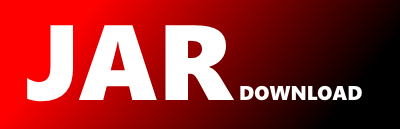
com.pulumi.gcp.serviceaccount.kotlin.inputs.GetAccountIdTokenPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.serviceaccount.kotlin.inputs
import com.pulumi.gcp.serviceaccount.inputs.GetAccountIdTokenPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getAccountIdToken.
* @property delegates Delegate chain of approvals needed to perform full impersonation. Specify the fully qualified service account name. Used only when using impersonation mode.
* @property includeEmail Include the verified email in the claim. Used only when using impersonation mode.
* @property targetAudience The audience claim for the `id_token`.
* @property targetServiceAccount The email of the service account being impersonated. Used only when using impersonation mode.
*/
public data class GetAccountIdTokenPlainArgs(
public val delegates: List? = null,
public val includeEmail: Boolean? = null,
public val targetAudience: String,
public val targetServiceAccount: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.serviceaccount.inputs.GetAccountIdTokenPlainArgs =
com.pulumi.gcp.serviceaccount.inputs.GetAccountIdTokenPlainArgs.builder()
.delegates(delegates?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.includeEmail(includeEmail?.let({ args0 -> args0 }))
.targetAudience(targetAudience.let({ args0 -> args0 }))
.targetServiceAccount(targetServiceAccount?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetAccountIdTokenPlainArgs].
*/
@PulumiTagMarker
public class GetAccountIdTokenPlainArgsBuilder internal constructor() {
private var delegates: List? = null
private var includeEmail: Boolean? = null
private var targetAudience: String? = null
private var targetServiceAccount: String? = null
/**
* @param value Delegate chain of approvals needed to perform full impersonation. Specify the fully qualified service account name. Used only when using impersonation mode.
*/
@JvmName("qoukphnfqlmbgwyg")
public suspend fun delegates(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.delegates = mapped
}
/**
* @param values Delegate chain of approvals needed to perform full impersonation. Specify the fully qualified service account name. Used only when using impersonation mode.
*/
@JvmName("aueeahsectpdxpnu")
public suspend fun delegates(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.delegates = mapped
}
/**
* @param value Include the verified email in the claim. Used only when using impersonation mode.
*/
@JvmName("fnisrgnwwkdmqqqj")
public suspend fun includeEmail(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.includeEmail = mapped
}
/**
* @param value The audience claim for the `id_token`.
*/
@JvmName("ihjjyhhodsnrcpct")
public suspend fun targetAudience(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.targetAudience = mapped
}
/**
* @param value The email of the service account being impersonated. Used only when using impersonation mode.
*/
@JvmName("agqbafkqguqdrvyw")
public suspend fun targetServiceAccount(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.targetServiceAccount = mapped
}
internal fun build(): GetAccountIdTokenPlainArgs = GetAccountIdTokenPlainArgs(
delegates = delegates,
includeEmail = includeEmail,
targetAudience = targetAudience ?: throw PulumiNullFieldException("targetAudience"),
targetServiceAccount = targetServiceAccount,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy