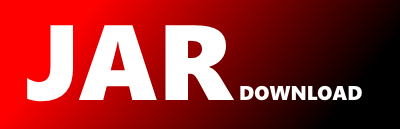
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSetting.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.sql.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property activationPolicy This specifies when the instance should be active. Can be either ALWAYS, NEVER or ON_DEMAND.
* @property activeDirectoryConfigs
* @property advancedMachineFeatures
* @property availabilityType The availability type of the Cloud SQL instance, high availability
* (REGIONAL) or single zone (ZONAL). For all instances, ensure that
* settings.backup_configuration.enabled is set to true.
* For MySQL instances, ensure that settings.backup_configuration.binary_log_enabled is set to true.
* For Postgres instances, ensure that settings.backup_configuration.point_in_time_recovery_enabled
* is set to true. Defaults to ZONAL.
* @property backupConfigurations
* @property collation The name of server instance collation.
* @property connectorEnforcement Specifies if connections must use Cloud SQL connectors.
* @property dataCacheConfigs Data cache configurations.
* @property databaseFlags
* @property deletionProtectionEnabled Configuration to protect against accidental instance deletion.
* @property denyMaintenancePeriods
* @property diskAutoresize Enables auto-resizing of the storage size. Defaults to true.
* @property diskAutoresizeLimit The maximum size, in GB, to which storage capacity can be automatically increased. The default value is 0, which specifies that there is no limit.
* @property diskSize The size of data disk, in GB. Size of a running instance cannot be reduced but can be increased. The minimum value is 10GB.
* @property diskType The type of data disk: PD_SSD or PD_HDD. Defaults to PD_SSD.
* @property edition The edition of the instance, can be ENTERPRISE or ENTERPRISE_PLUS.
* @property enableGoogleMlIntegration Enables Vertex AI Integration.
* @property insightsConfigs Configuration of Query Insights.
* @property ipConfigurations
* @property locationPreferences
* @property maintenanceWindows Declares a one-hour maintenance window when an Instance can automatically restart to apply updates. The maintenance window is specified in UTC time.
* @property passwordValidationPolicies
* @property pricingPlan Pricing plan for this instance, can only be PER_USE.
* @property sqlServerAuditConfigs
* @property tier To filter out the Cloud SQL instances based on the tier(or machine type) of the database instances.
* @property timeZone The time_zone to be used by the database engine (supported only for SQL Server), in SQL Server timezone format.
* @property userLabels A set of key/value user label pairs to assign to the instance.
* @property version Used to make sure changes to the settings block are atomic.
*/
public data class GetDatabaseInstancesInstanceSetting(
public val activationPolicy: String,
public val activeDirectoryConfigs: List,
public val advancedMachineFeatures: List,
public val availabilityType: String,
public val backupConfigurations: List,
public val collation: String,
public val connectorEnforcement: String,
public val dataCacheConfigs: List,
public val databaseFlags: List,
public val deletionProtectionEnabled: Boolean,
public val denyMaintenancePeriods: List,
public val diskAutoresize: Boolean,
public val diskAutoresizeLimit: Int,
public val diskSize: Int,
public val diskType: String,
public val edition: String,
public val enableGoogleMlIntegration: Boolean,
public val insightsConfigs: List,
public val ipConfigurations: List,
public val locationPreferences: List,
public val maintenanceWindows: List,
public val passwordValidationPolicies: List,
public val pricingPlan: String,
public val sqlServerAuditConfigs: List,
public val tier: String,
public val timeZone: String,
public val userLabels: Map,
public val version: Int,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.sql.outputs.GetDatabaseInstancesInstanceSetting): GetDatabaseInstancesInstanceSetting = GetDatabaseInstancesInstanceSetting(
activationPolicy = javaType.activationPolicy(),
activeDirectoryConfigs = javaType.activeDirectoryConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingActiveDirectoryConfig.Companion.toKotlin(args0)
})
}),
advancedMachineFeatures = javaType.advancedMachineFeatures().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingAdvancedMachineFeature.Companion.toKotlin(args0)
})
}),
availabilityType = javaType.availabilityType(),
backupConfigurations = javaType.backupConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingBackupConfiguration.Companion.toKotlin(args0)
})
}),
collation = javaType.collation(),
connectorEnforcement = javaType.connectorEnforcement(),
dataCacheConfigs = javaType.dataCacheConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingDataCacheConfig.Companion.toKotlin(args0)
})
}),
databaseFlags = javaType.databaseFlags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingDatabaseFlag.Companion.toKotlin(args0)
})
}),
deletionProtectionEnabled = javaType.deletionProtectionEnabled(),
denyMaintenancePeriods = javaType.denyMaintenancePeriods().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingDenyMaintenancePeriod.Companion.toKotlin(args0)
})
}),
diskAutoresize = javaType.diskAutoresize(),
diskAutoresizeLimit = javaType.diskAutoresizeLimit(),
diskSize = javaType.diskSize(),
diskType = javaType.diskType(),
edition = javaType.edition(),
enableGoogleMlIntegration = javaType.enableGoogleMlIntegration(),
insightsConfigs = javaType.insightsConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingInsightsConfig.Companion.toKotlin(args0)
})
}),
ipConfigurations = javaType.ipConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingIpConfiguration.Companion.toKotlin(args0)
})
}),
locationPreferences = javaType.locationPreferences().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingLocationPreference.Companion.toKotlin(args0)
})
}),
maintenanceWindows = javaType.maintenanceWindows().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingMaintenanceWindow.Companion.toKotlin(args0)
})
}),
passwordValidationPolicies = javaType.passwordValidationPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingPasswordValidationPolicy.Companion.toKotlin(args0)
})
}),
pricingPlan = javaType.pricingPlan(),
sqlServerAuditConfigs = javaType.sqlServerAuditConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.sql.kotlin.outputs.GetDatabaseInstancesInstanceSettingSqlServerAuditConfig.Companion.toKotlin(args0)
})
}),
tier = javaType.tier(),
timeZone = javaType.timeZone(),
userLabels = javaType.userLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
version = javaType.version(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy