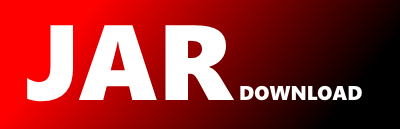
com.pulumi.gcp.storage.kotlin.inputs.BucketLifecycleRuleConditionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.storage.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.storage.inputs.BucketLifecycleRuleConditionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property age Minimum age of an object in days to satisfy this condition. If not supplied alongside another condition and without setting `no_age` to `true`, a default `age` of 0 will be set.
* @property createdBefore A date in the RFC 3339 format YYYY-MM-DD. This condition is satisfied when an object is created before midnight of the specified date in UTC.
* @property customTimeBefore A date in the RFC 3339 format YYYY-MM-DD. This condition is satisfied when the customTime metadata for the object is set to an earlier date than the date used in this lifecycle condition.
* @property daysSinceCustomTime Number of days elapsed since the user-specified timestamp set on an object.
* @property daysSinceNoncurrentTime Number of days elapsed since the noncurrent timestamp of an object. This
* condition is relevant only for versioned objects.
* @property matchesPrefixes One or more matching name prefixes to satisfy this condition.
* @property matchesStorageClasses [Storage Class](https://cloud.google.com/storage/docs/storage-classes) of objects to satisfy this condition. Supported values include: `STANDARD`, `MULTI_REGIONAL`, `REGIONAL`, `NEARLINE`, `COLDLINE`, `ARCHIVE`, `DURABLE_REDUCED_AVAILABILITY`.
* @property matchesSuffixes One or more matching name suffixes to satisfy this condition.
* @property noAge While set `true`, `age` value will be omitted from requests. This prevents a default age of `0` from being applied, and if you do not have an `age` value set, setting this to `true` is strongly recommended. When unset and other conditions are set to zero values, this can result in a rule that applies your action to all files in the bucket.
* @property noncurrentTimeBefore Creation date of an object in RFC 3339 (e.g. 2017-06-13) to satisfy this condition.
* @property numNewerVersions Relevant only for versioned objects. The number of newer versions of an object to satisfy this condition.
* @property withState Match to live and/or archived objects. Unversioned buckets have only live objects. Supported values include: `"LIVE"`, `"ARCHIVED"`, `"ANY"`.
*/
public data class BucketLifecycleRuleConditionArgs(
public val age: Output? = null,
public val createdBefore: Output? = null,
public val customTimeBefore: Output? = null,
public val daysSinceCustomTime: Output? = null,
public val daysSinceNoncurrentTime: Output? = null,
public val matchesPrefixes: Output>? = null,
public val matchesStorageClasses: Output>? = null,
public val matchesSuffixes: Output>? = null,
public val noAge: Output? = null,
public val noncurrentTimeBefore: Output? = null,
public val numNewerVersions: Output? = null,
public val withState: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.storage.inputs.BucketLifecycleRuleConditionArgs =
com.pulumi.gcp.storage.inputs.BucketLifecycleRuleConditionArgs.builder()
.age(age?.applyValue({ args0 -> args0 }))
.createdBefore(createdBefore?.applyValue({ args0 -> args0 }))
.customTimeBefore(customTimeBefore?.applyValue({ args0 -> args0 }))
.daysSinceCustomTime(daysSinceCustomTime?.applyValue({ args0 -> args0 }))
.daysSinceNoncurrentTime(daysSinceNoncurrentTime?.applyValue({ args0 -> args0 }))
.matchesPrefixes(matchesPrefixes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.matchesStorageClasses(matchesStorageClasses?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.matchesSuffixes(matchesSuffixes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.noAge(noAge?.applyValue({ args0 -> args0 }))
.noncurrentTimeBefore(noncurrentTimeBefore?.applyValue({ args0 -> args0 }))
.numNewerVersions(numNewerVersions?.applyValue({ args0 -> args0 }))
.withState(withState?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BucketLifecycleRuleConditionArgs].
*/
@PulumiTagMarker
public class BucketLifecycleRuleConditionArgsBuilder internal constructor() {
private var age: Output? = null
private var createdBefore: Output? = null
private var customTimeBefore: Output? = null
private var daysSinceCustomTime: Output? = null
private var daysSinceNoncurrentTime: Output? = null
private var matchesPrefixes: Output>? = null
private var matchesStorageClasses: Output>? = null
private var matchesSuffixes: Output>? = null
private var noAge: Output? = null
private var noncurrentTimeBefore: Output? = null
private var numNewerVersions: Output? = null
private var withState: Output? = null
/**
* @param value Minimum age of an object in days to satisfy this condition. If not supplied alongside another condition and without setting `no_age` to `true`, a default `age` of 0 will be set.
*/
@JvmName("elrddbwhykyaelbt")
public suspend fun age(`value`: Output) {
this.age = value
}
/**
* @param value A date in the RFC 3339 format YYYY-MM-DD. This condition is satisfied when an object is created before midnight of the specified date in UTC.
*/
@JvmName("giugisjvafnxhsoa")
public suspend fun createdBefore(`value`: Output) {
this.createdBefore = value
}
/**
* @param value A date in the RFC 3339 format YYYY-MM-DD. This condition is satisfied when the customTime metadata for the object is set to an earlier date than the date used in this lifecycle condition.
*/
@JvmName("kyrfubcyiyypapmt")
public suspend fun customTimeBefore(`value`: Output) {
this.customTimeBefore = value
}
/**
* @param value Number of days elapsed since the user-specified timestamp set on an object.
*/
@JvmName("swmyrtgpstqnujxy")
public suspend fun daysSinceCustomTime(`value`: Output) {
this.daysSinceCustomTime = value
}
/**
* @param value Number of days elapsed since the noncurrent timestamp of an object. This
* condition is relevant only for versioned objects.
*/
@JvmName("gcmjqecclkdlvjlm")
public suspend fun daysSinceNoncurrentTime(`value`: Output) {
this.daysSinceNoncurrentTime = value
}
/**
* @param value One or more matching name prefixes to satisfy this condition.
*/
@JvmName("lxjesudrvpvudmpb")
public suspend fun matchesPrefixes(`value`: Output>) {
this.matchesPrefixes = value
}
@JvmName("bfljvlqljjbohomv")
public suspend fun matchesPrefixes(vararg values: Output) {
this.matchesPrefixes = Output.all(values.asList())
}
/**
* @param values One or more matching name prefixes to satisfy this condition.
*/
@JvmName("odmqorledlrwimcu")
public suspend fun matchesPrefixes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy