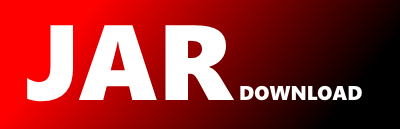
com.pulumi.gcp.storage.kotlin.inputs.BucketEncryptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.storage.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.storage.inputs.BucketEncryptionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property defaultKmsKeyName The `id` of a Cloud KMS key that will be used to encrypt objects inserted into this bucket, if no encryption method is specified.
* You must pay attention to whether the crypto key is available in the location that this bucket is created in.
* See [the docs](https://cloud.google.com/storage/docs/encryption/using-customer-managed-keys) for more details.
* > As per [the docs](https://cloud.google.com/storage/docs/encryption/using-customer-managed-keys) for customer-managed encryption keys, the IAM policy for the
* specified key must permit the [automatic Google Cloud Storage service account](https://cloud.google.com/storage/docs/projects#service-accounts) for the bucket's
* project to use the specified key for encryption and decryption operations.
* Although the service account email address follows a well-known format, the service account is created on-demand and may not necessarily exist for your project
* until a relevant action has occurred which triggers its creation.
* You should use the [`gcp.storage.getProjectServiceAccount`](https://www.terraform.io/docs/providers/google/d/storage_project_service_account.html) data source to obtain the email
* address for the service account when configuring IAM policy on the Cloud KMS key.
* This data source calls an API which creates the account if required, ensuring your provider applies cleanly and repeatedly irrespective of the
* state of the project.
* You should take care for race conditions when the same provider manages IAM policy on the Cloud KMS crypto key. See the data source page for more details.
*/
public data class BucketEncryptionArgs(
public val defaultKmsKeyName: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.storage.inputs.BucketEncryptionArgs =
com.pulumi.gcp.storage.inputs.BucketEncryptionArgs.builder()
.defaultKmsKeyName(defaultKmsKeyName.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BucketEncryptionArgs].
*/
@PulumiTagMarker
public class BucketEncryptionArgsBuilder internal constructor() {
private var defaultKmsKeyName: Output? = null
/**
* @param value The `id` of a Cloud KMS key that will be used to encrypt objects inserted into this bucket, if no encryption method is specified.
* You must pay attention to whether the crypto key is available in the location that this bucket is created in.
* See [the docs](https://cloud.google.com/storage/docs/encryption/using-customer-managed-keys) for more details.
* > As per [the docs](https://cloud.google.com/storage/docs/encryption/using-customer-managed-keys) for customer-managed encryption keys, the IAM policy for the
* specified key must permit the [automatic Google Cloud Storage service account](https://cloud.google.com/storage/docs/projects#service-accounts) for the bucket's
* project to use the specified key for encryption and decryption operations.
* Although the service account email address follows a well-known format, the service account is created on-demand and may not necessarily exist for your project
* until a relevant action has occurred which triggers its creation.
* You should use the [`gcp.storage.getProjectServiceAccount`](https://www.terraform.io/docs/providers/google/d/storage_project_service_account.html) data source to obtain the email
* address for the service account when configuring IAM policy on the Cloud KMS key.
* This data source calls an API which creates the account if required, ensuring your provider applies cleanly and repeatedly irrespective of the
* state of the project.
* You should take care for race conditions when the same provider manages IAM policy on the Cloud KMS crypto key. See the data source page for more details.
*/
@JvmName("fsbmylxoobjciglj")
public suspend fun defaultKmsKeyName(`value`: Output) {
this.defaultKmsKeyName = value
}
/**
* @param value The `id` of a Cloud KMS key that will be used to encrypt objects inserted into this bucket, if no encryption method is specified.
* You must pay attention to whether the crypto key is available in the location that this bucket is created in.
* See [the docs](https://cloud.google.com/storage/docs/encryption/using-customer-managed-keys) for more details.
* > As per [the docs](https://cloud.google.com/storage/docs/encryption/using-customer-managed-keys) for customer-managed encryption keys, the IAM policy for the
* specified key must permit the [automatic Google Cloud Storage service account](https://cloud.google.com/storage/docs/projects#service-accounts) for the bucket's
* project to use the specified key for encryption and decryption operations.
* Although the service account email address follows a well-known format, the service account is created on-demand and may not necessarily exist for your project
* until a relevant action has occurred which triggers its creation.
* You should use the [`gcp.storage.getProjectServiceAccount`](https://www.terraform.io/docs/providers/google/d/storage_project_service_account.html) data source to obtain the email
* address for the service account when configuring IAM policy on the Cloud KMS key.
* This data source calls an API which creates the account if required, ensuring your provider applies cleanly and repeatedly irrespective of the
* state of the project.
* You should take care for race conditions when the same provider manages IAM policy on the Cloud KMS crypto key. See the data source page for more details.
*/
@JvmName("whxqdeqeblxyrrwa")
public suspend fun defaultKmsKeyName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.defaultKmsKeyName = mapped
}
internal fun build(): BucketEncryptionArgs = BucketEncryptionArgs(
defaultKmsKeyName = defaultKmsKeyName ?: throw PulumiNullFieldException("defaultKmsKeyName"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy