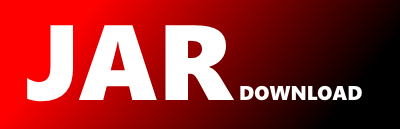
com.pulumi.gcp.vertex.kotlin.AiTensorboard.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.vertex.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.vertex.kotlin.outputs.AiTensorboardEncryptionSpec
import com.pulumi.gcp.vertex.kotlin.outputs.AiTensorboardEncryptionSpec.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [AiTensorboard].
*/
@PulumiTagMarker
public class AiTensorboardResourceBuilder internal constructor() {
public var name: String? = null
public var args: AiTensorboardArgs = AiTensorboardArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AiTensorboardArgsBuilder.() -> Unit) {
val builder = AiTensorboardArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AiTensorboard {
val builtJavaResource = com.pulumi.gcp.vertex.AiTensorboard(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AiTensorboard(builtJavaResource)
}
}
/**
* Tensorboard is a physical database that stores users' training metrics. A default Tensorboard is provided in each region of a GCP project. If needed users can also create extra Tensorboards in their projects.
* To get more information about Tensorboard, see:
* * [API documentation](https://cloud.google.com/vertex-ai/docs/reference/rest/v1/projects.locations.tensorboards)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/vertex-ai/docs)
* ## Example Usage
* ### Vertex Ai Tensorboard
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const tensorboard = new gcp.vertex.AiTensorboard("tensorboard", {
* displayName: "terraform",
* description: "sample description",
* labels: {
* key1: "value1",
* key2: "value2",
* },
* region: "us-central1",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* tensorboard = gcp.vertex.AiTensorboard("tensorboard",
* display_name="terraform",
* description="sample description",
* labels={
* "key1": "value1",
* "key2": "value2",
* },
* region="us-central1")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var tensorboard = new Gcp.Vertex.AiTensorboard("tensorboard", new()
* {
* DisplayName = "terraform",
* Description = "sample description",
* Labels =
* {
* { "key1", "value1" },
* { "key2", "value2" },
* },
* Region = "us-central1",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/vertex"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := vertex.NewAiTensorboard(ctx, "tensorboard", &vertex.AiTensorboardArgs{
* DisplayName: pulumi.String("terraform"),
* Description: pulumi.String("sample description"),
* Labels: pulumi.StringMap{
* "key1": pulumi.String("value1"),
* "key2": pulumi.String("value2"),
* },
* Region: pulumi.String("us-central1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.vertex.AiTensorboard;
* import com.pulumi.gcp.vertex.AiTensorboardArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var tensorboard = new AiTensorboard("tensorboard", AiTensorboardArgs.builder()
* .displayName("terraform")
* .description("sample description")
* .labels(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .region("us-central1")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* tensorboard:
* type: gcp:vertex:AiTensorboard
* properties:
* displayName: terraform
* description: sample description
* labels:
* key1: value1
* key2: value2
* region: us-central1
* ```
*
* ### Vertex Ai Tensorboard Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const tensorboard = new gcp.vertex.AiTensorboard("tensorboard", {
* displayName: "terraform",
* description: "sample description",
* labels: {
* key1: "value1",
* key2: "value2",
* },
* region: "us-central1",
* encryptionSpec: {
* kmsKeyName: "kms-name",
* },
* });
* const project = gcp.organizations.getProject({});
* const cryptoKey = new gcp.kms.CryptoKeyIAMMember("crypto_key", {
* cryptoKeyId: "kms-name",
* role: "roles/cloudkms.cryptoKeyEncrypterDecrypter",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-aiplatform.iam.gserviceaccount.com`),
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* tensorboard = gcp.vertex.AiTensorboard("tensorboard",
* display_name="terraform",
* description="sample description",
* labels={
* "key1": "value1",
* "key2": "value2",
* },
* region="us-central1",
* encryption_spec=gcp.vertex.AiTensorboardEncryptionSpecArgs(
* kms_key_name="kms-name",
* ))
* project = gcp.organizations.get_project()
* crypto_key = gcp.kms.CryptoKeyIAMMember("crypto_key",
* crypto_key_id="kms-name",
* role="roles/cloudkms.cryptoKeyEncrypterDecrypter",
* member=f"serviceAccount:service-{project.number}@gcp-sa-aiplatform.iam.gserviceaccount.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var tensorboard = new Gcp.Vertex.AiTensorboard("tensorboard", new()
* {
* DisplayName = "terraform",
* Description = "sample description",
* Labels =
* {
* { "key1", "value1" },
* { "key2", "value2" },
* },
* Region = "us-central1",
* EncryptionSpec = new Gcp.Vertex.Inputs.AiTensorboardEncryptionSpecArgs
* {
* KmsKeyName = "kms-name",
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var cryptoKey = new Gcp.Kms.CryptoKeyIAMMember("crypto_key", new()
* {
* CryptoKeyId = "kms-name",
* Role = "roles/cloudkms.cryptoKeyEncrypterDecrypter",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-aiplatform.iam.gserviceaccount.com",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/kms"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/vertex"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := vertex.NewAiTensorboard(ctx, "tensorboard", &vertex.AiTensorboardArgs{
* DisplayName: pulumi.String("terraform"),
* Description: pulumi.String("sample description"),
* Labels: pulumi.StringMap{
* "key1": pulumi.String("value1"),
* "key2": pulumi.String("value2"),
* },
* Region: pulumi.String("us-central1"),
* EncryptionSpec: &vertex.AiTensorboardEncryptionSpecArgs{
* KmsKeyName: pulumi.String("kms-name"),
* },
* })
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = kms.NewCryptoKeyIAMMember(ctx, "crypto_key", &kms.CryptoKeyIAMMemberArgs{
* CryptoKeyId: pulumi.String("kms-name"),
* Role: pulumi.String("roles/cloudkms.cryptoKeyEncrypterDecrypter"),
* Member: pulumi.String(fmt.Sprintf("serviceAccount:service-%[email protected]", project.Number)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.vertex.AiTensorboard;
* import com.pulumi.gcp.vertex.AiTensorboardArgs;
* import com.pulumi.gcp.vertex.inputs.AiTensorboardEncryptionSpecArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.kms.CryptoKeyIAMMember;
* import com.pulumi.gcp.kms.CryptoKeyIAMMemberArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var tensorboard = new AiTensorboard("tensorboard", AiTensorboardArgs.builder()
* .displayName("terraform")
* .description("sample description")
* .labels(Map.ofEntries(
* Map.entry("key1", "value1"),
* Map.entry("key2", "value2")
* ))
* .region("us-central1")
* .encryptionSpec(AiTensorboardEncryptionSpecArgs.builder()
* .kmsKeyName("kms-name")
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var cryptoKey = new CryptoKeyIAMMember("cryptoKey", CryptoKeyIAMMemberArgs.builder()
* .cryptoKeyId("kms-name")
* .role("roles/cloudkms.cryptoKeyEncrypterDecrypter")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* tensorboard:
* type: gcp:vertex:AiTensorboard
* properties:
* displayName: terraform
* description: sample description
* labels:
* key1: value1
* key2: value2
* region: us-central1
* encryptionSpec:
* kmsKeyName: kms-name
* cryptoKey:
* type: gcp:kms:CryptoKeyIAMMember
* name: crypto_key
* properties:
* cryptoKeyId: kms-name
* role: roles/cloudkms.cryptoKeyEncrypterDecrypter
* member: serviceAccount:service-${project.number}@gcp-sa-aiplatform.iam.gserviceaccount.com
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* Tensorboard can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{region}}/tensorboards/{{name}}`
* * `{{project}}/{{region}}/{{name}}`
* * `{{region}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, Tensorboard can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:vertex/aiTensorboard:AiTensorboard default projects/{{project}}/locations/{{region}}/tensorboards/{{name}}
* ```
* ```sh
* $ pulumi import gcp:vertex/aiTensorboard:AiTensorboard default {{project}}/{{region}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:vertex/aiTensorboard:AiTensorboard default {{region}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:vertex/aiTensorboard:AiTensorboard default {{name}}
* ```
*/
public class AiTensorboard internal constructor(
override val javaResource: com.pulumi.gcp.vertex.AiTensorboard,
) : KotlinCustomResource(javaResource, AiTensorboardMapper) {
/**
* Consumer project Cloud Storage path prefix used to store blob data, which can either be a bucket or directory. Does not end with a '/'.
*/
public val blobStoragePathPrefix: Output
get() = javaResource.blobStoragePathPrefix().applyValue({ args0 -> args0 })
/**
* The timestamp of when the Tensorboard was created in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* Description of this Tensorboard.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* User provided name of this Tensorboard.
* - - -
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
*/
public val effectiveLabels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy