://your.cloud.run.app/")
* cloudrun = http.get_http(url="https://your.cloud.run.app/",
* request_headers={
* "Authorization": f"Bearer {oidc.id_token}",
* })
* pulumi.export("cloudRunResponse", cloudrun.body)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* using Http = Pulumi.Http;
* return await Deployment.RunAsync(() =>
* {
* var oidc = Gcp.ServiceAccount.GetAccountIdToken.Invoke(new()
* {
* TargetAudience = "https://your.cloud.run.app/",
* });
* var cloudrun = Http.GetHttp.Invoke(new()
* {
* Url = "https://your.cloud.run.app/",
* RequestHeaders =
* {
* { "Authorization", $"Bearer {oidc.Apply(getAccountIdTokenResult => getAccountIdTokenResult.IdToken)}" },
* },
* });
* return new Dictionary
* {
* ["cloudRunResponse"] = cloudrun.Apply(getHttpResult => getHttpResult.Body),
* };
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi-http/sdk/go/http"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* oidc, err := serviceaccount.GetAccountIdToken(ctx, &serviceaccount.GetAccountIdTokenArgs{
* TargetAudience: "https://your.cloud.run.app/",
* }, nil)
* if err != nil {
* return err
* }
* cloudrun, err := http.GetHttp(ctx, &http.GetHttpArgs{
* Url: "https://your.cloud.run.app/",
* RequestHeaders: map[string]interface{}{
* "Authorization": fmt.Sprintf("Bearer %v", oidc.IdToken),
* },
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("cloudRunResponse", cloudrun.Body)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions;
* import com.pulumi.gcp.serviceaccount.inputs.GetAccountIdTokenArgs;
* import com.pulumi.http.HttpFunctions;
* import com.pulumi.http.inputs.GetHttpArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var oidc = ServiceaccountFunctions.getAccountIdToken(GetAccountIdTokenArgs.builder()
* .targetAudience("https://your.cloud.run.app/")
* .build());
* final var cloudrun = HttpFunctions.getHttp(GetHttpArgs.builder()
* .url("https://your.cloud.run.app/")
* .requestHeaders(Map.of("Authorization", String.format("Bearer %s", oidc.applyValue(getAccountIdTokenResult -> getAccountIdTokenResult.idToken()))))
* .build());
* ctx.export("cloudRunResponse", cloudrun.applyValue(getHttpResult -> getHttpResult.body()));
* }
* }
* ```
* ```yaml
* variables:
* oidc:
* fn::invoke:
* Function: gcp:serviceaccount:getAccountIdToken
* Arguments:
* targetAudience: https://your.cloud.run.app/
* cloudrun:
* fn::invoke:
* Function: http:getHttp
* Arguments:
* url: https://your.cloud.run.app/
* requestHeaders:
* Authorization: Bearer ${oidc.idToken}
* outputs:
* cloudRunResponse: ${cloudrun.body}
* ```
*
* @param argument A collection of arguments for invoking getAccountIdToken.
* @return A collection of values returned by getAccountIdToken.
*/
public suspend fun getAccountIdToken(argument: GetAccountIdTokenPlainArgs): GetAccountIdTokenResult =
getAccountIdTokenResultToKotlin(getAccountIdTokenPlain(argument.toJava()).await())
/**
* @see [getAccountIdToken].
* @param delegates Delegate chain of approvals needed to perform full impersonation. Specify the fully qualified service account name. Used only when using impersonation mode.
* @param includeEmail Include the verified email in the claim. Used only when using impersonation mode.
* @param targetAudience The audience claim for the `id_token`.
* @param targetServiceAccount The email of the service account being impersonated. Used only when using impersonation mode.
* @return A collection of values returned by getAccountIdToken.
*/
public suspend fun getAccountIdToken(
delegates: List? = null,
includeEmail: Boolean? = null,
targetAudience: String,
targetServiceAccount: String? = null,
): GetAccountIdTokenResult {
val argument = GetAccountIdTokenPlainArgs(
delegates = delegates,
includeEmail = includeEmail,
targetAudience = targetAudience,
targetServiceAccount = targetServiceAccount,
)
return getAccountIdTokenResultToKotlin(getAccountIdTokenPlain(argument.toJava()).await())
}
/**
* @see [getAccountIdToken].
* @param argument Builder for [com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountIdTokenPlainArgs].
* @return A collection of values returned by getAccountIdToken.
*/
public suspend fun getAccountIdToken(argument: suspend GetAccountIdTokenPlainArgsBuilder.() -> Unit): GetAccountIdTokenResult {
val builder = GetAccountIdTokenPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccountIdTokenResultToKotlin(getAccountIdTokenPlain(builtArgument.toJava()).await())
}
/**
* This data source provides a [self-signed JWT](https://cloud.google.com/iam/docs/create-short-lived-credentials-direct#sa-credentials-jwt). Tokens issued from this data source are typically used to call external services that accept JWTs for authentication.
* ## Example Usage
* Note: in order to use the following, the caller must have _at least_ `roles/iam.serviceAccountTokenCreator` on the `target_service_account`.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const foo = gcp.serviceaccount.getAccountJwt({
* targetServiceAccount: "[email protected]",
* payload: JSON.stringify({
* foo: "bar",
* sub: "subject",
* }),
* expiresIn: 60,
* });
* export const jwt = foo.then(foo => foo.jwt);
* ```
* ```python
* import pulumi
* import json
* import pulumi_gcp as gcp
* foo = gcp.serviceaccount.get_account_jwt(target_service_account="[email protected]",
* payload=json.dumps({
* "foo": "bar",
* "sub": "subject",
* }),
* expires_in=60)
* pulumi.export("jwt", foo.jwt)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var foo = Gcp.ServiceAccount.GetAccountJwt.Invoke(new()
* {
* TargetServiceAccount = "[email protected]",
* Payload = JsonSerializer.Serialize(new Dictionary
* {
* ["foo"] = "bar",
* ["sub"] = "subject",
* }),
* ExpiresIn = 60,
* });
* return new Dictionary
* {
* ["jwt"] = foo.Apply(getAccountJwtResult => getAccountJwtResult.Jwt),
* };
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "foo": "bar",
* "sub": "subject",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* foo, err := serviceaccount.GetAccountJwt(ctx, &serviceaccount.GetAccountJwtArgs{
* TargetServiceAccount: "[email protected]",
* Payload: json0,
* ExpiresIn: pulumi.IntRef(60),
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("jwt", foo.Jwt)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions;
* import com.pulumi.gcp.serviceaccount.inputs.GetAccountJwtArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var foo = ServiceaccountFunctions.getAccountJwt(GetAccountJwtArgs.builder()
* .targetServiceAccount("[email protected]")
* .payload(serializeJson(
* jsonObject(
* jsonProperty("foo", "bar"),
* jsonProperty("sub", "subject")
* )))
* .expiresIn(60)
* .build());
* ctx.export("jwt", foo.applyValue(getAccountJwtResult -> getAccountJwtResult.jwt()));
* }
* }
* ```
* ```yaml
* variables:
* foo:
* fn::invoke:
* Function: gcp:serviceaccount:getAccountJwt
* Arguments:
* targetServiceAccount: [email protected]
* payload:
* fn::toJSON:
* foo: bar
* sub: subject
* expiresIn: 60
* outputs:
* jwt: ${foo.jwt}
* ```
*
* @param argument A collection of arguments for invoking getAccountJwt.
* @return A collection of values returned by getAccountJwt.
*/
public suspend fun getAccountJwt(argument: GetAccountJwtPlainArgs): GetAccountJwtResult =
getAccountJwtResultToKotlin(getAccountJwtPlain(argument.toJava()).await())
/**
* @see [getAccountJwt].
* @param delegates Delegate chain of approvals needed to perform full impersonation. Specify the fully qualified service account name.
* @param expiresIn Number of seconds until the JWT expires. If set and non-zero an `exp` claim will be added to the payload derived from the current timestamp plus expires_in seconds.
* @param payload The JSON-encoded JWT claims set to include in the self-signed JWT.
* @param targetServiceAccount The email of the service account that will sign the JWT.
* @return A collection of values returned by getAccountJwt.
*/
public suspend fun getAccountJwt(
delegates: List? = null,
expiresIn: Int? = null,
payload: String,
targetServiceAccount: String,
): GetAccountJwtResult {
val argument = GetAccountJwtPlainArgs(
delegates = delegates,
expiresIn = expiresIn,
payload = payload,
targetServiceAccount = targetServiceAccount,
)
return getAccountJwtResultToKotlin(getAccountJwtPlain(argument.toJava()).await())
}
/**
* @see [getAccountJwt].
* @param argument Builder for [com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountJwtPlainArgs].
* @return A collection of values returned by getAccountJwt.
*/
public suspend fun getAccountJwt(argument: suspend GetAccountJwtPlainArgsBuilder.() -> Unit): GetAccountJwtResult {
val builder = GetAccountJwtPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccountJwtResultToKotlin(getAccountJwtPlain(builtArgument.toJava()).await())
}
/**
* Get service account public key. For more information, see [the official documentation](https://cloud.google.com/iam/docs/creating-managing-service-account-keys) and [API](https://cloud.google.com/iam/reference/rest/v1/projects.serviceAccounts.keys/get).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const myaccount = new gcp.serviceaccount.Account("myaccount", {accountId: "dev-foo-account"});
* const mykeyKey = new gcp.serviceaccount.Key("mykey", {serviceAccountId: myaccount.name});
* const mykey = gcp.serviceaccount.getAccountKeyOutput({
* name: mykeyKey.name,
* publicKeyType: "TYPE_X509_PEM_FILE",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* myaccount = gcp.serviceaccount.Account("myaccount", account_id="dev-foo-account")
* mykey_key = gcp.serviceaccount.Key("mykey", service_account_id=myaccount.name)
* mykey = gcp.serviceaccount.get_account_key_output(name=mykey_key.name,
* public_key_type="TYPE_X509_PEM_FILE")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var myaccount = new Gcp.ServiceAccount.Account("myaccount", new()
* {
* AccountId = "dev-foo-account",
* });
* var mykeyKey = new Gcp.ServiceAccount.Key("mykey", new()
* {
* ServiceAccountId = myaccount.Name,
* });
* var mykey = Gcp.ServiceAccount.GetAccountKey.Invoke(new()
* {
* Name = mykeyKey.Name,
* PublicKeyType = "TYPE_X509_PEM_FILE",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* myaccount, err := serviceaccount.NewAccount(ctx, "myaccount", &serviceaccount.AccountArgs{
* AccountId: pulumi.String("dev-foo-account"),
* })
* if err != nil {
* return err
* }
* mykeyKey, err := serviceaccount.NewKey(ctx, "mykey", &serviceaccount.KeyArgs{
* ServiceAccountId: myaccount.Name,
* })
* if err != nil {
* return err
* }
* _ = serviceaccount.GetAccountKeyOutput(ctx, serviceaccount.GetAccountKeyOutputArgs{
* Name: mykeyKey.Name,
* PublicKeyType: pulumi.String("TYPE_X509_PEM_FILE"),
* }, nil)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.Account;
* import com.pulumi.gcp.serviceaccount.AccountArgs;
* import com.pulumi.gcp.serviceaccount.Key;
* import com.pulumi.gcp.serviceaccount.KeyArgs;
* import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions;
* import com.pulumi.gcp.serviceaccount.inputs.GetAccountKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myaccount = new Account("myaccount", AccountArgs.builder()
* .accountId("dev-foo-account")
* .build());
* var mykeyKey = new Key("mykeyKey", KeyArgs.builder()
* .serviceAccountId(myaccount.name())
* .build());
* final var mykey = ServiceaccountFunctions.getAccountKey(GetAccountKeyArgs.builder()
* .name(mykeyKey.name())
* .publicKeyType("TYPE_X509_PEM_FILE")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myaccount:
* type: gcp:serviceaccount:Account
* properties:
* accountId: dev-foo-account
* mykeyKey:
* type: gcp:serviceaccount:Key
* name: mykey
* properties:
* serviceAccountId: ${myaccount.name}
* variables:
* mykey:
* fn::invoke:
* Function: gcp:serviceaccount:getAccountKey
* Arguments:
* name: ${mykeyKey.name}
* publicKeyType: TYPE_X509_PEM_FILE
* ```
*
* @param argument A collection of arguments for invoking getAccountKey.
* @return A collection of values returned by getAccountKey.
*/
public suspend fun getAccountKey(argument: GetAccountKeyPlainArgs): GetAccountKeyResult =
getAccountKeyResultToKotlin(getAccountKeyPlain(argument.toJava()).await())
/**
* @see [getAccountKey].
* @param name The name of the service account key. This must have format
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}/keys/{KEYID}`, where `{ACCOUNT}`
* is the email address or unique id of the service account.
* @param project The ID of the project that the service account will be created in.
* Defaults to the provider project configuration.
* @param publicKeyType The output format of the public key requested. TYPE_X509_PEM_FILE is the default output format.
* @return A collection of values returned by getAccountKey.
*/
public suspend fun getAccountKey(
name: String,
project: String? = null,
publicKeyType: String? = null,
): GetAccountKeyResult {
val argument = GetAccountKeyPlainArgs(
name = name,
project = project,
publicKeyType = publicKeyType,
)
return getAccountKeyResultToKotlin(getAccountKeyPlain(argument.toJava()).await())
}
/**
* @see [getAccountKey].
* @param argument Builder for [com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountKeyPlainArgs].
* @return A collection of values returned by getAccountKey.
*/
public suspend fun getAccountKey(argument: suspend GetAccountKeyPlainArgsBuilder.() -> Unit): GetAccountKeyResult {
val builder = GetAccountKeyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccountKeyResultToKotlin(getAccountKeyPlain(builtArgument.toJava()).await())
}
/**
* Retrieves the current IAM policy data for a service account.
* ## example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const foo = gcp.serviceaccount.getIamPolicy({
* serviceAccountId: testAccount.name,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* foo = gcp.serviceaccount.get_iam_policy(service_account_id=test_account["name"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var foo = Gcp.ServiceAccount.GetIamPolicy.Invoke(new()
* {
* ServiceAccountId = testAccount.Name,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := serviceaccount.GetIamPolicy(ctx, &serviceaccount.GetIamPolicyArgs{
* ServiceAccountId: testAccount.Name,
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.ServiceaccountFunctions;
* import com.pulumi.gcp.serviceaccount.inputs.GetIamPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var foo = ServiceaccountFunctions.getIamPolicy(GetIamPolicyArgs.builder()
* .serviceAccountId(testAccount.name())
* .build());
* }
* }
* ```
* ```yaml
* variables:
* foo:
* fn::invoke:
* Function: gcp:serviceaccount:getIamPolicy
* Arguments:
* serviceAccountId: ${testAccount.name}
* ```
*
* @param argument A collection of arguments for invoking getIamPolicy.
* @return A collection of values returned by getIamPolicy.
*/
public suspend fun getIamPolicy(argument: GetIamPolicyPlainArgs): GetIamPolicyResult =
getIamPolicyResultToKotlin(getIamPolicyPlain(argument.toJava()).await())
/**
* @see [getIamPolicy].
* @param serviceAccountId The fully-qualified name of the service account to apply policy to.
* @return A collection of values returned by getIamPolicy.
*/
public suspend fun getIamPolicy(serviceAccountId: String): GetIamPolicyResult {
val argument = GetIamPolicyPlainArgs(
serviceAccountId = serviceAccountId,
)
return getIamPolicyResultToKotlin(getIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getIamPolicy].
* @param argument Builder for [com.pulumi.gcp.serviceaccount.kotlin.inputs.GetIamPolicyPlainArgs].
* @return A collection of values returned by getIamPolicy.
*/
public suspend fun getIamPolicy(argument: suspend GetIamPolicyPlainArgsBuilder.() -> Unit): GetIamPolicyResult {
val builder = GetIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getIamPolicyResultToKotlin(getIamPolicyPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy