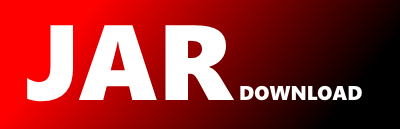
com.pulumi.gcp.sql.kotlin.SourceRepresentationInstance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.sql.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [SourceRepresentationInstance].
*/
@PulumiTagMarker
public class SourceRepresentationInstanceResourceBuilder internal constructor() {
public var name: String? = null
public var args: SourceRepresentationInstanceArgs = SourceRepresentationInstanceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend SourceRepresentationInstanceArgsBuilder.() -> Unit) {
val builder = SourceRepresentationInstanceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): SourceRepresentationInstance {
val builtJavaResource = com.pulumi.gcp.sql.SourceRepresentationInstance(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return SourceRepresentationInstance(builtJavaResource)
}
}
/**
* A source representation instance is a Cloud SQL instance that represents
* the source database server to the Cloud SQL replica. It is visible in the
* Cloud Console and appears the same as a regular Cloud SQL instance, but it
* contains no data, requires no configuration or maintenance, and does not
* affect billing. You cannot update the source representation instance.
* ## Example Usage
* ### Sql Source Representation Instance Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.sql.SourceRepresentationInstance("instance", {
* name: "my-instance",
* region: "us-central1",
* databaseVersion: "MYSQL_8_0",
* host: "10.20.30.40",
* port: 3306,
* username: "some-user",
* password: "password-for-the-user",
* dumpFilePath: "gs://replica-bucket/source-database.sql.gz",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.sql.SourceRepresentationInstance("instance",
* name="my-instance",
* region="us-central1",
* database_version="MYSQL_8_0",
* host="10.20.30.40",
* port=3306,
* username="some-user",
* password="password-for-the-user",
* dump_file_path="gs://replica-bucket/source-database.sql.gz")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.Sql.SourceRepresentationInstance("instance", new()
* {
* Name = "my-instance",
* Region = "us-central1",
* DatabaseVersion = "MYSQL_8_0",
* Host = "10.20.30.40",
* Port = 3306,
* Username = "some-user",
* Password = "password-for-the-user",
* DumpFilePath = "gs://replica-bucket/source-database.sql.gz",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/sql"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sql.NewSourceRepresentationInstance(ctx, "instance", &sql.SourceRepresentationInstanceArgs{
* Name: pulumi.String("my-instance"),
* Region: pulumi.String("us-central1"),
* DatabaseVersion: pulumi.String("MYSQL_8_0"),
* Host: pulumi.String("10.20.30.40"),
* Port: pulumi.Int(3306),
* Username: pulumi.String("some-user"),
* Password: pulumi.String("password-for-the-user"),
* DumpFilePath: pulumi.String("gs://replica-bucket/source-database.sql.gz"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.sql.SourceRepresentationInstance;
* import com.pulumi.gcp.sql.SourceRepresentationInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new SourceRepresentationInstance("instance", SourceRepresentationInstanceArgs.builder()
* .name("my-instance")
* .region("us-central1")
* .databaseVersion("MYSQL_8_0")
* .host("10.20.30.40")
* .port(3306)
* .username("some-user")
* .password("password-for-the-user")
* .dumpFilePath("gs://replica-bucket/source-database.sql.gz")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:sql:SourceRepresentationInstance
* properties:
* name: my-instance
* region: us-central1
* databaseVersion: MYSQL_8_0
* host: 10.20.30.40
* port: 3306
* username: some-user
* password: password-for-the-user
* dumpFilePath: gs://replica-bucket/source-database.sql.gz
* ```
*
* ### Sql Source Representation Instance Postgres
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.sql.SourceRepresentationInstance("instance", {
* name: "my-instance",
* region: "us-central1",
* databaseVersion: "POSTGRES_9_6",
* host: "10.20.30.40",
* port: 3306,
* username: "some-user",
* password: "password-for-the-user",
* dumpFilePath: "gs://replica-bucket/source-database.sql.gz",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.sql.SourceRepresentationInstance("instance",
* name="my-instance",
* region="us-central1",
* database_version="POSTGRES_9_6",
* host="10.20.30.40",
* port=3306,
* username="some-user",
* password="password-for-the-user",
* dump_file_path="gs://replica-bucket/source-database.sql.gz")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.Sql.SourceRepresentationInstance("instance", new()
* {
* Name = "my-instance",
* Region = "us-central1",
* DatabaseVersion = "POSTGRES_9_6",
* Host = "10.20.30.40",
* Port = 3306,
* Username = "some-user",
* Password = "password-for-the-user",
* DumpFilePath = "gs://replica-bucket/source-database.sql.gz",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/sql"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sql.NewSourceRepresentationInstance(ctx, "instance", &sql.SourceRepresentationInstanceArgs{
* Name: pulumi.String("my-instance"),
* Region: pulumi.String("us-central1"),
* DatabaseVersion: pulumi.String("POSTGRES_9_6"),
* Host: pulumi.String("10.20.30.40"),
* Port: pulumi.Int(3306),
* Username: pulumi.String("some-user"),
* Password: pulumi.String("password-for-the-user"),
* DumpFilePath: pulumi.String("gs://replica-bucket/source-database.sql.gz"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.sql.SourceRepresentationInstance;
* import com.pulumi.gcp.sql.SourceRepresentationInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new SourceRepresentationInstance("instance", SourceRepresentationInstanceArgs.builder()
* .name("my-instance")
* .region("us-central1")
* .databaseVersion("POSTGRES_9_6")
* .host("10.20.30.40")
* .port(3306)
* .username("some-user")
* .password("password-for-the-user")
* .dumpFilePath("gs://replica-bucket/source-database.sql.gz")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:sql:SourceRepresentationInstance
* properties:
* name: my-instance
* region: us-central1
* databaseVersion: POSTGRES_9_6
* host: 10.20.30.40
* port: 3306
* username: some-user
* password: password-for-the-user
* dumpFilePath: gs://replica-bucket/source-database.sql.gz
* ```
*
* ## Import
* SourceRepresentationInstance can be imported using any of these accepted formats:
* * `projects/{{project}}/instances/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, SourceRepresentationInstance can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:sql/sourceRepresentationInstance:SourceRepresentationInstance default projects/{{project}}/instances/{{name}}
* ```
* ```sh
* $ pulumi import gcp:sql/sourceRepresentationInstance:SourceRepresentationInstance default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:sql/sourceRepresentationInstance:SourceRepresentationInstance default {{name}}
* ```
*/
public class SourceRepresentationInstance internal constructor(
override val javaResource: com.pulumi.gcp.sql.SourceRepresentationInstance,
) : KotlinCustomResource(javaResource, SourceRepresentationInstanceMapper) {
/**
* The CA certificate on the external server. Include only if SSL/TLS is used on the external server.
*/
public val caCertificate: Output?
get() = javaResource.caCertificate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The client certificate on the external server. Required only for server-client authentication. Include only if SSL/TLS is used on the external server.
*/
public val clientCertificate: Output?
get() = javaResource.clientCertificate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The private key file for the client certificate on the external server. Required only for server-client authentication. Include only if SSL/TLS is used on the external server.
*/
public val clientKey: Output?
get() = javaResource.clientKey().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The MySQL version running on your source database server.
* Possible values are: `MYSQL_5_6`, `MYSQL_5_7`, `MYSQL_8_0`, `POSTGRES_9_6`, `POSTGRES_10`, `POSTGRES_11`, `POSTGRES_12`, `POSTGRES_13`, `POSTGRES_14`.
*/
public val databaseVersion: Output
get() = javaResource.databaseVersion().applyValue({ args0 -> args0 })
/**
* A file in the bucket that contains the data from the external server.
*/
public val dumpFilePath: Output?
get() = javaResource.dumpFilePath().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The IPv4 address and port for the external server, or the the DNS address for the external server. If the external server is hosted on Cloud SQL, the port is 5432.
* - - -
*/
public val host: Output
get() = javaResource.host().applyValue({ args0 -> args0 })
/**
* The name of the source representation instance. Use any valid Cloud SQL instance name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The password for the replication user account.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
public val password: Output?
get() = javaResource.password().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The externally accessible port for the source database server.
* Defaults to 3306.
*/
public val port: Output?
get() = javaResource.port().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The Region in which the created instance should reside.
* If it is not provided, the provider region is used.
*/
public val region: Output
get() = javaResource.region().applyValue({ args0 -> args0 })
/**
* The replication user account on the external server.
*/
public val username: Output?
get() = javaResource.username().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object SourceRepresentationInstanceMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.sql.SourceRepresentationInstance::class == javaResource::class
override fun map(javaResource: Resource): SourceRepresentationInstance =
SourceRepresentationInstance(javaResource as com.pulumi.gcp.sql.SourceRepresentationInstance)
}
/**
* @see [SourceRepresentationInstance].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [SourceRepresentationInstance].
*/
public suspend fun sourceRepresentationInstance(
name: String,
block: suspend SourceRepresentationInstanceResourceBuilder.() -> Unit,
): SourceRepresentationInstance {
val builder = SourceRepresentationInstanceResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [SourceRepresentationInstance].
* @param name The _unique_ name of the resulting resource.
*/
public fun sourceRepresentationInstance(name: String): SourceRepresentationInstance {
val builder = SourceRepresentationInstanceResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy