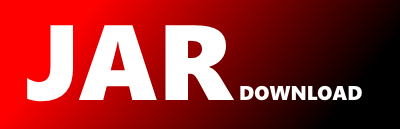
com.pulumi.gcp.storage.kotlin.BucketAccessControl.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.storage.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [BucketAccessControl].
*/
@PulumiTagMarker
public class BucketAccessControlResourceBuilder internal constructor() {
public var name: String? = null
public var args: BucketAccessControlArgs = BucketAccessControlArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BucketAccessControlArgsBuilder.() -> Unit) {
val builder = BucketAccessControlArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): BucketAccessControl {
val builtJavaResource = com.pulumi.gcp.storage.BucketAccessControl(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return BucketAccessControl(builtJavaResource)
}
}
/**
* Bucket ACLs can be managed authoritatively using the
* `storage_bucket_acl` resource. Do not use these two resources in conjunction to manage the same bucket.
* The BucketAccessControls resource manages the Access Control List
* (ACLs) for a single entity/role pairing on a bucket. ACLs let you specify who
* has access to your data and to what extent.
* There are three roles that can be assigned to an entity:
* READERs can get the bucket, though no acl property will be returned, and
* list the bucket's objects. WRITERs are READERs, and they can insert
* objects into the bucket and delete the bucket's objects. OWNERs are
* WRITERs, and they can get the acl property of a bucket, update a bucket,
* and call all BucketAccessControls methods on the bucket. For more
* information, see Access Control, with the caveat that this API uses
* READER, WRITER, and OWNER instead of READ, WRITE, and FULL_CONTROL.
* To get more information about BucketAccessControl, see:
* * [API documentation](https://cloud.google.com/storage/docs/json_api/v1/bucketAccessControls)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/storage/docs/access-control/lists)
* ## Example Usage
* ### Storage Bucket Access Control Public Bucket
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const bucket = new gcp.storage.Bucket("bucket", {
* name: "static-content-bucket",
* location: "US",
* });
* const publicRule = new gcp.storage.BucketAccessControl("public_rule", {
* bucket: bucket.name,
* role: "READER",
* entity: "allUsers",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* bucket = gcp.storage.Bucket("bucket",
* name="static-content-bucket",
* location="US")
* public_rule = gcp.storage.BucketAccessControl("public_rule",
* bucket=bucket.name,
* role="READER",
* entity="allUsers")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var bucket = new Gcp.Storage.Bucket("bucket", new()
* {
* Name = "static-content-bucket",
* Location = "US",
* });
* var publicRule = new Gcp.Storage.BucketAccessControl("public_rule", new()
* {
* Bucket = bucket.Name,
* Role = "READER",
* Entity = "allUsers",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* bucket, err := storage.NewBucket(ctx, "bucket", &storage.BucketArgs{
* Name: pulumi.String("static-content-bucket"),
* Location: pulumi.String("US"),
* })
* if err != nil {
* return err
* }
* _, err = storage.NewBucketAccessControl(ctx, "public_rule", &storage.BucketAccessControlArgs{
* Bucket: bucket.Name,
* Role: pulumi.String("READER"),
* Entity: pulumi.String("allUsers"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.storage.BucketAccessControl;
* import com.pulumi.gcp.storage.BucketAccessControlArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .name("static-content-bucket")
* .location("US")
* .build());
* var publicRule = new BucketAccessControl("publicRule", BucketAccessControlArgs.builder()
* .bucket(bucket.name())
* .role("READER")
* .entity("allUsers")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* publicRule:
* type: gcp:storage:BucketAccessControl
* name: public_rule
* properties:
* bucket: ${bucket.name}
* role: READER
* entity: allUsers
* bucket:
* type: gcp:storage:Bucket
* properties:
* name: static-content-bucket
* location: US
* ```
*
* ## Import
* BucketAccessControl can be imported using any of these accepted formats:
* * `{{bucket}}/{{entity}}`
* When using the `pulumi import` command, BucketAccessControl can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:storage/bucketAccessControl:BucketAccessControl default {{bucket}}/{{entity}}
* ```
*/
public class BucketAccessControl internal constructor(
override val javaResource: com.pulumi.gcp.storage.BucketAccessControl,
) : KotlinCustomResource(javaResource, BucketAccessControlMapper) {
/**
* The name of the bucket.
*/
public val bucket: Output
get() = javaResource.bucket().applyValue({ args0 -> args0 })
/**
* The domain associated with the entity.
*/
public val domain: Output
get() = javaResource.domain().applyValue({ args0 -> args0 })
/**
* The email address associated with the entity.
*/
public val email: Output
get() = javaResource.email().applyValue({ args0 -> args0 })
/**
* The entity holding the permission, in one of the following forms:
* user-userId
* user-email
* group-groupId
* group-email
* domain-domain
* project-team-projectId
* allUsers
* allAuthenticatedUsers
* Examples:
* The user [email protected] would be [email protected].
* The group [email protected] would be
* [email protected].
* To refer to all members of the Google Apps for Business domain
* example.com, the entity would be domain-example.com.
* - - -
*/
public val entity: Output
get() = javaResource.entity().applyValue({ args0 -> args0 })
/**
* The access permission for the entity.
* Possible values are: `OWNER`, `READER`, `WRITER`.
*/
public val role: Output?
get() = javaResource.role().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object BucketAccessControlMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.storage.BucketAccessControl::class == javaResource::class
override fun map(javaResource: Resource): BucketAccessControl = BucketAccessControl(
javaResource
as com.pulumi.gcp.storage.BucketAccessControl,
)
}
/**
* @see [BucketAccessControl].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [BucketAccessControl].
*/
public suspend fun bucketAccessControl(
name: String,
block: suspend BucketAccessControlResourceBuilder.() -> Unit,
): BucketAccessControl {
val builder = BucketAccessControlResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [BucketAccessControl].
* @param name The _unique_ name of the resulting resource.
*/
public fun bucketAccessControl(name: String): BucketAccessControl {
val builder = BucketAccessControlResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy