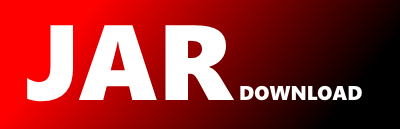
com.pulumi.gcp.tpu.kotlin.NodeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.tpu.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.tpu.NodeArgs.builder
import com.pulumi.gcp.tpu.kotlin.inputs.NodeSchedulingConfigArgs
import com.pulumi.gcp.tpu.kotlin.inputs.NodeSchedulingConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A Cloud TPU instance.
* To get more information about Node, see:
* * [API documentation](https://cloud.google.com/tpu/docs/reference/rest/v1/projects.locations.nodes)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/tpu/docs/)
* ## Example Usage
* ### Tpu Node Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const available = gcp.tpu.getTensorflowVersions({});
* const tpu = new gcp.tpu.Node("tpu", {
* name: "test-tpu",
* zone: "us-central1-b",
* acceleratorType: "v3-8",
* tensorflowVersion: available.then(available => available.versions?.[0]),
* cidrBlock: "10.2.0.0/29",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* available = gcp.tpu.get_tensorflow_versions()
* tpu = gcp.tpu.Node("tpu",
* name="test-tpu",
* zone="us-central1-b",
* accelerator_type="v3-8",
* tensorflow_version=available.versions[0],
* cidr_block="10.2.0.0/29")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var available = Gcp.Tpu.GetTensorflowVersions.Invoke();
* var tpu = new Gcp.Tpu.Node("tpu", new()
* {
* Name = "test-tpu",
* Zone = "us-central1-b",
* AcceleratorType = "v3-8",
* TensorflowVersion = available.Apply(getTensorflowVersionsResult => getTensorflowVersionsResult.Versions[0]),
* CidrBlock = "10.2.0.0/29",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/tpu"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* available, err := tpu.GetTensorflowVersions(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = tpu.NewNode(ctx, "tpu", &tpu.NodeArgs{
* Name: pulumi.String("test-tpu"),
* Zone: pulumi.String("us-central1-b"),
* AcceleratorType: pulumi.String("v3-8"),
* TensorflowVersion: pulumi.String(available.Versions[0]),
* CidrBlock: pulumi.String("10.2.0.0/29"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.tpu.TpuFunctions;
* import com.pulumi.gcp.tpu.inputs.GetTensorflowVersionsArgs;
* import com.pulumi.gcp.tpu.Node;
* import com.pulumi.gcp.tpu.NodeArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var available = TpuFunctions.getTensorflowVersions();
* var tpu = new Node("tpu", NodeArgs.builder()
* .name("test-tpu")
* .zone("us-central1-b")
* .acceleratorType("v3-8")
* .tensorflowVersion(available.applyValue(getTensorflowVersionsResult -> getTensorflowVersionsResult.versions()[0]))
* .cidrBlock("10.2.0.0/29")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* tpu:
* type: gcp:tpu:Node
* properties:
* name: test-tpu
* zone: us-central1-b
* acceleratorType: v3-8
* tensorflowVersion: ${available.versions[0]}
* cidrBlock: 10.2.0.0/29
* variables:
* available:
* fn::invoke:
* Function: gcp:tpu:getTensorflowVersions
* Arguments: {}
* ```
*
* ### Tpu Node Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const available = gcp.tpu.getTensorflowVersions({});
* const network = new gcp.compute.Network("network", {name: "tpu-node-network"});
* const serviceRange = new gcp.compute.GlobalAddress("service_range", {
* name: "my-global-address",
* purpose: "VPC_PEERING",
* addressType: "INTERNAL",
* prefixLength: 16,
* network: network.id,
* });
* const privateServiceConnection = new gcp.servicenetworking.Connection("private_service_connection", {
* network: network.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [serviceRange.name],
* });
* const tpu = new gcp.tpu.Node("tpu", {
* name: "test-tpu",
* zone: "us-central1-b",
* acceleratorType: "v3-8",
* tensorflowVersion: available.then(available => available.versions?.[0]),
* description: "Google Provider test TPU",
* useServiceNetworking: true,
* network: privateServiceConnection.network,
* labels: {
* foo: "bar",
* },
* schedulingConfig: {
* preemptible: true,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* available = gcp.tpu.get_tensorflow_versions()
* network = gcp.compute.Network("network", name="tpu-node-network")
* service_range = gcp.compute.GlobalAddress("service_range",
* name="my-global-address",
* purpose="VPC_PEERING",
* address_type="INTERNAL",
* prefix_length=16,
* network=network.id)
* private_service_connection = gcp.servicenetworking.Connection("private_service_connection",
* network=network.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[service_range.name])
* tpu = gcp.tpu.Node("tpu",
* name="test-tpu",
* zone="us-central1-b",
* accelerator_type="v3-8",
* tensorflow_version=available.versions[0],
* description="Google Provider test TPU",
* use_service_networking=True,
* network=private_service_connection.network,
* labels={
* "foo": "bar",
* },
* scheduling_config=gcp.tpu.NodeSchedulingConfigArgs(
* preemptible=True,
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var available = Gcp.Tpu.GetTensorflowVersions.Invoke();
* var network = new Gcp.Compute.Network("network", new()
* {
* Name = "tpu-node-network",
* });
* var serviceRange = new Gcp.Compute.GlobalAddress("service_range", new()
* {
* Name = "my-global-address",
* Purpose = "VPC_PEERING",
* AddressType = "INTERNAL",
* PrefixLength = 16,
* Network = network.Id,
* });
* var privateServiceConnection = new Gcp.ServiceNetworking.Connection("private_service_connection", new()
* {
* Network = network.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* serviceRange.Name,
* },
* });
* var tpu = new Gcp.Tpu.Node("tpu", new()
* {
* Name = "test-tpu",
* Zone = "us-central1-b",
* AcceleratorType = "v3-8",
* TensorflowVersion = available.Apply(getTensorflowVersionsResult => getTensorflowVersionsResult.Versions[0]),
* Description = "Google Provider test TPU",
* UseServiceNetworking = true,
* Network = privateServiceConnection.Network,
* Labels =
* {
* { "foo", "bar" },
* },
* SchedulingConfig = new Gcp.Tpu.Inputs.NodeSchedulingConfigArgs
* {
* Preemptible = true,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/tpu"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* available, err := tpu.GetTensorflowVersions(ctx, nil, nil)
* if err != nil {
* return err
* }
* network, err := compute.NewNetwork(ctx, "network", &compute.NetworkArgs{
* Name: pulumi.String("tpu-node-network"),
* })
* if err != nil {
* return err
* }
* serviceRange, err := compute.NewGlobalAddress(ctx, "service_range", &compute.GlobalAddressArgs{
* Name: pulumi.String("my-global-address"),
* Purpose: pulumi.String("VPC_PEERING"),
* AddressType: pulumi.String("INTERNAL"),
* PrefixLength: pulumi.Int(16),
* Network: network.ID(),
* })
* if err != nil {
* return err
* }
* privateServiceConnection, err := servicenetworking.NewConnection(ctx, "private_service_connection", &servicenetworking.ConnectionArgs{
* Network: network.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* serviceRange.Name,
* },
* })
* if err != nil {
* return err
* }
* _, err = tpu.NewNode(ctx, "tpu", &tpu.NodeArgs{
* Name: pulumi.String("test-tpu"),
* Zone: pulumi.String("us-central1-b"),
* AcceleratorType: pulumi.String("v3-8"),
* TensorflowVersion: pulumi.String(available.Versions[0]),
* Description: pulumi.String("Google Provider test TPU"),
* UseServiceNetworking: pulumi.Bool(true),
* Network: privateServiceConnection.Network,
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* SchedulingConfig: &tpu.NodeSchedulingConfigArgs{
* Preemptible: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.tpu.TpuFunctions;
* import com.pulumi.gcp.tpu.inputs.GetTensorflowVersionsArgs;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import com.pulumi.gcp.tpu.Node;
* import com.pulumi.gcp.tpu.NodeArgs;
* import com.pulumi.gcp.tpu.inputs.NodeSchedulingConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var available = TpuFunctions.getTensorflowVersions();
* var network = new Network("network", NetworkArgs.builder()
* .name("tpu-node-network")
* .build());
* var serviceRange = new GlobalAddress("serviceRange", GlobalAddressArgs.builder()
* .name("my-global-address")
* .purpose("VPC_PEERING")
* .addressType("INTERNAL")
* .prefixLength(16)
* .network(network.id())
* .build());
* var privateServiceConnection = new Connection("privateServiceConnection", ConnectionArgs.builder()
* .network(network.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(serviceRange.name())
* .build());
* var tpu = new Node("tpu", NodeArgs.builder()
* .name("test-tpu")
* .zone("us-central1-b")
* .acceleratorType("v3-8")
* .tensorflowVersion(available.applyValue(getTensorflowVersionsResult -> getTensorflowVersionsResult.versions()[0]))
* .description("Google Provider test TPU")
* .useServiceNetworking(true)
* .network(privateServiceConnection.network())
* .labels(Map.of("foo", "bar"))
* .schedulingConfig(NodeSchedulingConfigArgs.builder()
* .preemptible(true)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* tpu:
* type: gcp:tpu:Node
* properties:
* name: test-tpu
* zone: us-central1-b
* acceleratorType: v3-8
* tensorflowVersion: ${available.versions[0]}
* description: Google Provider test TPU
* useServiceNetworking: true
* network: ${privateServiceConnection.network}
* labels:
* foo: bar
* schedulingConfig:
* preemptible: true
* network:
* type: gcp:compute:Network
* properties:
* name: tpu-node-network
* serviceRange:
* type: gcp:compute:GlobalAddress
* name: service_range
* properties:
* name: my-global-address
* purpose: VPC_PEERING
* addressType: INTERNAL
* prefixLength: 16
* network: ${network.id}
* privateServiceConnection:
* type: gcp:servicenetworking:Connection
* name: private_service_connection
* properties:
* network: ${network.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${serviceRange.name}
* variables:
* available:
* fn::invoke:
* Function: gcp:tpu:getTensorflowVersions
* Arguments: {}
* ```
*
* ## Import
* Node can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{zone}}/nodes/{{name}}`
* * `{{project}}/{{zone}}/{{name}}`
* * `{{zone}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, Node can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:tpu/node:Node default projects/{{project}}/locations/{{zone}}/nodes/{{name}}
* ```
* ```sh
* $ pulumi import gcp:tpu/node:Node default {{project}}/{{zone}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:tpu/node:Node default {{zone}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:tpu/node:Node default {{name}}
* ```
* @property acceleratorType The type of hardware accelerators associated with this node.
* @property cidrBlock The CIDR block that the TPU node will use when selecting an IP
* address. This CIDR block must be a /29 block; the Compute Engine
* networks API forbids a smaller block, and using a larger block would
* be wasteful (a node can only consume one IP address).
* Errors will occur if the CIDR block has already been used for a
* currently existing TPU node, the CIDR block conflicts with any
* subnetworks in the user's provided network, or the provided network
* is peered with another network that is using that CIDR block.
* @property description The user-supplied description of the TPU. Maximum of 512 characters.
* @property labels Resource labels to represent user provided metadata.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property name The immutable name of the TPU.
* @property network The name of a network to peer the TPU node to. It must be a
* preexisting Compute Engine network inside of the project on which
* this API has been activated. If none is provided, "default" will be
* used.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property schedulingConfig Sets the scheduling options for this TPU instance.
* Structure is documented below.
* @property tensorflowVersion The version of Tensorflow running in the Node.
* - - -
* @property useServiceNetworking Whether the VPC peering for the node is set up through Service Networking API.
* The VPC Peering should be set up before provisioning the node. If this field is set,
* cidr_block field should not be specified. If the network that you want to peer the
* TPU Node to is a Shared VPC network, the node must be created with this this field enabled.
* @property zone The GCP location for the TPU. If it is not provided, the provider zone is used.
*/
public data class NodeArgs(
public val acceleratorType: Output? = null,
public val cidrBlock: Output? = null,
public val description: Output? = null,
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy