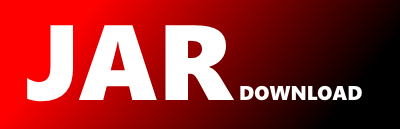
com.pulumi.gcp.sql.kotlin.inputs.DatabaseInstanceSettingsPasswordValidationPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.sql.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.sql.inputs.DatabaseInstanceSettingsPasswordValidationPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property complexity Checks if the password is a combination of lowercase, uppercase, numeric, and non-alphanumeric characters.
* @property disallowUsernameSubstring Prevents the use of the username in the password.
* @property enablePasswordPolicy Enables or disable the password validation policy.
* @property minLength Specifies the minimum number of characters that the password must have.
* @property passwordChangeInterval Specifies the minimum duration after which you can change the password.
* @property reuseInterval Specifies the number of previous passwords that you can't reuse.
*/
public data class DatabaseInstanceSettingsPasswordValidationPolicyArgs(
public val complexity: Output? = null,
public val disallowUsernameSubstring: Output? = null,
public val enablePasswordPolicy: Output,
public val minLength: Output? = null,
public val passwordChangeInterval: Output? = null,
public val reuseInterval: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.sql.inputs.DatabaseInstanceSettingsPasswordValidationPolicyArgs =
com.pulumi.gcp.sql.inputs.DatabaseInstanceSettingsPasswordValidationPolicyArgs.builder()
.complexity(complexity?.applyValue({ args0 -> args0 }))
.disallowUsernameSubstring(disallowUsernameSubstring?.applyValue({ args0 -> args0 }))
.enablePasswordPolicy(enablePasswordPolicy.applyValue({ args0 -> args0 }))
.minLength(minLength?.applyValue({ args0 -> args0 }))
.passwordChangeInterval(passwordChangeInterval?.applyValue({ args0 -> args0 }))
.reuseInterval(reuseInterval?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DatabaseInstanceSettingsPasswordValidationPolicyArgs].
*/
@PulumiTagMarker
public class DatabaseInstanceSettingsPasswordValidationPolicyArgsBuilder internal constructor() {
private var complexity: Output? = null
private var disallowUsernameSubstring: Output? = null
private var enablePasswordPolicy: Output? = null
private var minLength: Output? = null
private var passwordChangeInterval: Output? = null
private var reuseInterval: Output? = null
/**
* @param value Checks if the password is a combination of lowercase, uppercase, numeric, and non-alphanumeric characters.
*/
@JvmName("kpntjepkaaqcyebk")
public suspend fun complexity(`value`: Output) {
this.complexity = value
}
/**
* @param value Prevents the use of the username in the password.
*/
@JvmName("sbwcuqbdvohmrqvn")
public suspend fun disallowUsernameSubstring(`value`: Output) {
this.disallowUsernameSubstring = value
}
/**
* @param value Enables or disable the password validation policy.
*/
@JvmName("usaqhdkyervtegfh")
public suspend fun enablePasswordPolicy(`value`: Output) {
this.enablePasswordPolicy = value
}
/**
* @param value Specifies the minimum number of characters that the password must have.
*/
@JvmName("mtgqrqwcvoxkiifi")
public suspend fun minLength(`value`: Output) {
this.minLength = value
}
/**
* @param value Specifies the minimum duration after which you can change the password.
*/
@JvmName("lhepoqfyymhdlpdi")
public suspend fun passwordChangeInterval(`value`: Output) {
this.passwordChangeInterval = value
}
/**
* @param value Specifies the number of previous passwords that you can't reuse.
*/
@JvmName("gwjsvprvtybhwnco")
public suspend fun reuseInterval(`value`: Output) {
this.reuseInterval = value
}
/**
* @param value Checks if the password is a combination of lowercase, uppercase, numeric, and non-alphanumeric characters.
*/
@JvmName("vqyqqpjapphkdrnc")
public suspend fun complexity(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.complexity = mapped
}
/**
* @param value Prevents the use of the username in the password.
*/
@JvmName("satfcivsquxnnfuf")
public suspend fun disallowUsernameSubstring(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disallowUsernameSubstring = mapped
}
/**
* @param value Enables or disable the password validation policy.
*/
@JvmName("aiopbaoaomkeawir")
public suspend fun enablePasswordPolicy(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enablePasswordPolicy = mapped
}
/**
* @param value Specifies the minimum number of characters that the password must have.
*/
@JvmName("wqbvhpsdecufwvlq")
public suspend fun minLength(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minLength = mapped
}
/**
* @param value Specifies the minimum duration after which you can change the password.
*/
@JvmName("svjkeaocmwmfpqpc")
public suspend fun passwordChangeInterval(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.passwordChangeInterval = mapped
}
/**
* @param value Specifies the number of previous passwords that you can't reuse.
*/
@JvmName("ddunepqrnfaxpdqx")
public suspend fun reuseInterval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.reuseInterval = mapped
}
internal fun build(): DatabaseInstanceSettingsPasswordValidationPolicyArgs =
DatabaseInstanceSettingsPasswordValidationPolicyArgs(
complexity = complexity,
disallowUsernameSubstring = disallowUsernameSubstring,
enablePasswordPolicy = enablePasswordPolicy ?: throw
PulumiNullFieldException("enablePasswordPolicy"),
minLength = minLength,
passwordChangeInterval = passwordChangeInterval,
reuseInterval = reuseInterval,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy