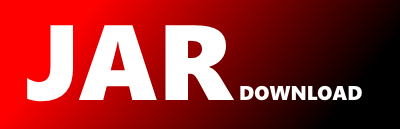
com.pulumi.gcp.sql.kotlin.inputs.DatabaseInstanceReplicaConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.sql.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.sql.inputs.DatabaseInstanceReplicaConfigurationArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property caCertificate PEM representation of the trusted CA's x509
* certificate.
* @property clientCertificate PEM representation of the replica's x509
* certificate.
* @property clientKey PEM representation of the replica's private key. The
* corresponding public key in encoded in the `client_certificate`.
* @property connectRetryInterval The number of seconds
* between connect retries. MySQL's default is 60 seconds.
* @property dumpFilePath Path to a SQL file in GCS from which replica
* instances are created. Format is `gs://bucket/filename`.
* @property failoverTarget Specifies if the replica is the failover target.
* If the field is set to true the replica will be designated as a failover replica.
* If the master instance fails, the replica instance will be promoted as
* the new master instance.
* > **NOTE:** Not supported for Postgres database.
* @property masterHeartbeatPeriod Time in ms between replication
* heartbeats.
* @property password Password for the replication connection.
* @property sslCipher Permissible ciphers for use in SSL encryption.
* @property username Username for replication connection.
* @property verifyServerCertificate True if the master's common name
* value is checked during the SSL handshake.
*/
public data class DatabaseInstanceReplicaConfigurationArgs(
public val caCertificate: Output? = null,
public val clientCertificate: Output? = null,
public val clientKey: Output? = null,
public val connectRetryInterval: Output? = null,
public val dumpFilePath: Output? = null,
public val failoverTarget: Output? = null,
public val masterHeartbeatPeriod: Output? = null,
public val password: Output? = null,
public val sslCipher: Output? = null,
public val username: Output? = null,
public val verifyServerCertificate: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.sql.inputs.DatabaseInstanceReplicaConfigurationArgs =
com.pulumi.gcp.sql.inputs.DatabaseInstanceReplicaConfigurationArgs.builder()
.caCertificate(caCertificate?.applyValue({ args0 -> args0 }))
.clientCertificate(clientCertificate?.applyValue({ args0 -> args0 }))
.clientKey(clientKey?.applyValue({ args0 -> args0 }))
.connectRetryInterval(connectRetryInterval?.applyValue({ args0 -> args0 }))
.dumpFilePath(dumpFilePath?.applyValue({ args0 -> args0 }))
.failoverTarget(failoverTarget?.applyValue({ args0 -> args0 }))
.masterHeartbeatPeriod(masterHeartbeatPeriod?.applyValue({ args0 -> args0 }))
.password(password?.applyValue({ args0 -> args0 }))
.sslCipher(sslCipher?.applyValue({ args0 -> args0 }))
.username(username?.applyValue({ args0 -> args0 }))
.verifyServerCertificate(verifyServerCertificate?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DatabaseInstanceReplicaConfigurationArgs].
*/
@PulumiTagMarker
public class DatabaseInstanceReplicaConfigurationArgsBuilder internal constructor() {
private var caCertificate: Output? = null
private var clientCertificate: Output? = null
private var clientKey: Output? = null
private var connectRetryInterval: Output? = null
private var dumpFilePath: Output? = null
private var failoverTarget: Output? = null
private var masterHeartbeatPeriod: Output? = null
private var password: Output? = null
private var sslCipher: Output? = null
private var username: Output? = null
private var verifyServerCertificate: Output? = null
/**
* @param value PEM representation of the trusted CA's x509
* certificate.
*/
@JvmName("qwbkiogcpwctrpxl")
public suspend fun caCertificate(`value`: Output) {
this.caCertificate = value
}
/**
* @param value PEM representation of the replica's x509
* certificate.
*/
@JvmName("ecihwmhulrktpxgy")
public suspend fun clientCertificate(`value`: Output) {
this.clientCertificate = value
}
/**
* @param value PEM representation of the replica's private key. The
* corresponding public key in encoded in the `client_certificate`.
*/
@JvmName("fakijgxyvbtgygoi")
public suspend fun clientKey(`value`: Output) {
this.clientKey = value
}
/**
* @param value The number of seconds
* between connect retries. MySQL's default is 60 seconds.
*/
@JvmName("wbgdaalakqrrvabh")
public suspend fun connectRetryInterval(`value`: Output) {
this.connectRetryInterval = value
}
/**
* @param value Path to a SQL file in GCS from which replica
* instances are created. Format is `gs://bucket/filename`.
*/
@JvmName("xfddtqbhbbalmxbr")
public suspend fun dumpFilePath(`value`: Output) {
this.dumpFilePath = value
}
/**
* @param value Specifies if the replica is the failover target.
* If the field is set to true the replica will be designated as a failover replica.
* If the master instance fails, the replica instance will be promoted as
* the new master instance.
* > **NOTE:** Not supported for Postgres database.
*/
@JvmName("tpfbojwpbeawmwls")
public suspend fun failoverTarget(`value`: Output) {
this.failoverTarget = value
}
/**
* @param value Time in ms between replication
* heartbeats.
*/
@JvmName("yvllvwuojkjkrecm")
public suspend fun masterHeartbeatPeriod(`value`: Output) {
this.masterHeartbeatPeriod = value
}
/**
* @param value Password for the replication connection.
*/
@JvmName("dswpmhawbdtvelwd")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value Permissible ciphers for use in SSL encryption.
*/
@JvmName("rkxearjxyishlyoj")
public suspend fun sslCipher(`value`: Output) {
this.sslCipher = value
}
/**
* @param value Username for replication connection.
*/
@JvmName("unhbbidtbmepybrj")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value True if the master's common name
* value is checked during the SSL handshake.
*/
@JvmName("pphxjmmrqefriiro")
public suspend fun verifyServerCertificate(`value`: Output) {
this.verifyServerCertificate = value
}
/**
* @param value PEM representation of the trusted CA's x509
* certificate.
*/
@JvmName("bsqmowamxsxdyrdi")
public suspend fun caCertificate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caCertificate = mapped
}
/**
* @param value PEM representation of the replica's x509
* certificate.
*/
@JvmName("mtrrrwfvgiglisvr")
public suspend fun clientCertificate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientCertificate = mapped
}
/**
* @param value PEM representation of the replica's private key. The
* corresponding public key in encoded in the `client_certificate`.
*/
@JvmName("plyhhspjxsxqohut")
public suspend fun clientKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientKey = mapped
}
/**
* @param value The number of seconds
* between connect retries. MySQL's default is 60 seconds.
*/
@JvmName("xoifwqdxnfdajlsy")
public suspend fun connectRetryInterval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectRetryInterval = mapped
}
/**
* @param value Path to a SQL file in GCS from which replica
* instances are created. Format is `gs://bucket/filename`.
*/
@JvmName("lipevorhivhhueju")
public suspend fun dumpFilePath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dumpFilePath = mapped
}
/**
* @param value Specifies if the replica is the failover target.
* If the field is set to true the replica will be designated as a failover replica.
* If the master instance fails, the replica instance will be promoted as
* the new master instance.
* > **NOTE:** Not supported for Postgres database.
*/
@JvmName("bbnwlpbyhvgiojei")
public suspend fun failoverTarget(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failoverTarget = mapped
}
/**
* @param value Time in ms between replication
* heartbeats.
*/
@JvmName("vlwnlivndajdeepq")
public suspend fun masterHeartbeatPeriod(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.masterHeartbeatPeriod = mapped
}
/**
* @param value Password for the replication connection.
*/
@JvmName("makhkoenvvhdvffd")
public suspend fun password(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value Permissible ciphers for use in SSL encryption.
*/
@JvmName("ojbyydlsrsehsftg")
public suspend fun sslCipher(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sslCipher = mapped
}
/**
* @param value Username for replication connection.
*/
@JvmName("yjmptwlfjpdhmcjk")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
/**
* @param value True if the master's common name
* value is checked during the SSL handshake.
*/
@JvmName("ufeikxqjnrlcgnan")
public suspend fun verifyServerCertificate(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.verifyServerCertificate = mapped
}
internal fun build(): DatabaseInstanceReplicaConfigurationArgs =
DatabaseInstanceReplicaConfigurationArgs(
caCertificate = caCertificate,
clientCertificate = clientCertificate,
clientKey = clientKey,
connectRetryInterval = connectRetryInterval,
dumpFilePath = dumpFilePath,
failoverTarget = failoverTarget,
masterHeartbeatPeriod = masterHeartbeatPeriod,
password = password,
sslCipher = sslCipher,
username = username,
verifyServerCertificate = verifyServerCertificate,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy