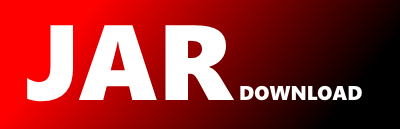
com.pulumi.gcp.sql.kotlin.inputs.DatabaseInstanceSettingsBackupConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.sql.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.sql.inputs.DatabaseInstanceSettingsBackupConfigurationArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property backupRetentionSettings Backup retention settings. The configuration is detailed below.
* @property binaryLogEnabled True if binary logging is enabled.
* Can only be used with MySQL.
* @property enabled True if backup configuration is enabled.
* @property location The region where the backup will be stored
* @property pointInTimeRecoveryEnabled True if Point-in-time recovery is enabled. Will restart database if enabled after instance creation. Valid only for PostgreSQL and SQL Server instances.
* @property startTime `HH:MM` format time indicating when backup
* configuration starts.
* @property transactionLogRetentionDays The number of days of transaction logs we retain for point in time restore, from 1-7. For PostgreSQL Enterprise Plus instances, the number of days of retained transaction logs can be set from 1 to 35.
*/
public data class DatabaseInstanceSettingsBackupConfigurationArgs(
public val backupRetentionSettings: Output? = null,
public val binaryLogEnabled: Output? = null,
public val enabled: Output? = null,
public val location: Output? = null,
public val pointInTimeRecoveryEnabled: Output? = null,
public val startTime: Output? = null,
public val transactionLogRetentionDays: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.sql.inputs.DatabaseInstanceSettingsBackupConfigurationArgs =
com.pulumi.gcp.sql.inputs.DatabaseInstanceSettingsBackupConfigurationArgs.builder()
.backupRetentionSettings(
backupRetentionSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.binaryLogEnabled(binaryLogEnabled?.applyValue({ args0 -> args0 }))
.enabled(enabled?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.pointInTimeRecoveryEnabled(pointInTimeRecoveryEnabled?.applyValue({ args0 -> args0 }))
.startTime(startTime?.applyValue({ args0 -> args0 }))
.transactionLogRetentionDays(transactionLogRetentionDays?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DatabaseInstanceSettingsBackupConfigurationArgs].
*/
@PulumiTagMarker
public class DatabaseInstanceSettingsBackupConfigurationArgsBuilder internal constructor() {
private var backupRetentionSettings:
Output? = null
private var binaryLogEnabled: Output? = null
private var enabled: Output? = null
private var location: Output? = null
private var pointInTimeRecoveryEnabled: Output? = null
private var startTime: Output? = null
private var transactionLogRetentionDays: Output? = null
/**
* @param value Backup retention settings. The configuration is detailed below.
*/
@JvmName("xspnmskgxvjdpvic")
public suspend fun backupRetentionSettings(`value`: Output) {
this.backupRetentionSettings = value
}
/**
* @param value True if binary logging is enabled.
* Can only be used with MySQL.
*/
@JvmName("jpqclpoxeyjoeqro")
public suspend fun binaryLogEnabled(`value`: Output) {
this.binaryLogEnabled = value
}
/**
* @param value True if backup configuration is enabled.
*/
@JvmName("vslfvxnidcjpilte")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value The region where the backup will be stored
*/
@JvmName("tlsacjhhlohwqpwx")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value True if Point-in-time recovery is enabled. Will restart database if enabled after instance creation. Valid only for PostgreSQL and SQL Server instances.
*/
@JvmName("tdhxkwtewwjdtvaq")
public suspend fun pointInTimeRecoveryEnabled(`value`: Output) {
this.pointInTimeRecoveryEnabled = value
}
/**
* @param value `HH:MM` format time indicating when backup
* configuration starts.
*/
@JvmName("woplclohdtekilqv")
public suspend fun startTime(`value`: Output) {
this.startTime = value
}
/**
* @param value The number of days of transaction logs we retain for point in time restore, from 1-7. For PostgreSQL Enterprise Plus instances, the number of days of retained transaction logs can be set from 1 to 35.
*/
@JvmName("rixrbuhqrsyqvepq")
public suspend fun transactionLogRetentionDays(`value`: Output) {
this.transactionLogRetentionDays = value
}
/**
* @param value Backup retention settings. The configuration is detailed below.
*/
@JvmName("mdhgokurpmkfkryv")
public suspend fun backupRetentionSettings(`value`: DatabaseInstanceSettingsBackupConfigurationBackupRetentionSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backupRetentionSettings = mapped
}
/**
* @param argument Backup retention settings. The configuration is detailed below.
*/
@JvmName("cvtpqhmypidktcds")
public suspend fun backupRetentionSettings(argument: suspend DatabaseInstanceSettingsBackupConfigurationBackupRetentionSettingsArgsBuilder.() -> Unit) {
val toBeMapped =
DatabaseInstanceSettingsBackupConfigurationBackupRetentionSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.backupRetentionSettings = mapped
}
/**
* @param value True if binary logging is enabled.
* Can only be used with MySQL.
*/
@JvmName("wkkwfyhnhlirmbxp")
public suspend fun binaryLogEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.binaryLogEnabled = mapped
}
/**
* @param value True if backup configuration is enabled.
*/
@JvmName("ploikofuhmuhojrj")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value The region where the backup will be stored
*/
@JvmName("yppwvssakkppminu")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value True if Point-in-time recovery is enabled. Will restart database if enabled after instance creation. Valid only for PostgreSQL and SQL Server instances.
*/
@JvmName("qfbkvifytpmeowmy")
public suspend fun pointInTimeRecoveryEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pointInTimeRecoveryEnabled = mapped
}
/**
* @param value `HH:MM` format time indicating when backup
* configuration starts.
*/
@JvmName("bngaaxaukmpbsqed")
public suspend fun startTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startTime = mapped
}
/**
* @param value The number of days of transaction logs we retain for point in time restore, from 1-7. For PostgreSQL Enterprise Plus instances, the number of days of retained transaction logs can be set from 1 to 35.
*/
@JvmName("hqkqvollsihdohyp")
public suspend fun transactionLogRetentionDays(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.transactionLogRetentionDays = mapped
}
internal fun build(): DatabaseInstanceSettingsBackupConfigurationArgs =
DatabaseInstanceSettingsBackupConfigurationArgs(
backupRetentionSettings = backupRetentionSettings,
binaryLogEnabled = binaryLogEnabled,
enabled = enabled,
location = location,
pointInTimeRecoveryEnabled = pointInTimeRecoveryEnabled,
startTime = startTime,
transactionLogRetentionDays = transactionLogRetentionDays,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy