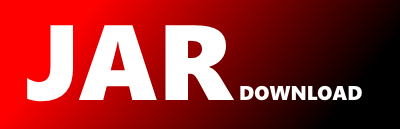
com.pulumi.gcp.workbench.kotlin.inputs.InstanceGceSetupDataDisksArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.workbench.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.workbench.inputs.InstanceGceSetupDataDisksArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property diskEncryption Optional. Input only. Disk encryption method used on the boot
* and data disks, defaults to GMEK.
* Possible values are: `GMEK`, `CMEK`.
* @property diskSizeGb Optional. The size of the disk in GB attached to this VM instance,
* up to a maximum of 64000 GB (64 TB). If not specified, this defaults to
* 100.
* @property diskType Optional. Input only. Indicates the type of the disk.
* Possible values are: `PD_STANDARD`, `PD_SSD`, `PD_BALANCED`, `PD_EXTREME`.
* @property kmsKey 'Optional. The KMS key used to encrypt the disks,
* only applicable if disk_encryption is CMEK. Format: `projects/{project_id}/locations/{location}/keyRings/{key_ring_id}/cryptoKeys/{key_id}`
* Learn more about using your own encryption keys.'
*/
public data class InstanceGceSetupDataDisksArgs(
public val diskEncryption: Output? = null,
public val diskSizeGb: Output? = null,
public val diskType: Output? = null,
public val kmsKey: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.workbench.inputs.InstanceGceSetupDataDisksArgs =
com.pulumi.gcp.workbench.inputs.InstanceGceSetupDataDisksArgs.builder()
.diskEncryption(diskEncryption?.applyValue({ args0 -> args0 }))
.diskSizeGb(diskSizeGb?.applyValue({ args0 -> args0 }))
.diskType(diskType?.applyValue({ args0 -> args0 }))
.kmsKey(kmsKey?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InstanceGceSetupDataDisksArgs].
*/
@PulumiTagMarker
public class InstanceGceSetupDataDisksArgsBuilder internal constructor() {
private var diskEncryption: Output? = null
private var diskSizeGb: Output? = null
private var diskType: Output? = null
private var kmsKey: Output? = null
/**
* @param value Optional. Input only. Disk encryption method used on the boot
* and data disks, defaults to GMEK.
* Possible values are: `GMEK`, `CMEK`.
*/
@JvmName("bnhicpyoeejbicfn")
public suspend fun diskEncryption(`value`: Output) {
this.diskEncryption = value
}
/**
* @param value Optional. The size of the disk in GB attached to this VM instance,
* up to a maximum of 64000 GB (64 TB). If not specified, this defaults to
* 100.
*/
@JvmName("cugkefbisqepuvna")
public suspend fun diskSizeGb(`value`: Output) {
this.diskSizeGb = value
}
/**
* @param value Optional. Input only. Indicates the type of the disk.
* Possible values are: `PD_STANDARD`, `PD_SSD`, `PD_BALANCED`, `PD_EXTREME`.
*/
@JvmName("rwrxavirkdrniqyy")
public suspend fun diskType(`value`: Output) {
this.diskType = value
}
/**
* @param value 'Optional. The KMS key used to encrypt the disks,
* only applicable if disk_encryption is CMEK. Format: `projects/{project_id}/locations/{location}/keyRings/{key_ring_id}/cryptoKeys/{key_id}`
* Learn more about using your own encryption keys.'
*/
@JvmName("ufluegjbwkfoooki")
public suspend fun kmsKey(`value`: Output) {
this.kmsKey = value
}
/**
* @param value Optional. Input only. Disk encryption method used on the boot
* and data disks, defaults to GMEK.
* Possible values are: `GMEK`, `CMEK`.
*/
@JvmName("qkkcdufqmuaxqgdi")
public suspend fun diskEncryption(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskEncryption = mapped
}
/**
* @param value Optional. The size of the disk in GB attached to this VM instance,
* up to a maximum of 64000 GB (64 TB). If not specified, this defaults to
* 100.
*/
@JvmName("tdyinxipxgrcnsug")
public suspend fun diskSizeGb(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskSizeGb = mapped
}
/**
* @param value Optional. Input only. Indicates the type of the disk.
* Possible values are: `PD_STANDARD`, `PD_SSD`, `PD_BALANCED`, `PD_EXTREME`.
*/
@JvmName("mghitgpfphfxvysj")
public suspend fun diskType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskType = mapped
}
/**
* @param value 'Optional. The KMS key used to encrypt the disks,
* only applicable if disk_encryption is CMEK. Format: `projects/{project_id}/locations/{location}/keyRings/{key_ring_id}/cryptoKeys/{key_id}`
* Learn more about using your own encryption keys.'
*/
@JvmName("ycdeaitwrnxoosoc")
public suspend fun kmsKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKey = mapped
}
internal fun build(): InstanceGceSetupDataDisksArgs = InstanceGceSetupDataDisksArgs(
diskEncryption = diskEncryption,
diskSizeGb = diskSizeGb,
diskType = diskType,
kmsKey = kmsKey,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy