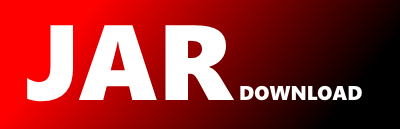
com.pulumi.gcp.storage.kotlin.ObjectAccessControl.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.storage.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.storage.kotlin.outputs.ObjectAccessControlProjectTeam
import com.pulumi.gcp.storage.kotlin.outputs.ObjectAccessControlProjectTeam.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [ObjectAccessControl].
*/
@PulumiTagMarker
public class ObjectAccessControlResourceBuilder internal constructor() {
public var name: String? = null
public var args: ObjectAccessControlArgs = ObjectAccessControlArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ObjectAccessControlArgsBuilder.() -> Unit) {
val builder = ObjectAccessControlArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ObjectAccessControl {
val builtJavaResource = com.pulumi.gcp.storage.ObjectAccessControl(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ObjectAccessControl(builtJavaResource)
}
}
/**
* The ObjectAccessControls resources represent the Access Control Lists
* (ACLs) for objects within Google Cloud Storage. ACLs let you specify
* who has access to your data and to what extent.
* There are two roles that can be assigned to an entity:
* READERs can get an object, though the acl property will not be revealed.
* OWNERs are READERs, and they can get the acl property, update an object,
* and call all objectAccessControls methods on the object. The owner of an
* object is always an OWNER.
* For more information, see Access Control, with the caveat that this API
* uses READER and OWNER instead of READ and FULL_CONTROL.
* To get more information about ObjectAccessControl, see:
* * [API documentation](https://cloud.google.com/storage/docs/json_api/v1/objectAccessControls)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/storage/docs/access-control/create-manage-lists)
* ## Example Usage
* ### Storage Object Access Control Public Object
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const bucket = new gcp.storage.Bucket("bucket", {
* name: "static-content-bucket",
* location: "US",
* });
* const object = new gcp.storage.BucketObject("object", {
* name: "public-object",
* bucket: bucket.name,
* source: new pulumi.asset.FileAsset("../static/img/header-logo.png"),
* });
* const publicRule = new gcp.storage.ObjectAccessControl("public_rule", {
* object: object.outputName,
* bucket: bucket.name,
* role: "READER",
* entity: "allUsers",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* bucket = gcp.storage.Bucket("bucket",
* name="static-content-bucket",
* location="US")
* object = gcp.storage.BucketObject("object",
* name="public-object",
* bucket=bucket.name,
* source=pulumi.FileAsset("../static/img/header-logo.png"))
* public_rule = gcp.storage.ObjectAccessControl("public_rule",
* object=object.output_name,
* bucket=bucket.name,
* role="READER",
* entity="allUsers")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var bucket = new Gcp.Storage.Bucket("bucket", new()
* {
* Name = "static-content-bucket",
* Location = "US",
* });
* var @object = new Gcp.Storage.BucketObject("object", new()
* {
* Name = "public-object",
* Bucket = bucket.Name,
* Source = new FileAsset("../static/img/header-logo.png"),
* });
* var publicRule = new Gcp.Storage.ObjectAccessControl("public_rule", new()
* {
* Object = @object.OutputName,
* Bucket = bucket.Name,
* Role = "READER",
* Entity = "allUsers",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* bucket, err := storage.NewBucket(ctx, "bucket", &storage.BucketArgs{
* Name: pulumi.String("static-content-bucket"),
* Location: pulumi.String("US"),
* })
* if err != nil {
* return err
* }
* object, err := storage.NewBucketObject(ctx, "object", &storage.BucketObjectArgs{
* Name: pulumi.String("public-object"),
* Bucket: bucket.Name,
* Source: pulumi.NewFileAsset("../static/img/header-logo.png"),
* })
* if err != nil {
* return err
* }
* _, err = storage.NewObjectAccessControl(ctx, "public_rule", &storage.ObjectAccessControlArgs{
* Object: object.OutputName,
* Bucket: bucket.Name,
* Role: pulumi.String("READER"),
* Entity: pulumi.String("allUsers"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.storage.BucketObject;
* import com.pulumi.gcp.storage.BucketObjectArgs;
* import com.pulumi.gcp.storage.ObjectAccessControl;
* import com.pulumi.gcp.storage.ObjectAccessControlArgs;
* import com.pulumi.asset.FileAsset;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .name("static-content-bucket")
* .location("US")
* .build());
* var object = new BucketObject("object", BucketObjectArgs.builder()
* .name("public-object")
* .bucket(bucket.name())
* .source(new FileAsset("../static/img/header-logo.png"))
* .build());
* var publicRule = new ObjectAccessControl("publicRule", ObjectAccessControlArgs.builder()
* .object(object.outputName())
* .bucket(bucket.name())
* .role("READER")
* .entity("allUsers")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* publicRule:
* type: gcp:storage:ObjectAccessControl
* name: public_rule
* properties:
* object: ${object.outputName}
* bucket: ${bucket.name}
* role: READER
* entity: allUsers
* bucket:
* type: gcp:storage:Bucket
* properties:
* name: static-content-bucket
* location: US
* object:
* type: gcp:storage:BucketObject
* properties:
* name: public-object
* bucket: ${bucket.name}
* source:
* fn::FileAsset: ../static/img/header-logo.png
* ```
*
* ## Import
* ObjectAccessControl can be imported using any of these accepted formats:
* * `{{bucket}}/{{object}}/{{entity}}`
* When using the `pulumi import` command, ObjectAccessControl can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:storage/objectAccessControl:ObjectAccessControl default {{bucket}}/{{object}}/{{entity}}
* ```
*/
public class ObjectAccessControl internal constructor(
override val javaResource: com.pulumi.gcp.storage.ObjectAccessControl,
) : KotlinCustomResource(javaResource, ObjectAccessControlMapper) {
/**
* The name of the bucket.
*/
public val bucket: Output
get() = javaResource.bucket().applyValue({ args0 -> args0 })
/**
* The domain associated with the entity.
*/
public val domain: Output
get() = javaResource.domain().applyValue({ args0 -> args0 })
/**
* The email address associated with the entity.
*/
public val email: Output
get() = javaResource.email().applyValue({ args0 -> args0 })
/**
* The entity holding the permission, in one of the following forms:
* * user-{{userId}}
* * user-{{email}} (such as "[email protected]")
* * group-{{groupId}}
* * group-{{email}} (such as "[email protected]")
* * domain-{{domain}} (such as "domain-example.com")
* * project-team-{{projectId}}
* * allUsers
* * allAuthenticatedUsers
*/
public val entity: Output
get() = javaResource.entity().applyValue({ args0 -> args0 })
/**
* The ID for the entity
*/
public val entityId: Output
get() = javaResource.entityId().applyValue({ args0 -> args0 })
/**
* The content generation of the object, if applied to an object.
*/
public val generation: Output
get() = javaResource.generation().applyValue({ args0 -> args0 })
/**
* The name of the object to apply the access control to.
*/
public val `object`: Output
get() = javaResource.`object`().applyValue({ args0 -> args0 })
/**
* The project team associated with the entity
* Structure is documented below.
*/
public val projectTeams: Output>
get() = javaResource.projectTeams().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
toKotlin(args0)
})
})
})
/**
* The access permission for the entity.
* Possible values are: `OWNER`, `READER`.
* - - -
*/
public val role: Output
get() = javaResource.role().applyValue({ args0 -> args0 })
}
public object ObjectAccessControlMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.storage.ObjectAccessControl::class == javaResource::class
override fun map(javaResource: Resource): ObjectAccessControl = ObjectAccessControl(
javaResource
as com.pulumi.gcp.storage.ObjectAccessControl,
)
}
/**
* @see [ObjectAccessControl].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ObjectAccessControl].
*/
public suspend fun objectAccessControl(
name: String,
block: suspend ObjectAccessControlResourceBuilder.() -> Unit,
): ObjectAccessControl {
val builder = ObjectAccessControlResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ObjectAccessControl].
* @param name The _unique_ name of the resulting resource.
*/
public fun objectAccessControl(name: String): ObjectAccessControl {
val builder = ObjectAccessControlResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy