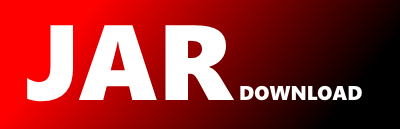
com.pulumi.gcp.sql.kotlin.SourceRepresentationInstanceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.sql.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.sql.SourceRepresentationInstanceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A source representation instance is a Cloud SQL instance that represents
* the source database server to the Cloud SQL replica. It is visible in the
* Cloud Console and appears the same as a regular Cloud SQL instance, but it
* contains no data, requires no configuration or maintenance, and does not
* affect billing. You cannot update the source representation instance.
* ## Example Usage
* ### Sql Source Representation Instance Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.sql.SourceRepresentationInstance("instance", {
* name: "my-instance",
* region: "us-central1",
* databaseVersion: "MYSQL_8_0",
* host: "10.20.30.40",
* port: 3306,
* username: "some-user",
* password: "password-for-the-user",
* dumpFilePath: "gs://replica-bucket/source-database.sql.gz",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.sql.SourceRepresentationInstance("instance",
* name="my-instance",
* region="us-central1",
* database_version="MYSQL_8_0",
* host="10.20.30.40",
* port=3306,
* username="some-user",
* password="password-for-the-user",
* dump_file_path="gs://replica-bucket/source-database.sql.gz")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.Sql.SourceRepresentationInstance("instance", new()
* {
* Name = "my-instance",
* Region = "us-central1",
* DatabaseVersion = "MYSQL_8_0",
* Host = "10.20.30.40",
* Port = 3306,
* Username = "some-user",
* Password = "password-for-the-user",
* DumpFilePath = "gs://replica-bucket/source-database.sql.gz",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/sql"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sql.NewSourceRepresentationInstance(ctx, "instance", &sql.SourceRepresentationInstanceArgs{
* Name: pulumi.String("my-instance"),
* Region: pulumi.String("us-central1"),
* DatabaseVersion: pulumi.String("MYSQL_8_0"),
* Host: pulumi.String("10.20.30.40"),
* Port: pulumi.Int(3306),
* Username: pulumi.String("some-user"),
* Password: pulumi.String("password-for-the-user"),
* DumpFilePath: pulumi.String("gs://replica-bucket/source-database.sql.gz"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.sql.SourceRepresentationInstance;
* import com.pulumi.gcp.sql.SourceRepresentationInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new SourceRepresentationInstance("instance", SourceRepresentationInstanceArgs.builder()
* .name("my-instance")
* .region("us-central1")
* .databaseVersion("MYSQL_8_0")
* .host("10.20.30.40")
* .port(3306)
* .username("some-user")
* .password("password-for-the-user")
* .dumpFilePath("gs://replica-bucket/source-database.sql.gz")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:sql:SourceRepresentationInstance
* properties:
* name: my-instance
* region: us-central1
* databaseVersion: MYSQL_8_0
* host: 10.20.30.40
* port: 3306
* username: some-user
* password: password-for-the-user
* dumpFilePath: gs://replica-bucket/source-database.sql.gz
* ```
*
* ### Sql Source Representation Instance Postgres
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.sql.SourceRepresentationInstance("instance", {
* name: "my-instance",
* region: "us-central1",
* databaseVersion: "POSTGRES_9_6",
* host: "10.20.30.40",
* port: 3306,
* username: "some-user",
* password: "password-for-the-user",
* dumpFilePath: "gs://replica-bucket/source-database.sql.gz",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.sql.SourceRepresentationInstance("instance",
* name="my-instance",
* region="us-central1",
* database_version="POSTGRES_9_6",
* host="10.20.30.40",
* port=3306,
* username="some-user",
* password="password-for-the-user",
* dump_file_path="gs://replica-bucket/source-database.sql.gz")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.Sql.SourceRepresentationInstance("instance", new()
* {
* Name = "my-instance",
* Region = "us-central1",
* DatabaseVersion = "POSTGRES_9_6",
* Host = "10.20.30.40",
* Port = 3306,
* Username = "some-user",
* Password = "password-for-the-user",
* DumpFilePath = "gs://replica-bucket/source-database.sql.gz",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/sql"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sql.NewSourceRepresentationInstance(ctx, "instance", &sql.SourceRepresentationInstanceArgs{
* Name: pulumi.String("my-instance"),
* Region: pulumi.String("us-central1"),
* DatabaseVersion: pulumi.String("POSTGRES_9_6"),
* Host: pulumi.String("10.20.30.40"),
* Port: pulumi.Int(3306),
* Username: pulumi.String("some-user"),
* Password: pulumi.String("password-for-the-user"),
* DumpFilePath: pulumi.String("gs://replica-bucket/source-database.sql.gz"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.sql.SourceRepresentationInstance;
* import com.pulumi.gcp.sql.SourceRepresentationInstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new SourceRepresentationInstance("instance", SourceRepresentationInstanceArgs.builder()
* .name("my-instance")
* .region("us-central1")
* .databaseVersion("POSTGRES_9_6")
* .host("10.20.30.40")
* .port(3306)
* .username("some-user")
* .password("password-for-the-user")
* .dumpFilePath("gs://replica-bucket/source-database.sql.gz")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:sql:SourceRepresentationInstance
* properties:
* name: my-instance
* region: us-central1
* databaseVersion: POSTGRES_9_6
* host: 10.20.30.40
* port: 3306
* username: some-user
* password: password-for-the-user
* dumpFilePath: gs://replica-bucket/source-database.sql.gz
* ```
*
* ## Import
* SourceRepresentationInstance can be imported using any of these accepted formats:
* * `projects/{{project}}/instances/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, SourceRepresentationInstance can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:sql/sourceRepresentationInstance:SourceRepresentationInstance default projects/{{project}}/instances/{{name}}
* ```
* ```sh
* $ pulumi import gcp:sql/sourceRepresentationInstance:SourceRepresentationInstance default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:sql/sourceRepresentationInstance:SourceRepresentationInstance default {{name}}
* ```
* @property caCertificate The CA certificate on the external server. Include only if SSL/TLS is used on the external server.
* @property clientCertificate The client certificate on the external server. Required only for server-client authentication. Include only if SSL/TLS is used on the external server.
* @property clientKey The private key file for the client certificate on the external server. Required only for server-client authentication. Include only if SSL/TLS is used on the external server.
* @property databaseVersion The MySQL version running on your source database server.
* Possible values are: `MYSQL_5_6`, `MYSQL_5_7`, `MYSQL_8_0`, `POSTGRES_9_6`, `POSTGRES_10`, `POSTGRES_11`, `POSTGRES_12`, `POSTGRES_13`, `POSTGRES_14`.
* @property dumpFilePath A file in the bucket that contains the data from the external server.
* @property host The IPv4 address and port for the external server, or the the DNS address for the external server. If the external server is hosted on Cloud SQL, the port is 5432.
* - - -
* @property name The name of the source representation instance. Use any valid Cloud SQL instance name.
* @property password The password for the replication user account.
* **Note**: This property is sensitive and will not be displayed in the plan.
* @property port The externally accessible port for the source database server.
* Defaults to 3306.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property region The Region in which the created instance should reside.
* If it is not provided, the provider region is used.
* @property username The replication user account on the external server.
*/
public data class SourceRepresentationInstanceArgs(
public val caCertificate: Output? = null,
public val clientCertificate: Output? = null,
public val clientKey: Output? = null,
public val databaseVersion: Output? = null,
public val dumpFilePath: Output? = null,
public val host: Output? = null,
public val name: Output? = null,
public val password: Output? = null,
public val port: Output? = null,
public val project: Output? = null,
public val region: Output? = null,
public val username: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.sql.SourceRepresentationInstanceArgs =
com.pulumi.gcp.sql.SourceRepresentationInstanceArgs.builder()
.caCertificate(caCertificate?.applyValue({ args0 -> args0 }))
.clientCertificate(clientCertificate?.applyValue({ args0 -> args0 }))
.clientKey(clientKey?.applyValue({ args0 -> args0 }))
.databaseVersion(databaseVersion?.applyValue({ args0 -> args0 }))
.dumpFilePath(dumpFilePath?.applyValue({ args0 -> args0 }))
.host(host?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.password(password?.applyValue({ args0 -> args0 }))
.port(port?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.region(region?.applyValue({ args0 -> args0 }))
.username(username?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SourceRepresentationInstanceArgs].
*/
@PulumiTagMarker
public class SourceRepresentationInstanceArgsBuilder internal constructor() {
private var caCertificate: Output? = null
private var clientCertificate: Output? = null
private var clientKey: Output? = null
private var databaseVersion: Output? = null
private var dumpFilePath: Output? = null
private var host: Output? = null
private var name: Output? = null
private var password: Output? = null
private var port: Output? = null
private var project: Output? = null
private var region: Output? = null
private var username: Output? = null
/**
* @param value The CA certificate on the external server. Include only if SSL/TLS is used on the external server.
*/
@JvmName("djybqtrwxangooew")
public suspend fun caCertificate(`value`: Output) {
this.caCertificate = value
}
/**
* @param value The client certificate on the external server. Required only for server-client authentication. Include only if SSL/TLS is used on the external server.
*/
@JvmName("cghwyiptpykhjphu")
public suspend fun clientCertificate(`value`: Output) {
this.clientCertificate = value
}
/**
* @param value The private key file for the client certificate on the external server. Required only for server-client authentication. Include only if SSL/TLS is used on the external server.
*/
@JvmName("jswlrvamfinwdlcj")
public suspend fun clientKey(`value`: Output) {
this.clientKey = value
}
/**
* @param value The MySQL version running on your source database server.
* Possible values are: `MYSQL_5_6`, `MYSQL_5_7`, `MYSQL_8_0`, `POSTGRES_9_6`, `POSTGRES_10`, `POSTGRES_11`, `POSTGRES_12`, `POSTGRES_13`, `POSTGRES_14`.
*/
@JvmName("ldpmwqythqrjqqaa")
public suspend fun databaseVersion(`value`: Output) {
this.databaseVersion = value
}
/**
* @param value A file in the bucket that contains the data from the external server.
*/
@JvmName("rqywrxqglofqthhl")
public suspend fun dumpFilePath(`value`: Output) {
this.dumpFilePath = value
}
/**
* @param value The IPv4 address and port for the external server, or the the DNS address for the external server. If the external server is hosted on Cloud SQL, the port is 5432.
* - - -
*/
@JvmName("tnyerdstwowudhfa")
public suspend fun host(`value`: Output) {
this.host = value
}
/**
* @param value The name of the source representation instance. Use any valid Cloud SQL instance name.
*/
@JvmName("rxmnbpxxmudctqoh")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The password for the replication user account.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("irfjfiermytkjyof")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value The externally accessible port for the source database server.
* Defaults to 3306.
*/
@JvmName("hayjhhcdyybfgosh")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("pbxqdvviuthpkjgp")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The Region in which the created instance should reside.
* If it is not provided, the provider region is used.
*/
@JvmName("yviiietlhchgajcu")
public suspend fun region(`value`: Output) {
this.region = value
}
/**
* @param value The replication user account on the external server.
*/
@JvmName("ybjqtkbwkfrtwane")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value The CA certificate on the external server. Include only if SSL/TLS is used on the external server.
*/
@JvmName("gqqwwfjiyfoadmky")
public suspend fun caCertificate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caCertificate = mapped
}
/**
* @param value The client certificate on the external server. Required only for server-client authentication. Include only if SSL/TLS is used on the external server.
*/
@JvmName("fwmvglkkfkwvsaki")
public suspend fun clientCertificate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientCertificate = mapped
}
/**
* @param value The private key file for the client certificate on the external server. Required only for server-client authentication. Include only if SSL/TLS is used on the external server.
*/
@JvmName("rygflawlxfrvibda")
public suspend fun clientKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientKey = mapped
}
/**
* @param value The MySQL version running on your source database server.
* Possible values are: `MYSQL_5_6`, `MYSQL_5_7`, `MYSQL_8_0`, `POSTGRES_9_6`, `POSTGRES_10`, `POSTGRES_11`, `POSTGRES_12`, `POSTGRES_13`, `POSTGRES_14`.
*/
@JvmName("rehkviffoeinpjec")
public suspend fun databaseVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseVersion = mapped
}
/**
* @param value A file in the bucket that contains the data from the external server.
*/
@JvmName("fcfghcrjjtjsecje")
public suspend fun dumpFilePath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dumpFilePath = mapped
}
/**
* @param value The IPv4 address and port for the external server, or the the DNS address for the external server. If the external server is hosted on Cloud SQL, the port is 5432.
* - - -
*/
@JvmName("werdckpsaidspxfg")
public suspend fun host(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.host = mapped
}
/**
* @param value The name of the source representation instance. Use any valid Cloud SQL instance name.
*/
@JvmName("rtwcgpnyferudmbw")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The password for the replication user account.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
@JvmName("wqibcgopsybtwdjo")
public suspend fun password(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value The externally accessible port for the source database server.
* Defaults to 3306.
*/
@JvmName("rskciykaefhgalhr")
public suspend fun port(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.port = mapped
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("cqrckbwbufpbndwk")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The Region in which the created instance should reside.
* If it is not provided, the provider region is used.
*/
@JvmName("ojwjiokxmdaujrhw")
public suspend fun region(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.region = mapped
}
/**
* @param value The replication user account on the external server.
*/
@JvmName("hryaanysjvfevbnn")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
internal fun build(): SourceRepresentationInstanceArgs = SourceRepresentationInstanceArgs(
caCertificate = caCertificate,
clientCertificate = clientCertificate,
clientKey = clientKey,
databaseVersion = databaseVersion,
dumpFilePath = dumpFilePath,
host = host,
name = name,
password = password,
port = port,
project = project,
region = region,
username = username,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy