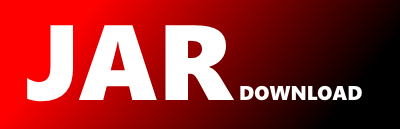
com.pulumi.gcp.storage.kotlin.outputs.GetBucketResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.storage.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getBucket.
* @property autoclasses
* @property cors
* @property customPlacementConfigs
* @property defaultEventBasedHold
* @property effectiveLabels
* @property enableObjectRetention
* @property encryptions
* @property forceDestroy
* @property id The provider-assigned unique ID for this managed resource.
* @property labels
* @property lifecycleRules
* @property location
* @property loggings
* @property name
* @property project
* @property projectNumber
* @property publicAccessPrevention
* @property pulumiLabels
* @property requesterPays
* @property retentionPolicies
* @property rpo
* @property selfLink
* @property softDeletePolicies
* @property storageClass
* @property uniformBucketLevelAccess
* @property url
* @property versionings
* @property websites
*/
public data class GetBucketResult(
public val autoclasses: List,
public val cors: List,
public val customPlacementConfigs: List,
public val defaultEventBasedHold: Boolean,
public val effectiveLabels: Map,
public val enableObjectRetention: Boolean,
public val encryptions: List,
public val forceDestroy: Boolean,
public val id: String,
public val labels: Map,
public val lifecycleRules: List,
public val location: String,
public val loggings: List,
public val name: String,
public val project: String? = null,
public val projectNumber: Int,
public val publicAccessPrevention: String,
public val pulumiLabels: Map,
public val requesterPays: Boolean,
public val retentionPolicies: List,
public val rpo: String,
public val selfLink: String,
public val softDeletePolicies: List,
public val storageClass: String,
public val uniformBucketLevelAccess: Boolean,
public val url: String,
public val versionings: List,
public val websites: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.storage.outputs.GetBucketResult): GetBucketResult =
GetBucketResult(
autoclasses = javaType.autoclasses().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketAutoclass.Companion.toKotlin(args0)
})
}),
cors = javaType.cors().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketCor.Companion.toKotlin(args0)
})
}),
customPlacementConfigs = javaType.customPlacementConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketCustomPlacementConfig.Companion.toKotlin(args0)
})
}),
defaultEventBasedHold = javaType.defaultEventBasedHold(),
effectiveLabels = javaType.effectiveLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
enableObjectRetention = javaType.enableObjectRetention(),
encryptions = javaType.encryptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketEncryption.Companion.toKotlin(args0)
})
}),
forceDestroy = javaType.forceDestroy(),
id = javaType.id(),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
lifecycleRules = javaType.lifecycleRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketLifecycleRule.Companion.toKotlin(args0)
})
}),
location = javaType.location(),
loggings = javaType.loggings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketLogging.Companion.toKotlin(args0)
})
}),
name = javaType.name(),
project = javaType.project().map({ args0 -> args0 }).orElse(null),
projectNumber = javaType.projectNumber(),
publicAccessPrevention = javaType.publicAccessPrevention(),
pulumiLabels = javaType.pulumiLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
requesterPays = javaType.requesterPays(),
retentionPolicies = javaType.retentionPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketRetentionPolicy.Companion.toKotlin(args0)
})
}),
rpo = javaType.rpo(),
selfLink = javaType.selfLink(),
softDeletePolicies = javaType.softDeletePolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketSoftDeletePolicy.Companion.toKotlin(args0)
})
}),
storageClass = javaType.storageClass(),
uniformBucketLevelAccess = javaType.uniformBucketLevelAccess(),
url = javaType.url(),
versionings = javaType.versionings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketVersioning.Companion.toKotlin(args0)
})
}),
websites = javaType.websites().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.storage.kotlin.outputs.GetBucketWebsite.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy