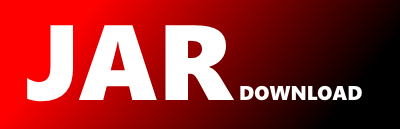
com.pulumi.gcp.vertex.kotlin.outputs.AiEndpointDeployedModel.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.vertex.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property automaticResources (Output)
* A description of resources that to large degree are decided by Vertex AI, and require only a modest additional configuration.
* Structure is documented below.
* @property createTime (Output)
* Output only. Timestamp when the DeployedModel was created.
* @property dedicatedResources (Output)
* A description of resources that are dedicated to the DeployedModel, and that need a higher degree of manual configuration.
* Structure is documented below.
* @property displayName Required. The display name of the Endpoint. The name can be up to 128 characters long and can consist of any UTF-8 characters.
* @property enableAccessLogging (Output)
* These logs are like standard server access logs, containing information like timestamp and latency for each prediction request. Note that Stackdriver logs may incur a cost, especially if your project receives prediction requests at a high queries per second rate (QPS). Estimate your costs before enabling this option.
* @property enableContainerLogging (Output)
* If true, the container of the DeployedModel instances will send `stderr` and `stdout` streams to Stackdriver Logging. Only supported for custom-trained Models and AutoML Tabular Models.
* @property id (Output)
* The ID of the DeployedModel. If not provided upon deployment, Vertex AI will generate a value for this ID. This value should be 1-10 characters, and valid characters are /[0-9]/.
* @property model (Output)
* The name of the Model that this is the deployment of. Note that the Model may be in a different location than the DeployedModel's Endpoint.
* @property modelVersionId (Output)
* Output only. The version ID of the model that is deployed.
* @property privateEndpoints (Output)
* Output only. Provide paths for users to send predict/explain/health requests directly to the deployed model services running on Cloud via private services access. This field is populated if network is configured.
* Structure is documented below.
* @property serviceAccount (Output)
* The service account that the DeployedModel's container runs as. Specify the email address of the service account. If this service account is not specified, the container runs as a service account that doesn't have access to the resource project. Users deploying the Model must have the `iam.serviceAccounts.actAs` permission on this service account.
* @property sharedResources (Output)
* The resource name of the shared DeploymentResourcePool to deploy on. Format: projects/{project}/locations/{location}/deploymentResourcePools/{deployment_resource_pool}
*/
public data class AiEndpointDeployedModel(
public val automaticResources: List? = null,
public val createTime: String? = null,
public val dedicatedResources: List? = null,
public val displayName: String? = null,
public val enableAccessLogging: Boolean? = null,
public val enableContainerLogging: Boolean? = null,
public val id: String? = null,
public val model: String? = null,
public val modelVersionId: String? = null,
public val privateEndpoints: List? = null,
public val serviceAccount: String? = null,
public val sharedResources: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.vertex.outputs.AiEndpointDeployedModel): AiEndpointDeployedModel = AiEndpointDeployedModel(
automaticResources = javaType.automaticResources().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.vertex.kotlin.outputs.AiEndpointDeployedModelAutomaticResource.Companion.toKotlin(args0)
})
}),
createTime = javaType.createTime().map({ args0 -> args0 }).orElse(null),
dedicatedResources = javaType.dedicatedResources().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.vertex.kotlin.outputs.AiEndpointDeployedModelDedicatedResource.Companion.toKotlin(args0)
})
}),
displayName = javaType.displayName().map({ args0 -> args0 }).orElse(null),
enableAccessLogging = javaType.enableAccessLogging().map({ args0 -> args0 }).orElse(null),
enableContainerLogging = javaType.enableContainerLogging().map({ args0 -> args0 }).orElse(null),
id = javaType.id().map({ args0 -> args0 }).orElse(null),
model = javaType.model().map({ args0 -> args0 }).orElse(null),
modelVersionId = javaType.modelVersionId().map({ args0 -> args0 }).orElse(null),
privateEndpoints = javaType.privateEndpoints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.vertex.kotlin.outputs.AiEndpointDeployedModelPrivateEndpoint.Companion.toKotlin(args0)
})
}),
serviceAccount = javaType.serviceAccount().map({ args0 -> args0 }).orElse(null),
sharedResources = javaType.sharedResources().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy