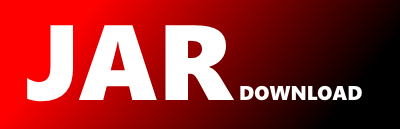
com.pulumi.gcp.sql.kotlin.inputs.DatabaseInstanceCloneArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.sql.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.sql.inputs.DatabaseInstanceCloneArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property allocatedIpRange The name of the allocated ip range for the private ip CloudSQL instance. For example: "google-managed-services-default". If set, the cloned instance ip will be created in the allocated range. The range name must comply with [RFC 1035](https://tools.ietf.org/html/rfc1035). Specifically, the name must be 1-63 characters long and match the regular expression a-z?.
* @property databaseNames (SQL Server only, use with `point_in_time`) Clone only the specified databases from the source instance. Clone all databases if empty.
* @property pointInTime The timestamp of the point in time that should be restored.
* A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits. Examples: "2014-10-02T15:01:23Z" and "2014-10-02T15:01:23.045123456Z".
* @property preferredZone (Point-in-time recovery for PostgreSQL only) Clone to an instance in the specified zone. If no zone is specified, clone to the same zone as the source instance. [clone-unavailable-instance](https://cloud.google.com/sql/docs/postgres/clone-instance#clone-unavailable-instance)
* @property sourceInstanceName Name of the source instance which will be cloned.
*/
public data class DatabaseInstanceCloneArgs(
public val allocatedIpRange: Output? = null,
public val databaseNames: Output>? = null,
public val pointInTime: Output? = null,
public val preferredZone: Output? = null,
public val sourceInstanceName: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.sql.inputs.DatabaseInstanceCloneArgs =
com.pulumi.gcp.sql.inputs.DatabaseInstanceCloneArgs.builder()
.allocatedIpRange(allocatedIpRange?.applyValue({ args0 -> args0 }))
.databaseNames(databaseNames?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.pointInTime(pointInTime?.applyValue({ args0 -> args0 }))
.preferredZone(preferredZone?.applyValue({ args0 -> args0 }))
.sourceInstanceName(sourceInstanceName.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DatabaseInstanceCloneArgs].
*/
@PulumiTagMarker
public class DatabaseInstanceCloneArgsBuilder internal constructor() {
private var allocatedIpRange: Output? = null
private var databaseNames: Output>? = null
private var pointInTime: Output? = null
private var preferredZone: Output? = null
private var sourceInstanceName: Output? = null
/**
* @param value The name of the allocated ip range for the private ip CloudSQL instance. For example: "google-managed-services-default". If set, the cloned instance ip will be created in the allocated range. The range name must comply with [RFC 1035](https://tools.ietf.org/html/rfc1035). Specifically, the name must be 1-63 characters long and match the regular expression a-z?.
*/
@JvmName("vrrplirhbwpshaja")
public suspend fun allocatedIpRange(`value`: Output) {
this.allocatedIpRange = value
}
/**
* @param value (SQL Server only, use with `point_in_time`) Clone only the specified databases from the source instance. Clone all databases if empty.
*/
@JvmName("qfeljgvjeaadabfg")
public suspend fun databaseNames(`value`: Output>) {
this.databaseNames = value
}
@JvmName("nshqmuqrblyqyupw")
public suspend fun databaseNames(vararg values: Output) {
this.databaseNames = Output.all(values.asList())
}
/**
* @param values (SQL Server only, use with `point_in_time`) Clone only the specified databases from the source instance. Clone all databases if empty.
*/
@JvmName("ysfmuycyborcoolt")
public suspend fun databaseNames(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy