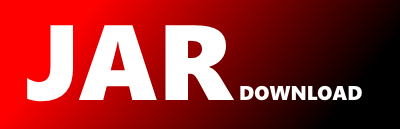
com.pulumi.gcp.storage.kotlin.inputs.TransferJobTransferSpecArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.storage.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.storage.inputs.TransferJobTransferSpecArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property awsS3DataSource An AWS S3 data source. Structure documented below.
* @property azureBlobStorageDataSource An Azure Blob Storage data source. Structure documented below.
* @property gcsDataSink A Google Cloud Storage data sink. Structure documented below.
* @property gcsDataSource A Google Cloud Storage data source. Structure documented below.
* @property httpDataSource A HTTP URL data source. Structure documented below.
* @property objectConditions Only objects that satisfy these object conditions are included in the set of data source and data sink objects. Object conditions based on objects' `last_modification_time` do not exclude objects in a data sink. Structure documented below.
* @property posixDataSink A POSIX data sink. Structure documented below.
* @property posixDataSource A POSIX filesystem data source. Structure documented below.
* @property sinkAgentPoolName Specifies the agent pool name associated with the posix data sink. When unspecified, the default name is used.
* @property sourceAgentPoolName Specifies the agent pool name associated with the posix data source. When unspecified, the default name is used.
* @property transferOptions Characteristics of how to treat files from datasource and sink during job. If the option `delete_objects_unique_in_sink` is true, object conditions based on objects' `last_modification_time` are ignored and do not exclude objects in a data source or a data sink. Structure documented below.
*/
public data class TransferJobTransferSpecArgs(
public val awsS3DataSource: Output? = null,
public val azureBlobStorageDataSource: Output? = null,
public val gcsDataSink: Output? = null,
public val gcsDataSource: Output? = null,
public val httpDataSource: Output? = null,
public val objectConditions: Output? = null,
public val posixDataSink: Output? = null,
public val posixDataSource: Output? = null,
public val sinkAgentPoolName: Output? = null,
public val sourceAgentPoolName: Output? = null,
public val transferOptions: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.storage.inputs.TransferJobTransferSpecArgs =
com.pulumi.gcp.storage.inputs.TransferJobTransferSpecArgs.builder()
.awsS3DataSource(awsS3DataSource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.azureBlobStorageDataSource(
azureBlobStorageDataSource?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.gcsDataSink(gcsDataSink?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.gcsDataSource(gcsDataSource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.httpDataSource(httpDataSource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.objectConditions(objectConditions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.posixDataSink(posixDataSink?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.posixDataSource(posixDataSource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sinkAgentPoolName(sinkAgentPoolName?.applyValue({ args0 -> args0 }))
.sourceAgentPoolName(sourceAgentPoolName?.applyValue({ args0 -> args0 }))
.transferOptions(
transferOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [TransferJobTransferSpecArgs].
*/
@PulumiTagMarker
public class TransferJobTransferSpecArgsBuilder internal constructor() {
private var awsS3DataSource: Output? = null
private var azureBlobStorageDataSource:
Output? = null
private var gcsDataSink: Output? = null
private var gcsDataSource: Output? = null
private var httpDataSource: Output? = null
private var objectConditions: Output? = null
private var posixDataSink: Output? = null
private var posixDataSource: Output? = null
private var sinkAgentPoolName: Output? = null
private var sourceAgentPoolName: Output? = null
private var transferOptions: Output? = null
/**
* @param value An AWS S3 data source. Structure documented below.
*/
@JvmName("ppownjqqwoxmjbkl")
public suspend fun awsS3DataSource(`value`: Output) {
this.awsS3DataSource = value
}
/**
* @param value An Azure Blob Storage data source. Structure documented below.
*/
@JvmName("tbcyrrtccnspjyfn")
public suspend fun azureBlobStorageDataSource(`value`: Output) {
this.azureBlobStorageDataSource = value
}
/**
* @param value A Google Cloud Storage data sink. Structure documented below.
*/
@JvmName("cqxuvrqltaagrqnn")
public suspend fun gcsDataSink(`value`: Output) {
this.gcsDataSink = value
}
/**
* @param value A Google Cloud Storage data source. Structure documented below.
*/
@JvmName("qjdwlqrxpocffqgl")
public suspend fun gcsDataSource(`value`: Output) {
this.gcsDataSource = value
}
/**
* @param value A HTTP URL data source. Structure documented below.
*/
@JvmName("lftoxlpjwisjmnld")
public suspend fun httpDataSource(`value`: Output) {
this.httpDataSource = value
}
/**
* @param value Only objects that satisfy these object conditions are included in the set of data source and data sink objects. Object conditions based on objects' `last_modification_time` do not exclude objects in a data sink. Structure documented below.
*/
@JvmName("tppocjyfroofrkby")
public suspend fun objectConditions(`value`: Output) {
this.objectConditions = value
}
/**
* @param value A POSIX data sink. Structure documented below.
*/
@JvmName("gmflbakplefwgtvl")
public suspend fun posixDataSink(`value`: Output) {
this.posixDataSink = value
}
/**
* @param value A POSIX filesystem data source. Structure documented below.
*/
@JvmName("rgmdtvpxdbwqcosm")
public suspend fun posixDataSource(`value`: Output) {
this.posixDataSource = value
}
/**
* @param value Specifies the agent pool name associated with the posix data sink. When unspecified, the default name is used.
*/
@JvmName("ugnvxsisnrfkabhd")
public suspend fun sinkAgentPoolName(`value`: Output) {
this.sinkAgentPoolName = value
}
/**
* @param value Specifies the agent pool name associated with the posix data source. When unspecified, the default name is used.
*/
@JvmName("djwskkpygcplvwmh")
public suspend fun sourceAgentPoolName(`value`: Output) {
this.sourceAgentPoolName = value
}
/**
* @param value Characteristics of how to treat files from datasource and sink during job. If the option `delete_objects_unique_in_sink` is true, object conditions based on objects' `last_modification_time` are ignored and do not exclude objects in a data source or a data sink. Structure documented below.
*/
@JvmName("spurwrvixstmxnir")
public suspend fun transferOptions(`value`: Output) {
this.transferOptions = value
}
/**
* @param value An AWS S3 data source. Structure documented below.
*/
@JvmName("jfgcmraolcwgghlt")
public suspend fun awsS3DataSource(`value`: TransferJobTransferSpecAwsS3DataSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.awsS3DataSource = mapped
}
/**
* @param argument An AWS S3 data source. Structure documented below.
*/
@JvmName("gypfkvusokmstjmc")
public suspend fun awsS3DataSource(argument: suspend TransferJobTransferSpecAwsS3DataSourceArgsBuilder.() -> Unit) {
val toBeMapped = TransferJobTransferSpecAwsS3DataSourceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.awsS3DataSource = mapped
}
/**
* @param value An Azure Blob Storage data source. Structure documented below.
*/
@JvmName("gxyprsatwludhmtr")
public suspend fun azureBlobStorageDataSource(`value`: TransferJobTransferSpecAzureBlobStorageDataSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.azureBlobStorageDataSource = mapped
}
/**
* @param argument An Azure Blob Storage data source. Structure documented below.
*/
@JvmName("swytavdrwhwbcbvi")
public suspend fun azureBlobStorageDataSource(argument: suspend TransferJobTransferSpecAzureBlobStorageDataSourceArgsBuilder.() -> Unit) {
val toBeMapped = TransferJobTransferSpecAzureBlobStorageDataSourceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.azureBlobStorageDataSource = mapped
}
/**
* @param value A Google Cloud Storage data sink. Structure documented below.
*/
@JvmName("xdjhqslwhdshpqfk")
public suspend fun gcsDataSink(`value`: TransferJobTransferSpecGcsDataSinkArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcsDataSink = mapped
}
/**
* @param argument A Google Cloud Storage data sink. Structure documented below.
*/
@JvmName("iiqrrumygirpphwm")
public suspend fun gcsDataSink(argument: suspend TransferJobTransferSpecGcsDataSinkArgsBuilder.() -> Unit) {
val toBeMapped = TransferJobTransferSpecGcsDataSinkArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.gcsDataSink = mapped
}
/**
* @param value A Google Cloud Storage data source. Structure documented below.
*/
@JvmName("otmakwgmebkxynxp")
public suspend fun gcsDataSource(`value`: TransferJobTransferSpecGcsDataSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcsDataSource = mapped
}
/**
* @param argument A Google Cloud Storage data source. Structure documented below.
*/
@JvmName("sngdpbidrmhwyqrk")
public suspend fun gcsDataSource(argument: suspend TransferJobTransferSpecGcsDataSourceArgsBuilder.() -> Unit) {
val toBeMapped = TransferJobTransferSpecGcsDataSourceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.gcsDataSource = mapped
}
/**
* @param value A HTTP URL data source. Structure documented below.
*/
@JvmName("bievpnogqfkdsiwh")
public suspend fun httpDataSource(`value`: TransferJobTransferSpecHttpDataSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpDataSource = mapped
}
/**
* @param argument A HTTP URL data source. Structure documented below.
*/
@JvmName("echpkbdvvmpwfofl")
public suspend fun httpDataSource(argument: suspend TransferJobTransferSpecHttpDataSourceArgsBuilder.() -> Unit) {
val toBeMapped = TransferJobTransferSpecHttpDataSourceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.httpDataSource = mapped
}
/**
* @param value Only objects that satisfy these object conditions are included in the set of data source and data sink objects. Object conditions based on objects' `last_modification_time` do not exclude objects in a data sink. Structure documented below.
*/
@JvmName("jeahntflbcwepehk")
public suspend fun objectConditions(`value`: TransferJobTransferSpecObjectConditionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.objectConditions = mapped
}
/**
* @param argument Only objects that satisfy these object conditions are included in the set of data source and data sink objects. Object conditions based on objects' `last_modification_time` do not exclude objects in a data sink. Structure documented below.
*/
@JvmName("tnpqaabklaksvxqc")
public suspend fun objectConditions(argument: suspend TransferJobTransferSpecObjectConditionsArgsBuilder.() -> Unit) {
val toBeMapped = TransferJobTransferSpecObjectConditionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.objectConditions = mapped
}
/**
* @param value A POSIX data sink. Structure documented below.
*/
@JvmName("fpioydqekewfpimj")
public suspend fun posixDataSink(`value`: TransferJobTransferSpecPosixDataSinkArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.posixDataSink = mapped
}
/**
* @param argument A POSIX data sink. Structure documented below.
*/
@JvmName("cflyndtfqktomghs")
public suspend fun posixDataSink(argument: suspend TransferJobTransferSpecPosixDataSinkArgsBuilder.() -> Unit) {
val toBeMapped = TransferJobTransferSpecPosixDataSinkArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.posixDataSink = mapped
}
/**
* @param value A POSIX filesystem data source. Structure documented below.
*/
@JvmName("wgcdafqtxwuacxli")
public suspend fun posixDataSource(`value`: TransferJobTransferSpecPosixDataSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.posixDataSource = mapped
}
/**
* @param argument A POSIX filesystem data source. Structure documented below.
*/
@JvmName("jywgtqqhvqybngfx")
public suspend fun posixDataSource(argument: suspend TransferJobTransferSpecPosixDataSourceArgsBuilder.() -> Unit) {
val toBeMapped = TransferJobTransferSpecPosixDataSourceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.posixDataSource = mapped
}
/**
* @param value Specifies the agent pool name associated with the posix data sink. When unspecified, the default name is used.
*/
@JvmName("qeympjrvxpcsfefq")
public suspend fun sinkAgentPoolName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sinkAgentPoolName = mapped
}
/**
* @param value Specifies the agent pool name associated with the posix data source. When unspecified, the default name is used.
*/
@JvmName("ovslcrutgpbyhgdd")
public suspend fun sourceAgentPoolName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceAgentPoolName = mapped
}
/**
* @param value Characteristics of how to treat files from datasource and sink during job. If the option `delete_objects_unique_in_sink` is true, object conditions based on objects' `last_modification_time` are ignored and do not exclude objects in a data source or a data sink. Structure documented below.
*/
@JvmName("kujvdcrrlnixnhrt")
public suspend fun transferOptions(`value`: TransferJobTransferSpecTransferOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.transferOptions = mapped
}
/**
* @param argument Characteristics of how to treat files from datasource and sink during job. If the option `delete_objects_unique_in_sink` is true, object conditions based on objects' `last_modification_time` are ignored and do not exclude objects in a data source or a data sink. Structure documented below.
*/
@JvmName("gaqfcwkhaoenpngx")
public suspend fun transferOptions(argument: suspend TransferJobTransferSpecTransferOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TransferJobTransferSpecTransferOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.transferOptions = mapped
}
internal fun build(): TransferJobTransferSpecArgs = TransferJobTransferSpecArgs(
awsS3DataSource = awsS3DataSource,
azureBlobStorageDataSource = azureBlobStorageDataSource,
gcsDataSink = gcsDataSink,
gcsDataSource = gcsDataSource,
httpDataSource = httpDataSource,
objectConditions = objectConditions,
posixDataSink = posixDataSink,
posixDataSource = posixDataSource,
sinkAgentPoolName = sinkAgentPoolName,
sourceAgentPoolName = sourceAgentPoolName,
transferOptions = transferOptions,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy