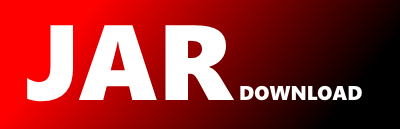
com.pulumi.gcp.sourcerepo.kotlin.RepositoryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.sourcerepo.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.sourcerepo.RepositoryArgs.builder
import com.pulumi.gcp.sourcerepo.kotlin.inputs.RepositoryPubsubConfigArgs
import com.pulumi.gcp.sourcerepo.kotlin.inputs.RepositoryPubsubConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A repository (or repo) is a Git repository storing versioned source content.
* To get more information about Repository, see:
* * [API documentation](https://cloud.google.com/source-repositories/docs/reference/rest/v1/projects.repos)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/source-repositories/)
* ## Example Usage
* ### Sourcerepo Repository Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my_repo = new gcp.sourcerepo.Repository("my-repo", {name: "my/repository"});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_repo = gcp.sourcerepo.Repository("my-repo", name="my/repository")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_repo = new Gcp.SourceRepo.Repository("my-repo", new()
* {
* Name = "my/repository",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/sourcerepo"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sourcerepo.NewRepository(ctx, "my-repo", &sourcerepo.RepositoryArgs{
* Name: pulumi.String("my/repository"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.sourcerepo.Repository;
* import com.pulumi.gcp.sourcerepo.RepositoryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .name("my/repository")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* my-repo:
* type: gcp:sourcerepo:Repository
* properties:
* name: my/repository
* ```
*
* ### Sourcerepo Repository Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const testAccount = new gcp.serviceaccount.Account("test_account", {
* accountId: "my-account",
* displayName: "Test Service Account",
* });
* const topic = new gcp.pubsub.Topic("topic", {name: "my-topic"});
* const my_repo = new gcp.sourcerepo.Repository("my-repo", {
* name: "my-repository",
* pubsubConfigs: [{
* topic: topic.id,
* messageFormat: "JSON",
* serviceAccountEmail: testAccount.email,
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* test_account = gcp.serviceaccount.Account("test_account",
* account_id="my-account",
* display_name="Test Service Account")
* topic = gcp.pubsub.Topic("topic", name="my-topic")
* my_repo = gcp.sourcerepo.Repository("my-repo",
* name="my-repository",
* pubsub_configs=[gcp.sourcerepo.RepositoryPubsubConfigArgs(
* topic=topic.id,
* message_format="JSON",
* service_account_email=test_account.email,
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var testAccount = new Gcp.ServiceAccount.Account("test_account", new()
* {
* AccountId = "my-account",
* DisplayName = "Test Service Account",
* });
* var topic = new Gcp.PubSub.Topic("topic", new()
* {
* Name = "my-topic",
* });
* var my_repo = new Gcp.SourceRepo.Repository("my-repo", new()
* {
* Name = "my-repository",
* PubsubConfigs = new[]
* {
* new Gcp.SourceRepo.Inputs.RepositoryPubsubConfigArgs
* {
* Topic = topic.Id,
* MessageFormat = "JSON",
* ServiceAccountEmail = testAccount.Email,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/sourcerepo"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* testAccount, err := serviceaccount.NewAccount(ctx, "test_account", &serviceaccount.AccountArgs{
* AccountId: pulumi.String("my-account"),
* DisplayName: pulumi.String("Test Service Account"),
* })
* if err != nil {
* return err
* }
* topic, err := pubsub.NewTopic(ctx, "topic", &pubsub.TopicArgs{
* Name: pulumi.String("my-topic"),
* })
* if err != nil {
* return err
* }
* _, err = sourcerepo.NewRepository(ctx, "my-repo", &sourcerepo.RepositoryArgs{
* Name: pulumi.String("my-repository"),
* PubsubConfigs: sourcerepo.RepositoryPubsubConfigArray{
* &sourcerepo.RepositoryPubsubConfigArgs{
* Topic: topic.ID(),
* MessageFormat: pulumi.String("JSON"),
* ServiceAccountEmail: testAccount.Email,
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.Account;
* import com.pulumi.gcp.serviceaccount.AccountArgs;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import com.pulumi.gcp.sourcerepo.Repository;
* import com.pulumi.gcp.sourcerepo.RepositoryArgs;
* import com.pulumi.gcp.sourcerepo.inputs.RepositoryPubsubConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var testAccount = new Account("testAccount", AccountArgs.builder()
* .accountId("my-account")
* .displayName("Test Service Account")
* .build());
* var topic = new Topic("topic", TopicArgs.builder()
* .name("my-topic")
* .build());
* var my_repo = new Repository("my-repo", RepositoryArgs.builder()
* .name("my-repository")
* .pubsubConfigs(RepositoryPubsubConfigArgs.builder()
* .topic(topic.id())
* .messageFormat("JSON")
* .serviceAccountEmail(testAccount.email())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* testAccount:
* type: gcp:serviceaccount:Account
* name: test_account
* properties:
* accountId: my-account
* displayName: Test Service Account
* topic:
* type: gcp:pubsub:Topic
* properties:
* name: my-topic
* my-repo:
* type: gcp:sourcerepo:Repository
* properties:
* name: my-repository
* pubsubConfigs:
* - topic: ${topic.id}
* messageFormat: JSON
* serviceAccountEmail: ${testAccount.email}
* ```
*
* ## Import
* Repository can be imported using any of these accepted formats:
* * `projects/{{project}}/repos/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, Repository can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:sourcerepo/repository:Repository default projects/{{project}}/repos/{{name}}
* ```
* ```sh
* $ pulumi import gcp:sourcerepo/repository:Repository default {{name}}
* ```
* @property name Resource name of the repository, of the form `{{repo}}`.
* The repo name may contain slashes. eg, `name/with/slash`
* - - -
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property pubsubConfigs How this repository publishes a change in the repository through Cloud Pub/Sub.
* Keyed by the topic names.
* Structure is documented below.
*/
public data class RepositoryArgs(
public val name: Output? = null,
public val project: Output? = null,
public val pubsubConfigs: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.sourcerepo.RepositoryArgs =
com.pulumi.gcp.sourcerepo.RepositoryArgs.builder()
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.pubsubConfigs(
pubsubConfigs?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [RepositoryArgs].
*/
@PulumiTagMarker
public class RepositoryArgsBuilder internal constructor() {
private var name: Output? = null
private var project: Output? = null
private var pubsubConfigs: Output>? = null
/**
* @param value Resource name of the repository, of the form `{{repo}}`.
* The repo name may contain slashes. eg, `name/with/slash`
* - - -
*/
@JvmName("bfldjuvwiiospupw")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("iihupixyqxwaghpp")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value How this repository publishes a change in the repository through Cloud Pub/Sub.
* Keyed by the topic names.
* Structure is documented below.
*/
@JvmName("hovfakugrwypwcpg")
public suspend fun pubsubConfigs(`value`: Output>) {
this.pubsubConfigs = value
}
@JvmName("inqtcolirledjadd")
public suspend fun pubsubConfigs(vararg values: Output) {
this.pubsubConfigs = Output.all(values.asList())
}
/**
* @param values How this repository publishes a change in the repository through Cloud Pub/Sub.
* Keyed by the topic names.
* Structure is documented below.
*/
@JvmName("ftqvqfnqcheojeer")
public suspend fun pubsubConfigs(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy