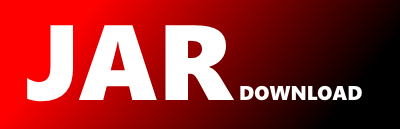
com.pulumi.gcp.storage.kotlin.inputs.GetObjectSignedUrlPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.storage.kotlin.inputs
import com.pulumi.gcp.storage.inputs.GetObjectSignedUrlPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getObjectSignedUrl.
* @property bucket The name of the bucket to read the object from
* @property contentMd5 The [MD5 digest](https://cloud.google.com/storage/docs/hashes-etags#_MD5) value in Base64.
* Typically retrieved from `google_storage_bucket_object.object.md5hash` attribute.
* If you provide this in the datasource, the client (e.g. browser, curl) must provide the `Content-MD5` HTTP header with this same value in its request.
* @property contentType If you specify this in the datasource, the client must provide the `Content-Type` HTTP header with the same value in its request.
* @property credentials What Google service account credentials json should be used to sign the URL.
* This data source checks the following locations for credentials, in order of preference: data source `credentials` attribute, provider `credentials` attribute and finally the GOOGLE_APPLICATION_CREDENTIALS environment variable.
* > **NOTE** the default google credentials configured by `gcloud` sdk or the service account associated with a compute instance cannot be used, because these do not include the private key required to sign the URL. A valid `json` service account credentials key file must be used, as generated via Google cloud console.
* @property duration For how long shall the signed URL be valid (defaults to 1 hour - i.e. `1h`).
* See [here](https://golang.org/pkg/time/#ParseDuration) for info on valid duration formats.
* @property extensionHeaders As needed. The server checks to make sure that the client provides matching values in requests using the signed URL.
* Any header starting with `x-goog-` is accepted but see the [Google Docs](https://cloud.google.com/storage/docs/xml-api/reference-headers) for list of headers that are supported by Google.
* @property httpMethod What HTTP Method will the signed URL allow (defaults to `GET`)
* @property path The full path to the object inside the bucket
*/
public data class GetObjectSignedUrlPlainArgs(
public val bucket: String,
public val contentMd5: String? = null,
public val contentType: String? = null,
public val credentials: String? = null,
public val duration: String? = null,
public val extensionHeaders: Map? = null,
public val httpMethod: String? = null,
public val path: String,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.storage.inputs.GetObjectSignedUrlPlainArgs =
com.pulumi.gcp.storage.inputs.GetObjectSignedUrlPlainArgs.builder()
.bucket(bucket.let({ args0 -> args0 }))
.contentMd5(contentMd5?.let({ args0 -> args0 }))
.contentType(contentType?.let({ args0 -> args0 }))
.credentials(credentials?.let({ args0 -> args0 }))
.duration(duration?.let({ args0 -> args0 }))
.extensionHeaders(
extensionHeaders?.let({ args0 ->
args0.map({ args0 ->
args0.key.to(args0.value)
}).toMap()
}),
)
.httpMethod(httpMethod?.let({ args0 -> args0 }))
.path(path.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetObjectSignedUrlPlainArgs].
*/
@PulumiTagMarker
public class GetObjectSignedUrlPlainArgsBuilder internal constructor() {
private var bucket: String? = null
private var contentMd5: String? = null
private var contentType: String? = null
private var credentials: String? = null
private var duration: String? = null
private var extensionHeaders: Map? = null
private var httpMethod: String? = null
private var path: String? = null
/**
* @param value The name of the bucket to read the object from
*/
@JvmName("tkxjkqvrxnuftjgr")
public suspend fun bucket(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.bucket = mapped
}
/**
* @param value The [MD5 digest](https://cloud.google.com/storage/docs/hashes-etags#_MD5) value in Base64.
* Typically retrieved from `google_storage_bucket_object.object.md5hash` attribute.
* If you provide this in the datasource, the client (e.g. browser, curl) must provide the `Content-MD5` HTTP header with this same value in its request.
*/
@JvmName("oaewppnhocriehic")
public suspend fun contentMd5(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.contentMd5 = mapped
}
/**
* @param value If you specify this in the datasource, the client must provide the `Content-Type` HTTP header with the same value in its request.
*/
@JvmName("ohaltmkpxhdxwnxh")
public suspend fun contentType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.contentType = mapped
}
/**
* @param value What Google service account credentials json should be used to sign the URL.
* This data source checks the following locations for credentials, in order of preference: data source `credentials` attribute, provider `credentials` attribute and finally the GOOGLE_APPLICATION_CREDENTIALS environment variable.
* > **NOTE** the default google credentials configured by `gcloud` sdk or the service account associated with a compute instance cannot be used, because these do not include the private key required to sign the URL. A valid `json` service account credentials key file must be used, as generated via Google cloud console.
*/
@JvmName("vwlscrtpolhsktol")
public suspend fun credentials(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.credentials = mapped
}
/**
* @param value For how long shall the signed URL be valid (defaults to 1 hour - i.e. `1h`).
* See [here](https://golang.org/pkg/time/#ParseDuration) for info on valid duration formats.
*/
@JvmName("eucaalluoumnjurt")
public suspend fun duration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.duration = mapped
}
/**
* @param value As needed. The server checks to make sure that the client provides matching values in requests using the signed URL.
* Any header starting with `x-goog-` is accepted but see the [Google Docs](https://cloud.google.com/storage/docs/xml-api/reference-headers) for list of headers that are supported by Google.
*/
@JvmName("vqmqhfepdmkktmxl")
public suspend fun extensionHeaders(`value`: Map?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.extensionHeaders = mapped
}
/**
* @param values As needed. The server checks to make sure that the client provides matching values in requests using the signed URL.
* Any header starting with `x-goog-` is accepted but see the [Google Docs](https://cloud.google.com/storage/docs/xml-api/reference-headers) for list of headers that are supported by Google.
*/
@JvmName("yhvleapsqlxghoxh")
public fun extensionHeaders(vararg values: Pair) {
val toBeMapped = values.toMap()
val mapped = toBeMapped.let({ args0 -> args0 })
this.extensionHeaders = mapped
}
/**
* @param value What HTTP Method will the signed URL allow (defaults to `GET`)
*/
@JvmName("jshfukhrtpluifrh")
public suspend fun httpMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.httpMethod = mapped
}
/**
* @param value The full path to the object inside the bucket
*/
@JvmName("vnxstskjmhsxukqf")
public suspend fun path(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.path = mapped
}
internal fun build(): GetObjectSignedUrlPlainArgs = GetObjectSignedUrlPlainArgs(
bucket = bucket ?: throw PulumiNullFieldException("bucket"),
contentMd5 = contentMd5,
contentType = contentType,
credentials = credentials,
duration = duration,
extensionHeaders = extensionHeaders,
httpMethod = httpMethod,
path = path ?: throw PulumiNullFieldException("path"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy