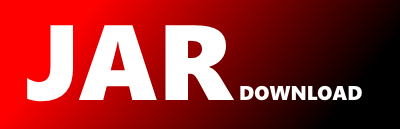
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.sql.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property activationPolicy This specifies when the instance should be
* active. Can be either `ALWAYS`, `NEVER` or `ON_DEMAND`.
* @property activeDirectoryConfig
* @property advancedMachineFeatures
* @property availabilityType The availability type of the Cloud SQL
* instance, high availability (`REGIONAL`) or single zone (`ZONAL`).' For all instances, ensure that
* `settings.backup_configuration.enabled` is set to `true`.
* For MySQL instances, ensure that `settings.backup_configuration.binary_log_enabled` is set to `true`.
* For Postgres and SQL Server instances, ensure that `settings.backup_configuration.point_in_time_recovery_enabled`
* is set to `true`. Defaults to `ZONAL`.
* @property backupConfiguration
* @property collation The name of server instance collation.
* @property connectorEnforcement Specifies if connections must use Cloud SQL connectors.
* @property dataCacheConfig Data cache configurations.
* @property databaseFlags
* @property deletionProtectionEnabled Configuration to protect against accidental instance deletion.
* @property denyMaintenancePeriod
* @property diskAutoresize Enables auto-resizing of the storage size. Defaults to `true`.
* @property diskAutoresizeLimit The maximum size to which storage capacity can be automatically increased. The default value is 0, which specifies that there is no limit.
* @property diskSize The size of data disk, in GB. Size of a running instance cannot be reduced but can be increased. The minimum value is 10GB.
* @property diskType The type of data disk: PD_SSD or PD_HDD. Defaults to `PD_SSD`.
* @property edition The edition of the instance, can be `ENTERPRISE` or `ENTERPRISE_PLUS`.
* @property enableGoogleMlIntegration Enables [Cloud SQL instances to connect to Vertex AI](https://cloud.google.com/sql/docs/postgres/integrate-cloud-sql-with-vertex-ai) and pass requests for real-time predictions and insights. Defaults to `false`.
* @property insightsConfig Configuration of Query Insights.
* @property ipConfiguration
* @property locationPreference
* @property maintenanceWindow Declares a one-hour maintenance window when an Instance can automatically restart to apply updates. The maintenance window is specified in UTC time.
* @property passwordValidationPolicy
* @property pricingPlan Pricing plan for this instance, can only be `PER_USE`.
* @property sqlServerAuditConfig
* @property tier The machine type to use. See [tiers](https://cloud.google.com/sql/docs/admin-api/v1beta4/tiers)
* for more details and supported versions. Postgres supports only shared-core machine types,
* and custom machine types such as `db-custom-2-13312`. See the [Custom Machine Type Documentation](https://cloud.google.com/compute/docs/instances/creating-instance-with-custom-machine-type#create) to learn about specifying custom machine types.
* @property timeZone The time_zone to be used by the database engine (supported only for SQL Server), in SQL Server timezone format.
* @property userLabels A set of key/value user label pairs to assign to the instance.
* @property version Used to make sure changes to the `settings` block are
* atomic.
*/
public data class DatabaseInstanceSettings(
public val activationPolicy: String? = null,
public val activeDirectoryConfig: DatabaseInstanceSettingsActiveDirectoryConfig? = null,
public val advancedMachineFeatures: DatabaseInstanceSettingsAdvancedMachineFeatures? = null,
public val availabilityType: String? = null,
public val backupConfiguration: DatabaseInstanceSettingsBackupConfiguration? = null,
public val collation: String? = null,
public val connectorEnforcement: String? = null,
public val dataCacheConfig: DatabaseInstanceSettingsDataCacheConfig? = null,
public val databaseFlags: List? = null,
public val deletionProtectionEnabled: Boolean? = null,
public val denyMaintenancePeriod: DatabaseInstanceSettingsDenyMaintenancePeriod? = null,
public val diskAutoresize: Boolean? = null,
public val diskAutoresizeLimit: Int? = null,
public val diskSize: Int? = null,
public val diskType: String? = null,
public val edition: String? = null,
public val enableGoogleMlIntegration: Boolean? = null,
public val insightsConfig: DatabaseInstanceSettingsInsightsConfig? = null,
public val ipConfiguration: DatabaseInstanceSettingsIpConfiguration? = null,
public val locationPreference: DatabaseInstanceSettingsLocationPreference? = null,
public val maintenanceWindow: DatabaseInstanceSettingsMaintenanceWindow? = null,
public val passwordValidationPolicy: DatabaseInstanceSettingsPasswordValidationPolicy? = null,
public val pricingPlan: String? = null,
public val sqlServerAuditConfig: DatabaseInstanceSettingsSqlServerAuditConfig? = null,
public val tier: String,
public val timeZone: String? = null,
public val userLabels: Map? = null,
public val version: Int? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.sql.outputs.DatabaseInstanceSettings): DatabaseInstanceSettings = DatabaseInstanceSettings(
activationPolicy = javaType.activationPolicy().map({ args0 -> args0 }).orElse(null),
activeDirectoryConfig = javaType.activeDirectoryConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsActiveDirectoryConfig.Companion.toKotlin(args0)
})
}).orElse(null),
advancedMachineFeatures = javaType.advancedMachineFeatures().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsAdvancedMachineFeatures.Companion.toKotlin(args0)
})
}).orElse(null),
availabilityType = javaType.availabilityType().map({ args0 -> args0 }).orElse(null),
backupConfiguration = javaType.backupConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsBackupConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
collation = javaType.collation().map({ args0 -> args0 }).orElse(null),
connectorEnforcement = javaType.connectorEnforcement().map({ args0 -> args0 }).orElse(null),
dataCacheConfig = javaType.dataCacheConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsDataCacheConfig.Companion.toKotlin(args0)
})
}).orElse(null),
databaseFlags = javaType.databaseFlags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsDatabaseFlag.Companion.toKotlin(args0)
})
}),
deletionProtectionEnabled = javaType.deletionProtectionEnabled().map({ args0 ->
args0
}).orElse(null),
denyMaintenancePeriod = javaType.denyMaintenancePeriod().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsDenyMaintenancePeriod.Companion.toKotlin(args0)
})
}).orElse(null),
diskAutoresize = javaType.diskAutoresize().map({ args0 -> args0 }).orElse(null),
diskAutoresizeLimit = javaType.diskAutoresizeLimit().map({ args0 -> args0 }).orElse(null),
diskSize = javaType.diskSize().map({ args0 -> args0 }).orElse(null),
diskType = javaType.diskType().map({ args0 -> args0 }).orElse(null),
edition = javaType.edition().map({ args0 -> args0 }).orElse(null),
enableGoogleMlIntegration = javaType.enableGoogleMlIntegration().map({ args0 ->
args0
}).orElse(null),
insightsConfig = javaType.insightsConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsInsightsConfig.Companion.toKotlin(args0)
})
}).orElse(null),
ipConfiguration = javaType.ipConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsIpConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
locationPreference = javaType.locationPreference().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsLocationPreference.Companion.toKotlin(args0)
})
}).orElse(null),
maintenanceWindow = javaType.maintenanceWindow().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsMaintenanceWindow.Companion.toKotlin(args0)
})
}).orElse(null),
passwordValidationPolicy = javaType.passwordValidationPolicy().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsPasswordValidationPolicy.Companion.toKotlin(args0)
})
}).orElse(null),
pricingPlan = javaType.pricingPlan().map({ args0 -> args0 }).orElse(null),
sqlServerAuditConfig = javaType.sqlServerAuditConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.DatabaseInstanceSettingsSqlServerAuditConfig.Companion.toKotlin(args0)
})
}).orElse(null),
tier = javaType.tier(),
timeZone = javaType.timeZone().map({ args0 -> args0 }).orElse(null),
userLabels = javaType.userLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
version = javaType.version().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy