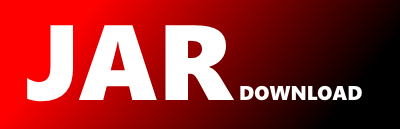
com.pulumi.gcp.storage.kotlin.DefaultObjectACLArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.storage.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.storage.DefaultObjectACLArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Authoritatively manages the default object ACLs for a Google Cloud Storage bucket
* without managing the bucket itself.
* > Note that for each object, its creator will have the `"OWNER"` role in addition
* to the default ACL that has been defined.
* For more information see
* [the official documentation](https://cloud.google.com/storage/docs/access-control/lists)
* and
* [API](https://cloud.google.com/storage/docs/json_api/v1/defaultObjectAccessControls).
* > Want fine-grained control over default object ACLs? Use `gcp.storage.DefaultObjectAccessControl`
* to control individual role entity pairs.
* ## Example Usage
* Example creating a default object ACL on a bucket with one owner, and one reader.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const image_store = new gcp.storage.Bucket("image-store", {
* name: "image-store-bucket",
* location: "EU",
* });
* const image_store_default_acl = new gcp.storage.DefaultObjectACL("image-store-default-acl", {
* bucket: image_store.name,
* roleEntities: [
* "OWNER:[email protected]",
* "READER:group-mygroup",
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* image_store = gcp.storage.Bucket("image-store",
* name="image-store-bucket",
* location="EU")
* image_store_default_acl = gcp.storage.DefaultObjectACL("image-store-default-acl",
* bucket=image_store.name,
* role_entities=[
* "OWNER:[email protected]",
* "READER:group-mygroup",
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var image_store = new Gcp.Storage.Bucket("image-store", new()
* {
* Name = "image-store-bucket",
* Location = "EU",
* });
* var image_store_default_acl = new Gcp.Storage.DefaultObjectACL("image-store-default-acl", new()
* {
* Bucket = image_store.Name,
* RoleEntities = new[]
* {
* "OWNER:[email protected]",
* "READER:group-mygroup",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.NewBucket(ctx, "image-store", &storage.BucketArgs{
* Name: pulumi.String("image-store-bucket"),
* Location: pulumi.String("EU"),
* })
* if err != nil {
* return err
* }
* _, err = storage.NewDefaultObjectACL(ctx, "image-store-default-acl", &storage.DefaultObjectACLArgs{
* Bucket: image_store.Name,
* RoleEntities: pulumi.StringArray{
* pulumi.String("OWNER:[email protected]"),
* pulumi.String("READER:group-mygroup"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.storage.DefaultObjectACL;
* import com.pulumi.gcp.storage.DefaultObjectACLArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var image_store = new Bucket("image-store", BucketArgs.builder()
* .name("image-store-bucket")
* .location("EU")
* .build());
* var image_store_default_acl = new DefaultObjectACL("image-store-default-acl", DefaultObjectACLArgs.builder()
* .bucket(image_store.name())
* .roleEntities(
* "OWNER:[email protected]",
* "READER:group-mygroup")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* image-store:
* type: gcp:storage:Bucket
* properties:
* name: image-store-bucket
* location: EU
* image-store-default-acl:
* type: gcp:storage:DefaultObjectACL
* properties:
* bucket: ${["image-store"].name}
* roleEntities:
* - OWNER:[email protected]
* - READER:group-mygroup
* ```
*
* ## Import
* This resource does not support import.
* @property bucket The name of the bucket it applies to.
* @property roleEntities List of role/entity pairs in the form `ROLE:entity`.
* See [GCS Object ACL documentation](https://cloud.google.com/storage/docs/json_api/v1/objectAccessControls) for more details.
* Omitting the field is the same as providing an empty list.
*/
public data class DefaultObjectACLArgs(
public val bucket: Output? = null,
public val roleEntities: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.storage.DefaultObjectACLArgs =
com.pulumi.gcp.storage.DefaultObjectACLArgs.builder()
.bucket(bucket?.applyValue({ args0 -> args0 }))
.roleEntities(roleEntities?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [DefaultObjectACLArgs].
*/
@PulumiTagMarker
public class DefaultObjectACLArgsBuilder internal constructor() {
private var bucket: Output? = null
private var roleEntities: Output>? = null
/**
* @param value The name of the bucket it applies to.
*/
@JvmName("lbjncwbfgscgtdmk")
public suspend fun bucket(`value`: Output) {
this.bucket = value
}
/**
* @param value List of role/entity pairs in the form `ROLE:entity`.
* See [GCS Object ACL documentation](https://cloud.google.com/storage/docs/json_api/v1/objectAccessControls) for more details.
* Omitting the field is the same as providing an empty list.
*/
@JvmName("lhexkmrigwvqmebi")
public suspend fun roleEntities(`value`: Output>) {
this.roleEntities = value
}
@JvmName("sgextmkrubgaceac")
public suspend fun roleEntities(vararg values: Output) {
this.roleEntities = Output.all(values.asList())
}
/**
* @param values List of role/entity pairs in the form `ROLE:entity`.
* See [GCS Object ACL documentation](https://cloud.google.com/storage/docs/json_api/v1/objectAccessControls) for more details.
* Omitting the field is the same as providing an empty list.
*/
@JvmName("uadryidymdhxrrlt")
public suspend fun roleEntities(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy